Optimizing Spring Profiles: Streamlining Configuration for Different Environments
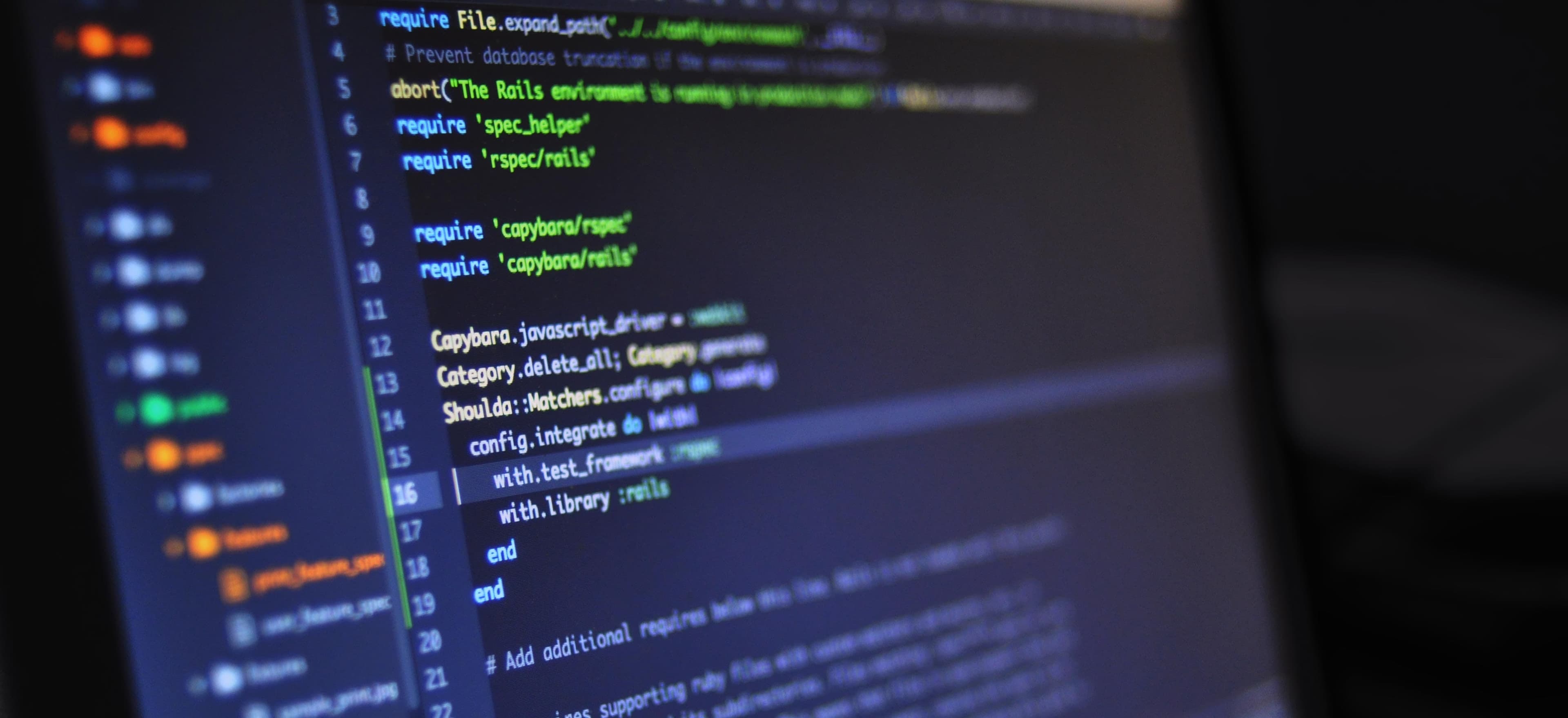
- Published on
Optimizing Spring Profiles: Streamlining Configuration for Different Environments
In a complex software system, it is imperative to have different configurations for different environments such as development, testing, and production. Spring Framework provides a powerful feature called Spring Profiles, which allows us to define different sets of configuration properties based on the environment we are running our application in.
With Spring Profiles, we can easily switch between different configurations using a simple configuration file or command-line argument. This flexibility enables us to streamline our application's configuration for different environments, leading to cleaner and more efficient code.
In this blog post, we will explore how to optimize Spring Profiles to streamline configuration for different environments, and how it can improve the overall development and deployment process of a Java application.
What are Spring Profiles?
Spring Profiles, introduced in Spring Framework 3.1, are a way to define multiple configurations for different environments in a Spring application. A profile represents a specific environment, such as "dev", "test", or "prod", and contains a set of configuration properties.
By using Spring Profiles, we can easily create different configurations for different environments without duplicating configuration code. This allows us to have separate configurations for development, testing, and production, ensuring that our application runs smoothly in each environment.
Configuring Spring Profiles
To start using Spring Profiles, we need to configure our application to recognize and activate the appropriate profile based on the environment it is running in. There are multiple ways to configure Spring Profiles in a Spring application.
1. Using the spring.profiles.active
Property
One way to activate a specific profile is by setting the spring.profiles.active
property in our application's configuration file. This property specifies the active profile for our application.
spring.profiles.active=dev
In the above example, we have set the active profile to dev
. This means that when we run our application, Spring will load all the configuration properties defined for the dev
profile.
2. Using Command-Line Arguments
Another way to activate profiles is by using command-line arguments when starting our application. We can use the -Dspring.profiles.active
argument followed by the desired profile name.
java -jar myapp.jar -Dspring.profiles.active=dev
By specifying this argument, we can override the property defined in the configuration file and activate the desired profile during the application startup.
3. Using the @Profile
Annotation
In addition to the above methods, we can also use the @Profile
annotation to selectively enable or disable beans based on the active profiles.
@Configuration
public class MyConfiguration {
@Bean
@Profile("dev")
public DataSource devDataSource() {
// Create and configure the data source for the development environment
}
@Bean
@Profile("prod")
public DataSource prodDataSource() {
// Create and configure the data source for the production environment
}
}
In the above example, we have defined two beans, devDataSource
and prodDataSource
, each annotated with @Profile
. The devDataSource
bean will only be created and injected when the dev
profile is active, while the prodDataSource
bean will only be created and injected when the prod
profile is active.
By using the @Profile
annotation, we can easily segregate our beans based on the active profiles, providing a clean and modular configuration for different environments.
Benefits of Using Spring Profiles
Now that we understand how to configure Spring Profiles, let's discuss the numerous benefits they provide.
Simplified Configuration Management
Spring Profiles simplify configuration management by providing an elegant way to handle different environment-specific configurations. By organizing our configuration properties into separate profiles, we can easily manage and modify them without affecting other environments. This improves maintainability and reduces the risks associated with configuration changes.
Environment-Specific Customization
With Spring Profiles, we can customize our application's behavior based on different environments. For example, we can define slower log levels for debugging purposes in the development environment, while enabling a higher log level for production to catch potential issues early on. Additionally, we can configure different databases, external service URLs, or any other environment-specific settings based on the active profile.
This level of customization allows us to optimize our application for each environment, leading to more efficient development and testing cycles.
Easy Testing and Debugging
Spring Profiles make testing and debugging easier by providing a mechanism to isolate configurations and simulate different environments. We can easily switch between profiles during testing to ensure that the application behaves correctly in each environment. This ability to quickly switch profiles facilitates faster debugging and makes it easier to reproduce and fix issues.
Efficient Builds and Deployments
By segregating configurations using Spring Profiles, we can eliminate unnecessary code and effectively reduce the size and complexity of our application during builds and deployments. This results in faster, more efficient builds and deployments.
For example, we can exclude test-related configurations when packaging the application for production. Similarly, we can exclude development-specific configurations when deploying to the production environment. This allows us to optimize resource usage and minimize the attack surface of our application.
Improved Collaboration
Spring Profiles enable improved collaboration among team members working on different environments. Each team member can work with their own profile without interfering with others. This ensures smoother collaboration and reduces potential conflicts arising from environment-specific configurations.
Best Practices for Optimizing Spring Profiles
To get the most out of Spring Profiles, it is important to follow some best practices. Let's discuss a few of them:
Clear and Consistent Profile Naming
When creating profiles, it is essential to use clear and consistent naming conventions. Clear naming helps in understanding and maintaining the codebase, while consistent naming conventions make it easier to navigate and manage profiles across different projects.
Using commonly accepted names like "dev", "test", and "prod" for standard environments ensures consistency and makes it easy for new team members to understand the project's structure.
Separate Configuration Files
In addition to organizing our properties based on profiles, we should also consider keeping separate configuration files for each profile. This allows us to have a clean separation of configuration properties and makes it easier to manage and modify them.
For example, we can have a application-dev.properties
file for the dev
profile, a application-test.properties
file for the test
profile, and a application-prod.properties
file for the prod
profile.
Externalize Sensitive Information
Sensitive information like passwords, API keys, or database credentials should never be stored in configuration files, especially those that are part of the source code. Instead, we should externalize such information using environment variables or properties provided by the deployment environment.
By externalizing sensitive information, we can maintain a higher level of security and avoid exposing credentials accidentally.
Use Default Profile
Using a default profile is a good practice to ensure that our application has a valid profile activated even if we forget to specify one explicitly. By defining a default profile, we can avoid unexpected failures due to missing or misconfigured profiles.
For example, we can create a default
profile and activate it when no other profile is specified. This can be achieved by setting the spring.profiles.default
property in our application's configuration file.
spring.profiles.default=default
Leverage Spring Boot Features
If we are using Spring Boot, we can leverage its features to make working with Spring Profiles even easier. Spring Boot provides additional utilities and configuration options that simplify the management of profiles in a Spring application.
For example, we can use the spring.profiles.include
property to include additional profiles along with the active profile. We can also use Spring Boot's @ConfigurationProperties
annotation to bind profile-specific properties directly to our configuration classes.
Lessons Learned
Optimizing Spring Profiles is crucial for streamlining configuration for different environments in a Java application. By taking advantage of Spring Profiles, we can simplify configuration management, customize our application for different environments, facilitate testing and debugging, improve build and deployment efficiency, and foster collaboration among team members.
Following best practices like clear and consistent profile naming, separate configuration files, externalizing sensitive information, using a default profile, and leveraging Spring Boot features can further enhance our experience with Spring Profiles.
With the power of Spring Profiles, we can create more robust and adaptable Java applications that seamlessly transition between different environments, delivering a highly tailored experience for each scenario.
So, go ahead and start optimizing your Spring Profiles to unlock the full potential of your Java application!