Solve REST API Glitches with Tomcat & Spring CXF!
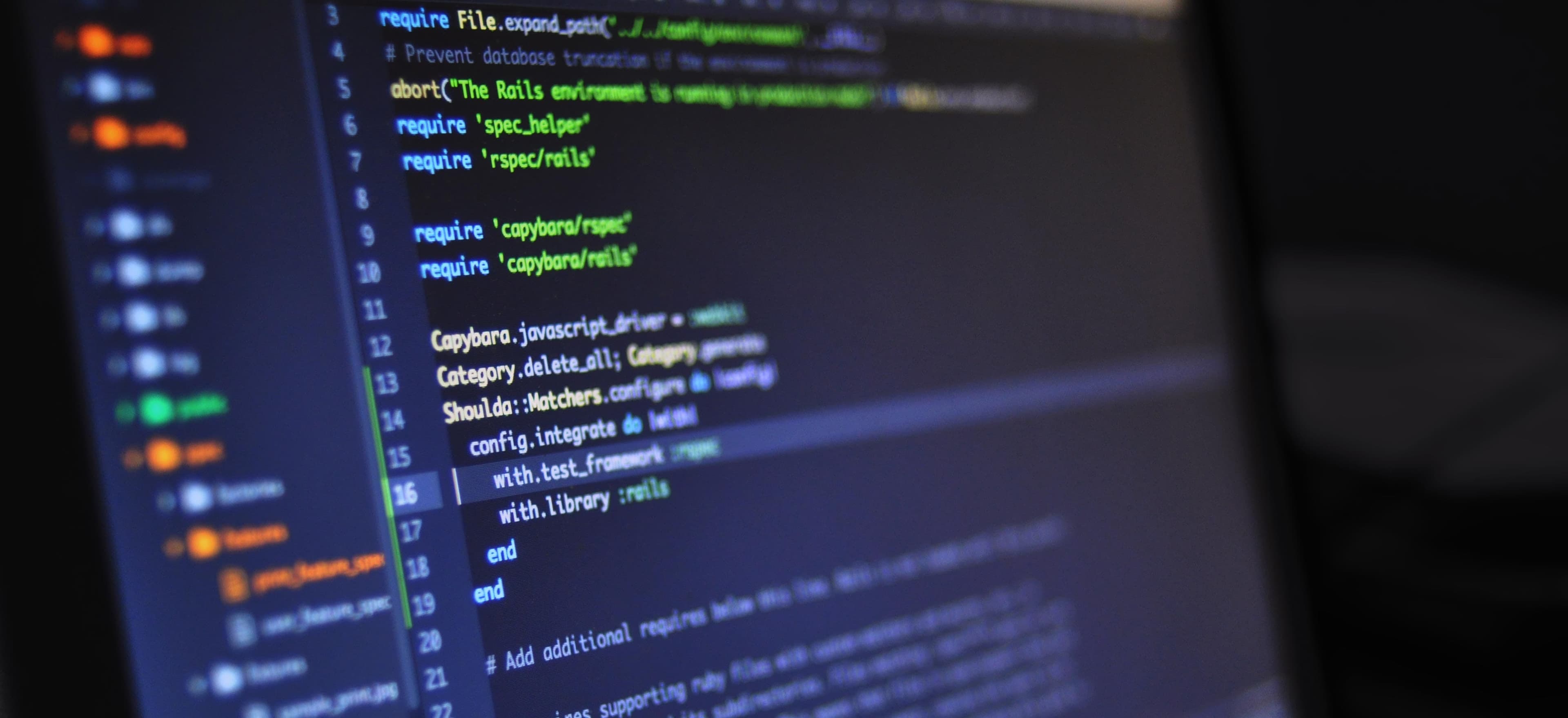
- Published on
Solve REST API Glitches with Tomcat & Spring CXF: A Developer's Guide
In the world of Java development, creating smooth, glitch-free REST APIs is a common goal for many developers. Leveraging powerful frameworks such as Spring and tools like Apache Tomcat can make this task significantly easier. However, even with these technologies, developers can encounter glitches that impede the functionality of their APIs. This article delves into troubleshooting REST API issues when using Spring CXF with Tomcat, providing actionable solutions to ensure a seamless experience for your users.
Understanding Spring CXF and Tomcat
Before we dive into solving REST API glitches, let's briefly cover what Spring CXF and Tomcat are.
Spring Framework is one of the most popular application frameworks for Java. Its flexibility and comprehensive set of features make it an excellent choice for building web applications, including REST APIs.
Apache CXF is an open-source services framework that aids in building web services. CXF integrates with Spring to provide a seamless development experience when creating REST APIs.
Apache Tomcat is a widely used web server that provides a "pure Java" HTTP server environment for running Java code. It is often the go-to choice for deploying Java web applications like Spring-powered REST APIs.
Now, let's address some common REST API glitches and their solutions while working with Spring CXF and Tomcat.
Glitch #1: NoHttpResponseException in Clients
One common issue when clients interact with a REST API is the NoHttpResponseException
. This occurs when the Tomcat server closes a connection that a client was using.
// Example NoHttpResponseException
org.apache.http.NoHttpResponseException: The target server failed to respond
Solution: Configure Tomcat's server.xml
to better manage connection timeouts. This ensures that connections aren't closed prematurely.
<!-- server.xml -->
<Connector port="8080"
protocol="HTTP/1.1"
connectionTimeout="20000"
redirectPort="8443" />
Glitch #2: Slow Response Times
If you're experiencing slow response times, it could be due to inadequate server resources or inefficient code.
Solution: Optimize your REST API code by profiling and identifying bottlenecks. Moreover, tweak Tomcat's thread pool settings to handle more concurrent requests:
<!-- server.xml -->
<Connector port="8080"
protocol="HTTP/1.1"
maxThreads="200"
minSpareThreads="50"
connectionTimeout="20000"
redirectPort="8443" />
Glitch #3: Serialization Glitches
Java object serialization and deserialization is crucial in REST APIs. A glitch here can result in incorrect data representation or even complete failures.
Solution: Ensure that your Java objects are correctly annotated for serialization. For instance, using Jackson annotations with CXF:
import com.fasterxml.jackson.annotation.JsonProperty;
public class User {
@JsonProperty("id")
private long id;
@JsonProperty("name")
private String name;
// Getters and setters omitted for brevity
}
Glitch #4: Versioning and Endpoint Conflicts
REST API versioning and endpoint conflicts are common sources of frustration.
Solution: Design your REST API with versioning in mind from the start. Use URL patterns or request headers to manage versions. For example:
import javax.ws.rs.Path;
import javax.ws.rs.GET;
import javax.ws.rs.Produces;
import javax.ws.rs.core.MediaType;
@Path("/api/v1/users")
public class UserResourceV1 {
@GET
@Produces(MediaType.APPLICATION_JSON)
public Response getUsersV1() {
// Your code to fetch and return users for version 1
}
}
@Path("/api/v2/users")
public class UserResourceV2 {
@GET
@Produces(MediaType.APPLICATION_JSON)
public Response getUsersV2() {
// Your code to fetch and return users for version 2
}
}
Glitch #5: Inconsistent Error Handling
Inconsistent and unclear error responses are a major problem for REST API integrators.
Solution: Implement a global exception handler in Spring to return clear and consistent error payloads.
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.http.ResponseEntity;
import org.springframework.http.HttpStatus;
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(Exception.class)
public ResponseEntity<Object> handleException(Exception ex) {
ApiError apiError = new ApiError(HttpStatus.INTERNAL_SERVER_ERROR, ex.getMessage());
return new ResponseEntity<>(apiError, apiError.getStatus());
}
// Define ApiError class according to your error format
}
Integrating Spring CXF with Tomcat
Here's a quick overview of setting up a simple REST API using Spring CXF and deploying it to Tomcat.
Step 1: Maven Dependencies
Add the necessary Maven dependencies for Spring, CXF, and your project-specific needs.
<!-- pom.xml -->
<dependencies>
<!-- Spring framework -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-web</artifactId>
<version>{spring-version}</version>
</dependency>
<!-- Apache CXF -->
<dependency>
<groupId>org.apache.cxf</groupId>
<artifactId>cxf-rt-frontend-jaxrs</artifactId>
<version>{cxf-version}</version>
</dependency>
<!-- Other dependencies here -->
</dependencies>
Step 2: Defining the REST Endpoint
Create your REST resources with annotations for Spring and JAX-RS (the Java API for RESTful Web Services).
import org.springframework.stereotype.Component;
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
import javax.ws.rs.core.MediaType;
@Component
@Path("/greetings")
public class GreetingResource {
@GET
@Produces(MediaType.TEXT_PLAIN)
public String sayHello() {
return "Hello, World!";
}
}
Step 3: Configuring Spring and CXF
Configure Spring application context and CXF servlet in your web.xml
or with Java-based configuration.
import org.apache.cxf.jaxrs.JAXRSServerFactoryBean;
import org.apache.cxf.transport.servlet.CXFServlet;
@Bean
public ServletRegistrationBean cxfServlet() {
return new ServletRegistrationBean(new CXFServlet(), "/api/*");
}
@Bean
public JAXRSServerFactoryBean restService() {
JAXRSServerFactoryBean factoryBean = new JAXRSServerFactoryBean();
factoryBean.setServiceBeans(Arrays.asList(new GreetingResource())); // Add REST resources here
factoryBean.setAddress("/");
return factoryBean;
}
Step 4: Building and Deploying the Application
Build the application with Maven and deploy the resulting .war
file to Tomcat.
mvn clean install
Drag and drop the generated .war
file into Tomcat's webapps
directory or use the Tomcat Manager App to upload and deploy your application.
Conclusion
By utilizing the right configuration, clear API design, and efficient coding practices, you can troubleshoot and solve common REST API glitches with Tomcat and Spring CXF. Remember to profile, optimize, and regularly review your API's health to maintain a smooth, error-free service for your clients.
For further reading on Spring, CXF, and Tomcat, visit the official documentation:
Always ensure that your REST APIs are reliable and robust by actively addressing these common glitches. Your users will thank you for the smooth performance and stability of your service. Happy coding, and may your API calls always return with a 200 OK!