Fix Git Mistakes: A Beginner's Guide to Undoing Errors
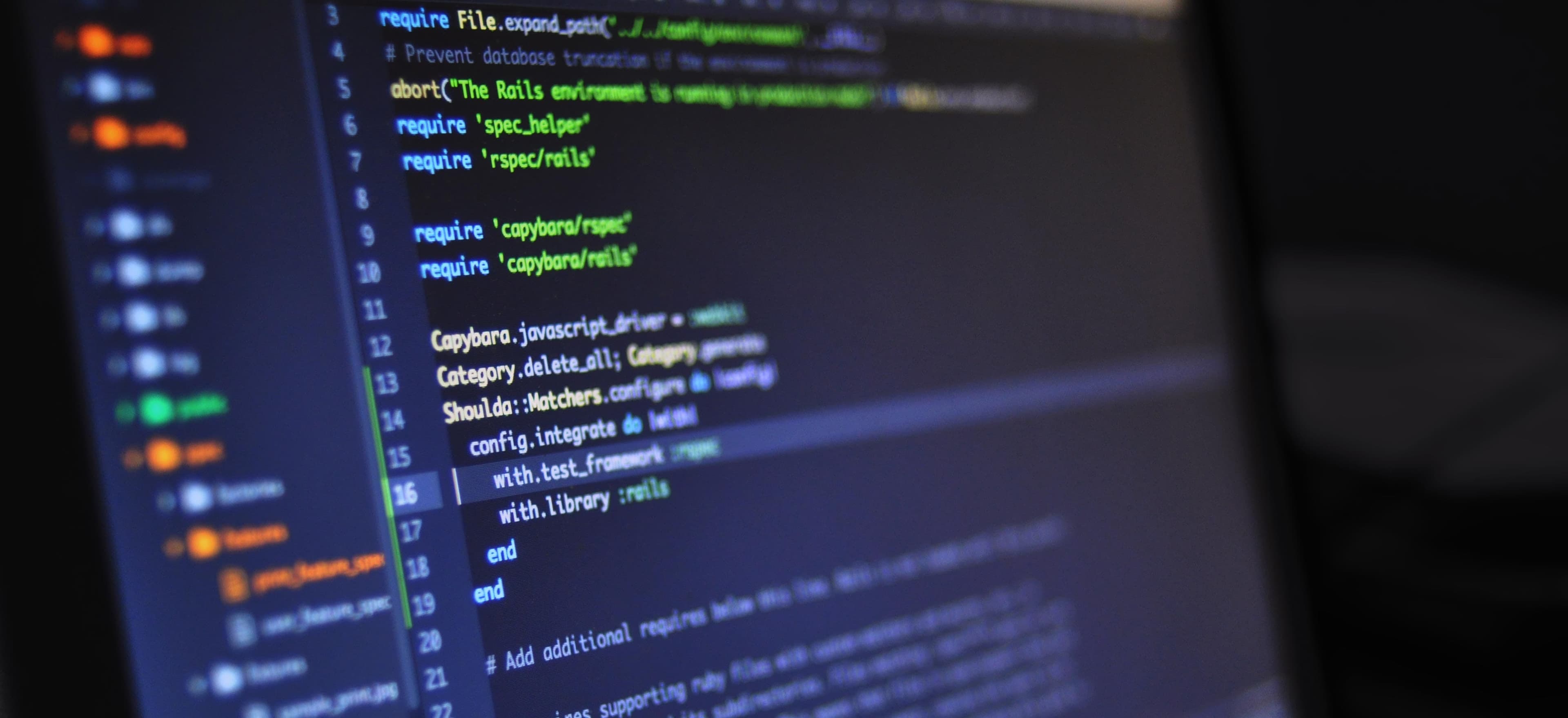
- Published on
Fix Git Mistakes: A Beginner's Guide to Undoing Errors
When you're just getting started with Git, understanding how to effectively roll back errors and undo changes is as crucial as making the changes themselves. Everyone makes mistakes, but in the Git universe, there's almost always a command to undo them. Let's explore the most common scenarios and the Git commands that can save the day.
Understanding Git's Safety Net
Git is a distributed version control system that is widely used in software development, including Java projects, for tracking changes in source code during development. Its popularity stems from its flexibility and robustness in handling both small and large projects effectively.
In this post, we'll delve into some practical ways to undo mistakes in Git. We'll go from basic commands, like git revert
and git reset
, to slightly more complex scenarios using git reflog
. But first, a primer on Git's internal mechanisms will give you the understanding necessary to wield these commands with confidence.
Undoing Uncommitted Changes
Discarding Local Changes in a File
git checkout -- <file>
Let's start simple. If you've made a mistake in a file and haven't yet committed it, git checkout -- <file>
is your first line of defense. This will revert the file to the last committed state, discarding any local changes you made. The double dash --
is a common convention in Unix command-line utilities that signifies the end of command options, after which only positional parameters are expected. In this context, it helps to avoid confusion in case your file name coincidentally matches a branch name.
Resetting Staged Changes
git reset HEAD <file>
Maybe you've gone a step further and staged your changes with git add
, but you've now realized there's an error. You can unstage the file using git reset HEAD <file>
, which removes the changes from the staging area without altering the current working directory.
Rewriting History: Before and After Committing
Amending the Last Commit
git commit --amend
If you've made a typo in your last commit message or forgot to include a small change, git commit --amend
is quite handy. This will open your configured text editor, allowing you to modify the commit message or update the commit contents with staged changes.
Reverting a Commit
git revert <commit>
To undo a specific commit without altering the history, git revert <commit>
creates a new commit that applies the inverse of the specified commit's changes. This is the preferred method of undoing changes that have already been shared with others. More on using git revert.
Resetting to a Previous Commit
git reset --hard <commit>
For the more aggressive approach, git reset --hard <commit>
will reset the current branch's HEAD to the specified commit and discard all changes in the working directory and staging area. This is a powerful command that can result in data loss, so it's essential to use it with care.
git reset --soft <commit>
Conversely, if you wish to keep your working directory and staging area but still reset the HEAD to a previous commit, git reset --soft <commit>
is the command of choice.
Utilizing Reflog to Navigate the Commit History
git reflog
When you've lost track of your commits after an aggressive reset, git reflog
is the lifeline you need. This command shows a log of where the HEAD and other reference logs have been, making it possible to identify a commit you may have orphaned inadvertently. Check out Git's documentation on reflog.
Recovering Lost Commits
git checkout -b <new-branch> <orphaned-commit>
Did you reset too far back and orphan some commits? Not to worry. Once you've identified the orphaned commit with git reflog
, you can create a new branch from that commit using git checkout -b <new-branch> <orphaned-commit>
. This recovers the orphaned commit onto a new branch.
Code Snippets and Commentary
Amending the Last Commit with Java Code
Perhaps you've just committed a Java class and realized you missed documenting a method. Here's a simple Java method before amendment:
public class Calculator {
// Adds two numbers together
public int add(int a, int b) {
return a + b;
}
}
You quickly add the missing documentation:
public class Calculator {
/**
* Adds two numbers together.
* @param a first number to add
* @param b second number to add
* @return the sum of a and b
*/
public int add(int a, int b) {
return a + b;
}
}
After staging this change with git add Calculator.java
, you amend the previous commit:
git commit --amend
Now the commit includes not only the method but also the proper documentation.
Reverting a Harmful Commit
Imagine we have a commit that introduced a bug in our Java code:
// This commit unfortunately introduced a bug
public class Calculator {
// Incorrect method - subtracts two numbers
public int add(int a, int b) {
return a - b; // Oops, this should have been a plus sign
}
}
To correct this, we use the git revert
command:
git revert <commit-id>
This will create a new commit that contains the fix, effectively inverting the buggy commit while maintaining the project's history intact.
Soft Resetting a Java Project to an Earlier State
If a set of commits on your JavaProject
doesn't make sense, and you want to stage them in a different way, you just need to find the commit before the mistakes began. Let's say it's commit1234
. To reset softly to this state:
git reset --soft commit1234
Now, all the changes from the commits after commit1234
are staged and ready to be re-committed in a cleaner manner.
Lessons Learned
Mastering the art of undoing mistakes with Git ensures that you can keep your Java projects' histories clean and manageable. Using commands like git checkout
, git reset
, git commit --amend
, git revert
, and git reflog
, you can confidently navigate and fix errors in your development journey. Remember, a good Git workflow includes regularly committing, pushing to remote repositories, and using branches to organize features or experiments.
Becoming adept with these undoing techniques is a valuable part of that workflow, offering the reassurance that no mistake is irreversible. With this guide, you're now better equipped to take control of your Git history and keep your Java code on track. Remember to check the official Git documentation for more details on these commands and to explore further functionalities. Keep coding, keep committing, and when needed, keep undoing!
Checkout our other articles