JCLAP 101: Debugging Common Java CLI Parsing Issues
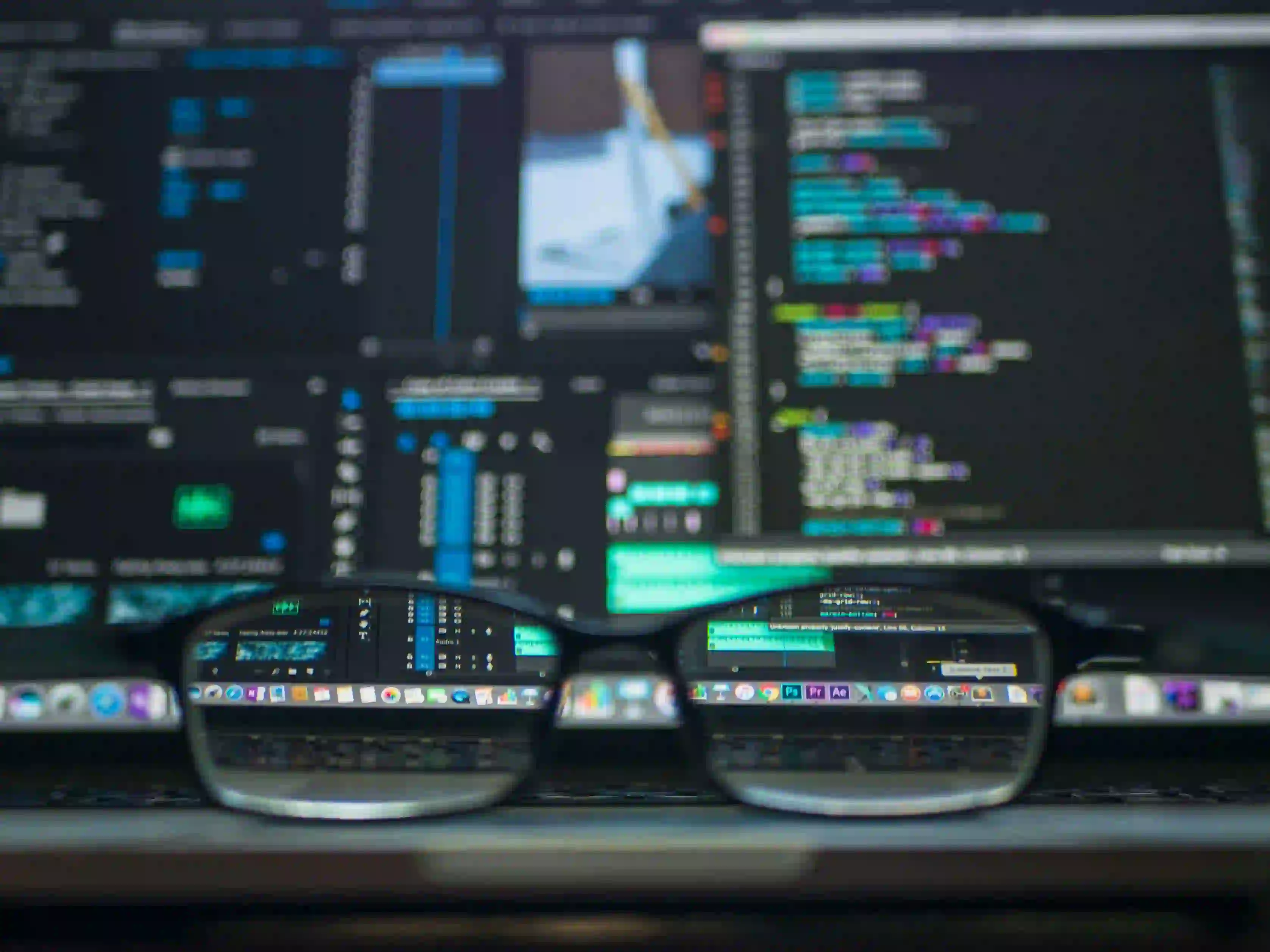
JCLAP 101: Debugging Common Java CLI Parsing Issues
Creating a seamless command-line interface (CLI) is a critical step for many Java applications. For developers and end-users alike, efficient and bug-free CLIs add immense value to the software utilization experience. Enter Java Command-Line Argument Parser (JCLAP), a tool designed to streamline the process. However, with the intricacies of command-line parsing, issues are almost inevitable. Let's deep-dive into the art of debugging common Java CLI parsing issues using JCLAP.
Understanding JCLAP
JCLAP is a handy library in Java aimed at simplifying the parsing of command-line arguments for developers. It abstracts the complexities involved in interpreting the CLI input, providing a straightforward API for both simple and advanced parsing needs.
When implementing JCLAP, you're likely to encounter a series of common issues, such as incorrect argument types, missing required arguments, and mishandled default values. In this post, we'll tackle these problems, providing actionable solutions that guarantee a robust CLI experience for your Java application.
Delving into Argument Types
One key aspect of Java CLI parsing is ensuring that argument types are correctly identified and processed. With JCLAP, each option should be associated with the proper handler for the type of data expected. Mistakes here often lead to ClassCastException
or NumberFormatException
.
OptionParser parser = new OptionParser();
parser.addOption(new Option("p", "port", true, "Listen on provided port.", "Integer"));
In the above snippet, we're telling JCLAP that the port
option expects an integer value.
Why this matters: Specifying the argument type enables JCLAP to cast the provided argument to the desired data type, triggering a built-in validation mechanism.
Enforcing Required Arguments
Often, your application will have certain non-negotiable arguments without which it cannot function. JCLAP allows you to mark these arguments as required.
Option requiredOption = new Option("c", "config", true, "Path to configuration file.", "String");
requiredOption.setRequired(true);
parser.addOption(requiredOption);
Why this is crucial: Failure to enforce required arguments will leave your code vulnerable to NullPointerException
s and confusing behavior when the user omits critical arguments.
Handling Default Values
Not every argument fed to your Java CLI is critical, and some can safely assume default values. Defining these fallbacks is a best practice to ensure user convenience and program stability.
Option optionWithDefault = new Option("l", "loglevel", true, "Set the logging level.", "String");
optionWithDefault.setDefaultValue("WARN");
parser.addOption(optionWithDefault);
We set the default logging level to WARN
, safeguarding the program from unexpected verbosity or silence.
Why default values matter: They ensure that your application behaves predictably even when users do not provide all arguments, striking the right balance between flexibility and control.
The Parsing Dance
With everything in place, it's time to parse and act upon the command-line arguments.
try {
CommandLine commandLine = parser.parse(args);
// Act on the parsed arguments
} catch (ParseException e) {
System.err.println(e.getMessage());
parser.printUsage();
System.exit(1);
}
Why catching exceptions is vital: CLI parsing is fraught with human error, and we must handle these situations gracefully, providing helpful feedback to the user.
Common CLI Parsing Issues and Their Solutions
-
Unrecognized options: This occurs when the parser encounters an argument that has not been defined. Ensure that all possible inputs are well-defined and consider using the
HelpFormatter
class to guide users. -
Missing required options: A clear error message indicating which required option is missing can guide the user. This happens when the
required
property is rightfully set, but the user failed to provide the value. -
Argument value type mismatch: Provide comprehensive usage guidelines and validate input types before parsing to prevent
ClassCastException
. Always specify the type expected in youroption
constructors. -
Missing argument values: Detecting when an option that requires an argument is provided without one is key. Prompt the user with a proper error message, indicating the correct format.
-
Unexpected exceptions: Whenever an unanticipated error occurs, log the exception details and provide a relevant message to aid in debugging.
Conclusion
Java command-line parsing doesn't have to be daunting. With JCLAP and a robust error-handling mechanism, you can prevent and gracefully handle the common pitfalls that developers and users frequently encounter.
Remember to specify argument types carefully, enforce mandatory parameters, provide sensible defaults, and parse arguments vigilantly, catching exceptions to offer productive feedback. With these practices, you'll elevate your Java CLI to a professional level, ensuring a smooth interaction for everyone involved.
Advance your knowledge: Explore the JCLAP Documentation for more insights and advanced usage patterns. Additionally, familiarize yourself with the Java CLI Best Practices to further refine your cli applications.
Keep debugging smart, and your Java CLI will be the paragon of efficiency and reliability!