Mastering SOA: Tackling Common Integration Issues
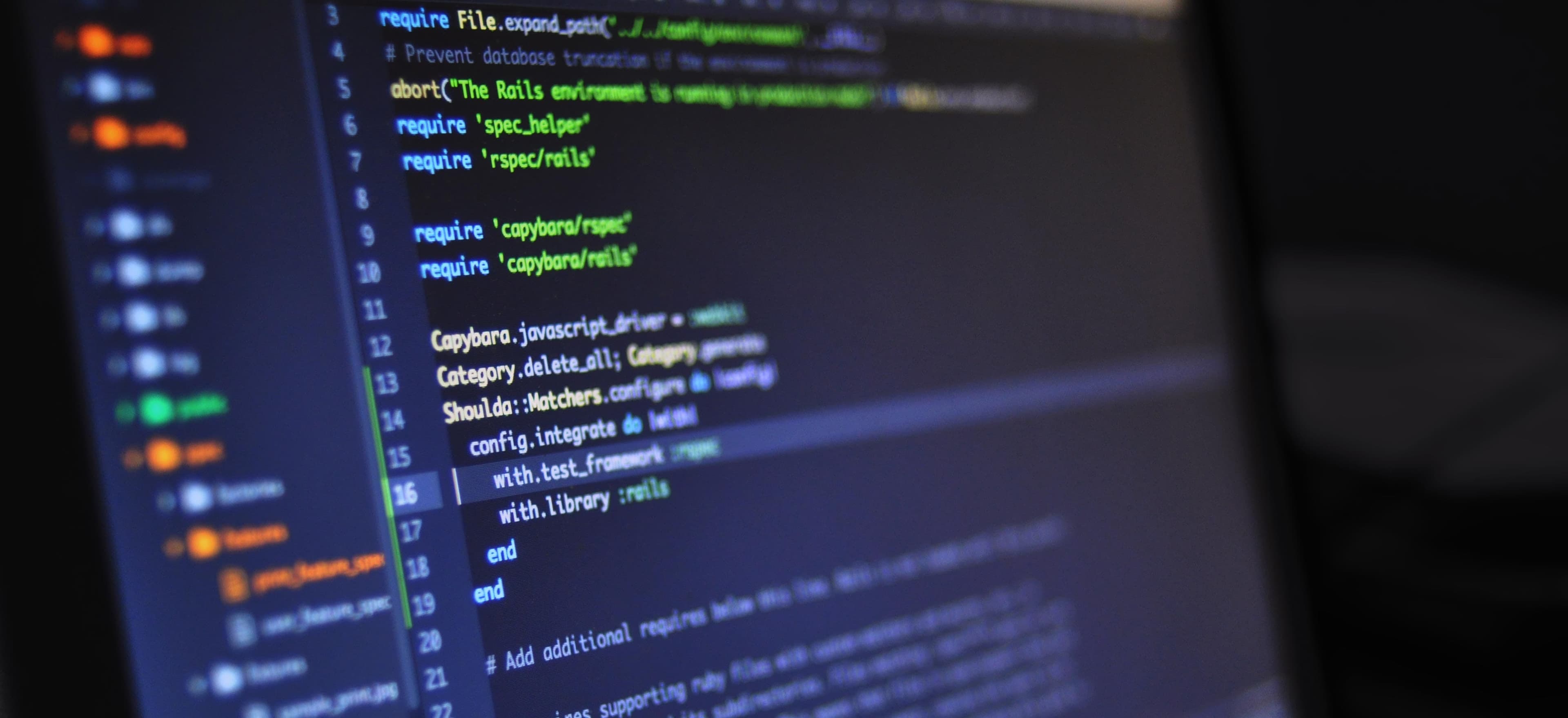
- Published on
Mastering SOA: Tackling Common Integration Issues with Java
Service-Oriented Architecture (SOA) revolutionized the way businesses approach enterprise integration and application design. By emphasizing reusable services, SOA provides a framework that supports agility, scalability, and efficiency. However, while integrating applications using SOA, developers often encounter common issues that can hinder the full realization of SOA benefits. In this blog post, we dive deep into these challenges and offer Java-based solutions, complete with code snippets and clear explanations to help you master SOA integration.
What is SOA?
Before we get into the nitty-gritty, let's briefly recap the concept of SOA. SOA is an architectural pattern that allows services to communicate over a network through a loose coupling of components. It enables different services to work together, independent of individual implementation details or platforms.
Common SOA Integration Challenges
Challenge 1: Handling Service Interoperability
In SOA, services are often built using different technologies and platforms, creating interoperability issues. How do you get these services to communicate effectively?
Solution: Use Web Services and Standard Protocols
Java provides a robust platform for creating interoperable services with JAX-WS (Java API for XML Web Services). It allows Java services to communicate using standard web service protocols like SOAP.
package com.example.soaservices;
import javax.jws.WebService;
import javax.jws.WebMethod;
@WebService
public class OrderProcessingService {
@WebMethod
public String processOrder(String orderDetails) {
// Business logic to process order details
return "Order processed successfully";
}
}
This code snippet defines a simple web service in Java for processing orders. The @WebService
annotation makes it a web service, and the @WebMethod
annotation exposes the processOrder
method as a service operation.
Challenge 2: Maintaining Service Scalability
As demand grows, services need to scale without causing disruptions or performance bottlenecks.
Solution: Implement Microservices Architecture
Java's Spring Boot framework simplifies building microservices, which can be independently deployed and scaled. Here's a glimpse into defining a Spring Boot microservice:
package com.example.soamicroservices;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class InventoryServiceApplication {
public static void main(String[] args) {
SpringApplication.run(InventoryServiceApplication.class, args);
}
}
This snippet demonstrates a Spring Boot application for an inventory microservice. The @SpringBootApplication
annotation does the heavy lifting by auto-configuring your application for microservice architecture.
Challenge 3: Ensuring Message Reliability
SOA services must communicate reliably, even in the face of network or system failures.
Solution: Implement Messaging Queues
Java Message Service (JMS) API facilitates reliable communication between SOA services using message queues. Here's how you can send a message with JMS in Java:
import javax.jms.Connection;
import javax.jms.ConnectionFactory;
import javax.jms.Destination;
import javax.jms.JMSContext;
import javax.jms.JMSProducer;
import javax.jms.Message;
import javax.jms.Session;
// Assume connectionFactory is injected or looked up from JNDI
ConnectionFactory connectionFactory = ...
try (JMSContext context = connectionFactory.createContext(Session.AUTO_ACKNOWLEDGE)) {
Destination destination = context.createQueue("orderQueue");
JMSProducer producer = context.createProducer();
Message message = context.createTextMessage("Order details message");
producer.send(destination, message);
}
With this code, you create a JMS context from a ConnectionFactory
, create a message for the order details, and send it to an order queue. JMS takes care of the rest, ensuring the message is delivered reliably.
Challenge 4: Managing Service Versioning
When services are updated or evolved, clients using the old versions might face compatibility issues.
Solution: Version Services Thoughtfully
To manage service versions in Java, keep backward compatibility whenever possible, and if not, version your APIs thoughtfully. Here's an example of how you can approach this:
package com.example.soaservices.v1;
import javax.jws.WebMethod;
import javax.jws.WebService;
// Version 1 of the OrderService
@WebService(endpointInterface = "com.example.soaservices.v1.OrderService")
public class OrderService {
@WebMethod
public String placeOrder(String orderDetails) {
// Logic for placing an order
return "Order placed successfully";
}
}
If you need to evolve this service, instead of modifying it directly, you can create a new version:
package com.example.soaservices.v2;
import javax.jws.WebMethod;
import javax.jws.WebService;
// Version 2 of the OrderService
@WebService(endpointInterface = "com.example.soaservices.v2.OrderService")
public class OrderService {
@WebMethod
public String placeOrder(String orderDetails, boolean expedited) {
// Updated logic for placing an order with an option for expedited shipping
return expedited ? "Expedited order placed successfully" : "Order placed successfully";
}
}
By maintaining separate packages and endpoint interfaces, you can ensure that clients have the time to adapt to new versions without breaking existing functionality.
Challenge 5: Handling Service Discovery
In a large SOA ecosystem, keeping track of available services and their endpoints can be difficult.
Solution: Implement a Service Registry
With frameworks like Eureka from the Netflix OSS suite integrated with Spring Cloud, service discovery becomes manageable. Here's a Spring application main class that registers itself with Eureka:
package com.example.soaservices;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.netflix.eureka.EnableEurekaClient;
@SpringBootApplication
@EnableEurekaClient
public class PaymentServiceApplication {
public static void main(String[] args) {
SpringApplication.run(PaymentServiceApplication.class, args);
}
}
The @EnableEurekaClient
annotation indicates that the service should be discovered via Eureka. This way, services can dynamically discover each other without hard-coded endpoints.
Conclusion
SOA integration can be fraught with challenges, but armed with Java and the right strategies, these obstacles can be transformed into opportunities for creating robust, scalable, and interoperable services. Additionally, mastering the use of frameworks and libraries like Spring Boot, Spring Cloud, JAX-WS, and JMS ensures that your Java SOA services stand the test of time and scale.
Whether you're grappling with service interoperability or the intricacies of versioning and discovery, Java's rich ecosystem offers a solution. By leveraging these techniques and best practices, you are well on your way to mastering SOA and pushing the boundaries of enterprise integration.
Implement these Java solutions in your SOA efforts, and you'll be sure to notice a smoother, more efficient service landscape that's ready to adapt to the ever-evolving demands of modern business.