Stop Bugs Cold: Java Devs Must-Have Persistent Breakpoints
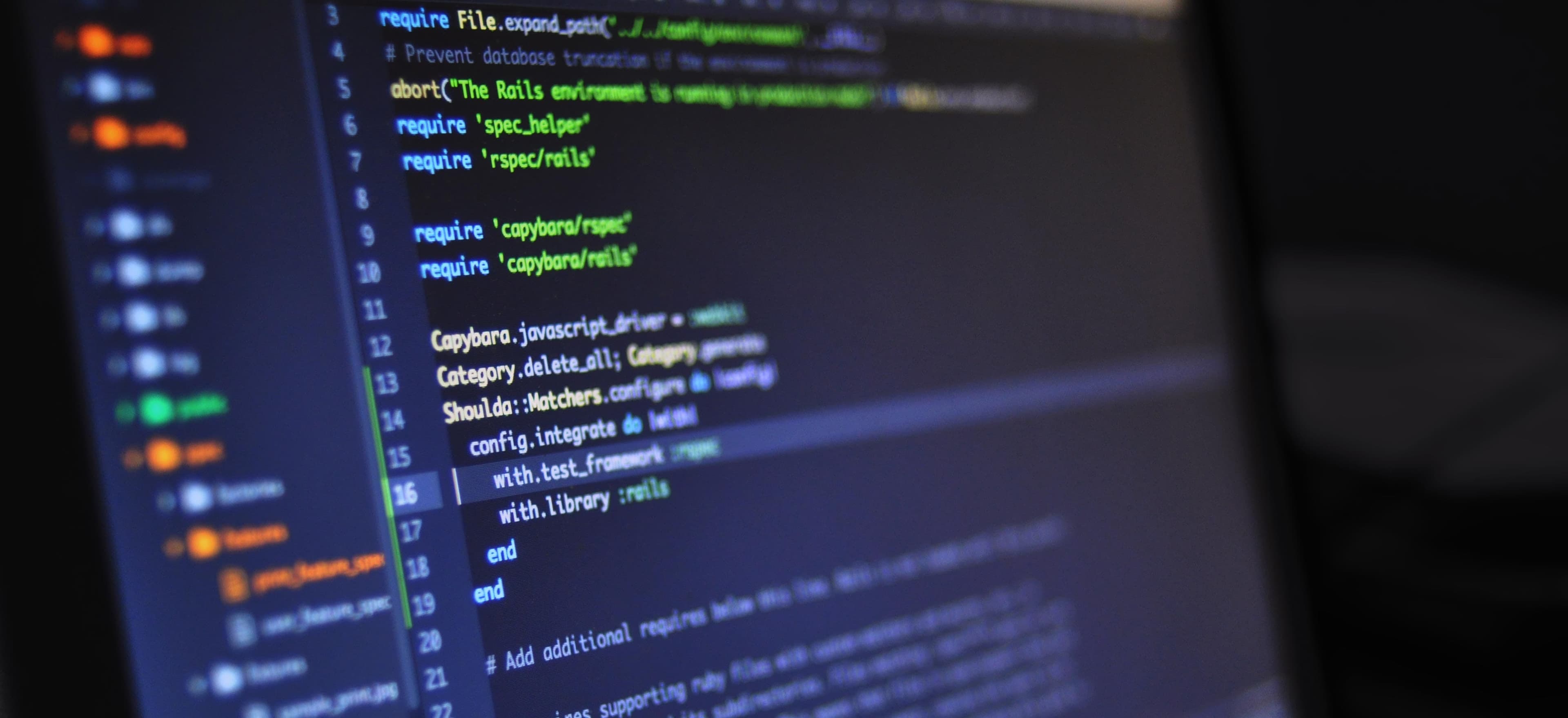
- Published on
Stop Bugs Cold: Java Devs Must-Have Persistent Breakpoints
In the quest to develop flawless applications, Java developers often find themselves in a chaotic cycle of coding, testing, and debugging. One of the most potent weapons in a Java developer's arsenal to combat relentless bugs is the art of using persistent breakpoints.
But what sets persistent breakpoints apart in the debugging landscape? How can Java devs leverage this feature to streamline their workflow and squash bugs more efficiently? In this post, we will delve into the significance of persistent breakpoints in Java debugging and provide actionable insights to harness their full potential.
What are Persistent Breakpoints?
When we talk about breakpoints, we refer to deliberate pause points in the execution of a program, set by developers within their Integrated Development Environment (IDE) or debugger. These are not just any hiatus in your code; they are strategic stops that allow developers to inspect the state of an application at specific code locations. Persistent breakpoints, in essence, are breakpoint settings that remain active across IDE restarts or even system reboots.
Why Persistent Breakpoints Matter
Imagine this: You're deep into a debugging session, you have breakpoints scattered throughout your monolithic application, variables monitored at each turn, and then it happens — an unexpected IDE crash or a required system update forces everything to halt. If your breakpoints vanish, you are back to square one — a dire situation that persistent breakpoints can prevent.
By ensuring that breakpoint settings stick around, developers can:
- Save valuable time setting up debugging environments
- Maintain mental focus on solving the problem at hand
- Establish a consistent routine for iterative debugging
- Facilitate collaboration with team members by sharing breakpoints
- Inhibit loss of context in the event of interruptions
Implementing Persistent Breakpoints in Java
Most modern Java IDEs like IntelliJ IDEA and Eclipse come with built-in support for persistent breakpoints. Here's a step-by-step guide on how to use this feature in popular Java IDEs:
Using Persistent Breakpoints in IntelliJ IDEA
In IntelliJ IDEA, setting up persistent breakpoints is as easy as clicking the left gutter next to the line of code where you want your breakpoint. IntelliJ IDEA takes care of the rest, ensuring your breakpoints persist across sessions by default.
public class BugHunter {
public static void main(String[] args) {
// Begin iterating from 1 to 10
for (int i = 1; i <= 10; i++) {
int result = processNumber(i);
System.out.println("Processed: " + result);
}
}
// Process and return the number
private static int processNumber(int number) {
// Set a persistent breakpoint here to inspect 'number' value
return number * 2;
}
}
In the above code snippet, by placing a persistent breakpoint at the line return number * 2;
, you can examine the value of number
every time the method processNumber
is called.
Using Persistent Breakpoints in Eclipse
Eclipse also supports persistent breakpoints right out of the box. To set a breakpoint in Eclipse, you simply double-click the left margin next to the code line, or right-click and select "Toggle Breakpoint."
public class CodeSleuth {
public static void detectBug(int[] data) {
// Potential bug when processing data array
for (int datum : data) {
if (datum < 0) {
// Breakpoint that remains even after IDE restarts
System.out.println("Data error: negative value found.");
}
}
}
}
With a persistent breakpoint inside the if
statement, if your application unexpectedly encounters a negative value in the data
array, the breakpoint will trigger, allowing you to halt the program and investigate the issue.
Maximizing Efficiency with Breakpoint Management
Persistent breakpoints are a critical feature, but managing them effectively is equally important. Here are some tips to maximize the efficiency of your debugging process with breakpoint management:
-
Organize Breakpoints: Assign descriptive names to your breakpoints and group them meaningfully. Both IntelliJ IDEA and Eclipse allow you to make these adjustments.
-
Enable/Disable in Bulk: Learn how to quickly enable or disable groups of breakpoints. Sometimes you want to ignore certain breakpoints without deleting them completely.
-
Use Conditions: Setting conditional breakpoints can be game-changing. They will only halt the execution when the specified condition is true, thereby avoiding unnecessary stops.
-
Export/Import Breakpoints: Share your breakpoints with team members to synchronize debugging efforts. Both IntelliJ IDEA and Eclipse provide options to export breakpoints which can then be imported by other team members.
-
Integrate with Version Control: Keeping your breakpoints under version control can be beneficial, especially when they are tied to specific issues or tickets. Use your IDE's version control system (VCS) integration to keep track of breakpoints changes on a project level.
Best Practices for Debugging with Persistent Breakpoints
Alongside persistent breakpoints, here are some best practices to follow for Java debugging:
- Keep Breakpoints Minimal: Only set breakpoints where you truly need them. Excessive breakpoints can clutter your workflow and make debugging more complex than it needs to be.
- Document Reasoning: Whenever you add a breakpoint, comment on why it's there. This practice helps prevent confusion, especially in collaborative environments.
- Use Version Control Wisely: Regularly commit your debugging progress, including adding, updating, or removing breakpoints, to avoid losing important changes.
- Stay Updated: Keep your Java Development Kit (JDK) and IDE up-to-date to take advantage of the latest debugging features.
In Conclusion
Persistent breakpoints are a Java developer's safeguard against time wastage and frustration when tracking down elusive bugs. By adopting an intelligent approach to debugging with persistent breakpoints — alongside diligent management and best practices — Java developers can master the art of bug hunting, maintain a seamless coding experience, and ensure their applications perform exceptionally in the wild.
Remember, the next time you find yourself delving into a complex Java application littered with troublesome bugs, persistent breakpoints could very well be the linchpin that transforms a tedious debug session into a swift and triumphant bug resolution. Embrace persistent breakpoints, and stop those bugs cold!
Checkout our other articles