Mastering Scala: Solving Common XML Parsing Issues
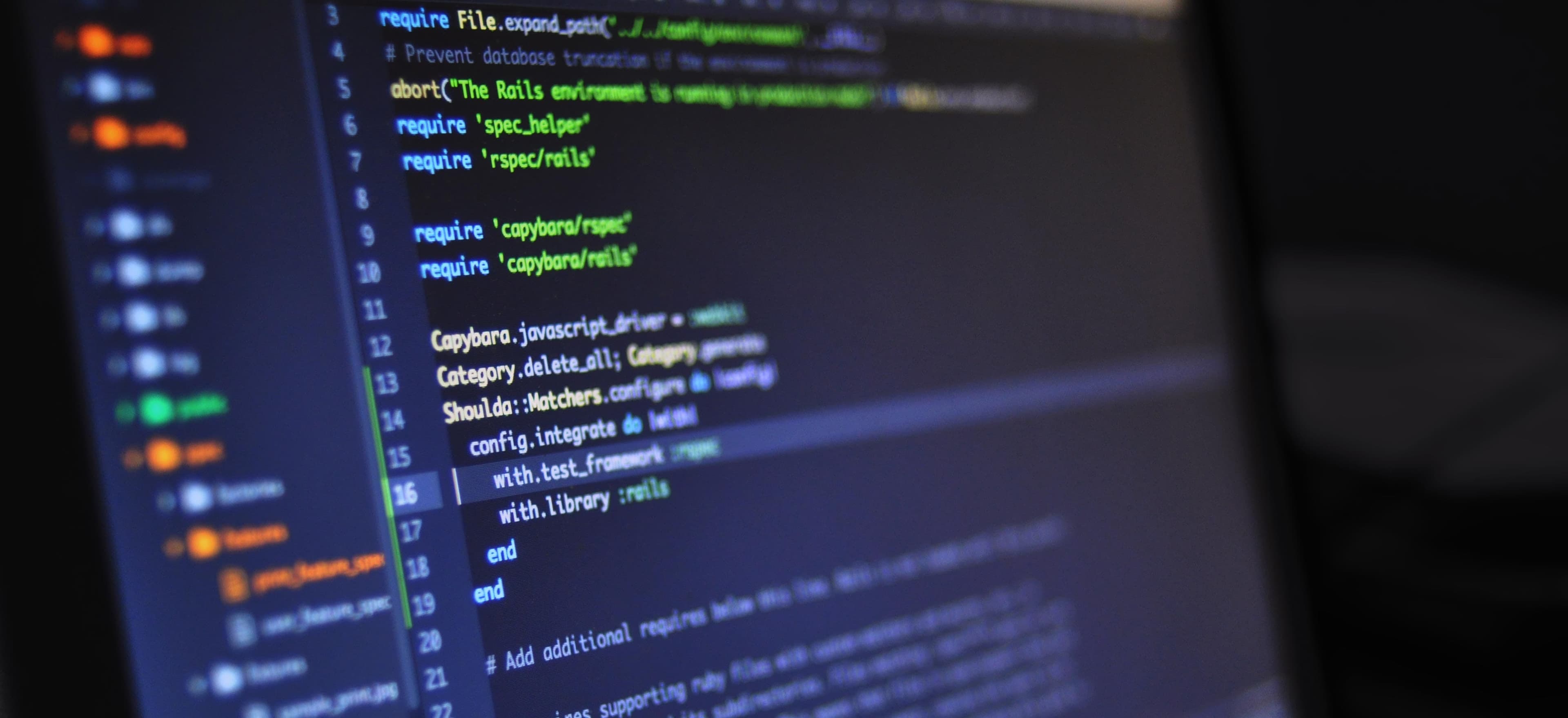
- Published on
Mastering Scala: Solving Common XML Parsing Issues
Working with XML data is a common requirement in many enterprise applications, and Scala, being a functional and object-oriented programming language, provides robust tools for XML parsing. However, dealing with XML can often introduce challenges that developers need to overcome. In this blog post, we'll delve into common XML parsing issues in Scala and offer practical solutions to handle these effectively.
Before we jump into troubleshooting, it's crucial to understand that Scala XML library is not part of the standard library as of Scala 2.13. However, it's still widely used and is available as a separate library that you can pull into your project. Let’s dissect common XML parsing problems and tackle each with a solution to enhance your Scala prowess.
The Setup
Firstly, ensure you’ve included the Scala XML library in your build.sbt if you're working with Scala 2.13 or later:
libraryDependencies += "org.scala-lang.modules" %% "scala-xml" % "1.3.0"
Now, let's start with some common issues and how to resolve them.
1. Handling Large XML Files
One of the first challenges you might encounter is performance issues when parsing large XML files. Loading a massive XML document into memory can lead to OutOfMemoryError
and slow processing.
Solution: Streaming
Use a streaming API like Scala's built-in scala.xml.pull.XMLEventReader
class to handle large XML files efficiently. This approach reads the document piece-by-piece, significantly reducing memory usage.
import scala.xml.pull._
import scala.io.Source
val xml = new XMLEventReader(Source.fromFile("large.xml"))
xml.foreach {
case EvElemStart(_, "element", attrs, _) =>
println(s"Start of element with attrs: $attrs")
case EvElemEnd(_, "element") =>
println("End of element.")
case _ => // Ignore other events
}
Why Streaming?
Streaming ensures you only have the parts of the document you need at any given time in memory, thus preventing out-of-memory issues and increasing performance.
2. Extracting Data with Poor XPath Support
Another common problem is dealing with XML libraries that have limited XPath support, which can make it difficult to query XML documents effectively.
Solution: Pattern Matching
Scala's powerful pattern matching can serve as an alternative to XPath by providing an expressive way to deconstruct and extract data from XML nodes.
val xml = <root><child name="foo">bar</child></root>
xml match {
case <root>{ children @ _* }</root> =>
for (child @ <child>{ contents }</child> <- children) {
println(s"Found child with contents: $contents")
}
}
Why Pattern Matching?
Pattern matching in Scala is declarative and easy to read. It provides a clear and concise way to navigate and extract data from XML nodes, much like XPath but with Scala's language features.
3. Namespace Handling
XML namespaces are another common stumbling block, particularly when working with documents with multiple or nested namespaces.
Solution: Scoped Binding
When parsing and querying XML with namespaces, use Scala's scoped binding feature. You can bind a prefix to a namespace URI and leverage that within your code to handle nodes correctly.
val ns = "http://example.com/ns"
val xml = <root xmlns={ns}><child>content</child></root>
def extractFromScope(node: scala.xml.NodeSeq): Seq[scala.xml.Node] = {
node.scope.getPrefix(ns) match {
case null => Seq.empty
case prefix =>
node \ prefix \ "child"
}
}
println(extractFromScope(xml))
Why Scoped Binding?
Scoped binding keeps your code clean when dealing with namespaces, significantly reducing the complexity of namespace handling in XML documents.
4. Dealing with Optional Elements
In XML, not all elements are guaranteed to be present, leading to issues when you assume all parts of the data structure are filled.
Solution: Option Type
The Option
type in Scala is perfect for handling the existence (or lack thereof) of XML nodes. It makes the absence of data explicit and safe to work with.
val xml = <person><name>John Doe</name></person>
val age = (xml \ "age").headOption.flatMap(n => Some(n.text.toInt))
age match {
case Some(a) => println(s"Age is $a.")
case None => println("Age is not specified.")
}
Why Option Type?
Using Option
prevents NullPointerExceptions
that can occur when accessing missing elements. It's a type-safe way to work with data that might be undefined.
5. Handling Invalid XML Documents
Your application might face XML data with broken structure or invalid characters, leading to failed parsing attempts.
Solution: Validation and Sanitization
Before parsing, validate your XML against a schema if possible, and use sanitization functions to ensure that the data is well-formed and safe to parse.
def sanitize(input: String): String = {
// Placeholder for sanitization logic
input.filter(_.isDigit || _.isLetter || "<>".contains(_))
}
val rawData = "...Invalid XML data..."
val cleanData = sanitize(rawData)
// Optionally, validate against a schema here
val xml = scala.xml.XML.loadString(cleanData)
Why Validation and Sanitization?
Sanitization ensures the XML data is free of illegal characters that can break the parsing process. Validation against a schema guarantees the structure is as expected, catching errors before they lead to runtime exceptions.
Conclusion
Scala provides powerful tools for XML processing, but it's not without its complexities. By understanding how to handle large files, parse without XPath, deal with namespaces, manage optional elements, and clean up invalid documents, you're better equipped to tackle XML parsing issues in Scala.
With the strategies outlined in this blog post, you'll navigate the intricacies of XML parsing and elevate your Scala development to new heights. Don't forget to test-drive these solutions in your next Scala project and appreciate the language's full potential when working with XML. Happy coding!
For further reading on Scala XML parsing, consider reviewing the official Scala XML documentation and the Scala Language Specification. Remember, practice makes perfect. Keep experimenting and solving issues as they arise to refine your Scala XML parsing skills.