Mastering Java VM: Conquering YoungGen Space Issues
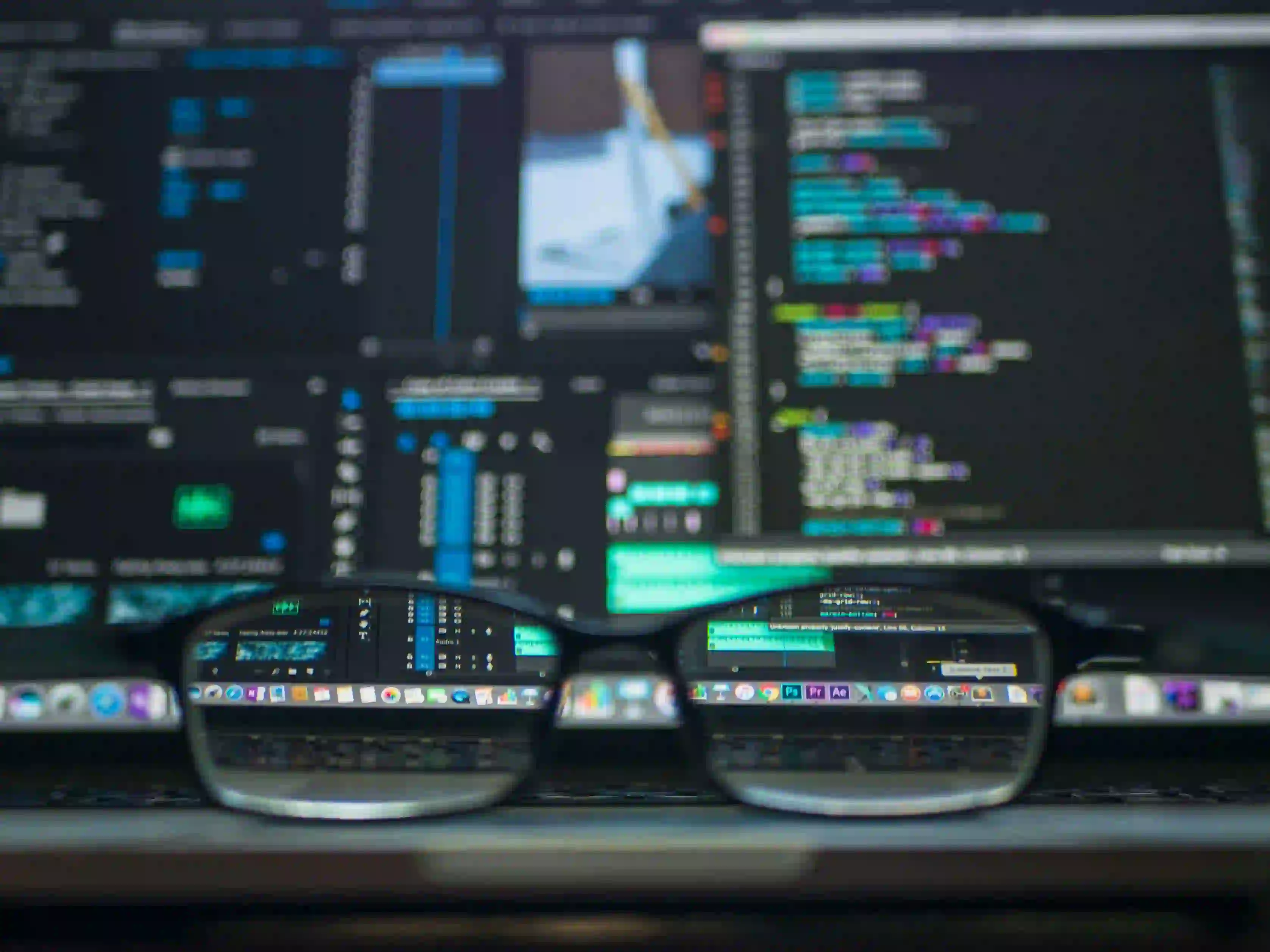
Mastering Java VM: Conquering YoungGen Space Issues
Java Virtual Machine (JVM) is the cornerstone of Java's write-once-run-anywhere principle. For developers and dev-ops professionals, understanding its inner workings, especially the memory management aspect, is vital for creating efficient Java applications. In this blog post, we will focus on the Young Generation (YoungGen) space within the JVM, why it matters, and how to optimize it to prevent common issues.
Understanding Java Memory Structure
Before diving into the YoungGen space, let's briefly outline the JVM memory structure. The JVM memory is divided into several regions:
- Heap memory: Where objects are stored.
- Non-Heap memory: Used to store class metadata, method codes, etc.
- Young Generation: Part of the heap for newly created objects.
- Old Generation (OldGen): For objects that have survived the garbage collection in YoungGen.
- Permanent Generation (PermGen) / Metaspace: For storing class metadata (PermGen is used in JDK 7 and earlier, Metaspace in JDK 8 and later).
Each area has a specific purpose and understanding the implications of how they work is key to optimizing Java performance.
The Importance of YoungGen
The Young Generation space is crucial because it's often the first stop for newly created objects. The JVM uses a garbage collection (GC) algorithm called a 'minor GC' to clean it up frequently, which is typically faster than the 'major GC' that cleans the OldGen space because it contains short-lived objects (thus, "young").
The YoungGen space is further divided into three parts:
- Eden Space: Where most objects are created initially.
- Survivor Spaces (S0 and S1): Here objects are moved from Eden when it gets cleaned up, and then they toggle between these spaces until they're either collected or moved to OldGen.
Managing the YoungGen is a balancing act. Set it too small, and you'll have frequent minor GCs which can affect performance. Too large, and you could be underutilizing memory and still face performance issues.
Addressing YoungGen Issues
Ensuring optimal performance of the YoungGen space involves:
- Correct Sizing: Understanding memory demands and tuning the YoungGen to the right size using JVM options like
-Xmn
or more granularly with-XX:NewRatio
. - Garbage Collection Tuning: Picking the right GC algorithm and tuning its parameters. Recent JVMs use the G1 GC algorithm by default, but there are others like Parallel GC, CMS, and ZGC, each with its own strengths.
- Memory Leak Prevention: Writing code that does not create unnecessary objects and allows the garbage collector to reclaim memory efficiently.
Code Snippets and Tuning Examples
Let's take a look at how you might write code and tune the JVM to manage YoungGen space effectively.
Efficient Object Creation
Ensure you are not creating unnecessary objects within your code:
public class CustomerService {
// Avoid creating objects within frequently called methods
public Customer findCustomer(String id) {
// Bad Practice: Creates a new DateFormat instance on every call
DateFormat df = new SimpleDateFormat("yyyyMMdd");
// ... perform search and return Customer Object
}
// Instead, reuse objects whenever possible
private static final DateFormat df = new SimpleDateFormat("yyyyMMdd");
public Customer findCustomerImproved(String id) {
// Reuses a single DateFormat instance - better for memory management
// ... perform search and return Customer Object
}
}
Why it helps: By not creating a DateFormat
instance every time the method is called, you're reducing the number of objects entering YoungGen space. This makes minor GC more efficient.
JVM Tuning with Command-line Options
To adjust the size of the YoungGen space, you'd use the -Xmn
option or a combination of -XX:NewSize
and -XX:MaxNewSize
. Here's an example command-line argument for a JVM:
java -Xms2G -Xmx2G -Xmn1G -XX:SurvivorRatio=8 MyJavaApp
Why these settings:
-Xms2G
and-Xmx2G
set the initial and maximum size of the heap to 2GB.-Xmn1G
sets the size of the YoungGen to 1 GB.-XX:SurvivorRatio=8
indicates that the Eden space will be eight times larger than one of the Survivor spaces.
Remember that these ratios and sizes need to be tailored for your specific application workload. You might have to iterate through several configurations before finding the most optimal one.
Monitoring and Analysis
Analyzing your application's garbage collection patterns and memory usage can lead to better tuning decisions. Use JVM monitoring tools like VisualVM or jConsole to gather data about how your application manages memory.
// Simple logging of memory usage
long memoryUsed = Runtime.getRuntime().totalMemory() - Runtime.getRuntime().freeMemory();
System.out.println("Memory Used: " + memoryUsed);
The above code can log the memory usage, which can provide insights informally, but it's no substitution for comprehensive profiling tools that can offer in-depth analysis.
Going Deeper: GC Algorithms
Java developers should also familiarize themselves with garbage collection algorithms that predominantly affect the YoungGen space.
- Serial GC: Good for small applications with a single-threaded garbage collector.
- Parallel GC: A multi-threaded counterpart of Serial GC, suited for medium to large-sized applications.
- Concurrent Mark Sweep (CMS): Focuses on low-latency and reduced GC pause times.
- Garbage-First (G1): Divides the heap into uniformly sized regions and balances throughput with pause times.
- Z Garbage Collector (ZGC) and Shenandoah: Aim for low-latency with large heaps, introduced in later Java versions (Java 11 and beyond).
Choosing the proper GC algorithm and tuning its parameters is a matter of balancing memory requirements, pause times, and application throughput.
For further reading on GC algorithms and how to tune them for YoungGen, the Oracle Garbage Collection Handbook is an excellent resource.
Conclusion: Balancing Act
Conquering YoungGen space issues in Java is all about understanding the balance between memory use and performance. It involves knowing your application's memory requirements, writing memory-efficient code, and tuning JVM settings to match your application's needs.
Remember, there's no one-size-fits-all solution; it takes monitoring, analysis, and keen insights into how your application interacts with JVM internals. With due diligence and a methodical approach, mastering the YoungGen space and enhancing your Java application's performance is completely achievable.
Happy coding and remember, the best-tuned JVM is one that complements your application's unique behavior and workload patterns!