Cracking Java Keystore Security: A How-To Guide
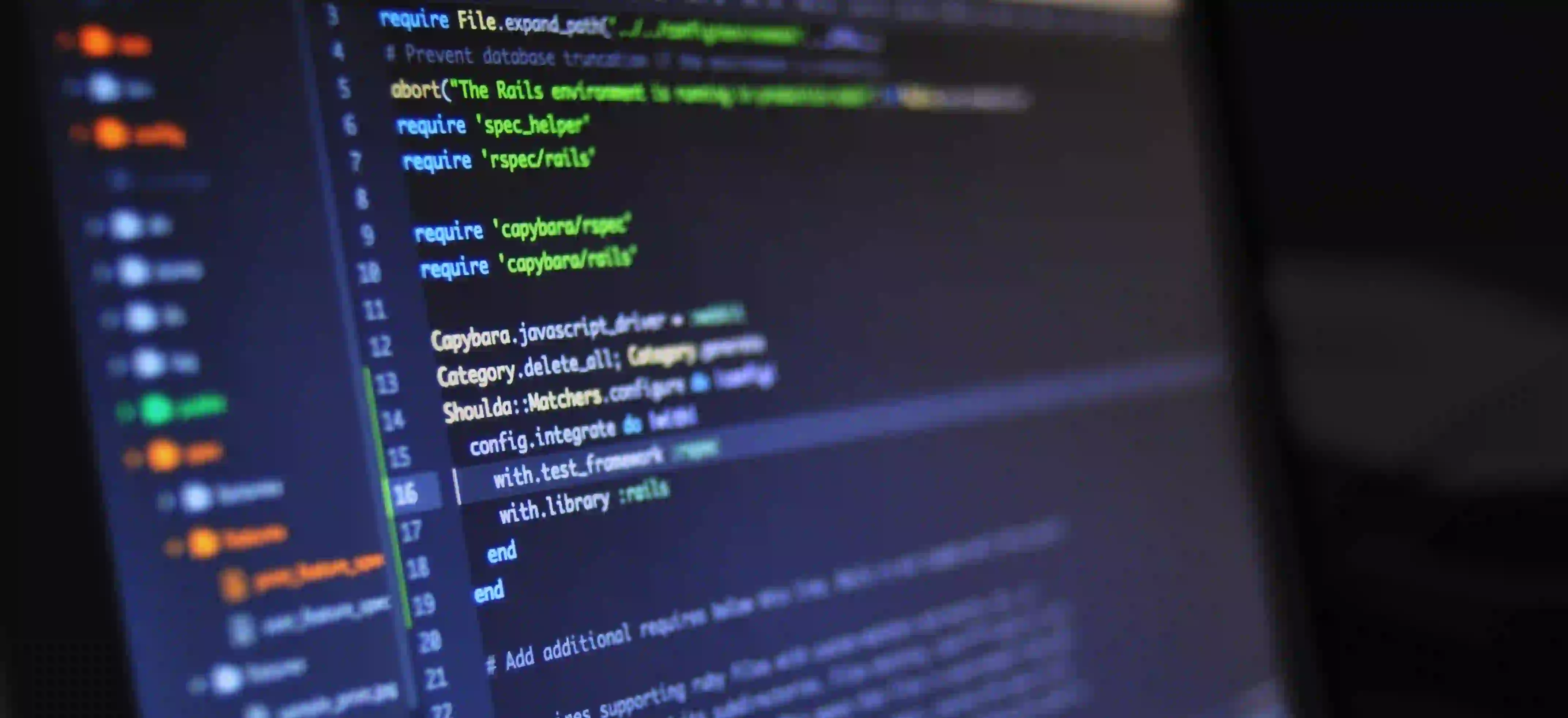
Cracking Java Keystore Security: A How-To Guide for Developers
The Java Keystore is an essential component in the Java universe for storing cryptographic keys and certificates. It ensures secured communication between servers and clients and protects sensitive data. However, as robust as the Java Keystore is, it's not impervious to security threats. In this blog post, we delve into the vulnerabilities of the Java Keystore and provide a detailed guide on how to exploit these weaknesses, primarily for educational and security testing purposes.
Disclaimer: The information shared in this post is for educational purposes only. Unauthorized hacking into, or cracking other people's systems or accounts is illegal and unethical.
Understanding Java Keystore
Before we attempt to crack the Java Keystore, let's understand what it is. A Java Keystore is a repository of security certificates – either authorization certificates or public key certificates – and private keys that Java applications use for various security purposes. Keystores are managed through the keytool
utility that ships with the JDK.
For a more in-depth understanding, here's the official documentation on keytool
.
Common Java Keystore Types
- JKS (Java KeyStore): This is the original keystore type for Java. However, it's considered less secure due to its reliance on a proprietary format.
- PKCS12: This is an industry-standard format and is recommended over JKS due to its increased security.
Why Crack a Java Keystore?
There are several legitimate reasons for wanting to crack a Java Keystore:
- Recover Lost Passwords: Sometimes, administrators inherit systems without proper documentation and need to recover the passwords to the existing keystores.
- Penetration Testing: As part of a security audit, security professionals might need to assess the strength of the keystore's password.
- Educational Purposes: Understanding how keystores can be compromised can lead to developing better security practices.
Tools Required for Cracking Java Keystore
- Java Keytool: Comes with the Java Development Kit (JDK).
- Brute Force Tools: Tools like
John The Ripper
orhashcat
are used for brute-forcing passwords.
The Process: Cracking Java Keystore
To crack a Java Keystore, follow these general steps:
Step 1: Install Required Tools
You must have the Java Development Kit (JDK) installed, and if you're using brute force tools like John The Ripper
or hashcat
, ensure they are properly installed on your machine. Here's how you might install John The Ripper on a Unix-like system:
sudo apt-get update
sudo apt-get install john
Step 2: Extract the Hash
To begin, you'll need to extract the hash from the keystore using keytool:
keytool -list -keystore <your_keystore.jks>
You will be prompted for the keystore password, which, if unknown, is where the brute force tools come into play.
Step 3: Convert the Keystore to a John The Ripper-Compatible Format
Once you have the keystore file, use a keytool2john
or similar script to convert the keystore into a format that John The Ripper can work with:
/usr/share/john/keytool2john.py <your_keystore.jks> > hash.txt
Step 4: Brute Force the Password
With the hash in hand, use John The Ripper to attempt to crack the password:
john --wordlist=<your_wordlist.txt> hash.txt
Replace <your_wordlist.txt>
with your desired wordlist file. There are many wordlists available online like rockyou.txt
which could be used for this purpose.
Step 5: Assess the Results
John The Ripper will attempt to brute force the password and, if successful, will display it. Keep in mind, the complexity of the password and the size of the wordlist will greatly affect the time required to crack the keystore.
Writing Secure Java Code
As developers, it’s crucial to write secure code to minimize vulnerabilities. Here are some Java practices relating to keystore security:
KeyStore ks = KeyStore.getInstance(KeyStore.getDefaultType());
// Load the keystore
char[] password = System.console().readPassword("Enter the keystore password: ");
try (FileInputStream fis = new FileInputStream("path/to/keystore.jks")) {
ks.load(fis, password);
} catch (IOException | NoSuchAlgorithmException | CertificateException e) {
e.printStackTrace();
}
// After using the password, immediately overwrite the character array for security.
Arrays.fill(password, '0');
Why this code matters:
- It uses the
KeyStore
class to work with the keystore in a secure way. - The password is read from the console, which is more secure than hardcoding it.
- It immediately overwrites the password array, which minimizes the window where it could be exploited.
Best Practices for Java Keystore Security
- Strong Passwords: Use complex passwords for keystores and change them regularly.
- Secure Transmission: Never transmit keystores or passwords in plaintext.
- Minimum Privilege: Restrict keystore file permissions to only the necessary users.
- Regular Audits: Perform regular security audits on your keystores.
Conclusion
While it's possible to crack Java Keystore security, the primary goal should always be to prevent unauthorized access through robust security practices. By understanding the vulnerabilities and how cracking can be done, you can take measures to secure your keystores against such attacks.
Remember, secure coding and keystore management is a part of the bigger picture of Java application security. Make use of the tools and practices mentioned wisely to ensure the integrity and confidentiality of your Java applications' sensitive data.
Stay informed, stay secure!