Securing JBoss AS 7: Crafting Custom Login Modules
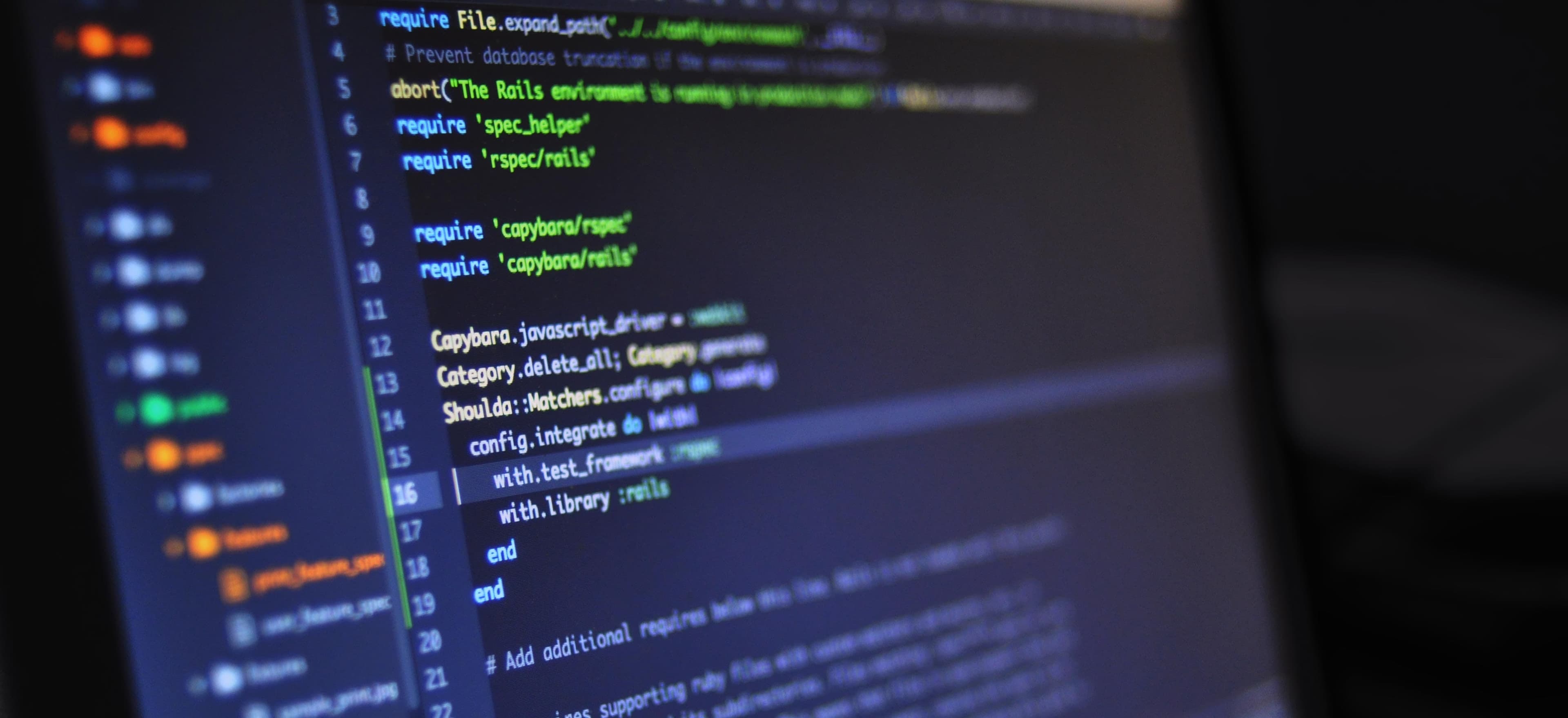
- Published on
Securing JBoss AS 7: Crafting Custom Login Modules
When it comes to securing Java EE applications, understanding the nuts and bolts of the underlying application server can mean the difference between a robust application and a vulnerable one. JBoss AS 7 JBoss Application Server, now known as WildFly, is among the leading open-source servers for hosting Java applications. Its flexibility and Java EE compliance make it a favorite choice among many enterprise developers.
The Java Authentication and Authorization Service (JAAS) provides a way for a Java EE application to authenticate and authorize a user or group of users to ensure security at various levels. In this post, we're going to focus on the customization of login modules in JBoss AS 7 to create a tailored authentication mechanism.
Understanding Login Modules
Login modules are the building blocks of authentication in JAAS. They are pluggable components responsible for various types of authentication such as username/password check, certificate authentication, or even more sophisticated methods involving biometrics.
To customize a login module in JBoss AS 7, we need to create a class that extends javax.security.auth.spi.LoginModule
. Then we configure our custom login module as part of a security domain in the JBoss standalone.xml
or domain.xml
configuration file.
Here's why customized login modules can be game changers:
- Tailor authentication to match your proprietary security requirements.
- Integrate with legacy systems or third-party authentication services.
- Implement complex security protocols not supported by generic modules.
Crafting a Custom Login Module
Step 1: Create the Login Module Class
Let's create a basic custom login module that simply checks if the username and password are correct.
import javax.security.auth.login.LoginException;
import javax.security.auth.spi.LoginModule;
import javax.security.auth.callback.*;
import java.util.Map;
public class MyCustomLoginModule implements LoginModule {
private CallbackHandler callbackHandler;
@Override
public void initialize(Subject subject, CallbackHandler callbackHandler,
Map<String, ?> sharedState, Map<String, ?> options) {
this.callbackHandler = callbackHandler;
}
@Override
public boolean login() throws LoginException {
Callback[] callbacks = new Callback[2];
callbacks[0] = new NameCallback("Username: ");
callbacks[1] = new PasswordCallback("Password: ", false);
try {
callbackHandler.handle(callbacks);
} catch (Exception e) {
throw new LoginException("Error retrieving username and password");
}
String username = ((NameCallback) callbacks[0]).getName();
char[] passwordCharArray = ((PasswordCallback) callbacks[1]).getPassword();
String password = new String(passwordCharArray);
// Dummy validation, replace with your own mechanism (e.g. against a database)
return "admin".equals(username) && "secret".equals(password);
}
// Other methods like commit, abort, logout need to be implemented as well
// ...
}
Explanation:
initialize()
sets the state for the login module.login()
retrieves the username and password and validates them.NameCallback
andPasswordCallback
are used to fetch user credentials.- Replace the dummy validation logic with your own authentication mechanism.
Step 2: Configuration in standalone.xml
or domain.xml
The next step is to define this login module in JBoss's security domain configuration.
<security-domain name="MySecurityDomain" cache-type="default">
<authentication>
<login-module code="com.mydomain.security.MyCustomLoginModule" flag="required">
<module-option name="param1" value="value1"/>
<!-- Add more module options if needed -->
</login-module>
</authentication>
</security-domain>
Explanation:
- The
security-domain
element defines a domain name. - The
login-module
element’scode
attribute points to the fully qualified class name of our custom login module. - The
flag
attribute can be set to "required", "requisite", "sufficient", or "optional", defining module behavior. module-option
elements allow you to pass additional parameters to the login module.
Step 3: Link the Security Domain to the Application
In your application's jboss-web.xml
file (which should be located in the WEB-INF
folder of your web application), specify the security domain that you have configured.
<jboss-web>
<security-domain>MySecurityDomain</security-domain>
</jboss-web>
Explanation:
security-domain
tells JBoss that our app is secured using the custom logic defined inMyCustomLoginModule
.
Managing Dependencies and Security Risks
When developing custom login modules, it's critical to manage dependencies and security risks appropriately.
- Maven Dependencies: Ensure your
pom.xml
includes the necessary JBoss/WildFly artifacts for local development/testing. - Secure Credential Storage: Never hard-code credentials; use secure storage mechanisms, such as hashed or encrypted passwords.
- Input Validation: Always validate inputs to prevent common security threats like SQL Injection or Cross-Site Scripting (XSS).
Conclusion
Custom login modules in JBoss AS 7 provide an extended level of flexibility and control over authentication mechanisms. By implementing the LoginModule
interface, you can define specific behavior tailored to your security requirements. Remember to update your server configuration accordingly and maintain security best practices at all times.
In enterprise environments, security isn't just a feature but a foundational component of the application. Making smart use of technologies like JBoss AS 7 and its extensible security framework can empower businesses to protect sensitive data and ensure user trust.
For additional resources, you may find the JBoss AS 7 documentation helpful.
Secure your applications by starting with a solid authentication strategy, and always keep an eye out for new security trends and updates!