Boost Speed: Mitigate Scripting Drag in Processes
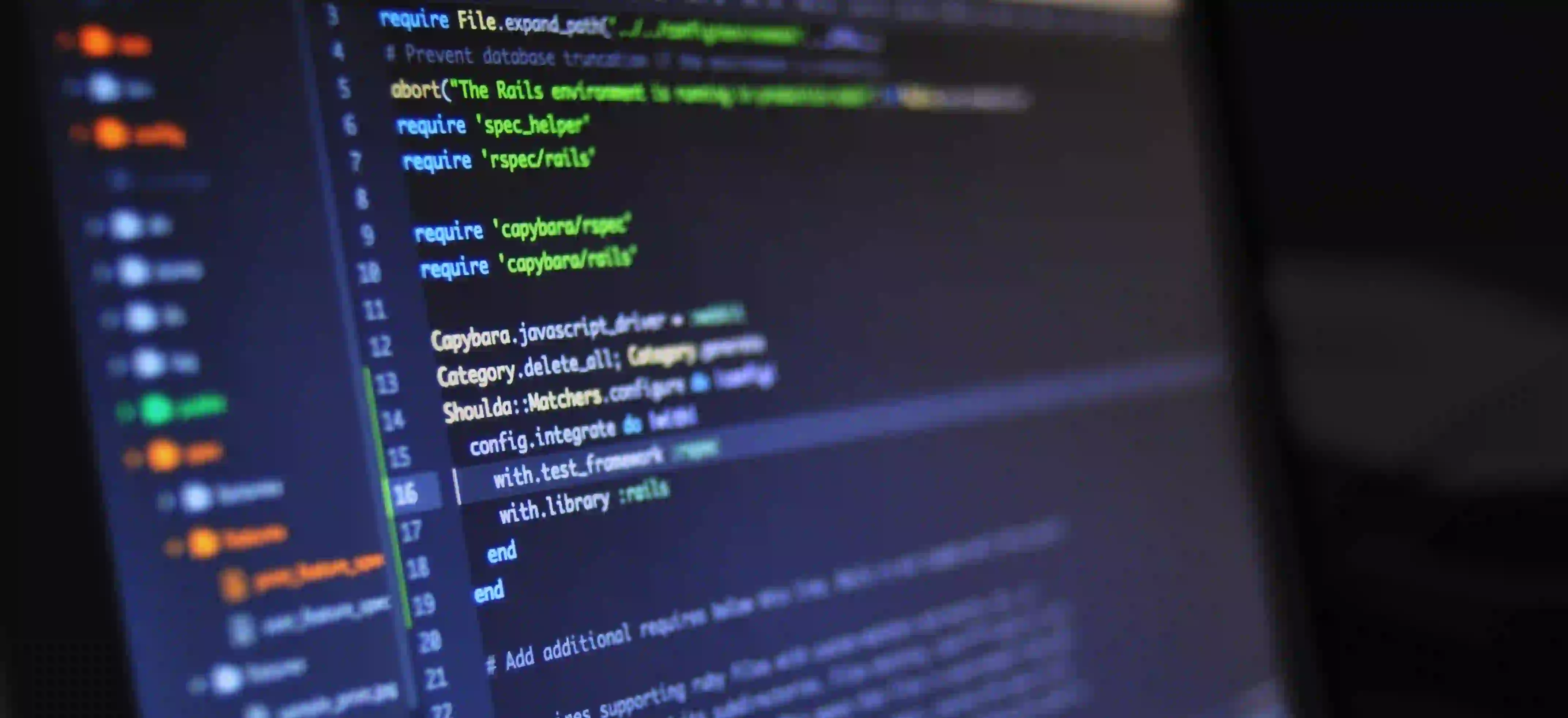
Boost Speed: Mitigate Scripting Drag in Processes
In the realms of software development and IT operations, scripting remains an indispensable tool. But sometimes, scripting can introduce unexpected latency into system processes, dragging down performance and efficiency.
Understanding Scripting Overhead
Every seasoned Java developer knows that scripting is like the Swiss Army knife of programming -- versatile and convenient. However, it's not always the fastest. Scripts, especially those not well-optimized, can add overhead that slows down execution. It's critical to understand where the drag comes from to mitigate it effectively.
The Bottlenecks
- Interpretation Overhead: Unlike Java bytecode which is compiled once and runs on the Java Virtual Machine (JVM), scripts are interpreted at runtime, adding an extra processing layer.
- I/O Bound Processes: Scripting often involves reading from or writing to a file system or network, which is inherently slower than in-memory operations.
- Suboptimal Algorithms: Poorly written scripts with inefficient algorithms can significantly slow down execution.
Let's deep dive into ways to alleviate these pain points and enhance your scripting within Java.
Optimize Your Scripting
Choose the Right Tool
Sometimes it's better to compile Java code for repetitive tasks rather than relying on scripts. For example, if you're processing large datasets frequently, consider using Java's Stream API.
List<String> myList = Files.readAllLines(Paths.get("largefile.txt"));
myList.stream()
.filter(s -> s.contains("important"))
.map(String::toUpperCase)
.forEach(System.out::println);
In the code snippet above, we're processing a file line by line with a stream, filtering, and then manipulating the data. This approach leverages Java's strengths: speed and efficiency.
Minimize I/O Operations
Reduce file or network operations by batching them or by using asynchronous I/O where possible. Here's an example of using BufferedWriter
to minimize I/O interactions.
try (BufferedWriter writer = new BufferedWriter(new FileWriter("output.txt"))) {
for (String data : dataList) {
writer.write(data);
}
}
This code writes data in larger chunks, reducing the number of I/O calls, which in turn minimizes the scripting drag.
Use Efficient Algorithms and Data Structures
Always aim for the most efficient algorithm and suitable data structure for the task at hand. Below is an example comparing two ways of searching in a list, demonstrating why choice of algorithm matters:
// Inefficient: Linear Search
for(String item : myList) {
if(item.equals("searchTerm")) {
// item found
break;
}
}
// Efficient: HashSet for O(1) lookup times
Set<String> mySet = new HashSet<>(myList);
if(mySet.contains("searchTerm")) {
// item found
}
The second method uses a HashSet, offering constant time complexity for lookups, which is significantly faster than a linear search through a list.
Profile and Monitor Scripts
Always instrument your scripts to have an insight into their performance. Java profilers and monitoring tools such as VisualVM or JProfiler can help you pinpoint the sources of scripting drag.
The Case for Multithreading
Java multithreading can be leveraged to perform multiple scripting tasks in parallel, dramatically speeding up the overall process. However, it is essential to use multi-threading judiciously, as it can introduce complexity and new kinds of bottlenecks if not managed properly.
Here's an example using Java's ExecutorService
to execute tasks in parallel:
ExecutorService executorService = Executors.newFixedThreadPool(4);
for (int i = 0; i < 10; i++) {
Runnable task = createYourTask(i);
executorService.execute(task);
}
executorService.shutdown();
With this pattern, instead of running tasks sequentially, they are distributed across a fixed number of threads, which can be particularly beneficial if the tasks are I/O bound.
Embracing JIT Compilation
Just-In-Time (JIT) compilation in Java can significantly improve the performance of your scripts. JIT compiles parts of the bytecode that have similar functionality at runtime, thus enhancing speed. For performance-critical applications, consider ahead-of-time (AOT) compilation which compiles bytecode into machine code before execution.
Here’s how you can leverage GraalVM, a high-performance JVM, for JIT or AOT compilation to speed up scripts:
# JIT with GraalVM
java -jar myscript.jar
# AOT compilation with GraalVM’s native-image
native-image --no-server -jar myscript.jar
# Run the native image
./myscript
AOT compilation with GraalVM may offer faster startup time and less memory consumption, which is excellent for scripting applications where performance is paramount.
The Power of Caching
Caching is another effective strategy to speed up scripts that perform repetitive tasks. If your script performs the same expensive operation repeatedly, use a caching mechanism to store and retrieve results quickly.
Below is a simple example of caching in Java using a HashMap
:
private Map<String, Object> cache = new HashMap<>();
public Object expensiveOperation(String key) {
return cache.computeIfAbsent(key, k -> {
// perform the expensive operation
return new Object();
});
}
This pattern avoids performing the expensive operation if the result is already available in the cache, thus reducing scripting overhead.
Conclusion
Improving the performance of scripts within Java applications is a nuanced challenge but one that yields substantial improvements in speed and efficiency. By understanding the nature of scripting drag and implementing strategies to minimize it, you can ensure that your Java applications remain nimble and responsive.
Whether you're choosing the right tools, optimizing I/O operations, using efficient algorithms, or leveraging multithreading, there's always room to refine your scripts. Remember to monitor performance closely with profiling tools, embrace JIT or AOT compilation for critical tasks, and don't forget the power of caching. Motor through your Java projects with scripts that enhance, not hinder, your application performance.