Unlocking Efficiency: Resolving Java 19 Virtual Thread Issues
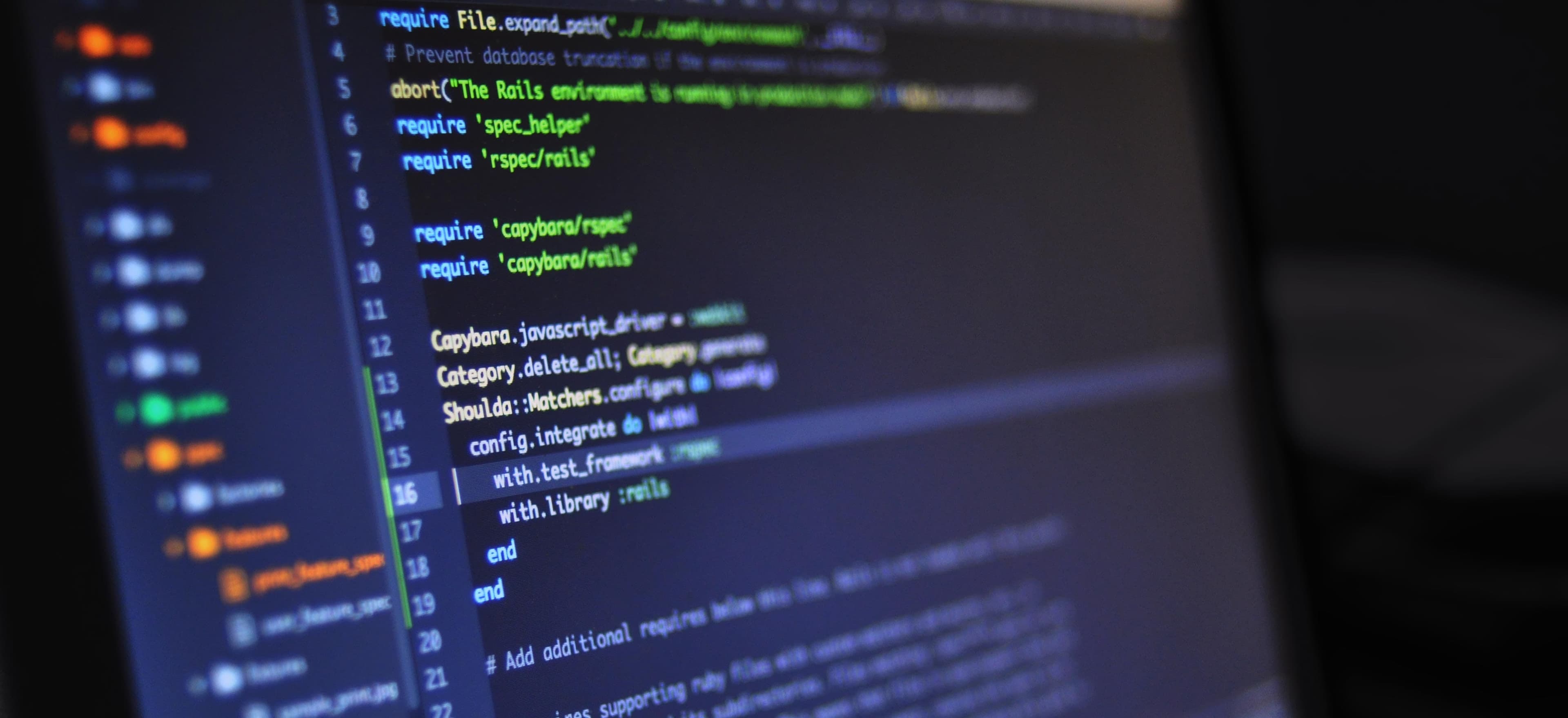
- Published on
Unlocking Efficiency: Resolving Java 19 Virtual Thread Issues
Java continually evolves, introducing features that aim to improve developer productivity and application performance. Java 19 introduces a revolutionary feature named Project Loom. One of the pivotal elements of Project Loom is the introduction of Virtual Threads, which are lightweight threads designed to simplify concurrent programming in Java by reducing the complexity and overhead associated with traditional threads.
In this post, we'll uncover the potential issues that could arise while working with Java 19's virtual threads, offering practical solutions and code snippets, ensuring that you can harness the full power of this feature and efficiently troubleshoot any problems.
Understanding Virtual Threads
Virtual Threads, also known as user-mode threads or fibers, are an abstraction of the underlying operating system's threads. They are lightweight and managed by the Java Virtual Machine (JVM), allowing you to create many virtual threads, far beyond what would be feasible with platform threads.
To contextualize the significance, let’s quickly compare:
- Platform Threads: Tied closely to the operating system, resource-heavy, and limited in number.
- Virtual Threads: Managed by the JVM, requiring fewer resources, and can be spawned in vast numbers.
Example Code: Creating a Virtual Thread
Creating a virtual thread in Java 19 is straightforward:
import java.util.concurrent.Executors;
public class VirtualThreadExample {
public static void main(String[] args) {
var executor = Executors.newVirtualThreadPerTaskExecutor();
executor.submit(() -> System.out.println("Hello from a virtual thread!"));
executor.shutdown();
}
}
In the above snippet, the newVirtualThreadPerTaskExecutor
factory method from Executors
class is used to create an executor optimized for virtual threads. The submit
method then runs a simple task on a virtual thread, printing a message to the console.
Identifying and Resolving Virtual Thread Issues
While virtual threads aim to reduce complexity, as with any cutting-edge feature, they introduce new challenges. Knowing how to identify and resolve these issues is critical.
Issue 1: Resource Starvation
When you indiscriminately create millions of virtual threads, you could run into resource starvation. Unlike platform threads, the virtual ones still consume heap space and could exhaust memory if not managed correctly.
Solution: Throttle Creation or Reuse Virtual Threads
Limit the number of concurrently running virtual threads using an executor service with a bounded queue or by reusing threads for multiple tasks.
Code Snippet: Limiting Virtual Threads
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.TimeUnit;
public class LimitedVirtualThreadExecutor {
public static void main(String[] args) throws InterruptedException {
ExecutorService executor = Executors.newVirtualThreadPerTaskExecutor();
for (int i = 0; i < 1_000_000; i++) {
executor.submit(() -> {
try {
// Simulating work
TimeUnit.MILLISECONDS.sleep(10);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
});
}
executor.shutdown();
executor.awaitTermination(1, TimeUnit.HOURS);
}
}
In this example, tasks are submitted too quickly to an unbounded executor service, which could cause memory issues. Modifying the executor service to have a bounded queue or rate-limiting submissions would alleviate this problem.
Issue 2: Deadlocks
Virtual threads do not remove the potential for deadlocks. These can still occur if virtual threads are waiting indefinitely for locks held by other threads.
Solution: Timeout-based Lock Acquisitions
Use timeouts for lock acquisitions or the try-lock pattern to prevent deadlocks by failing fast if a lock cannot be acquired within a certain period.
Code Snippet: Using Lock with Timeout
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
public class TimeoutLockExample {
private final Lock lock = new ReentrantLock();
public void safeMethod() {
boolean acquired = lock.tryLock();
try {
if (acquired) {
// Critical section
} else {
// Handle lock acquisition failure
}
} finally {
if (acquired) {
lock.unlock();
}
}
}
}
Here, the tryLock
method attempts to acquire the lock without waiting, reducing the risk of deadlocks in systems with many virtual threads.
Issue 3: Handling Interruptions and Cancellation
Virtual threads can be interrupted just like platform threads, but due to their sheer number and quicker lifecycle, handling interruptions can be tricky.
Solution: Proper Interruption Handling
Make sure interruption policies are consistently applied and that the interrupt status of threads is checked regularly during execution to allow for graceful completion or cleanup.
Code Snippet: Handling Interruptions
public class InterruptionHandlingExample {
public void performTask() {
while (!Thread.currentThread().isInterrupted()) {
try {
// Task code that may throw InterruptedException
} catch (InterruptedException e) {
// Restore the interrupted status
Thread.currentThread().interrupt();
// Break out of the loop or perform cleanup
return;
}
}
}
}
In this function, we are constantly checking the interrupted status of the current thread, ensuring that the task can respond to interruptions appropriately.
Issue 4: Monitoring and Debugging
Given the potential high number of virtual threads, traditional monitoring and debugging tools may not cope well, leading to difficulties in observing system behavior.
Solution: Utilize Enhanced Tooling for Virtual Threads
Adopt new or updated tools that have been designed or improved to handle the intricacies of monitoring and debugging applications that use virtual threads heavily.
No specific code snippet is provided for this issue, as solutions largely depend on the tooling and monitoring systems available, such as updated versions of VisualVM or JConsole that support virtual threads.
Conclusion
Java 19's virtual threads are a powerful tool for simplifying concurrent programming and maximizing resource utilization. However, as we've seen, they come with their own set of challenges. By learning to identify issues such as resource starvation, deadlocks, difficult interruption handling, and monitoring challenges, and applying the provided solutions and coding patterns, you'll be well-equipped to tackle these obstacles with confidence.
For more about Java 19 and virtual threads, visit the official Oracle documentation. As Java continues to grow and evolve, staying up-to-date is crucial for any developer looking to maximize their efficiency and the performance of their applications.
Happy coding!