Troubleshooting QRGen: Fixing Java QR Code Woes
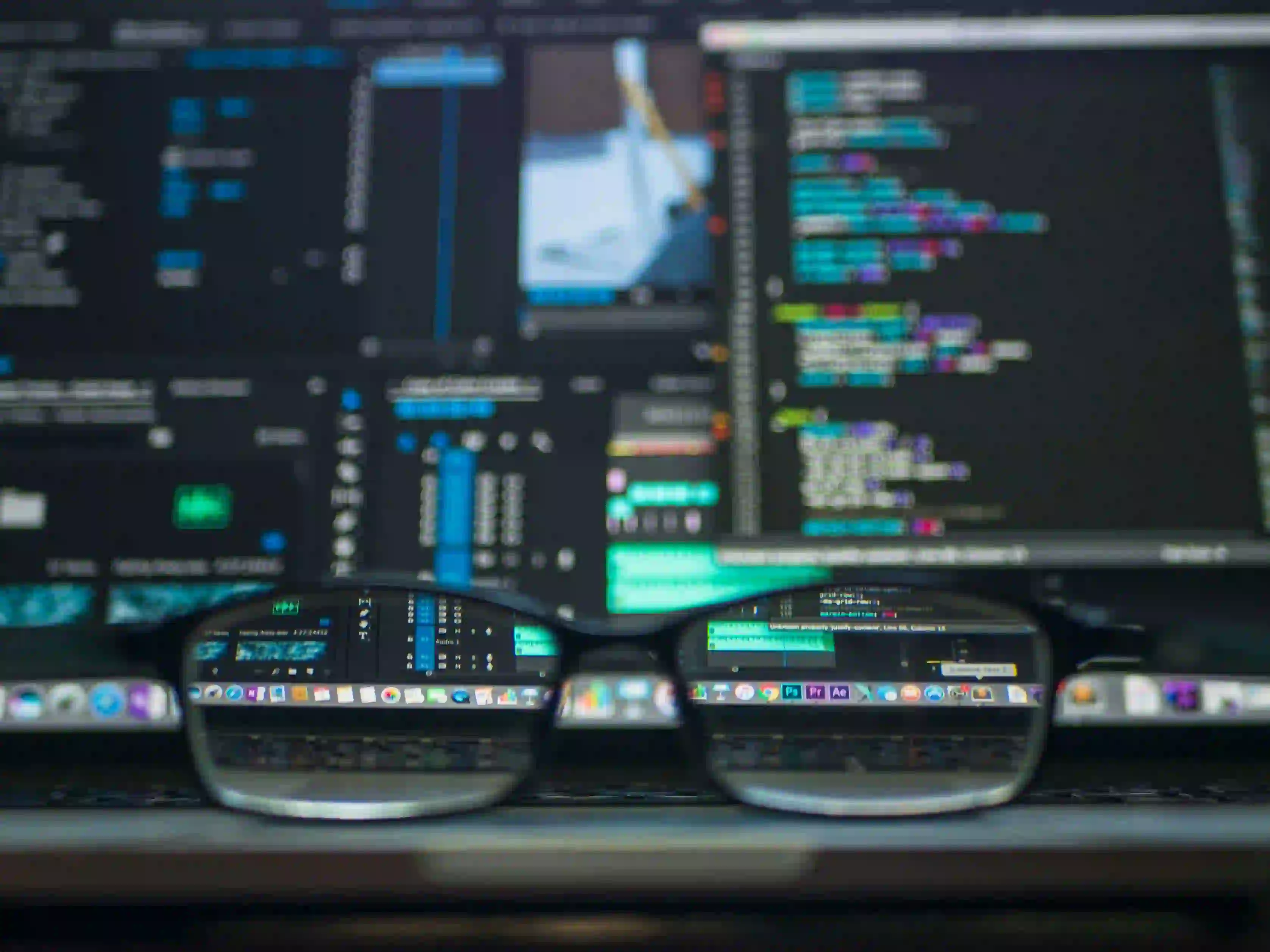
Troubleshooting QRGen: Fixing Java QR Code Woes
QR codes have become an integral part of our digital lives, seamlessly linking the physical world with the virtual. They store information in a machine-readable format that can be scanned and interpreted by mobile devices. As a Java developer, generating QR codes can be a frequent requirement for projects. And when you face issues with QR code generation in Java, it can lead to frustration and delays. In this post, we'll address common problems encountered while using the QRGen library to generate QR codes in Java, providing clear solutions to get your project back on track.
The Basics of QRGen
QRGen is a simple library for QR code generation in Java, built on top of the popular ZXing ("Zebra Crossing") library. It simplifies creating QR codes to just a few lines of code, but with simplicity can come confusion when things don't work as expected. Here's how you can generate a QR code with QRGen:
import net.glxn.qrgen.javase.QRCode;
public class QRCodeGenerator {
public static void main(String[] args) {
QRCode.from("https://www.example.com").file();
}
}
This basic code will create a QR code that, when scanned, will direct the user to https://www.example.com
. But what if your QR code doesn't scan, or what if you encounter an error during the generation process? Let's troubleshoot some common issues.
Issue 1: QR Code Doesn't Scan
Check Your QR Code Content
Make sure the content you're embedding in the QR code is correct and scan-friendly:
- URLs must be valid and prefaced with
http://
orhttps://
. - Text should not be overly long, as it can make the code dense and hard to scan.
- Formats like vCard need to adhere to the standard vCard format.
Adjust the Size
If your QR code isn't scanning, the size may be too small or too large. QRGen allows you to specify a size:
QRCode.from("https://www.example.com").withSize(250, 250).file();
Ensure Adequate Contrast
Ensure that there's sufficient contrast between the QR code and the background. A common practice is to have a dark color for the QR blocks on a light background.
Issue 2: Errors in Generation
Handle Exceptions
QR code generation might throw exceptions. Always wrap your code in a try-catch block:
try {
QRCode.from("https://www.example.com").file();
} catch (Exception e) {
e.printStackTrace(); // Handle the exception appropriately
}
Check Dependencies
Ensure that you have the correct and all necessary dependencies loaded in your project. An incomplete classpath can lead to runtime errors.
Issue 3: QR Code Quality Issues
Image Format
The default image format is PNG, which is ideal for QR codes. However, if you need to change it, QRGen supports different formats:
QRCode.from("https://www.example.com").to(ImageType.JPG).file();
Margin Specification
A QR code should have a margin to be scanned correctly. You can specify this using QRGen:
QRCode.from("https://www.example.com").withSize(250, 250).withMargin(10).file();
Error Correction Level
Sometimes you need to set an error correction level for the QR code, especially if it will be printed and subjected to wear and tear:
import net.glxn.qrgen.core.scheme.VCard;
VCard johnDoe = new VCard("John Doe")
.setEmail("john.doe@example.com")
.setAddress("123 Main Street, Anytown, USA")
.setTitle("Mr")
.setCompany("John Doe Inc.")
.setPhoneNumber("1234")
.setWebsite("www.example.com");
QRCode.from(johnDoe).withCharset("UTF-8").withErrorCorrection(ErrorCorrectionLevel.H).file();
The ErrorCorrectionLevel.H
is the highest level of error correction, providing the most resilience against errors.
Advanced QRGen Features
So far, we've discussed troubleshooting when things go wrong. Now, let's explore some advanced features that QRGen offers, which you can use to enhance your QR codes.
Styling QR Codes
Beyond just encoding information, you can style QR codes. QRGen allows you to change colors:
QRCode.from("https://www.example.com").withColor(0xFF0000, 0xFFFFFF).file();
Overlay Images
Sometimes, including a logo or icon at the center of your QR code is necessary for branding purposes. QRGen's overlay functionality makes this possible:
File file = QRCode.from("https://www.example.com").withSize(250, 250)
.overlay(new File("path/to/overlay.png")).file();
Saving to Specific Paths
By default, QRGen saves QR codes to your project directory. To specify a save path:
File file = QRCode.from("https://www.example.com").file("QRCode.png");
Conclusion
QR codes serve as bridges to digital worlds and must be generated accurately. QRGen is an excellent library for Java developers to create QR codes, but troubleshooting is an essential skill when you hit a snag. Addressing QR code scanning problems, generation errors, and quality concerns are just a few aspects that developers must be equipped to handle.
When you fully understand the hows and whys of QR code generation, and remember to incorporate exception handling, validate your content, and pay attention to image quality specifics, you'll be able to create robust QR codes with QRGen that function reliably in a wide range of environments. Future-proof your QR code generation with these insights, and ensure your QR codes work as intended every time.
Stay tuned for more content on Java and development best practices. Happy coding!