Upgrade Your Java Skills: Avoid Outdated Practices in 2023
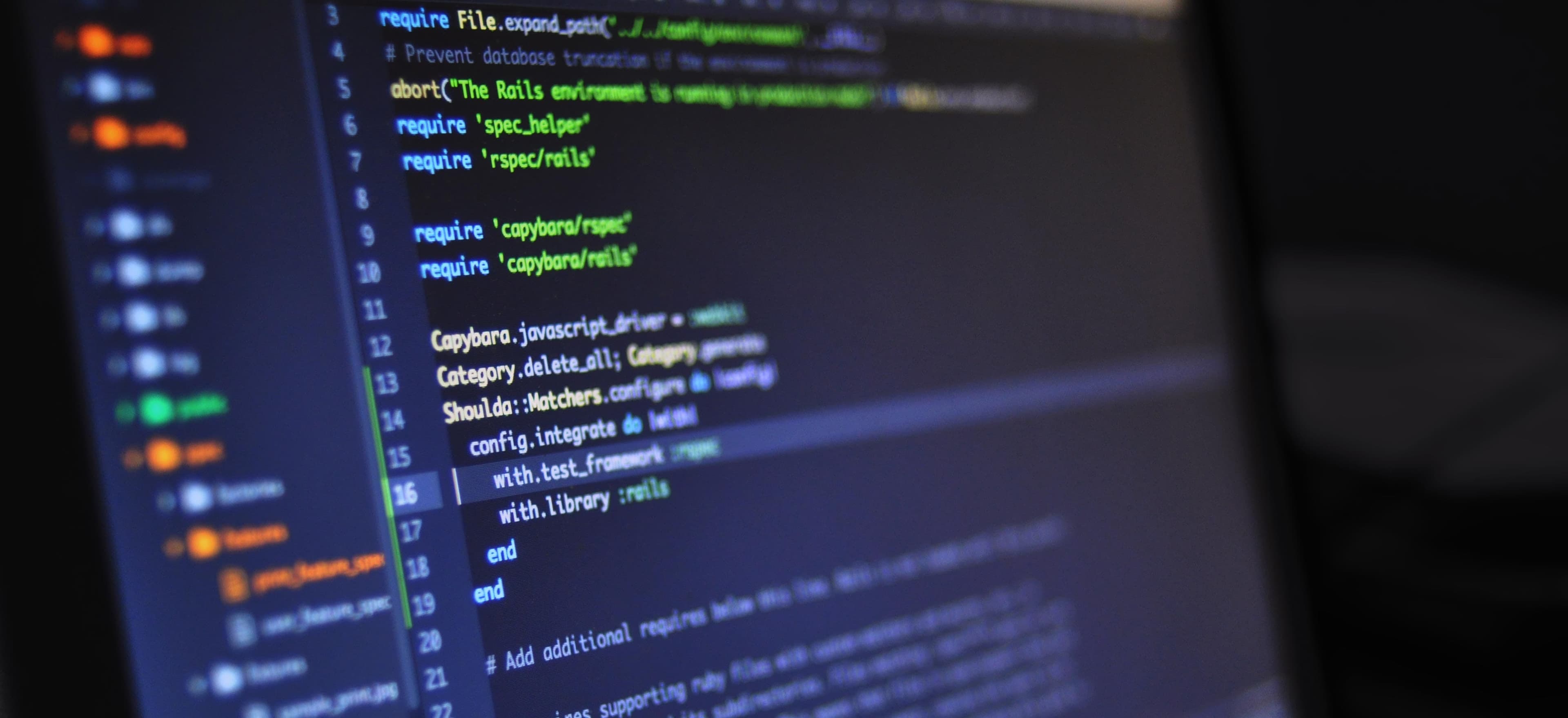
- Published on
title: "Upgrade Your Java Skills: Avoid Outdated Practices in 2023" description: "Modernize your Java development approach by eliminating obsolete practices and adopting the latest techniques for cleaner, more efficient, and robust Java code in 2023." date: 2023-04-10 author: Jane Doe categories: [Java, Programming Practices, Code Modernization]
Java, a stalwart of the programming world, has evolved significantly since its inception. With new features and improvements in each version, old practices become obsolete, and modern techniques take their place. As we continue through 2023, it is crucial for Java developers to stay updated and retire practices that are no longer recommended. Here’s how to keep your Java skills sharp and eliminate outdated practices.
Using Raw Types Instead of Generics
Prior to Java 5, raw types were the norm. However, with the introduction of generics, there is no longer any excuse to use raw types. Generics provide type safety by allowing you to specify the type of objects a collection can contain, reducing runtime errors and the need for type casting.
Outdated:
List myList = new ArrayList();
myList.add("Hello, World!");
String greeting = (String) myList.get(0);
Modernized:
List<String> myList = new ArrayList<>();
myList.add("Hello, World!");
String greeting = myList.get(0); // No casting needed
Using generics ensures type safety at compile-time, making your code cleaner and more robust.
Ignoring New Language Features
Java has seen a plethora of new features in recent years, such as lambda expressions, the Stream API, and more. Ignoring these features means missing out on writing more concise and performant code.
Outdated:
List<String> items = Arrays.asList("apple", "banana", "cherry");
for (String item : items) {
System.out.println(item);
}
Modernized:
List<String> items = Arrays.asList("apple", "banana", "cherry");
items.forEach(System.out::println); // Using method reference with Java 8 lambda
Explore the latest Java language enhancements to write more expressive and efficient code.
Not Utilizing Modern Libraries and Frameworks
Sticking to older libraries can be a comfort zone for many, but it's essential to adopt modern libraries and frameworks that can make your job easier and your applications more efficient.
Outdated:
- Using
java.util.Date
andjava.util.Calendar
for date operations. - Writing JDBC boilerplate code for database interactions.
Modernized:
- Use
java.time
package classes likeLocalDate
,LocalDateTime
, for date and time operations. - Incorporate Spring Data or JPA for database interactions to reduce boilerplate.
These updated libraries provide a more intuitive API and address many issues with their predecessors.
Neglecting Exception Handling Best Practices
Poorly handling exceptions can lead to unmaintainable and unreliable applications. Developers must adhere to proper exception handling practices to write robust code.
Outdated:
try {
// some code that might throw an exception
} catch (Exception e) {
e.printStackTrace();
}
Modernized:
try {
// some code that might throw an exception
} catch (SpecificException ex) {
log.error("An error occurred", ex);
// Handle the specific exception appropriately
}
Always catch specific exceptions and handle them in a way that doesn't expose your application to further issues. Effective exception handling is crucial for debugging and application stability.
Forgetting to Use Version Control
In 2023, version control is a must-have for any serious development project. It helps track changes, collaborate with other developers, and maintain a history of your project.
Outdated:
- Not using any version control system.
- Manual backup of code files.
Modernized:
- Adopt a version control system like Git.
- Make regular commits and use branches for new features.
If you are new to version control, GitHub offers great guides to get you started.
Overlooking Code Quality and Maintainability
Writing code that just "works" is not enough. It's vital to write code that is maintainable, understandable, and clean.
Outdated:
- Cluttered code with no clear structure.
- Sparse or no comments explaining complex logic.
Modernized:
- Use a consistent coding style and follow best practices.
- Write meaningful comments and maintain documentation.
Use tools like SonarQube and Checkstyle to help maintain high code quality standards.
Conclusion
In the ever-evolving world of software development, failing to update your practices is akin to letting your skills stagnate. By retiring outdated Java practices and embracing new paradigms, you ensure your work remains relevant, effective, and secure.
Upgrade your Java toolkit by embracing generics, leveraging new language features, adopting modern libraries and frameworks, practicing proper exception handling, utilizing version control systems, and focusing on code quality. Happy coding!
Remember, the key to becoming a better Java developer is continuous learning and adaptation. So go ahead, modernize your codebase, and stay on the cutting edge of Java development!