Boost Throughput: Solving Messaging Bottlenecks
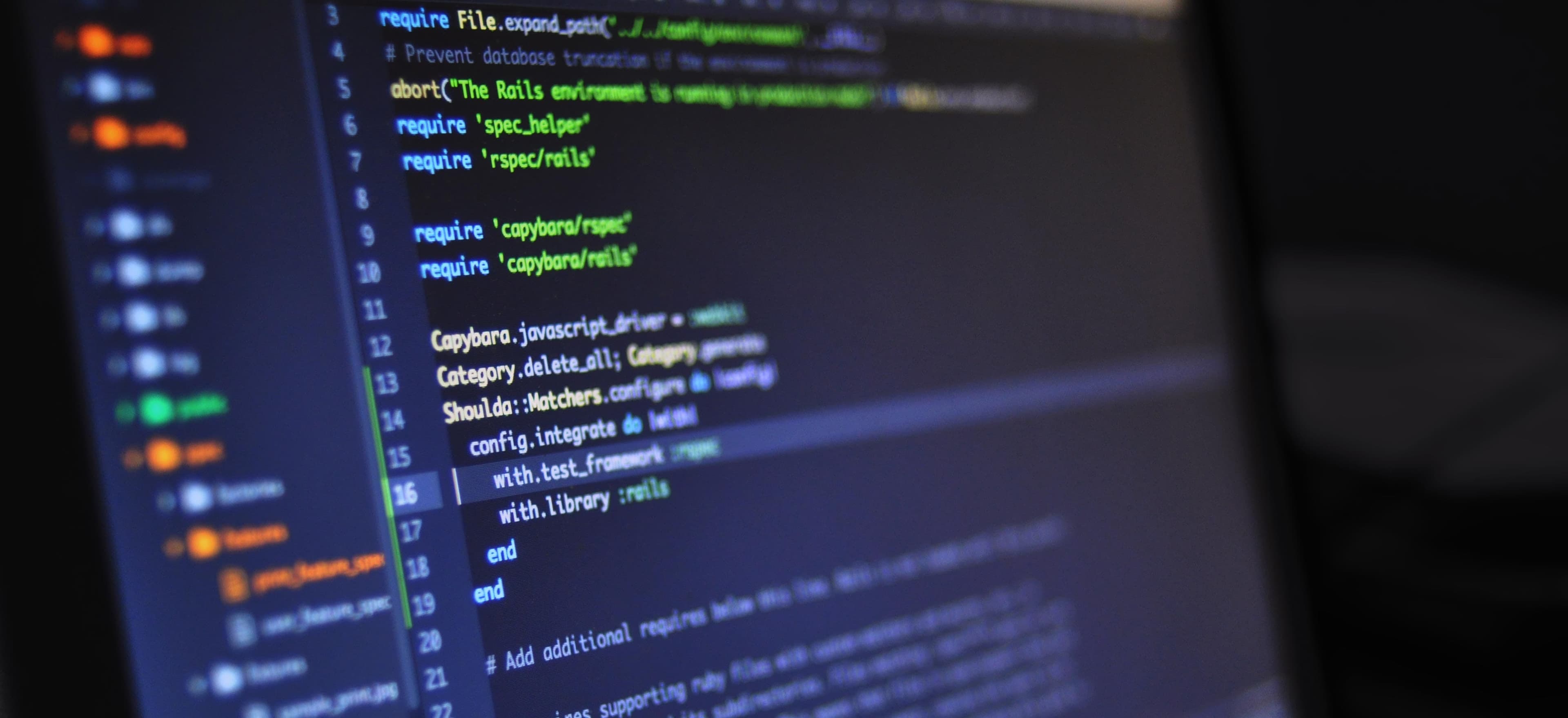
- Published on
Boost Throughput: Solving Messaging Bottlenecks in Java Applications
Messaging systems are the backbone of modern distributed Java applications, providing critical infrastructure for asynchronous communication and data processing. However, high load can create bottlenecks, adversely impacting throughput and overall performance. How do you keep your system running smoothly under pressure?
This in-depth blog post explores practical strategies to alleviate messaging bottlenecks in Java applications. By implementing the right techniques and utilizing robust Java libraries, you can ensure your messaging system runs efficiently, even as demand escalates.
Understanding the Problem
Before we dive into solutions, it's paramount to grasp the root causes of messaging bottlenecks. In Java applications, such bottlenecks can arise due to:
- Inefficient resource usage (like CPU, memory, I/O)
- Poorly tuned messaging infrastructure
- Unoptimized message processing code
To address these issues, we'll cover various optimization strategies that can substantially increase throughput.
Strategies to Optimize Messaging Throughput
1. Parallel Processing
Concurrency is a powerful tool in Java for increasing throughput. Java's concurrent utilities like Executors
and CompletableFuture
allow for efficient parallel processing of messages.
ExecutorService executorService = Executors.newFixedThreadPool(10);
for (Message message : messages) {
executorService.submit(() -> processMessage(message));
}
private void processMessage(Message message) {
// Message processing logic
}
Why this works: A fixed thread pool facilitates processing multiple messages concurrently, thus leveraging multicore processors to boost throughput.
2. Batch Processing
Instead of processing one message at a time, it can be more efficient to process groups of messages.
public void processMessages(List<Message> messages) {
for (Message message : messages) {
// Process each message
}
// Acknowledge all messages at once
}
Why this works: Reducing overhead by batching can drastically decrease processing time and resource consumption, enhancing throughput.
3. Tune Messaging Infrastructure
Configuring your messaging system correctly can make a world of difference. Many messaging middleware like Kafka or RabbitMQ have settings for batch size, commit interval, consumer threads, and prefetch counts that should be tuned.
Why this works: Proper configuration ensures the messaging infrastructure can handle high loads effectively and avoids becoming the bottleneck itself.
4. Use Non-Blocking I/O
When dealing with I/O operations, using non-blocking APIs like Java NIO can improve performance significantly.
AsynchronousFileChannel fileChannel = AsynchronousFileChannel.open(path, StandardOpenOption.READ);
ByteBuffer buffer = ByteBuffer.allocateDirect(1024);
fileChannel.read(buffer, 0, buffer, new CompletionHandler<Integer, ByteBuffer>() {
@Override
public void completed(Integer result, ByteBuffer attachment) {
// Handle the result of the read operation
}
@Override
public void failed(Throwable exc, ByteBuffer attachment) {
// Handle the read operation failure
}
});
Why this works: By avoiding blocking, your threads can do meaningful work instead of idly waiting for I/O operations.
5. Optimize Message Payloads
Reducing the size of the messages being transmitted by using efficient serialization protocols such as Protocol Buffers or Avro can also lead to higher throughput.
Why this works: Smaller messages mean less data to transfer and serialize/deserialize, leading to lower latency and higher throughput.
6. Use Caching
Caching frequently accessed data can significantly reduce the need for costly operations like database queries or file I/O, which can slow down message processing.
Why this works: Accessing in-memory data is orders of magnitude faster than disk or network operations, directly benefiting throughput.
7. Profile and Optimize Code
Using a profiler can help identify hotspots in your code. Once identified, optimizing these can have a dramatic effect on performance.
Why this works: Improving your code efficiency directly translates to better use of resources and faster processing.
Common Pitfalls to Avoid
While optimizing, beware of the following pitfalls:
- Over-optimization: Avoid premature optimization. Understand your bottlenecks before acting.
- Neglecting Hardware Constraints: Optimization is not only about software. Ensure your hardware can support your throughput goals.
- Thread Overhead: While concurrency is beneficial, too many threads can lead to context switching overhead, negating any gains from parallelism.
Monitoring and Testing
Monitoring your messaging system is crucial. Use tools like JMX or Prometheus to watch metrics that matter.
Testing your optimizations is equally important. Employ benchmarking tools such as JMH to quantitatively assess your throughput improvements.
Conclusion
Enhancing messaging throughput in Java applications involves a multi-faceted approach. By implementing concurrent processing, optimizing message payloads, caching, profiling, and correctly tuning your messaging infrastructure, you can effectively improve your system's performance.
Moreover, avoiding common pitfalls, diligent monitoring, and rigorous testing will safeguard your optimizations and provide verifiable results. With these strategies, your Java applications can achieve remarkable throughput levels, ready to handle increasing loads with grace and efficiency.
Remember, optimization is an ongoing journey. Stay updated with Java's latest developments to ensure your messaging systems remain at peak performance.
To keep expanding your Java knowledge and stay abreast of the latest trends and best practices, don't forget to check out key resources like the Oracle Java Documentation and industry blogs. Happy coding!