Boost Coding Efficiency: Mastering New Programming Techniques
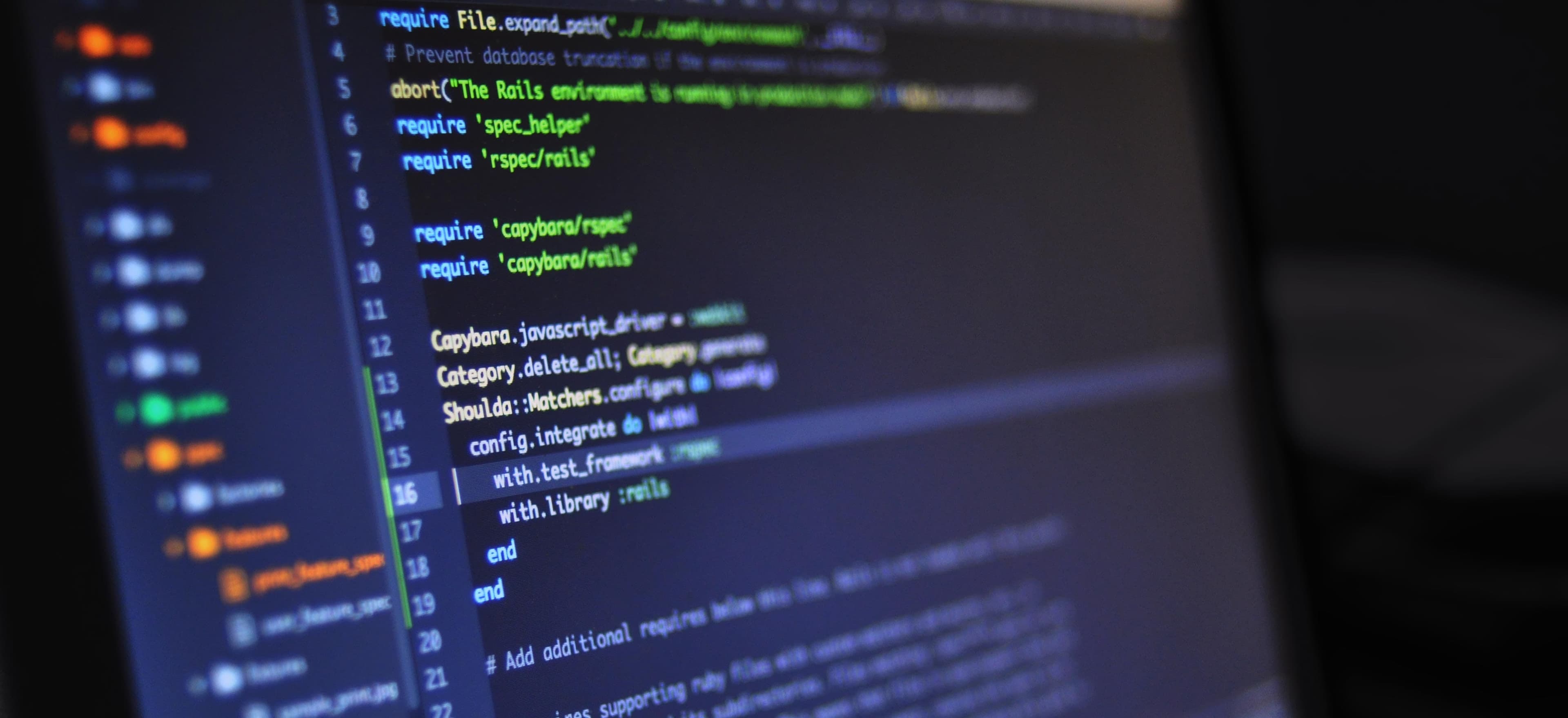
- Published on
Boost Coding Efficiency: Mastering New Programming Techniques in Java
As the digital world evolves at an unprecedented pace, the demand for more refined and efficient coding techniques has surged. Java, being one of the most widely-utilized programming languages, offers a vast arena for developers to hone their skills and craft code that not only meets functionality requirements but also performs optimally under the hood.
In this deep dive, we will uncover how you can escalate your Java programming efficiency. We will delve into technically rich discussions filled with code snippets and explore how small tweaks can lead to significant performance improvements. Additionally, we will navigate the delicate balance between code readability and efficiency – ensuring your Java code is as clear to understand as it is performant.
Adopting Best Practices for High-Performance Java Code
Understand The Collections Framework
Java Collections are the bedrock of data management within the language, and understanding them is a must for writing efficient code.
List<String> names = new ArrayList<>();
// Why: ArrayList is typically faster for iterating and retrieving elements.
names.add("Alice");
names.add("Bob");
Set<Integer> uniqueNumbers = new HashSet<>();
// Why: HashSet is used because it prevents duplicates and is faster for lookups.
uniqueNumbers.add(23);
uniqueNumbers.add(42);
Leveraging the correct collection type can vastly decrease memory overheads and speed up operations like search and sort. Here, choosing ArrayList
over a LinkedList
can improve random access speed, and HashSet
aids in faster search operations. Oracle's documentation provides a wealth of information on collections.
Streamline Your Loops
Avoid unnecessary work inside loops. For example, consider refactoring variable declarations outside of loops and look to use enhanced for-loops for clarity and reduced overhead.
int sum = 0;
for (int i : numbers) {
sum += i;
}
// Why: Enhanced for-loops remove the need for index handling and are easier to read.
Immutable Objects for the Win
Immutable objects guarantee that once an object is created, its state cannot change. This can lead to significant simplifications in concurrency situations and remove the need for defensive copies.
public final class Address {
private final String street;
private final String city;
public Address(String street, String city) {
this.street = street;
this.city = city;
}
// No setters, state cannot change.
public String getStreet() { return street; }
public String getCity() { return city; }
}
// Why: Making Address immutable means safer code when dealing with concurrency.
Lambda Expressions and Functional Interfaces
Lambda expressions
in Java enhance code readability and conciseness. They pair wonderfully with functional interfaces in the Java API like Predicate
, Function
, and Consumer
.
List<String> filteredList = list.stream()
.filter(s -> s.startsWith("A"))
.collect(Collectors.toList());
// Why: Functional-style operations reduce boilerplate code and can exploit parallel computation.
Stream
operations come with a caveat though: they can be less performant than imperative code structures in some cases, so use them judiciously.
Write Cleaner, Maintainable Code
Leverage Var for Local Variable Type Inference
Java 10 introduced var
, allowing for local variable type inference.
var list = new ArrayList<String>();
// Why: Simplifies writing and readability without compromising type safety.
The use of var
makes your code neat, particularly when dealing with generic types.
Comment Wisely
Excessive commenting can clutter your code, but strategic documentation helps maintain readability and provides context for complex logic.
// Calculate the Fibonacci number for n
public int fibonacci(int n) {
// Why: Comments here give a quick understanding of the method's purpose.
// ...
}
Refactor Ruthlessly
Don't hesitate to break down complex methods or classes into smaller, more manageable pieces.
public void processData() {
// Original complex logic
}
// Why: Splitting into more specific methods not only improves readability but also promotes code reuse.
public void fetchInput() { /*...*/ }
public void validateData() { /*...*/ }
public void persistResults() { /*...*/ }
Testing Affairs
Testing is not directly related to Java coding, but indirectly, it's necessary for maintaining code efficiency and performance.
Unit Testing
Employ unit testing to ensure individual components perform as expected.
@Test
public void testFibonacci() {
assertEquals(5, fibonacci(5));
// Why: Testing individual units of code ensures they work correctly in isolation.
}
Tools such as JUnit provide comprehensive frameworks for Java unit testing.
Profiling Tools
Use profiling tools like JProfiler or VisualVM to identify bottlenecks in your application. Implement performance test cases to catch regressions.
Stay Updated with Java's Evolution
Java is a language that has stood the test of time thanks to its continued evolution. Staying abreast of the latest Java updates, such as Project Loom's developments in lightweight concurrency, or keeping an eye on upcoming Project Panama, which aims to improve Java's connection with native code, can furnish you with fresh approaches to address old and new coding challenges alike. The OpenJDK website is an excellent resource for this.
Remember the Basics
Lastly, never overlook the foundations: adhere to naming conventions, avoid premature optimization, and always measure the performance impacts of your changes.
In conclusion, becoming a master at crafting efficient Java code is not an overnight journey, but a continuous process of learning, practicing, and refining your approach. Keep iterating over your development practices, incorporate the aforementioned techniques, and watch your code's performance soar.
The intersection of skillful use of data structures, judicious application of new language features, and a relentless commitment to code quality is where truly efficient Java programming lies. By focusing on both the micro-optimizations within your code and the broader strokes of writing clear, maintainable, and robust Java, you can confidently push the envelope of what you can achieve with this versatile language.