Dodge the Cache: Mastering Maven in Docker Builds
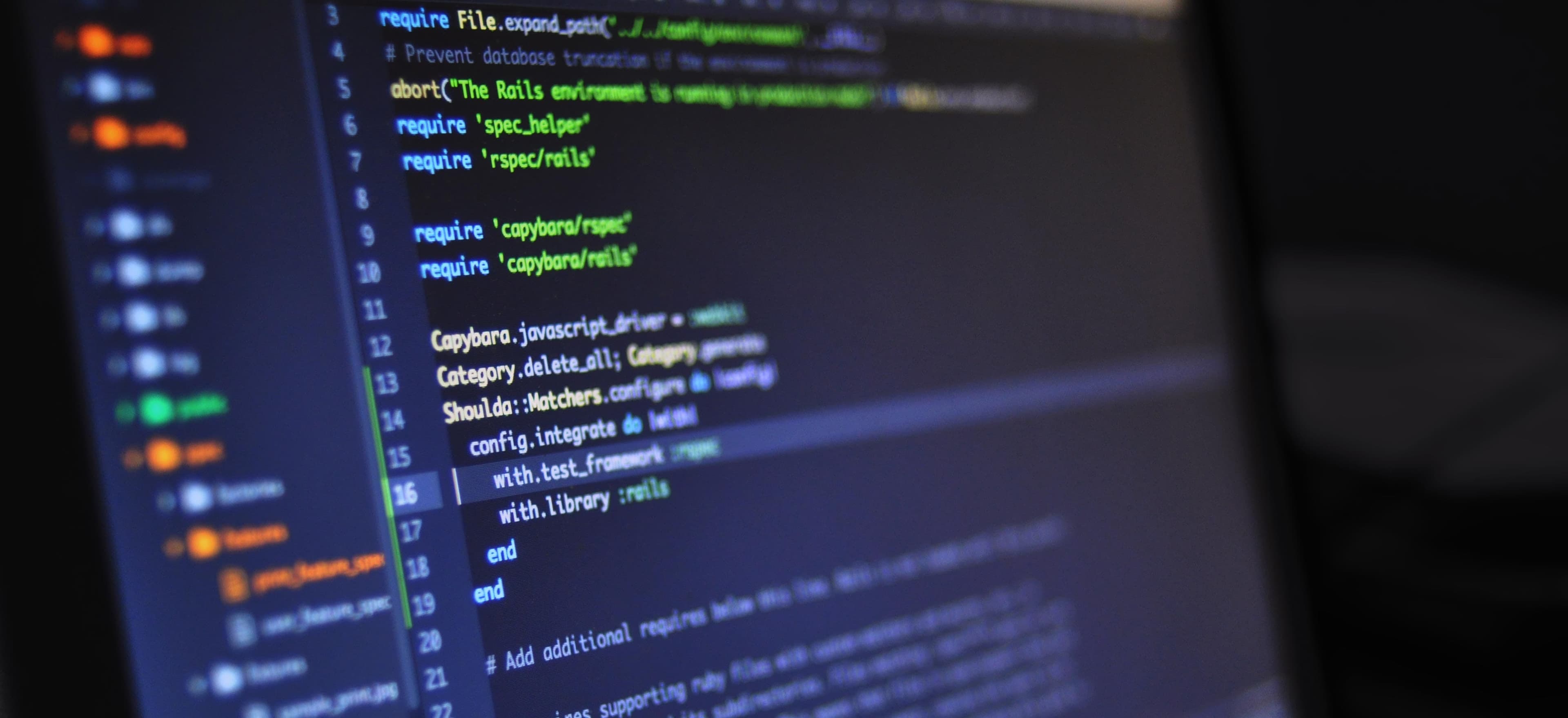
- Published on
Dodge the Cache: Mastering Maven in Docker Builds
In the contemporary flux of software development, speed and efficiency are the beating hearts that keep the system alive. Maven, a powerhouse of a build tool, orchestrates the Java world's rhythms. But when Maven meets Docker, the dance can either be a seamless waltz or a clumsy tango. It all comes down to caching: a hidden maestro that can make your builds swift or painfully slow.
Let’s groove through how to optimize the use of Maven within Docker, ensuring swift builds through savvy caching strategies.
The Maven Whisperer: Understanding Maven Dependencies
Maven operates on the principle of convention-over-configuration, meaning it assumes a lot about your project so you don't have to micromanage its build process. It handles dependency resolution, compilation, packaging, and even deployment – all through what might seem like an arcane pom.xml
script to the uninitiated.
Maven Caching: A Double-Edged Sword
Maven's dependency management can be a blessing and a curse. It simplifies complex builds by effortlessly fetching all your project needs. However, in the context of Docker, Maven's default behavior – which sprawls dependencies across your filesystem – can negate Docker's caching advantage.
<!-- A snippet from a typical Maven pom.xml showing dependency management -->
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- ... other dependencies ... -->
</dependencies>
With each build, Maven will seek out and download the dependencies listed, unless they've been cached. Hence the need for a strategy.
Docker Caches and How Maven Fits In
Docker employs a layer-based caching mechanism. When building an image, if a layer's content hasn't changed since the last build, Docker will use the previous layer from the cache. This mechanism is what makes Docker build efficiently, if utilized correctly.
A common Docker build problem with Maven is that the cache benefits can be thwarted due to the nature of dependency changes or even minor code changes. Thus, achieving an optimal arrangement necessitates savvy configuration.
Building a Java Maven project with Dockerfile
Here is an example of a Dockerfile used to build a Java Maven project. Note how it is optimized for caching.
# Start with a base image containing Java runtime and Maven
FROM maven:3.8.1-jdk-11 as build
# 1) Optimize dependency caching by first copying the POM file
COPY pom.xml /usr/src/myapp/
WORKDIR /usr/src/myapp
# 2) Download the dependencies - this layer will be cached unless the POM changes
RUN mvn dependency:go-offline -B
# 3) Now copy the rest of the project - any changes here will not invalidate the above layer
COPY src /usr/src/myapp/src
# 4) Build the project - since dependencies are cached, this is all about your code
RUN mvn clean package -DskipTests
# Use OpenJDK for lighter snapshot
FROM openjdk:11-jre-slim
# 5) Copy over the built artifact from the Maven container
COPY --from=build /usr/src/myapp/target/myapp-1.0.jar /usr/app/myapp-1.0.jar
WORKDIR /usr/app
CMD ["java", "-jar", "myapp-1.0.jar"]
In this Dockerfile, we've clearly separated dependency resolution from the compilation of our project's code. This ensures that a change in the source code doesn't prompt a re-download of all dependencies, thereby saving time and bandwidth.
Advanced Moves: Multistage Builds for Additional Optimization
There's a fancier step you can take: multistage builds. This innovative Docker feature allows you to create temporary images for compiling or setting up your application, after which you can copy only the essential artifacts to the final image. The benefits are twofold: smaller final images and an additional layer of cache optimization.
A Maven Multistage Dockerfile Example
Let's look at a more advanced Dockerfile using multistage builds.
# Build stage: Here, we are only concerned about building the app and not running it.
FROM maven:3.8.1-jdk-11 as build
COPY pom.xml /build/
COPY src /build/src/
WORKDIR /build/
RUN mvn package -DskipTests
# Package stage: Here, we are creating a slim image containing only the necessary runtime and our compiled jar.
FROM openjdk:11-jre-slim
COPY --from=build /build/target/*.jar /app/myapp.jar
WORKDIR /app
CMD ["java", "-jar", "myapp.jar"]
With this approach, all the cruft left over from compiling the Java application doesn't find its way into the final image, ensuring a lighter, more secure footprint.
Benchmarking Your Success
How do you know if your cache-fu is strong? Benchmarking before and after applying these optimization tricks can reveal the performance gains. Look for improvements in build time, but also consider the consistency of those times for repeated builds and the size of the resultant Docker images.
The Maintenance Tango: Keep Dancing with Updates
Remember, dependency management isn't a set-it-and-forget-it affair. You'll still need to periodically invalidate the Maven cache to update dependencies to their latest version, ensuring security and performance improvements are integrated into your builds. Handle with care, though; an updated dependency might introduce breaking changes.
Maintenance commands:
# To update the project dependencies forcefully
mvn versions:use-latest-versions
# To clean the local repository of downloaded artifacts
mvn dependency:purge-local-repository
Watch for those pom.xml
updates, and when they occur, let the cache be invalidated and the fresh dependencies pour in.
Wrapping Up: The Grand Finale
No longer must Maven and Docker tread on each other's toes. The synergy of decisive Dockerfile crafting and proper Maven management can create a ballet of builds that are both speedy and robust. Just remember: the key is in appreciating how Docker caching works and then exploiting it to its maximum with Maven's dependency resolution strategies.
Leverage the power of caching, strike a balance with updates, and your Java builds will be as graceful and efficient as a swan across the lake. Happy building!
Further Reading on Maven Best Practices Deep Dive into Docker Layer Caching The Fundamentals of Multistage Builds