Stop Spam: Master Email Filtering with AspectJ & Spring
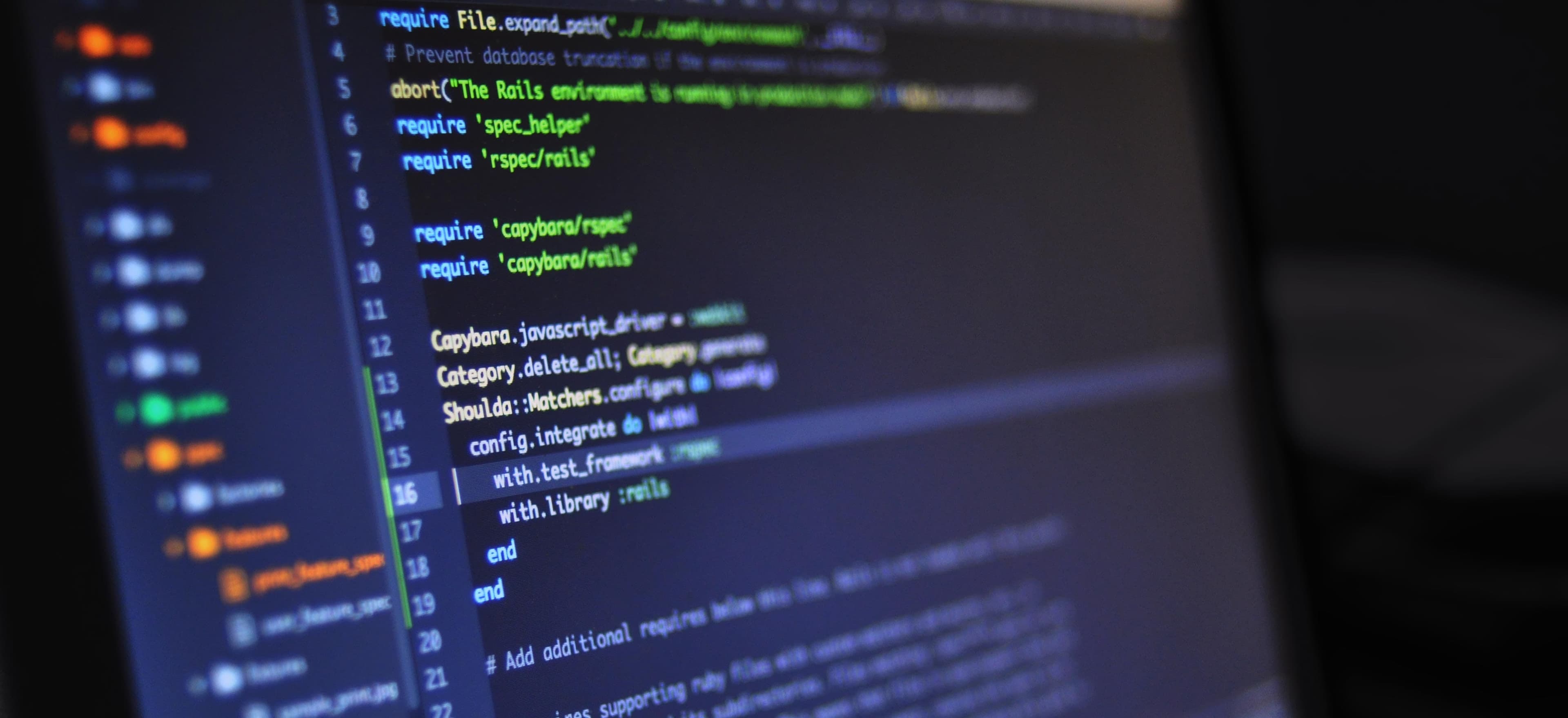
- Published on
Stop Spam: Master Email Filtering with AspectJ and Spring
In the digital era, our inboxes are often inundated with uninvited guests—spams that not only cause frustration but also threaten our cybersecurity. As developers and engineers, creating robust email filtering systems is no longer just a feature; it's a necessity. In this post, we'll dive deep into integrating AspectJ with Spring, harnessing the power of Aspect-Oriented Programming (AOP) to craft a spam filter that can fortify your application's email functionality.
The Role of AspectJ in Email Filtering
AspectJ, an aspect-oriented extension to Java, seeks to modularize concerns such as logging, security, or transaction management, that cut across multiple types of objects and components. Spam filtering is a cross-cutting concern because it's applicable wherever emails are sent or received, irrespective of the business logic.
AOP complements Object-Oriented Programming (OOP) by allowing us to intercept method calls (advice), react to object changes (join points), and encapsulate behaviors that impact multiple classes (aspects). This is pivotal for email filtering as it minimizes code intrusion, allowing us to maintain clean and single-purpose classes while still elegantly handling spam.
Getting Started with AspectJ and Spring
Before we write any code, ensure you have the following setup:
- JDK 1.8 or later
- Maven or Gradle (for dependency management)
- Spring Framework (we'll use Spring Boot for simplicity)
Crafting the Email Filter Aspect
Here's how you can create an Aspect for email filtering:
Pom.xml Configuration (Maven)
Add AspectJ and Spring AOP dependencies to your pom.xml
:
<dependencies>
<!-- Other dependencies -->
<!-- Spring AOP + AspectJ -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-aop</artifactId>
</dependency>
<dependency>
<groupId>org.aspectj</groupId>
<artifactId>aspectjweaver</artifactId>
</dependency>
</dependencies>
Gradle Configuration
For Gradle users:
dependencies {
// Other dependencies
// Spring AOP + AspectJ
implementation 'org.springframework.boot:spring-boot-starter-aop'
implementation 'org.aspectj:aspectjweaver'
}
Defining the Aspect
Create an aspect that will handle the email filtering logic:
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Before;
import org.springframework.stereotype.Component;
@Aspect
@Component
public class EmailFilterAspect {
@Before("execution(* com.yourapp.service.EmailService.sendEmail(..)) && args(emailAddress, ..)")
public void beforeEmailSent(String emailAddress) {
if (isSpam(emailAddress)) {
throw new RuntimeException("Detected spam address. Aborting email send.");
}
}
private boolean isSpam(String emailAddress) {
// Implement your spam detection logic here
return emailAddress.contains("spam");
}
}
Why This Code?
The @Aspect
annotation denotes that this is an aspect class. The @Component
makes it a Spring-managed bean, and the @Before
advice indicates the action to take place before the sendEmail
method of EmailService
is executed. It checks for spam using the isSpam
method and if true, prevents the email from being sent by throwing an exception.
Let's dissect the @Before
annotation's expression:
execution(* com.yourapp.service.EmailService.sendEmail(..))
: This pointcut expression matches the execution of thesendEmail
method inEmailService
class within your application.args(emailAddress, ..)
: This binds the first argument of thesendEmail
method to theemailAddress
parameter of the before advice, allowing us to use it within our aspect method.
Enhancing Spam Detection
The basic isSpam
function above merely checks if the word "spam" is included in the email address, which is a simplistic strategy. For more sophistication, integrate with a real-time database of known spammers, apply regex patterns, or even machine learning models to predict the probability of spam.
Integrating the Aspect with Email Service
Now, let's wire up our aspect with an email service:
package com.yourapp.service;
import org.springframework.stereotype.Service;
@Service
public class EmailService {
public void sendEmail(String emailAddress, String content) {
// Email sending logic
System.out.println("Email sent to " + emailAddress);
}
}
In this snippet, EmailService
serves as a standard service class in a Spring application. When the sendEmail
method is called, the EmailFilterAspect
comes into play, executing before the email is sent.
AspectJ’s Power for Email Filtering
Leveraging the power of AspectJ within a Spring application provides several benefits for email filtering:
- Modularity: Keeps the spam detection logic decoupled from core business logic.
- Maintainability: Changes to the aspect apply across all points where it's woven, making maintenance simpler.
- Separation of Concerns: Helps clearly define the bounds between spam filtering and other application concerns.
Conclusion
AspectJ and Spring together create a formidable alliance in combatting the perpetual issue of spam. By designing an adept email filter aspect, applications remain secure, maintainable, and free from the bloat of cross-cutting concerns interwoven with core functionalities.
For further elaboration on AspectJ and its offerings, explore the AspectJ Programming Guide. To delve deeper into the Spring AOP, the Spring AOP Documentation is an invaluable resource.
By embedding cross-cutting concerns within aspects, separating them from your business logic allows for more readable, efficient, and elegant codebases. Take the plunge into AspectJ and Spring AOP to safeguard your applications from spam and ensure a secure, user-friendly email experience!
Next Steps
For readers eager to continue expanding their knowledge:
- Experiment with advanced spam detection algorithms.
- Integrate with external services for dynamic spam databases.
- Consider rate limiting to deter spamming behavior at an API level.
Embark on the journey; your clean, spam-free inbox awaits!
Checkout our other articles