Escaping Deadlocks: Unlocking Concurrency Woes!
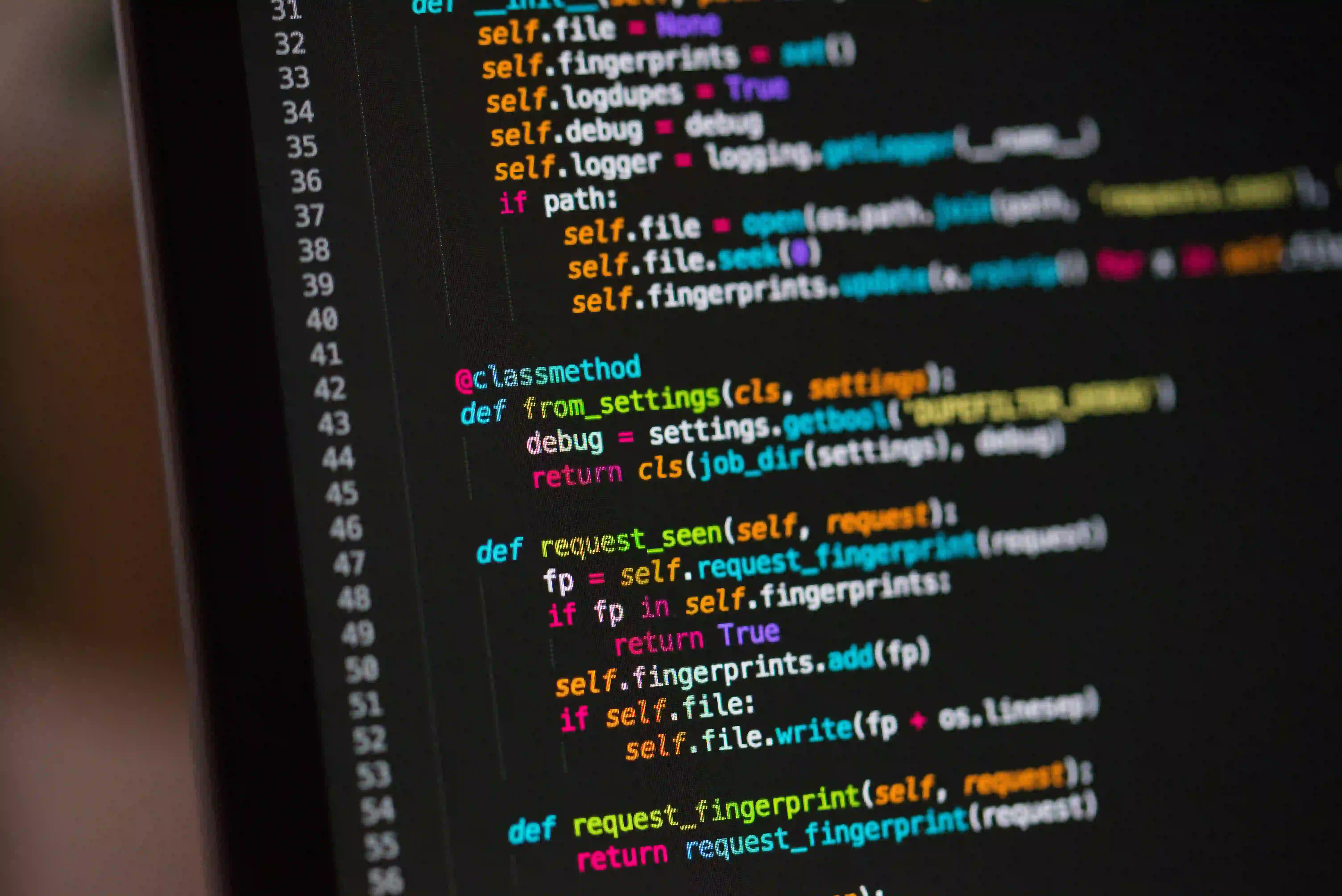
Escaping Deadlocks: Unlocking Concurrency Woes in Java
In the modern era of software development, concurrency is the backbone that enables applications to perform efficiently and handle multiple tasks simultaneously. However, with the power of concurrency in Java comes the great responsibility of managing it correctly. One of the most notorious bugs that can bring your multithreaded application to its knees is the deadlock. In this post, we delve deep into avoiding and resolving deadlocks to ensure that your Java application remains responsive and robust.
What is a Deadlock?
A deadlock in Java occurs when two or more threads are blocked forever, each waiting for the other to release a lock. Picture a standstill situation where thread A holds lock 1 and waits for lock 2 while thread B holds lock 2 and waits for lock 1 – neither can proceed, thus locking the application in a state of perpetual waiting.
Identifying Deadlock Conditions
To effectively escape deadlocks, it's crucial to understand the Coffman conditions which characterize a deadlock situation:
- Mutual Exclusion: At least one resource must be held in a non-shareable mode.
- Hold and Wait: A thread must be holding at least one resource and waiting to acquire additional resources.
- No Preemption: Resources cannot be preempted and must be released voluntarily by the thread holding them.
- Circular Wait: There must be a circular chain of two or more threads, each of which is waiting for a resource held by the next member in the chain.
Let's move onto some practical strategies to prevent and recover from deadlocks in Java.
Deadlock Prevention Strategies
1. Lock Ordering
One way to steer clear of deadlocks is to impose a strict order in which locks must be acquired. If all threads acquire locks in a consistent global order, the circular wait condition is effectively broken.
// Example of a well-ordered lock acquisition
class Account {
private int balance;
private final Object lock = new Object();
public void transfer(Account to, int amount) {
Object firstLock = this.lock.hashCode() < to.lock.hashCode() ? this.lock : to.lock;
Object secondLock = this.lock.hashCode() < to.lock.hashCode() ? to.lock : this.lock;
synchronized (firstLock) {
synchronized (secondLock) {
this.balance -= amount;
to.balance += amount;
}
}
}
}
Here, the locks are ordered based on their hash codes to decide which lock to acquire first. This simple rule eliminates the possibility of a circular wait.
2. Lock Timeout
Another technique to avoid deadlocks is to introduce timeouts while trying to acquire locks. By using try-lock with a timeout, a thread can back off and retry if it cannot get all required locks within a given time.
// Example of lock timeout using ReentrantLock
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
import java.util.concurrent.TimeUnit;
class Resource {
private final Lock lock = new ReentrantLock();
public boolean safeMethod() throws InterruptedException {
if (lock.tryLock(1000, TimeUnit.MILLISECONDS)) {
try {
// Critical section
} finally {
lock.unlock();
}
return true;
} else {
// Could not acquire the lock
return false;
}
}
}
The tryLock
with a timeout prevents the thread from waiting indefinitely for the lock and allows it to either retry after some time or take alternative actions.
3. Deadlock Detection
Sometimes preventing deadlock may not be feasible, and in such cases, detecting and recovering from deadlocks becomes essential. Java provides tools like JConsole and VisualVM that can help detect deadlocks in running applications.
4. Avoid Nested Locks
Avoiding nested locks can greatly reduce the risk of deadlock. Try to minimize the scope of synchronized blocks and avoid calling a method that acquires another lock from within a synchronized block.
class AvoidNestedLocks {
private final Object lock1 = new Object();
private final Object lock2 = new Object();
public void method1() {
synchronized (lock1) {
// Critical section
}
}
public void method2() {
synchronized (lock2) {
// Critical section
}
}
}
Each method locks independently, avoiding nested lock acquisition, thereby reducing deadlock risk.
Deadlock Resolution
Even with all precautions, if a deadlock situation does happen, it's not the end of the world. Recovery is possible by identifying the threads involved in the deadlock and resources they're waiting for, usually through logging or using JVM tools. After identifying the deadlock, you can interrupt one or more of the threads involved or restart the application, though these are last-resort options.
Conclusion
Deadlocks can be complicated and tricky, but with careful design, they are preventable and manageable. By adhering to strategies such as lock ordering, using lock timeouts, and avoiding nested locks, you can keep your Java applications free of these concurrency traps. Remember to test thoroughly, as deadlocks can be elusive and might show up only under specific timing conditions.
Explore the Java Concurrency API to deepen your understanding and toolset for writing concurrent applications. Your journey to mastering concurrency is not just about avoiding deadlocks but also about writing efficient and scalable Java code. Keep learning, keep coding, and unlock the full potential of concurrency in your Java applications!