Boosting Speed: When Direct Memory Access Wins!
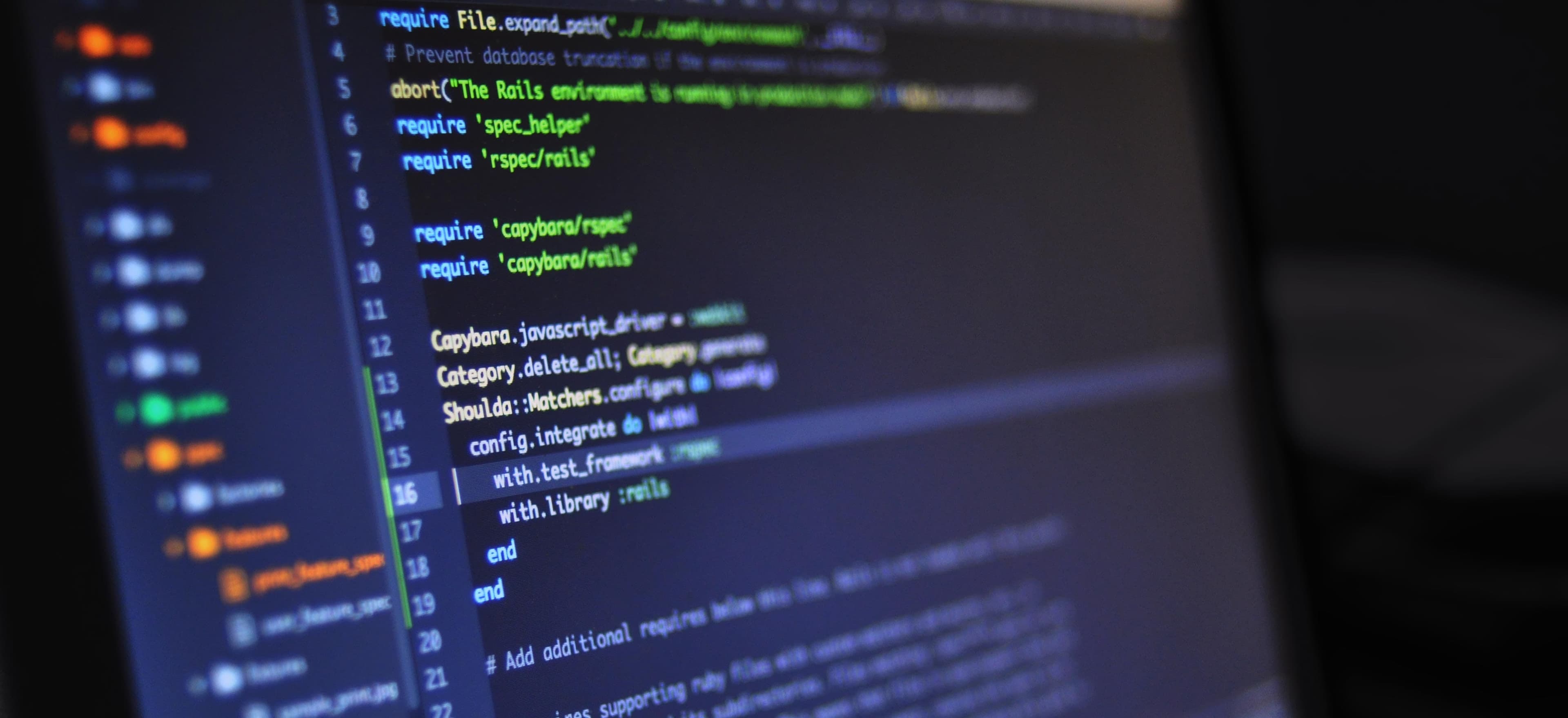
- Published on
Boosting Speed: When Direct Memory Access Wins in Java!
In the world of high-performance computing, milliseconds can make or break complex applications, and every byte of memory matters. The Java platform, renowned for its robustness, has long been critiqued on grounds of speed and control over memory when compared to languages like C++. However, Java is not without its own set of high-performing features, such as Direct Memory Access (DMA) via the ByteBuffer
class within its New I/O (NIO) package. This blog post digs into the how and why DMA can be a game-changer when you’re reaching for those speed stars.
What is Direct Memory Access?
In the Java universe, Direct Memory Access (DMA) is the ability to read or write data directly to memory from a channel, skirting the traditional detour through the Java heap. This is an essential technique for bypassing the Garbage Collector (GC), reducing overhead, and smashing through latency barriers.
NIO's ByteBuffer
provides DMA by allowing Java to interact with the operating system's native I/O operations effectively. The off-heap memory allocated by these buffers is managed by the OS, not the JVM, which hosts several benefits:
- Reduced Garbage Collection: Since off-heap memory isn’t subject to GC, there is no pause attributed to buffer data collection.
- Memory Efficiency: Large datasets do not clutter the heap, keeping application memory demands lean.
- High Performance: With less latency, applications can handle greater throughput.
When to Use Direct Memory Access
DMA is not the default mode in Java because it isn't a one-size-fits-all solution. Typical applications hosting moderately-sized data may never need the muscle of DMA. However, certain use cases scream for it:
- High-frequency trading systems where microseconds matter.
- Large-scale, big-data processing applications that shuffle terabytes of data.
- Real-time systems requiring consistent low-latency response times.
- Applications that integrate with high-speed, persistent storage solutions.
Utilizing ByteBuffer
for DMA in Java
To demonstrate the might of ByteBuffer
, let’s dive into a simple code snippet:
import java.nio.ByteBuffer;
public class DirectBufferExample {
public static void main(String[] args) {
// Allocate a direct byte buffer with a capacity of 1024 bytes
ByteBuffer directBuffer = ByteBuffer.allocateDirect(1024);
// Write data into the buffer
for (int i = 0; i < directBuffer.capacity(); i++) {
directBuffer.put((byte) i);
}
// Flip the buffer to prepare for reading
directBuffer.flip();
// Read and print the data from the buffer
while(directBuffer.hasRemaining()) {
byte b = directBuffer.get();
System.out.print(b + " ");
}
}
}
Commentary:
- Why Direct:
ByteBuffer.allocateDirect()
is used to create a direct buffer, which will allocate memory outside of the GC's domain. - Capacity Selection: We've chosen 1024 bytes (
1KB
) because it’s large enough to demonstrate without being overly extravagant. - Putting Data: Here, we write 1024 bytes into the buffer. Each byte corresponds to its index. This is meant to exemplify writing data to the buffer.
- Flipping the Buffer: The
flip()
method is crucial; it transitions the buffer from writing to reading mode. - Reading Data: The while loop prints out the buffer's contents, emphasizing the buffer's ability to directly access memory.
The power in this simplicity cannot be overstated. With off-heap buffers, you gain tight control over memory lifecycle, rid yourself of GC-induced pauses, but carry the extra responsibility of ensuring these resources are properly managed.
Management Strategies for Off-Heap Memory
Since off-heap memory is not managed by the JVM's Garbage Collector, the responsibility falls to the developer to keep this memory in check. Memory leaks outside the heap are grave perils causing the application to exhaust available system memory if not handled meticulous care. Here’s how you can stay on top of it:
- Explicit Buffer Release: Once you’re done with a direct buffer, call its
cleaner()
method to free up memory. This is more manual than heap management but essential for resource control.
((sun.misc.Cleaner)directBuffer.cleaner()).clean();
Note: The cleaner()
method is not part of the official Java API (though readily used), and it may change in future updates. This code functions in Java up to version 8. In later versions, you might need to use reflection or a third-party library to achieve the same outcome.
-
Buffer Pooling: Reuse buffers by creating a pool and recycling them throughout the application's lifetime to minimize allocation/deallocation overhead.
-
Monitoring: Proactively monitor direct memory usage with Java Management Extensions (JMX) or profiling tools to detect possible memory leaks.
Risks and Considerations
Implementing DMA in your Java application is not without its risks:
- Memory Leaks: Without the safeguard of GC, you risk memory leaks which may cause your application or even the OS to crash.
- Complexity: Managing memory manually complicates your codebase, increasing development and maintenance efforts.
- Portability Issues: Direct buffers tie your application more closely to the underlying hardware, which might affect portability.
- Debugging: Debugging memory issues off-heap can be more challenging than on-heap as standard tools may not support direct buffer introspection properly.
For all these reasons, it's key to ensure that the performance benefits outweigh these risks and downsides before diving headfirst into DMA.
Conclusion
Direct Memory Access in Java, while not universally applicable, can be a potent optimization for compute and memory-intensive applications. By moving away from heap allocation and GC passes with ByteBuffer
’s off-heap memory allocation, you harness greater control over memory and performance.
It’s a powerful approach for those willing to tackle the associated challenges, and it can be just what’s needed to boost your application into the high-performance bracket.
For more detailed information about the NIO package and memory management in Java, Oracle's documentation is a valuable resource. Dive into the official Java documentation on Buffers or check out resources on Java's Memory Management for wider context on optimization and handling memory in Java.
Before signing off, remember that high-performance coding in Java relies as much on skillful coding as on using the right tools for the job. Time to unlock the potential of Direct Memory Access and give your Java applications a speed boost that could make all the difference!
Happy coding!