Unit Testing: How Skipping It Can Spell Disaster
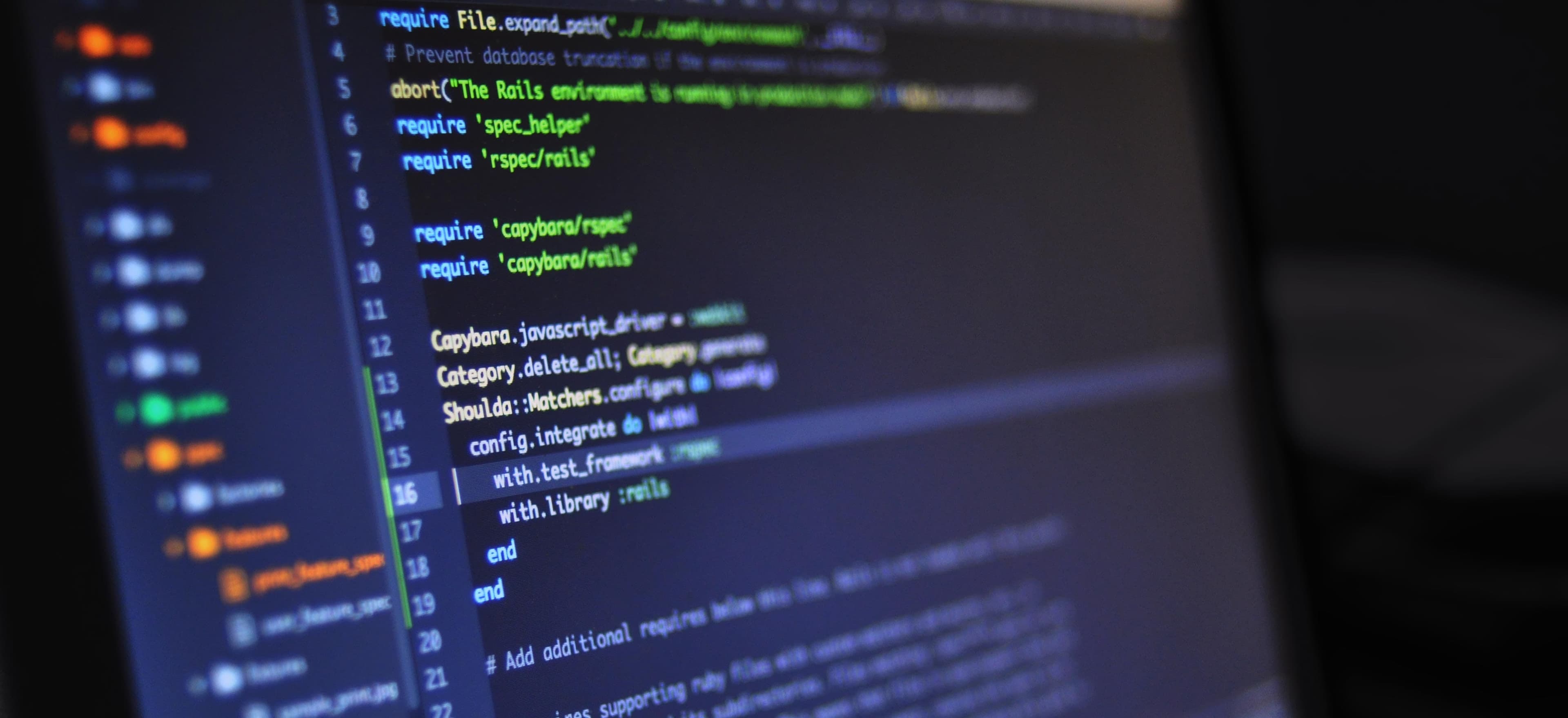
- Published on
Unit Testing: How Skipping It Can Spell Disaster
Introduction:
In the world of software development, unit testing plays a vital role in ensuring the quality and reliability of code. Unit testing involves testing individual units of code, typically functions or methods, to ensure they work as expected. This article will delve into the importance of unit testing and explore the consequences of skipping this crucial step. We will also provide examples of using JUnit, a popular Java testing framework, and discuss how to integrate unit testing into the development process.
The Importance of Unit Testing:
Unit testing is not just an optional task, but an essential practice for maintaining a high-quality codebase. Here are some reasons why unit testing is valuable:
-
Enhancing code quality: Unit tests act as a safety net, catching errors and bugs early in the development process. By writing tests, developers can identify problems in their code and fix them before they impact the end-users. This leads to cleaner and more reliable code.
-
Facilitating refactoring: Refactoring is a crucial part of the software development lifecycle. It involves making changes to the codebase to improve its structure and readability. With proper unit tests in place, developers can confidently refactor their code, knowing that any regressions will be caught by the tests.
-
Improving documentation: Unit tests can serve as living documentation that showcases the expected behavior of a unit of code. By reading the tests, developers can quickly understand how a particular function or method should be used and what its expected output or behavior is.
-
Providing a safety net for changes: As a codebase evolves, new features are added and existing functionality is modified. Without unit tests, developers might inadvertently introduce bugs or regressions. By having a comprehensive suite of tests, they can quickly validate that everything still works as expected after making changes.
Real-World Consequences of Skipping Tests:
Skipping unit tests can have severe consequences that extend beyond the code itself. Let's take a look at a few real-world scenarios where the lack of tests led to significant issues:
-
The Knight Capital Group Trading Disaster: In 2012, the Knight Capital Group, a financial services company, experienced a catastrophic software failure that cost them over $440 million. The failure occurred due to a code deployment in which a critical piece of software was not adequately tested. As a result, the firm executed numerous erroneous trades within minutes, prompting huge financial losses and eventually leading to the company's bankruptcy.
-
The Mars Climate Orbiter Incident: In 1999, NASA's Mars Climate Orbiter spacecraft burned up in the Martian atmosphere instead of entering orbit. The failure occurred because different parts of the system used different units of measure. A simple unit test that verified the correct units of measure could have prevented this disastrous mishap, saving millions of dollars and valuable scientific data.
These examples demonstrate the potential business impacts of not implementing unit tests. Skipping tests can lead to financial losses, damage to reputation, loss of customer trust, and in some cases, the outright failure of a project or company.
Unit Testing in Java: Best Practices
When it comes to unit testing in Java, JUnit is the most popular framework. JUnit provides a simple and expressive way to write tests and comes bundled with many development environments. Here are some best practices for unit testing in Java using JUnit:
-
Test naming conventions: Use meaningful names for your test methods that convey their purpose and what they're testing. This makes it easier for other developers to understand the tests and their intent.
-
Arrange-Act-Assert (AAA) pattern: Structure your tests using the AAA pattern, where you arrange the necessary preconditions, act on the code under test, and assert the expected outcome or behavior. This pattern promotes clarity and helps other developers understand the flow of the test.
-
Mock objects and frameworks: In unit testing, it's often necessary to isolate units of code from their dependencies to test them in isolation. Mock objects and frameworks like Mockito can help you create mock implementations of dependencies, allowing you to focus on testing the particular unit of code without worrying about the real dependencies.
Java Unit Testing Example
To illustrate the concepts discussed above, let's consider a simple Java class, Calculator
, which contains two methods, add
and subtract
, that perform basic arithmetic operations. Here's an example of how we can write unit tests for this class:
public class Calculator {
public int add(int a, int b) {
return a + b;
}
public int subtract(int a, int b) {
return a - b;
}
}
@Test
public void givenTwoIntegers_whenAdd_thenCorrectSum() {
Calculator calc = new Calculator();
int sum = calc.add(5, 10);
assertEquals(15, sum);
}
@Test
public void givenTwoIntegers_whenSubtract_thenCorrectDifference() {
Calculator calc = new Calculator();
int difference = calc.subtract(10, 5);
assertEquals(5, difference);
}
In the above code, we have defined a Calculator
class with add
and subtract
methods, and we have written two simple unit tests using JUnit. The givenTwoIntegers_whenAdd_thenCorrectSum
test verifies that the add
method correctly calculates the sum of two integers. The givenTwoIntegers_whenSubtract_thenCorrectDifference
test checks if the subtract
method correctly calculates the difference between two integers.
These tests cover the expected behavior of the Calculator
class, ensure it functions as intended, and act as a safety net against future regressions or bugs.
How to Integrate Unit Testing into Your Development Process:
Integrating unit testing into your development process is crucial for maintaining code quality and preventing issues down the line. Here are a few ways you can incorporate unit testing into your workflow:
-
Continuous Integration & Continuous Deployment (CI/CD): Set up a CI/CD pipeline that includes running unit tests as part of the build and deployment process. This ensures that any code changes are thoroughly tested before being deployed to production.
-
Test-Driven Development (TDD): Adopt a test-driven development approach, where tests are written before the code is implemented. This ensures that the code meets the desired behavior defined by the tests and serves as living documentation for future development.
-
Integration with build tools like Maven or Gradle: Build tools like Maven or Gradle offer seamless integration with testing frameworks like JUnit. Configure your build tool to automatically run tests as part of the build process, making it easy to incorporate testing into your daily development workflow.
Conclusion:
Unit testing is a critical practice that should not be overlooked in software development. It enhances code quality, facilitates refactoring, improves documentation, and provides a safety net for changes. Skipping unit testing can have severe consequences, as evidenced by real-world incidents where the lack of tests led to significant failures and financial losses.
In the Java ecosystem, JUnit is the go-to framework for unit testing. Its simplicity and extensive tooling support make it an excellent choice for writing tests. By integrating unit testing into your development process, whether through CI/CD pipelines, TDD, or build tool integration, you can ensure the reliability and quality of your codebase.
Remember, the cost of not implementing unit tests far outweighs the time and effort required to write them. Embrace unit testing as a regular practice, and you'll reap the benefits of safer and more robust code.
Checkout our other articles