Java's Type Inference: Resolving Var Misuse
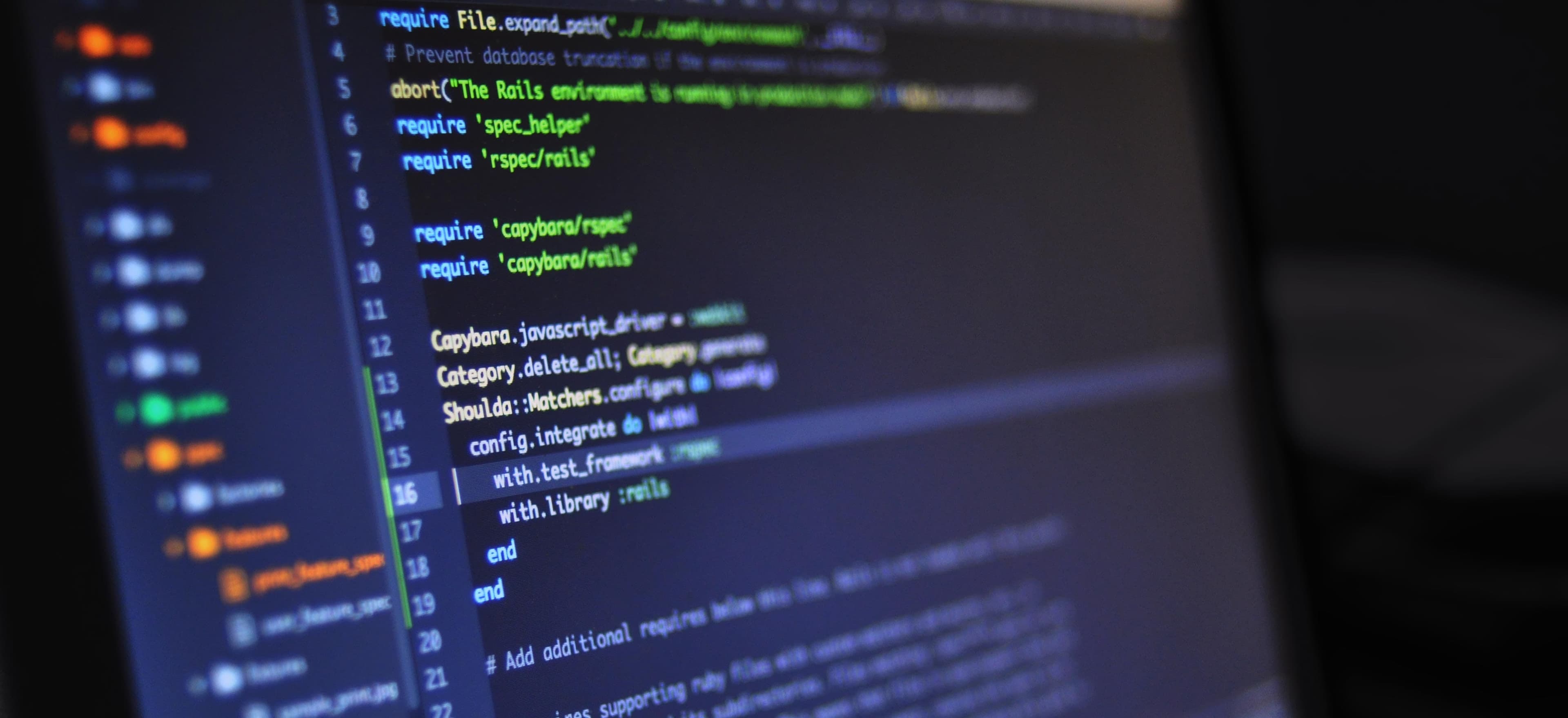
- Published on
Java's Type Inference: Navigating Through var
and Avoiding Misuse
Java, a statically-typed programming language renowned for its robustness and object-oriented frameworks, introduced a new feather in its cap with the launch of Java 10 in March 2018—local variable type inference with the var
keyword. This addition aims to enhance the Java programmer's experience by adding a sprinkle of syntactical sugar, which allows for less verbosity and more readable code.
What is var
in Java?
In Java, the var
keyword permits the omission of the explicit type declaration when the compiler can infer the type from the context. This feature is limited to local variables with initializers, indexes in the enhanced for-loop, and locals declared in a traditional for-loop.
var list = new ArrayList<String>(); // Inferred as ArrayList<String>
var stream = list.stream(); // Inferred as Stream<String>
Why use var
? The simple answer is readability and succinctness. var
can make your code cleaner, especially when dealing with generics or long type names.
Harnessing the Power of var
: Best Practices
Use var
when the Type is Obvious
The primary rule of thumb is to use var
when the variable's type is crystal clear from its initializer.
var message = "Hello, World!"; // Clearly a String
var userMap = new HashMap<>(); // Clearly a HashMap
Avoid var
when the Type is Obscure
However, it's also easy to misuse var
, especially in scenarios where the type isn't discernible at a glance. If the right-hand side of the assignment doesn't make it blatantly obvious what the type is, it's better to stick to an explicit type declaration.
// Not recommended
var result = myMethod(); // What is result's type?
// Recommended
MyType result = myMethod(); // Type is explicit
Favor var
with Complex Generic Types
One of var
's superpowers is simplifying the declaration of variables with complex generic types.
// Without var, quite verbose
Map<User, List<Account>> userAccounts = new HashMap<>();
// With var, much cleaner
var userAccounts = new HashMap<User, List<Account>>();
Consistent Coding Style
Consistency is key. Teams should decide on the usage patterns of var
to avoid a confusing mix of inferred and explicit typing. Code style guides can help maintain a balance, ensuring that all developers on a team are on the same page.
Potential Pitfalls and How to Navigate Them
Just because you can use var
, doesn't mean you should. Misusing var
can lead to less readable and maintainable code.
Loss of Clarity
Using var
indiscriminately can obscure the developer's intent, which can be a significant disadvantage, especially when working with poorly named methods or unfamiliar APIs.
// What does getData() return?
var data = getData();
A reader might have to navigate to the getData
implementation to understand the data type, increasing cognitive load.
Increased Risk of Errors
Type inference mistakes can lead to subtle bugs. Always ensure that the inferred type aligns with your expectations.
var id = getUserID(); // Is this an Integer, String, or a custom ID type?
Compatibility with Older Versions of Java
Remember, var
is only available from Java 10 onward. If you're writing library code or APIs intended for use in environments that run older Java versions, var
should be avoided.
Practical Examples: The Right Way to Use var
in Java
Let's jump into some code examples to illuminate the concepts we've discussed so far.
Example 1: Stream Operations
Stream operations frequently involve complex generics, making var
an excellent choice for enhancing readability.
var stream = list.stream();
var filteredList = stream.filter(e -> e.isActive())
.collect(Collectors.toList());
Here, the use of var
makes the stream operations fluent and easy to follow without the clutter of generic types.
Example 2: try-with-resources
with var
The try-with-resources
block is another perfect candidate for var
, where the type is evident from the context of the AutoCloseable resource.
// Before Java 10, explicit type needed
try (InputStream in = new FileInputStream("path/to/file.txt")) {
// Process the file
}
// With Java 10 and var, cleaner code
try (var in = new FileInputStream("path/to/file.txt")) {
// Process the file
}
Example 3: Large Nested Data Structures
Large nested data structures can benefit significantly from var
to avoid verbose type declarations.
// Without var, very cumbersome
Map<String, Map<String, List<Product>>> categoryMap = new HashMap<>();
// With var, much more digestible
var categoryMap = new HashMap<String, Map<String, List<Product>>>();
By using var
, we make the code cleaner while still keeping the type information accessible at the variable declaration.
When Not to Use var
in Java
Despite the advantages of var
, certain situations call for caution or even avoiding its use altogether.
In Public APIs
When defining public interfaces, classes, or methods, explicit typing provides clear contracts to the users of the API. Thus, var
should be generally avoided in public API design.
In Ambiguous Initializations
Any initialization that doesn't make the type self-evident should not use var
.
// Unclear initialization
var db = getDatabaseConnection(); // What is the type of db?
For Primitive Types
Typically, there's little to gain from using var
with primitive types, as the type names are already succinct.
// Not much benefit here
var index = 0; // vs int index = 0;
Conclusion
Java's introduction of var
offers a great tool to reduce verbosity and increase code legibility when used judiciously. By following best practices and avoiding potential pitfalls, Java developers can harness the power of type inference to write cleaner, more readable code.
To continue your journey of mastering Java's features, consider exploring other enhancements in recent Java versions, and always remember to write code that's as self-explanatory as possible. Happy coding!