Deploy Woes: Master Spring Boot on AWS EC2!
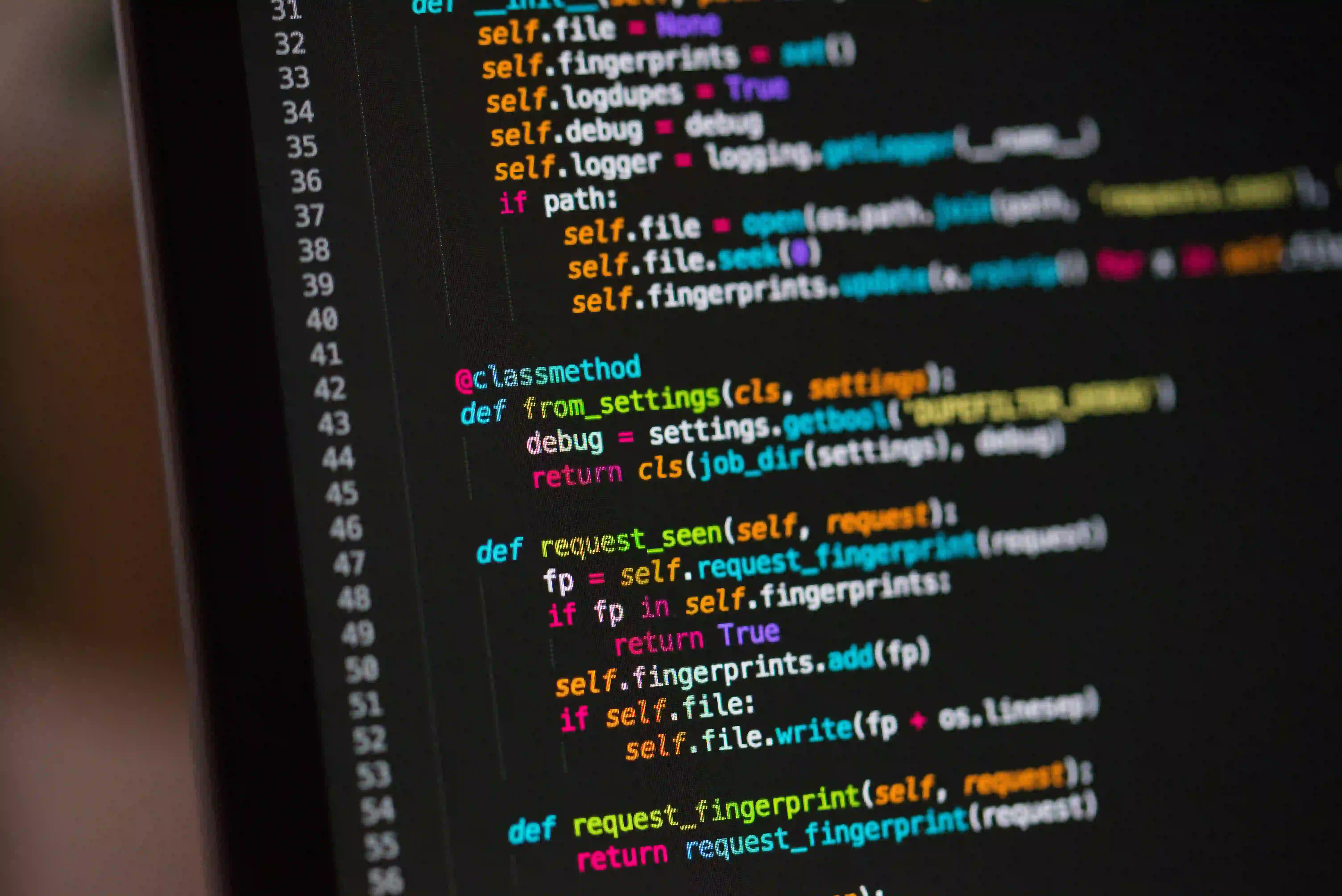
Deploy Woes: Master Spring Boot on AWS EC2!
Deploying applications is like solving a complex puzzle with high stakes. If you're a Java developer who cherishes writing elegant code, the deployment phase can feel like stepping into a different world. But fret not; once you grasp the principles it's quite rewarding, and with Amazon Web Services (AWS) EC2, running your Spring Boot application in the cloud can be a breeze.
Why AWS EC2 for Spring Boot?
Amazon EC2 (Elastic Compute Cloud) offers flexible, scalable, and cost-effective computing resources. This makes it an excellent option for deploying Spring Boot applications, given Spring Boot's quick ramp-up and emphasis on convention over configuration. With AWS EC2, developers can focus on writing code without worrying about the underlying infrastructure.
Preparing Your Spring Boot Application
Before you delve into the AWS wilderness, ensure your Spring Boot application is production-ready. This involves steps like incorporating Spring profiles for different environments and storing sensitive information in environment variables or secret management services.
Example application.properties
for Production:
server.error.include-message=always
spring.jpa.hibernate.ddl-auto=update
spring.datasource.url=jdbc:postgresql://<db-url>:<port>/<db-name>
spring.datasource.username=${DB_USERNAME}
spring.datasource.password=${DB_PASSWORD}
Notice the use of environment variables DB_USERNAME
and DB_PASSWORD
. They provide a secure way to access your database credentials without hard-coding them into your application.
AWS EC2 Setup
The first step is to set up an EC2 instance. Log in to the AWS Management Console and follow these steps:
- Select the EC2 service.
- Click Launch Instance.
- Choose your desired AMI (Amazon Machine Image), like the basic Amazon Linux 2 AMI.
- Select an instance type –
t2.micro
should suffice for small-scale applications. - Configure your instance and add your SSH keys.
- Set your security groups – allow traffic on port 8080 (default Spring Boot), and port 22 for SSH.
- Review and launch the instance.
Connecting to the Instance
To connect to your instance via SSH, retrieve your instance's public DNS from the EC2 Management Console and use your private key:
ssh -i /path/my-key-pair.pem ec2-user@ec2-198-51-100-1.compute-1.amazonaws.com
Replace /path/my-key-pair.pem
with the path to your key file and ec2-user@ec2-198-51-100-1.compute-1.amazonaws.com
with your instance's user and public DNS.
Deploying Spring Boot on EC2
Step 1: Install Java
Spring Boot applications run on the JVM, so Java must be installed:
sudo yum update -y
sudo yum install java-1.8.0-openjdk-devel
This will install OpenJDK 8, which you can check with java -version
.
Step 2: Transfer Your JAR
Use scp
to securely copy your Spring Boot application's JAR file to your EC2 instance:
scp -i /path/my-key-pair.pem /path/my-spring-boot-app.jar ec2-user@ec2-198-51-100-1.compute-1.amazonaws.com:~
Replace the placeholders with your key path, JAR path, and instance details.
Step 3: Run Your JAR
Now, execute the JAR file on your EC2:
java -jar my-spring-boot-app.jar &
The &
suffix allows the app to run in the background.
Your application should now be accessible over the internet using your EC2 instance's public DNS/IP followed by the appropriate port!
Automating Deployments with AWS CodeDeploy
While manual deployments are informative, they aren't practical for busy devs. That's where AWS CodeDeploy steps in.
AWS CodeDeploy automates deployments, ensuring they're repeatable, scalable, and safe. It works with EC2, AWS Fargate, AWS Lambda, and your on-premises servers.
To get started, you'll need to create an appspec.yml
file to define the deployment actions and lifecycle event hooks for your application.
Example appspec.yml
:
version: 0.0
os: linux
files:
- source: /target/my-spring-boot-app.jar
destination: /home/ec2-user/
hooks:
BeforeInstall:
- location: scripts/stop_server.sh
AfterInstall:
- location: scripts/start_server.sh
ApplicationStart:
- location: scripts/validate_service.sh
Scripts in hooks
handle stopping the application before updating it, starting it after the update, and validating that it's running correctly.
AWS RDS with Spring Boot
Most applications need a database, and AWS RDS (Relational Database Service) is a seamless choice. When creating a database on RDS, remember to adjust the security group to allow your EC2 instance to communicate with it.
Database Configuration:
Update your application.properties
with your RDS endpoint:
spring.datasource.url=jdbc:postgresql://<rds-endpoint>:5432/mydb
spring.datasource.username=admin
spring.datasource.password=secret
Replace <rds-endpoint>
, admin
, and secret
with actual values.
Final Thoughts
With these guidelines in hand, you're now ready to elevate your Spring Boot application into the AWS EC2 cloud. Automated deployments, security best practices, and tight integration with essential services like AWS RDS provide a robust environment to scale and manage your applications.
While we've covered the essentials of deploying a Spring Boot application to AWS EC2 in this post, remember, AWS provides comprehensive documentation and further resources to broaden your knowledge.
Just remember this: your journey to cloud mastery might start with a few hiccups, but with consistent learning and practice, deploying on AWS will become second nature. Embrace the path ahead, and may your applications run smoothly in the expansive sky of AWS cloud services.