Securing Java: Avoid Common Animation Vulnerabilities
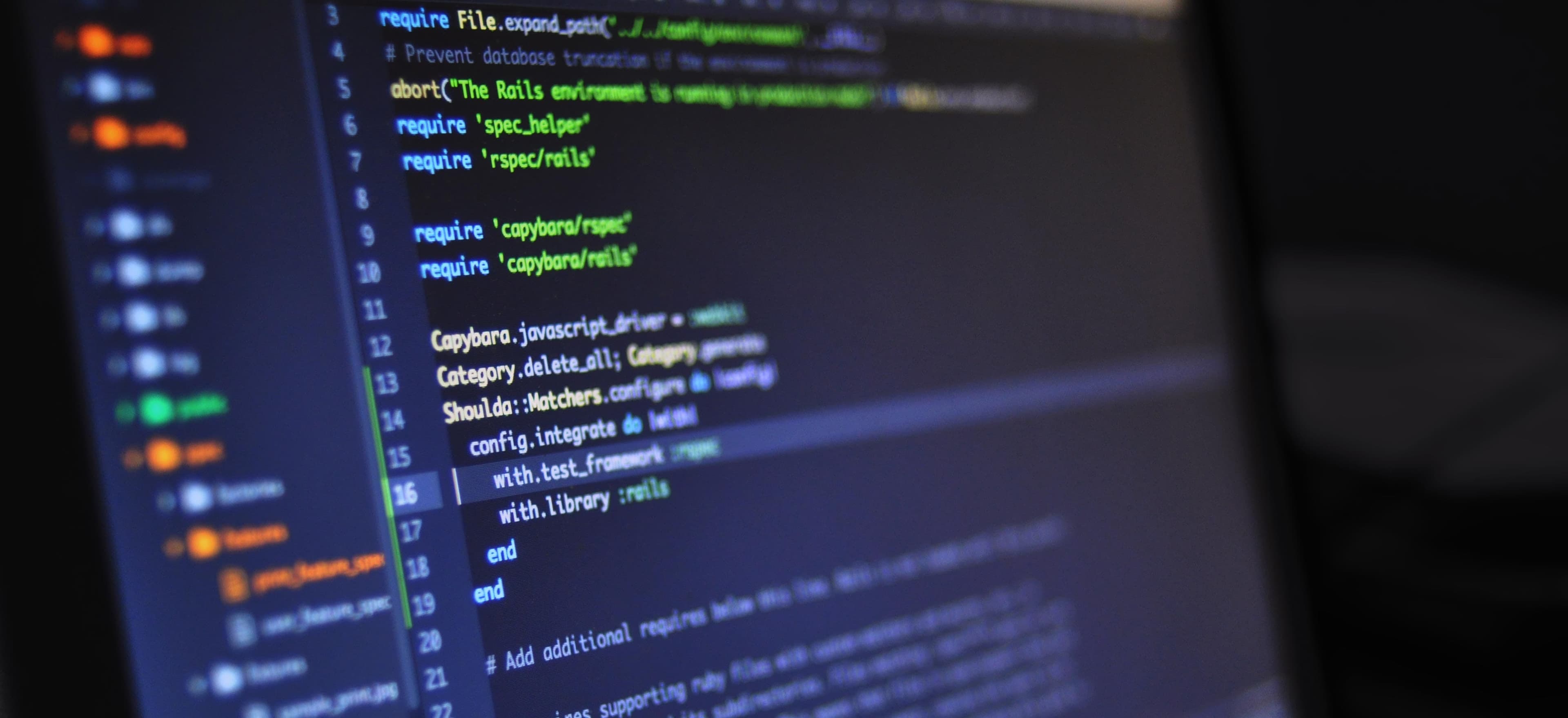
- Published on
Securing Java: Avoid Common Animation Vulnerabilities
Java applications are robust and can be made incredibly secure, but as developers, we must be vigilant against the myriad of vulnerabilities that could be exploited by attackers. Today, we're taking a deep dive into one type of vulnerability that's less often discussed but no less dangerous: animation vulnerabilities. In this post, we’ll explore what they are, why they matter, and how you can protect your Java applications from being compromised through animation exploits.
Understanding Animation Vulnerabilities
Animation vulnerabilities occur when animating graphics, such as in video games or interactive UI components, opens the door to potential attacks like buffer overflows, race conditions, or issues with memory management that can compromise the security of Java applications. Java's rich set of animation APIs in libraries such as JavaFX or third-party libraries can inadvertently introduce security holes if not used properly.
Why Focus on Animation Security?
While animations might seem innocuous, insecure animation handling can lead to a host of security issues including:
- Information Disclosure: Leaking sensitive user information through animation timing or state.
- Denial of Service (DoS): Overloading the system by forcing it to render complex animations repeatedly.
- Remote Code Execution (RCE): Exploiting buffer overflow vulnerabilities to execute arbitrary code.
Let's discuss some best practices and coding strategies to mitigate these risks.
Secure Coding Practices with Java Animations
1. Validate Input Data
Always validate input data when animations are based on user-driven events or data streams. Here's how to do it effectively:
private void validateAnimationData(AnimationData data) {
if (data.getFrameCount() < 0 || data.getFrameRate() <= 0) {
throw new IllegalArgumentException("Invalid animation data provided");
}
// Additional validation logic...
}
Why? By ensuring that animation data follows expected parameters, you prevent many common vulnerabilities related to incorrect or malicious input.
2. Employ Safe Memory Management
Java handles memory allocation and garbage collection for you, but when using native libraries with your animations, you must be careful:
try (
var memorySegment = MemorySegment.allocateNative(animationSize)
) {
AnimationInterface.startAnimation(memorySegment);
} catch(OutOfMemoryError e) {
// Handle memory error
}
Why? Explicitly managing the life cycle of native resources helps prevent memory leaks and buffer overflows.
3. Avoid Race Conditions with Thread Synchronization
Ensure animation state changes occur in a thread-safe manner to avoid race conditions:
public class ThreadSafeAnimator {
private final Object lock = new Object();
private AnimationState state;
public void changeState(AnimationState newState) {
synchronized(lock) {
state = newState;
}
}
}
Why? Synchronizing state changes prevents multiple threads from causing inconsistent animation states.
4. Limit Animation Resources
Avoid DoS attacks by limiting the resources an animation can use:
public class ResourceLimitedAnimation extends Animation {
// Maximum allowed duration for the animation
private static final Duration MAX_DURATION = Duration.ofSeconds(10);
public ResourceLimitedAnimation(Duration duration) {
if (duration.compareTo(MAX_DURATION) > 0) {
throw new IllegalArgumentException("Animation duration exceeds maximum limit.");
}
// Set up the animation...
}
}
Why? Limiting resources constrains animation usage and prevents the abuse of animation features to degrade application performance.
5. Update Libraries and Dependencies
Frequently update your Java libraries and dependencies to patch known vulnerabilities:
Why? Using the latest versions protect your applications from known security issues that have been fixed by maintainers.
6. Employ Proper Exception Handling
Catch and handle animation-specific exceptions to prevent information leakage and maintain application flow:
try {
runAnimation();
} catch (AnimationException e) {
log.error("Animation error occurred", e);
// Gracefully degrade animation or notify the user...
}
Why? Properly handling exceptions prevents attackers from gleaning system information from unhandled error messages and ensures that users are not left with a broken application experience.
7. Use Secure Development Tools
Incorporate security-focused development tools into your workflow:
Why? These tools help catch security vulnerabilities early in the development process, greatly reducing the risk of introducing security flaws into the production code.
Real-World Example: Securing JavaFX Animations
Now, let's apply these principles to a JavaFX animation to see how we can secure it against common animation vulnerabilities:
public class SecureFadeAnimation extends FadeTransition {
public SecureFadeAnimation(Duration duration, Node node) {
super(duration, node);
if (node == null) {
throw new IllegalArgumentException("Target node cannot be null.");
}
setCycleCount(2);
setAutoReverse(true);
setOnFinished(this::animationFinished);
}
private void animationFinished(ActionEvent event) {
System.out.println("Animation completed securely.");
// Additional handling after animation completion...
}
}
In this JavaFX example, the SecureFadeAnimation
class is designed with security in mind:
- Input validation: The constructor throws an
IllegalArgumentException
if the targetnode
is null. - Limited Resources: The
setCycleCount()
method ensures the fade animation only runs a set number of times. - Exception Handling: The
animationFinished
method provides a way to handle completion events, which can include error logging or alternative actions if the animation fails.
Conclusion
Security is paramount in the world of software development, and animation handling is no exception. While Java provides many tools to help automate memory management and other potential pitfalls, it is ultimately the responsibility of you, the developer, to write code that protects against exploitation.
Remember, the path to secure Java applications starts with an awareness of potential vulnerabilities, followed by a commitment to implementing best practices such as input validation, resource limitation, and up-to-date libraries. Combine these practices with secure coding techniques, and you have a robust defense against the unseen dangers lurking in the seemingly innocuous world of animations.
Keep learning, keep coding securely, and your Java applications will not only perform beautifully but will also safeguard your users' data against potential threats. Stay tuned for more insights into securing Java applications in future posts. Happy coding!
Note: The code samples provided in this blog post are illustrative and may require additional context or dependencies to function as intended in a production environment.