Tackling Concurrency: From Java 7 Futures to Akka
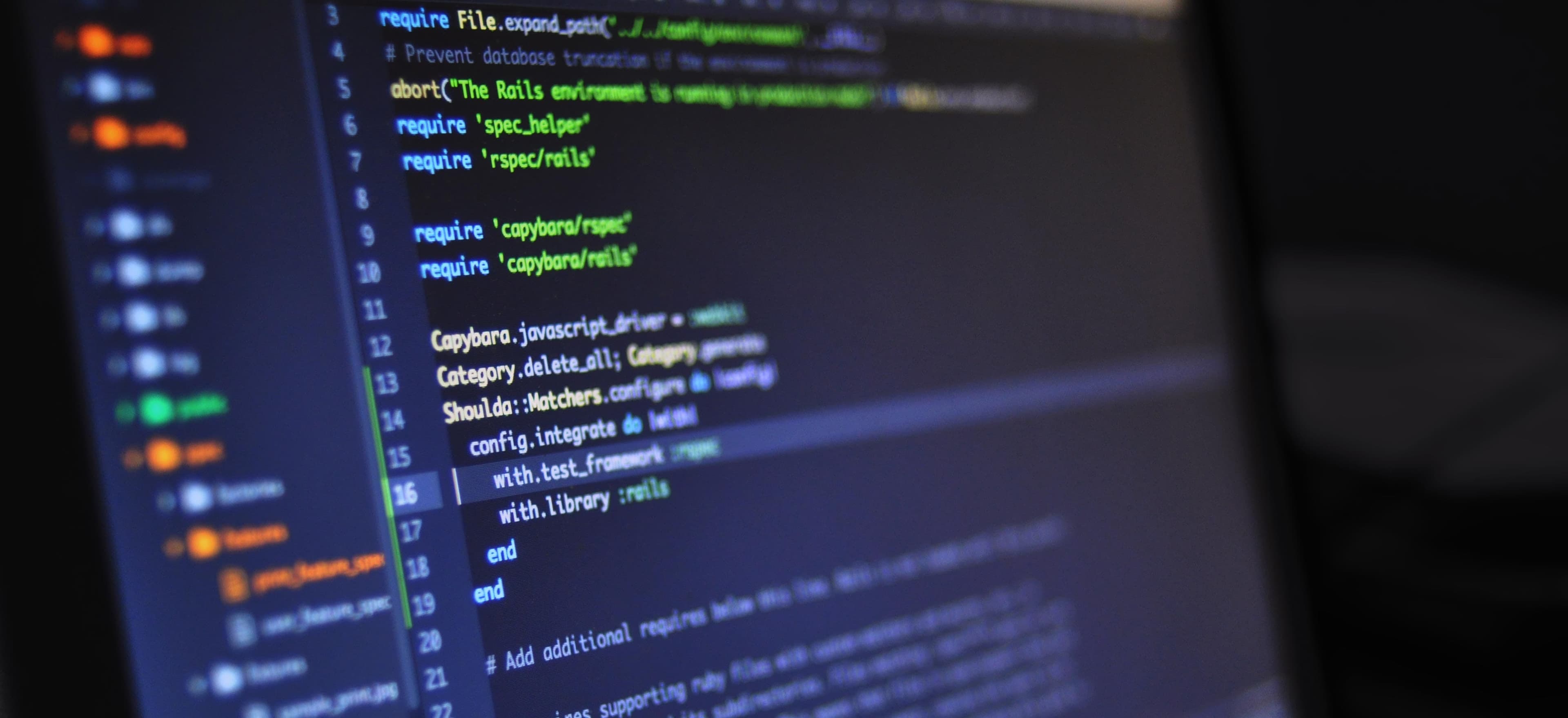
- Published on
Tackling Concurrency: From Java 7 Futures to Akka
In the realm of multi-threaded programming, Java has a storied history of providing robust solutions. Over time, these solutions have evolved, allowing developers to write safer, more scalable concurrent applications. From Java 7 Futures to sophisticated libraries like Akka, this post dives into the mechanisms Java offers to handle concurrency, each with its own set of advantages.
Understanding Java 7 Futures
First introduced in Java 5, and refined in subsequent versions, with Java 7 bringing in new helpful features, java.util.concurrent.Future
represents the result of an asynchronous computation - a pattern that has been a game-changer for Java developers dealing with concurrent programming.
Why use Futures?
When you have tasks that are independent of one another, being able to run them concurrently can improve the performance of your application significantly. This could involve fetching data from a database, calling a remote service, or any I/O operation.
Futures allow you to kick off a task and continue doing other work while you wait for the result. Instead of your thread being blocked, it can get on with other tasks, leading to better resource utilization and a snappier application.
A Basic Future Example
Here's an example to illustrate a simple use case for Future
in Java:
// Example with Java 7 Futures
import java.util.concurrent.*;
public class FutureExample {
private static final ExecutorService executorService = Executors.newCachedThreadPool();
public static void main(String[] args) {
Future<String> future = executorService.submit(new Callable<String>() {
@Override
public String call() throws Exception {
// Simulate a long-running task
Thread.sleep(2000);
return "Result from the future";
}
});
// Do something else while the future is being computed
doSomethingElse();
try {
// Retrieve the result of the Future, with a timeout
String result = future.get(3, TimeUnit.SECONDS);
System.out.println(result);
} catch (InterruptedException | ExecutionException | TimeoutException e) {
e.printStackTrace();
} finally {
// Don't forget to shutdown the executor
executorService.shutdown();
}
}
private static void doSomethingElse() {
// Other operations can be performed here
System.out.println("Doing something else...");
}
}
In this example, the Future
object is used to handle a potentially long-running task, freeing up the main thread to perform other operations. This snippet exemplifies the 'why' aspect of using Future
: it provides a placeholder for the result of the asynchronous operation and allows for non-blocking operations and resource efficiency.
Moving Beyond Java 7: The CompletableFuture
Come Java 8, and developers were introduced to CompletableFuture
, an enhancement to Future that allows for a more streamlined and functional approach to handling concurrency. This allows for chaining asynchronous operations together, combining them, or handling exceptions without the boilerplate code associated with plain old Future
objects.
CompletableFuture in Action
Using CompletableFuture
, the previous example becomes more concise and flexible:
import java.util.concurrent.*;
public class CompletableFutureExample {
// An Executor is not always necessary but recommended for custom thread management
private static final ExecutorService executorService = Executors.newFixedThreadPool(10);
public static void main(String[] args) {
CompletableFuture<String> completableFuture = CompletableFuture.supplyAsync(() -> {
try {
// Simulate a long-running task
Thread.sleep(2000);
} catch (InterruptedException e) {
throw new IllegalStateException(e);
}
return "Result from CompletableFuture";
}, executorService);
// Async operations can be chained
completableFuture.thenAccept(result -> System.out.println(result));
// Perform other tasks in parallel
doSomethingElse();
// Block and get the result of the computation synchronously if necessary
try {
String result = completableFuture.get(3, TimeUnit.SECONDS);
System.out.println(result);
} catch (InterruptedException | ExecutionException | TimeoutException e) {
e.printStackTrace();
} finally {
executorService.shutdown();
}
}
private static void doSomethingElse() {
// Perform other operations while the future completes
System.out.println("Working on something else...");
}
}
CompletableFuture
offers methods such as thenApply
, thenAccept
, and exceptionally
, enabling you to compose asynchronous logic similar to JavaScript Promises. This functional approach to concurrency encourages flatter, more readable code which is easier to reason about and maintain.
The Akka Framework for Concurrent Applications
For even more control and scalability, one can turn to the Akka toolkit. Akka uses the Actor Model to offer a higher level of abstraction for writing concurrent and distributed systems.
Why Akka?
The Actor Model encapsulates state and behavior into individual actor instances, which communicate with each other using messages. This avoids shared state and the need for synchronization which can lead to deadlocks or race conditions.
Akka actors can be distributed across a cluster of machines, making it suitable for building high-performance, scalable applications that can handle massive amounts of transactions. Plus, Akka provides a neat way to recover from failures in a granular and controlled manner.
Akka in Practice
Here's a rudimentary example of using Akka to create a simple actor that greets whoever sends it a message:
// Akka example (Scala code for brevity and clarity)
import akka.actor.Actor
import akka.actor.ActorSystem
import akka.actor.Props
// Define the Greet message
case class Greet(name: String)
// Define the Greeter actor
class GreeterActor extends Actor {
def receive = {
case Greet(name) => println(s"Hello, $name!")
}
}
object AkkaExample {
def main(args: Array[String]): Unit = {
// Create the actor system
val system = ActorSystem("HelloSystem")
// Create the 'greeter' actor
val greeter = system.actorOf(Props[GreeterActor], name = "greeter")
// Send a Greet message to the 'greeter' actor
greeter ! Greet("World")
// Remember to shutdown the system
system.terminate()
}
}
In the spirit of this post, use Akka when you need a robust framework for building concurrent and distributed systems. Its actor-based concurrency model, supervision strategies, and ease of scalability make it a fine choice for complex applications.
Conclusion
Concurrency is a vital aspect of modern software development, and Java has significantly improved its concurrency toolkit over time. Starting from Future
in Java 7 to CompletableFuture
in Java 8, and moving towards actor-based concurrency models like Akka, developers are equipped with a growing arsenal of tools to write safe, concurrent applications that perform at scale.
While Future
provides a solid foundation and CompletableFuture
brings functional-style composition to asynchronous operations, Akka presents an even more robust solution with its Actor Model, especially suitable for highly concurrent, distributed systems.
To delve deeper into these topics, consider exploring the official Java Concurrency tutorial, the CompletableFuture documentation, and the Akka documentation. Choose the right tool for your concurrency needs, and your Java applications will be well on their way to efficiently handling multiple tasks at once.