Mastering Configs: Solving Netflix Archaius Integration Woes
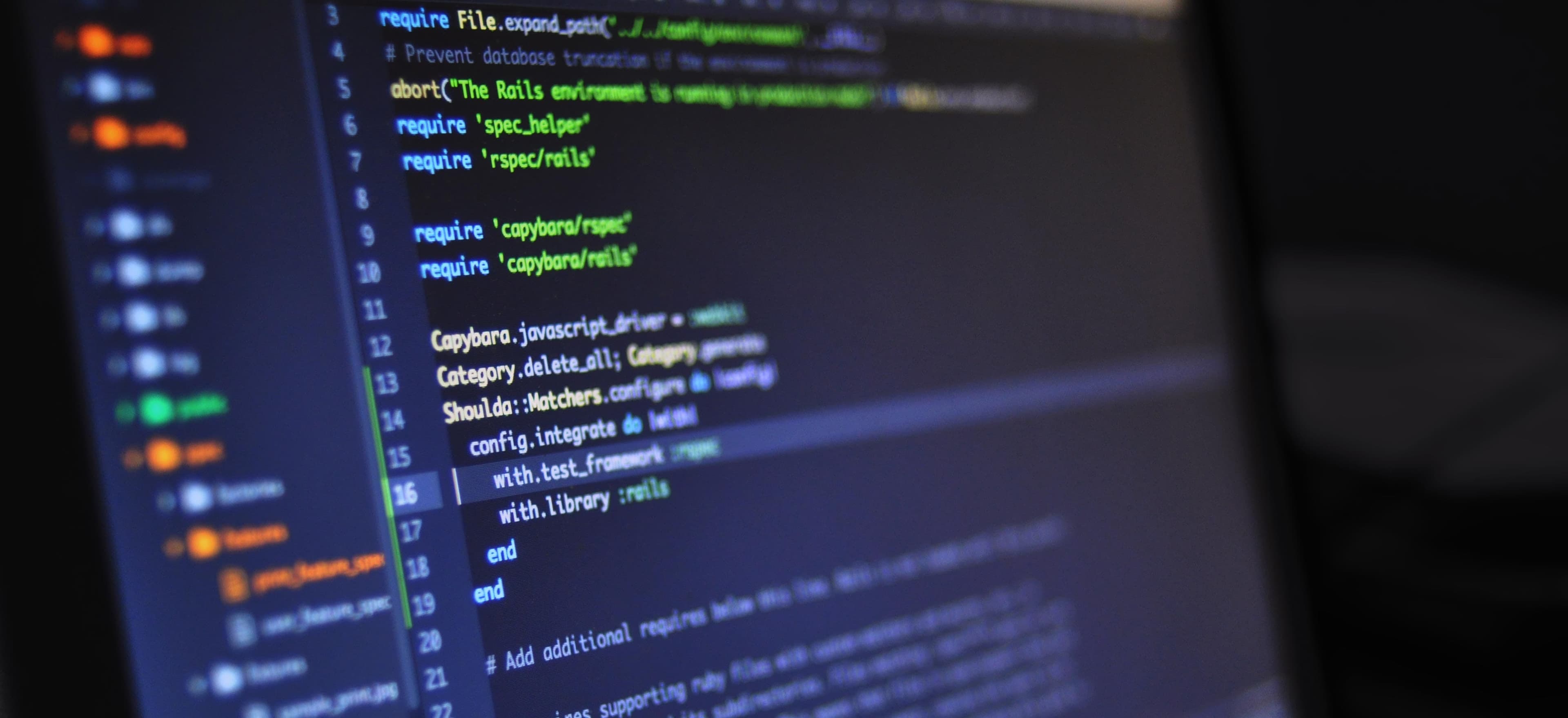
- Published on
Mastering Configs: Solving Netflix Archaius Integration Woes
The Roadmap
In today's modern application landscape, managing configurations is a critical aspect of building reliable and scalable systems. As applications become more distributed and complex, the need for a robust and flexible configuration management solution arises. Netflix Archaius is a powerful library that addresses the challenges of configuration management in a cloud environment. In this article, we will explore Archaius and learn how to integrate it into a Java application.
Contextual Importance
Configuration management plays a vital role in the success of any software application. Configurations define how an application behaves, and managing them effectively is crucial for maintaining the stability and scalability of the system. In distributed systems, managing configurations becomes even more challenging due to the need for consistency across multiple instances of an application.
Without a proper configuration management system, developers face various problems. It becomes difficult to handle configuration changes dynamically without restarting the application. Managing configurations across different environments becomes a manual and error-prone task. Additionally, there is a lack of high availability and fault tolerance when it comes to configuration sources.
Netflix Archaius Overview
Netflix Archaius is an open-source library developed by Netflix for managing configurations in a cloud environment. It provides a dynamic and flexible way to manage configurations in distributed systems. Archaius is widely adopted by developers due to its features like dynamic configuration reloading, high availability, and support for various configuration sources.
What is Netflix Archaius?
Netflix Archaius is a configuration management library that helps developers manage application configurations with ease. It provides a unified API to load and access configurations from various sources, such as property files, databases, and REST endpoints. Archaius is designed to be highly configurable and can be seamlessly integrated into existing Java applications.
Key Features of Archaius
Archaius offers several key features that make it a powerful configuration management tool:
Dynamic Configuration
Archaius supports dynamic configuration reloading, allowing configurations to be updated without restarting the application. This feature is especially useful in distributed systems where multiple instances of an application need to be synchronized with the latest configuration changes.
High Availability
Archaius provides built-in support for managing configuration sources in a highly available manner. It automatically handles failures and fallbacks, ensuring that applications always have access to the latest configuration.
Typed Configuration Management
Archaius allows developers to define configuration properties with specific types, making it easier to work with configurations. It provides type-specific getter methods and automatic type conversion for properties.
Setting up Netflix Archaius
Setting up Netflix Archaius in a Java application is a straightforward process. This section will guide you through the steps required to get started with Archaius.
Dependencies and Initial Configuration
To use Archaius in your Java project, you need to add the necessary dependencies to your build file (e.g., Maven or Gradle). Here are the Maven dependencies you need to include:
<!-- Archaius Core -->
<dependency>
<groupId>com.netflix.archaius</groupId>
<artifactId>archaius-core</artifactId>
<version>{latest_version}</version>
</dependency>
<!-- Archaius Commons -->
<dependency>
<groupId>com.netflix.archaius</groupId>
<artifactId>archaius-commons</artifactId>
<version>{latest_version}</version>
</dependency>
Replace {latest_version}
with the latest version of Archaius.
Next, you need to initialize Archaius in your application. Add the following code snippet to your application's entry point, such as the main
method:
import com.netflix.config.ConfigurationManager;
public class MyApp {
public static void main(String[] args) {
ConfigurationManager.loadCascadedPropertiesFromResources("config");
}
}
The loadCascadedPropertiesFromResources
method loads properties from the classpath resource named "config". You can customize the resource name and path as per your application's configuration.
Loading Configuration Sources
Archaius supports various configuration sources, and this section will demonstrate how to load configurations from popular sources.
Property Files Configuration
Property files are a commonly used configuration source, and Archaius provides built-in support for loading properties from them. Here's an example of how to load configurations from a property file:
ArchaiusConfigurationSource source = new FileConfigurationSource(new File("config.properties"));
DynamicConfiguration configuration = new DynamicConfiguration(
source,
new FixedDelayPollingScheduler()
);
ConfigurationManager.install(configuration);
In the above code snippet, we create a FileConfigurationSource
by providing the file path of the property file. We then create a DynamicConfiguration
instance with the source and a polling scheduler. Finally, we install the configuration into the ConfigurationManager
.
Dynamic Property Management
One of the most powerful features of Archaius is its ability to dynamically manage properties at runtime. You can get and set properties dynamically, as well as listen to changes in the configuration. Here's an example of how to work with dynamic properties:
DynamicStringProperty countProperty = DynamicPropertyFactory.getInstance().getStringProperty("myapp.count", "default");
System.out.println("Initial value: " + countProperty.get());
countProperty.addCallback(() -> {
System.out.println("New value: " + countProperty.get());
});
countProperty.set("5");
In the above code, we create a DynamicStringProperty
instance by calling the getStringProperty
method on the DynamicPropertyFactory
. We provide a key for the property and a default value. We can then get the current value of the property using the get
method, and add a callback to be notified of changes. Finally, we can set a new value for the property using the set
method.
Troubleshooting Common Integration Issues
While integrating Netflix Archaius into your Java projects, you might come across some common issues. Here are a few of them along with their possible solutions.
Issue 1: Dependency Conflicts
Archaius relies on several dependencies, and it's possible to encounter conflicts with other libraries in your project. To resolve dependency conflicts, you can use dependency management tools like Maven or Gradle to handle the conflicting versions. It's important to ensure that you have compatible versions of all the dependencies.
Issue 2: Refreshing Configurations
Sometimes, you might want to refresh configurations without restarting the application. Archaius provides a PollingScheduler
interface that allows you to define custom polling intervals to fetch updated configurations. Here's an example:
PollingScheduler scheduler = new FixedDelayPollingScheduler(5000, 1000, false);
DynamicConfiguration configuration = new DynamicConfiguration(
source,
scheduler
);
In the above code snippet, we create a FixedDelayPollingScheduler
with a delay of 5000 milliseconds and an initial delay of 1000 milliseconds. This means that Archaius will poll for changes every 5 seconds, with an initial delay of 1 second. The last parameter, false
, indicates that Archaius should not schedule periodic tasks.
Issue 3: Custom Configuration Sources
Archaius supports various configuration sources out of the box, but you might come across situations where you need to integrate custom sources. Archaius provides an extensible API that allows you to create custom configuration sources. You can implement the ConfigurationSource
interface and provide your own logic for loading and managing configurations from your custom source.
Best Practices
Integrating Netflix Archaius in a Java project requires careful consideration to ensure the stability and reliability of the application. Here are some best practices to keep in mind:
Version Controlling Configurations
It's essential to version control your configuration files to track changes and ensure consistent deployment across different environments. Consider using a version control system like Git to manage your configuration files.
Security Considerations
When managing configurations, it's crucial to handle sensitive information securely. Avoid storing sensitive information like passwords or API keys in plain text configuration files. Instead, consider using secure storage mechanisms like environment variables or a secure configuration store.
Testing
Testing configurations managed by Archaius is an important part of the development process. Write unit tests to verify that the expected configurations are loaded correctly. You can use tools like JUnit and Archaius's ConfigurationTestBuilder
to facilitate testing.
Bringing It All Together
Netflix Archaius is a powerful configuration management library that offers dynamic and flexible solutions for managing configurations in Java applications. In this article, we explored the features of Archaius and learned how to integrate it into a Java project. We also discussed common integration issues and best practices to follow while working with Archaius.
By leveraging Archaius, developers can efficiently manage configurations in distributed systems, ensuring that applications stay flexible and responsive to changing requirements. As you continue on your journey of mastering configuration management, we encourage you to explore Netflix Archaius further and experiment with its capabilities.
If you have any feedback or questions, we would love to hear from you. Please leave a comment below or reach out to us on social media.
Additional Resources:
- Netflix Archaius GitHub
- Official Netflix Archaius Documentation