Top Java Pitfalls: Navigating Its Worst Features
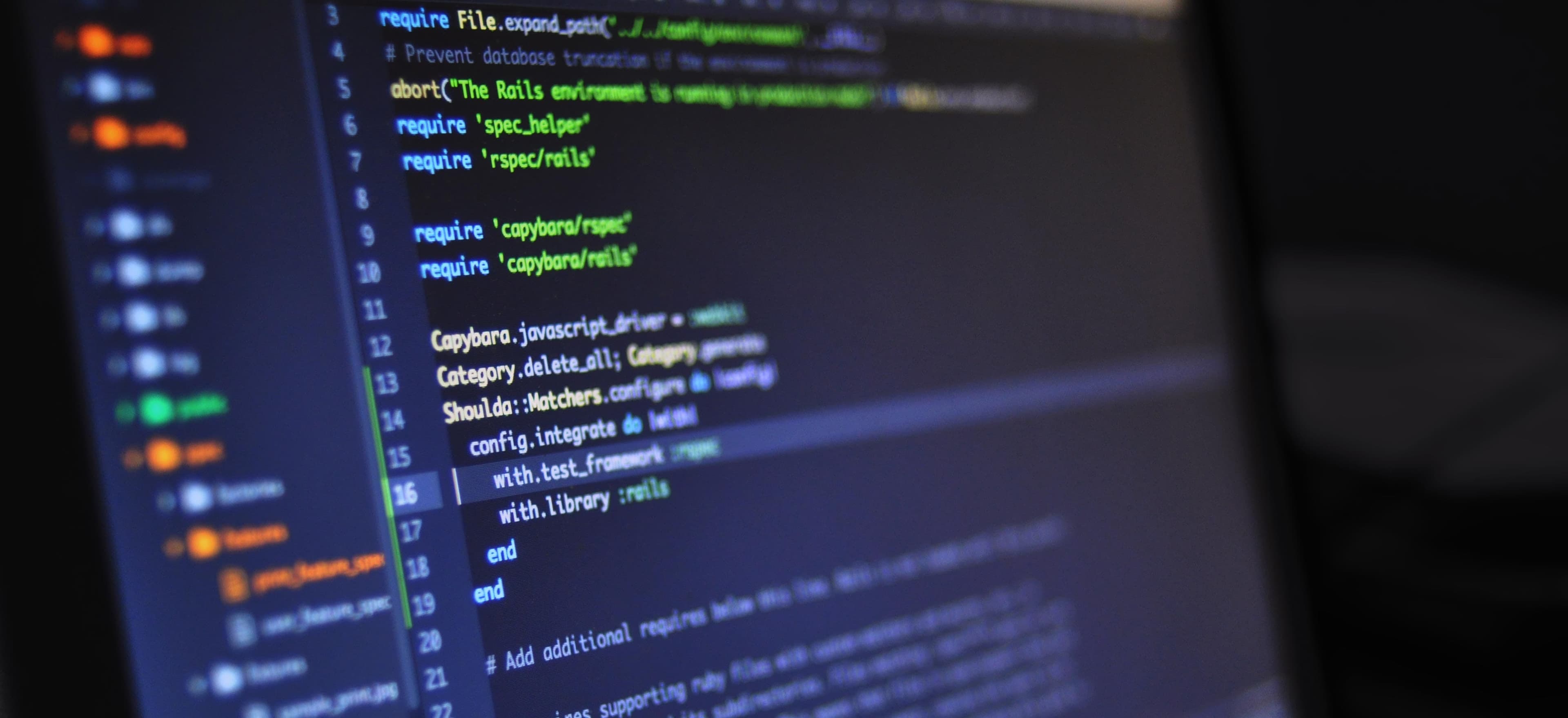
- Published on
Top Java Pitfalls: Navigating Its Worst Features
Before We Begin
Java is undoubtedly one of the most widely used programming languages in the software development landscape. It offers a robust ecosystem, cross-platform compatibility, and extensive libraries that make it a top choice for building a wide range of applications. However, like any technology, Java has its fair share of pitfalls that can trip up even experienced developers.
In this article, we'll explore some of the common pitfalls in Java, understand why they can cause problems, and learn how to navigate these issues. By being aware of these pitfalls and applying best practices, you can write better, more reliable, and maintainable code.
Pitfall 1: Null Pointer Exceptions
A Null Pointer Exception (NPE) occurs when you try to access or perform an operation on a null
object reference. It is one of the most common runtime exceptions in Java, responsible for countless hours of debugging.
The prevalence of NPEs in Java programs is due to the language's default behavior of allowing variables to have null
as a value. Developers often forget to check if an object is null
before using it, leading to unexpected crashes and bugs.
Code Snippet: Using Optional
One way to address the issue of NPEs is by using the Optional
class introduced in Java 8. Optional
is a container object that may or may not contain a non-null value. It provides a more explicit way of handling the absence of a value, eliminating the risk of NPEs.
Here's an example of how to use Optional
to prevent NPEs:
public String getUserName(User user) {
return Optional.ofNullable(user)
.map(User::getName)
.orElse("Unknown User");
}
In this code snippet, we create an Optional
object using Optional.ofNullable(user)
. We then use the map
method to extract the name from the User
object if it is present. Finally, we use the orElse
method to provide a default value in case the User
object is null
. This approach enhances both code safety and readability.
Pitfall 2: Memory Leaks
While Java's garbage collector (GC) takes care of memory management, it does not prevent all memory leaks. Memory leaks occur when objects are no longer needed but are still referenced, preventing the GC from reclaiming their memory.
Developers can inadvertently introduce memory leaks through scenarios such as failing to remove event listeners, retaining unnecessary object references in caches, or using excessive static fields.
Code Snippet: Preventing Memory Leaks
One effective way to prevent memory leaks in Java is to use the try-with-resources
statement for managing resources that require explicit closing, such as database connections and file streams. The try-with-resources
statement automatically closes the resources, even if an exception occurs, preventing leaks.
Here's an example that demonstrates how to correctly manage resources using try-with-resources
:
public void readDataFromFile(String filePath) {
try (BufferedReader reader = new BufferedReader(new FileReader(filePath))) {
String line;
while ((line = reader.readLine()) != null) {
// Process the line
}
} catch (IOException e) {
// Handle the exception
}
}
In this code snippet, the BufferedReader
is automatically closed when it is no longer needed, regardless of whether an exception occurs or not. This approach is more convenient and reliable than manually managing resource cleanup.
Pitfall 3: Concurrency Issues
Writing thread-safe code in Java can be challenging due to the potential for concurrency issues such as race conditions and deadlocks. These issues arise when multiple threads access shared resources concurrently, leading to unpredictable behavior and even program crashes.
Code Snippet: Safe Concurrency Practices
Java provides several mechanisms to help developers write thread-safe code, such as synchronized blocks, the java.util.concurrent
package, and atomic variables.
Here's an example that demonstrates the use of synchronized blocks to ensure thread safety:
public class Counter {
private int count;
public synchronized void increment() {
count++;
}
public synchronized int getCount() {
return count;
}
}
In this code snippet, the synchronized
keyword ensures that only one thread can execute the methods increment
and getCount
at a time. This prevents race conditions where multiple threads may attempt to modify or access the count
variable simultaneously.
Java's java.util.concurrent
package also provides high-level concurrency utilities that can simplify dealing with shared resources in multithreaded environments. Additionally, atomic variables offer a simple way to perform atomic operations without worrying about thread interference.
Pitfall 4: Exception Handling
Improper exception handling can lead to code that is difficult to debug and maintain. Java offers powerful exception handling capabilities, but if used incorrectly, exceptions can be mismanaged or ignored, resulting in code that is prone to errors.
Code Snippet: Proper Exception Handling
To handle exceptions correctly, it's important to follow best practices such as using try-catch-finally blocks and throwing appropriate exceptions.
Here's an example of a try-catch-finally block with proper exception handling:
public void readFile(String filePath) throws IOException {
BufferedReader reader = null;
try {
reader = new BufferedReader(new FileReader(filePath));
String line;
while ((line = reader.readLine()) != null) {
// Process the line
}
} catch (FileNotFoundException e) {
// Handle the exception
} catch (IOException e) {
// Handle the exception
throw e; // Rethrow the exception if necessary
} finally {
if (reader != null) {
try {
reader.close();
} catch (IOException e) {
// Handle the exception
}
}
}
}
In this code snippet, the try
block contains the code that may throw an exception. The catch
blocks handle specific types of exceptions, allowing for more targeted exception handling. The finally
block ensures that the resource is closed, regardless of whether an exception occurs or not.
This approach makes the code more transparent and maintainable, as it clearly indicates how exceptions are handled and resources are released.
Pitfall 5: Overuse of Patterns and Abstractions
In Java, there is a tendency to overengineer solutions by applying complex design patterns and excessive abstractions. While design patterns and abstractions have their place in software development, overusing them can lead to unnecessary complexity and reduced code clarity.
Code Snippet: Simplicity in Design
To avoid the trap of overusing patterns and abstractions, it's essential to strike the right balance and prioritize simplicity when it makes sense.
Consider the following example where a simple solution is more appropriate than a complex design pattern:
public class Calculator {
public int add(int a, int b) {
return a + b;
}
}
In this code snippet, a straightforward add
method is implemented in the Calculator
class. There is no need for a complex abstract factory, strategy, or decorator pattern to perform a basic arithmetic operation.
By keeping the design simple and avoiding unnecessary abstractions, you can achieve more maintainable code that is easier to understand and debug.
Closing the Chapter
Java is a powerful and versatile programming language, but it is not without its pitfalls. By being aware of and understanding these common issues, you can write better Java code that is more robust and maintainable.
In this article, we explored some of the top Java pitfalls, including Null Pointer Exceptions, memory leaks, concurrency issues, exception handling, and the overuse of patterns and abstractions. We provided tips and code examples to help you navigate these pitfalls and write code that is less error-prone.
Remember, continuous learning and staying up-to-date with best practices are key to mastering any programming language. If you'd like to dive deeper into any of these topics, check out the links below for further reading:
We encourage you to share your own experiences or additional pitfalls you've encountered in Java by leaving a comment below. Together, we can continue to learn and grow as Java developers. Happy coding!