Java's LTS Leap: Mastering the Move from 8 to 17
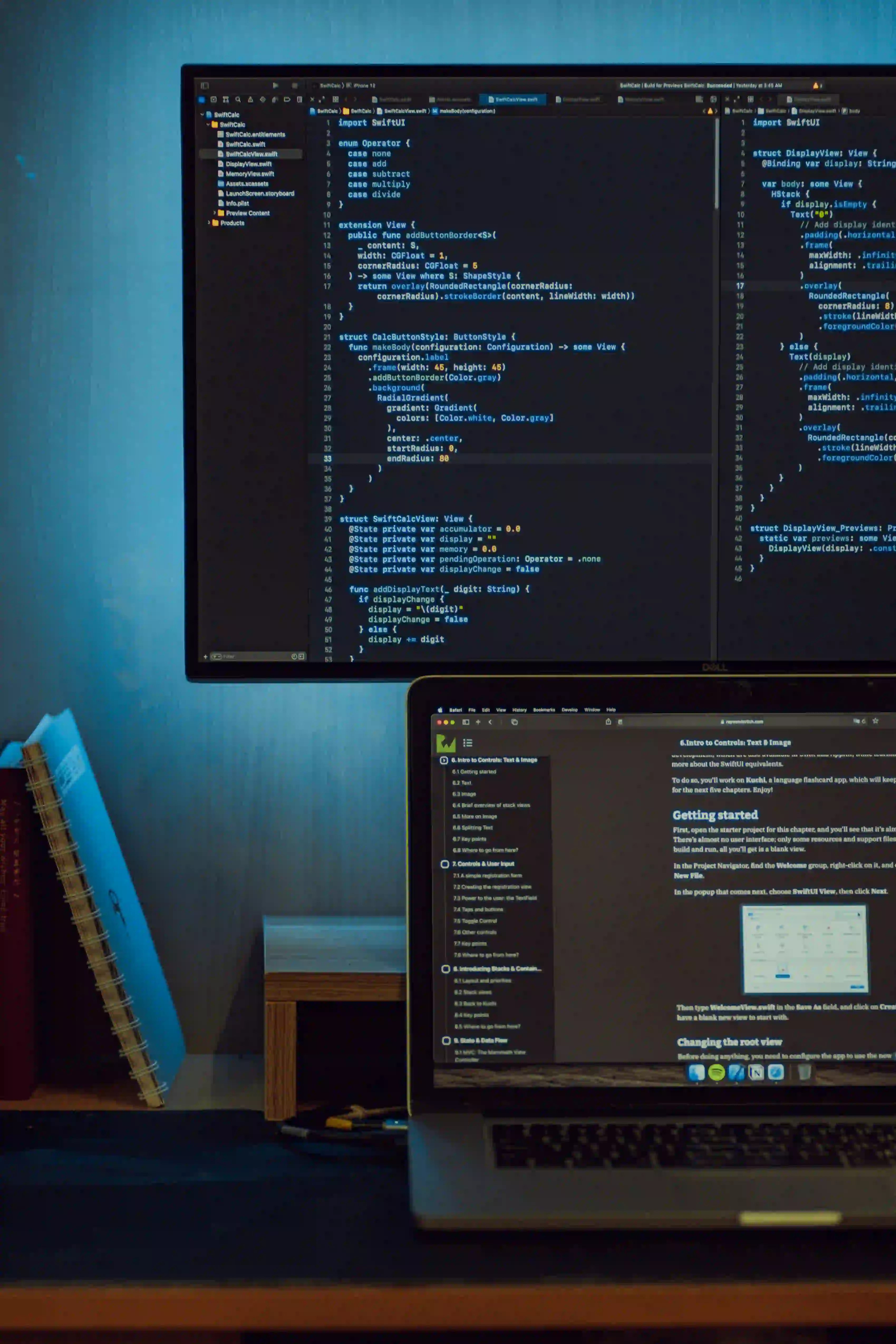
Java's LTS Leap: Mastering the Move from 8 to 17
The Approach
Java 8 has been a staple in the software development industry since its release in 2014. It introduced several groundbreaking features such as lambda expressions, functional interfaces, and the Stream API, revolutionizing the way Java developers write code. However, as technology rapidly evolves, so does the need for newer versions of programming languages.
In the world of Java, Long Term Support (LTS) versions play a critical role in ensuring stability and ongoing support for businesses. These LTS versions, unlike non-LTS versions, provide extended support and security updates, making them the preferred choice for organizations. With the recent release of Java 17, the latest LTS version, it's time for businesses to master the move from Java 8 to 17 and embrace the new features and improvements it offers.
Understanding the Importance of Long Term Support (LTS)
Long Term Support (LTS) versions are specifically designed to cater to the needs of enterprises and organizations that rely on Java for their critical applications. LTS versions undergo a more rigorous testing and stabilization process, ensuring that they are stable, reliable, and secure. This stability is particularly valuable for businesses as it minimizes the risk of disruption caused by frequent upgrades and changes.
Using non-LTS versions may provide access to new features and improvements sooner, but they come with the risk of compatibility issues and limited support. These versions are primarily intended for early adopters and developers who are eager to explore and experiment with the latest advancements in the Java ecosystem.
For businesses, sticking to LTS versions like Java 8 and Java 17 is the recommended approach. With LTS versions, organizations can confidently rely on long-term support, ensuring that security patches and bug fixes are provided for an extended period. This allows businesses to focus on their core operations without disruption caused by frequent upgrades.
Key Features in Java 17 That Weren't in Java 8
Java 17 brings several significant features and improvements that were not available in Java 8. Let's explore some of the key features that make upgrading from Java 8 to Java 17 an enticing proposition.
Sealed Classes
Sealed classes are an exciting addition to Java 17, introducing a new way to define class hierarchies and control their extensions. A sealed class restricts the set of classes that can extend or implement it, providing more control over the hierarchy and preventing unwanted subclassing.
Here's an example that demonstrates the definition of a sealed class:
public sealed interface Shape permits Circle, Rectangle, Triangle {
// Common methods and variables
}
public final class Circle implements Shape {
// Implementation specific to Circle
}
public final class Rectangle implements Shape {
// Implementation specific to Rectangle
}
public final class Triangle implements Shape {
// Implementation specific to Triangle
}
In this example, the Shape
interface is defined as sealed, and it permits only specific classes (Circle
, Rectangle
, and Triangle
) to implement it. Any other class trying to implement this interface will result in a compilation error. Sealed classes provide stronger encapsulation and promote safer frameworks by explicitly defining the allowed subclasses.
Pattern Matching for instanceof
Java 17 introduces an enhancement to the instanceof
operator, called pattern matching for instanceof
. This new feature makes type checking more concise and less error-prone, resulting in cleaner and more readable code.
Before Java 17:
if (obj instanceof Circle) {
Circle circle = (Circle) obj;
// Perform operations specific to Circle
}
With pattern matching for instanceof
in Java 17:
if (obj instanceof Circle circle) {
// Perform operations specific to Circle using the 'circle' variable
}
The new syntax eliminates the need for explicit type casting and introduces a more streamlined approach to working with specific types. This improves code readability and reduces the risk of type-related bugs.
Text Blocks for Better String Literals
Java 17 introduces text blocks, a feature that simplifies the representation of multi-line string literals. In Java 8 and earlier versions, representing multi-line strings required concatenating multiple strings or relying on escape characters.
Here's an example to demonstrate the use of text blocks:
String html = """
<html>
<body>
<h1>Welcome to Java 17!</h1>
<p>This is a multi-line string representation.</p>
</body>
</html>
""";
The text block begins and ends with three double quotes ("""
) and preserves the indentation of the string contents. This makes it much easier to write and read multi-line strings, improving code maintainability and reducing the chances of errors caused by missing escape characters or incorrect line breaks.
Records for Data Carrying Classes
Records are a new kind of class introduced in Java 14 but gained significant improvements in Java 17. A record is a concise way to define a class whose primary purpose is to store data. It simplifies the creation of data-holding classes by automatically generating essential methods such as getters, setters, equals, hashCode, and toString.
Here's an example that illustrates the use of a record:
public record Person(String name, int age) {
}
In this example, the Person
record declares two fields: name
of type String
and age
of type int
. The record implicitly generates all the necessary methods, making it easy to create simple data classes without the boilerplate code.
Records are especially useful in scenarios where immutability and data integrity are crucial, such as modeling data transfer objects (DTOs), Value Objects, or simple POJOs.
Other Notable Features
In addition to the key features mentioned above, Java 17 comes with several other notable improvements and enhancements. Here are some of them:
-
Z Garbage Collector (ZGC): Java 17 introduces the Z Garbage Collector as a production-ready feature. This garbage collector focuses on low latency and scalability, making it suitable for large-scale applications or microservices.
-
JEP 356: Enhanced Pseudo-Random Number Generators: This improvement enhances the flexibility and security of random number generation in Java applications.
These features, along with several performance improvements and bug fixes, make Java 17 a compelling option for businesses looking to leverage the full potential of the Java platform.
Migration Challenges and Solutions
Migrating from Java 8 to Java 17 involves several challenges. To tackle these challenges effectively, businesses need to be aware of common hurdles and have a clear plan in place. Let's explore some key areas that require attention during the migration process and discuss potential solutions.
Dependency Management
One of the critical challenges in upgrading Java applications is managing dependencies. As newer versions of Java may introduce breaking changes, it is essential to ensure that all project dependencies are compatible with Java 17.
To manage dependencies effectively, it is recommended to use build tools such as Maven or Gradle, which provide dependency management capabilities. These tools can automatically resolve and update dependencies during the migration process. Additionally, checking the documentation and release notes of each dependencies' new version can highlight any incompatible changes or required updates.
Code Refactoring
When migrating from Java 8 to Java 17, it is likely that some code refactoring will be required. This may involve updating deprecated APIs, replacing outdated constructs with newer ones, or adapting to new language features. Refactoring tools and IDEs can greatly assist in these efforts by automating many of the required changes.
Using an IDE like IntelliJ IDEA or Eclipse, developers can leverage the built-in refactoring capabilities to identify and apply code changes across the entire codebase. These tools provide automated suggestions, making the code migration process smoother and less error-prone.
Testing Strategies
Before making the leap to Java 17, having a robust testing strategy in place is crucial. Comprehensive testing helps ensure that the application behaves as expected and that any regressions or compatibility issues are identified and addressed.
Test automation tools, frameworks, and best practices play a vital role in efficient testing. Tools like JUnit, Mockito, and TestNG provide a solid foundation for writing unit tests. Additionally, integration tests and system-level tests can be implemented using tools such as Selenium or Spring Boot Test.
By following continuous integration and continuous delivery (CI/CD) practices, organizations can automate the testing process, enabling faster feedback cycles and more confident deployments.
Update CI/CD Pipeline
Updating the CI/CD pipeline is often overlooked when migrating to a new Java version. However, ensuring that the CI/CD infrastructure supports Java 17 is crucial for a smooth transition.
Organizations should review their CI/CD pipeline configuration and update it to accommodate Java 17. This may involve updating build environments, configuring the appropriate JDK version, and ensuring compatibility with any build scripts or tools used in the pipeline.
Taking the time to update the CI/CD pipeline during the migration process simplifies future updates and ensures that the development and deployment workflow remains seamless.
Adopting Java 17: Step-by-Step Migration Guide
Migrating from Java 8 to Java 17 requires a systematic and well-planned approach. Follow these steps to ensure a smooth transition to the latest LTS version:
Step 1: Prepare the Environment
Before starting the migration process, ensure that you have the necessary tools and resources in place. Download and install the latest version of the Java Development Kit (JDK) compatible with Java 17. Oracle provides JDK downloads on their official website, and installation guides are available to help you set up the development environment.
Step 2: Assess the Current State
Perform a thorough analysis of your codebase to understand its compatibility and readiness for Java 17. Several tools can assist in this process, such as IDE plugins, static code analysis tools, and migration assessment frameworks. These tools identify potential issues, highlighting areas that require attention during the migration process.
Step 3: Update Dependencies
Next, update your project's dependencies to ensure that they are compatible with Java 17. Review the documentation and release notes of each dependency to identify any breaking changes or migration steps required. It is recommended to update each dependency incrementally, verifying compatibility and functionality after each update.
Using build tools like Maven or Gradle, update the dependency versions in the project configuration files. The build tools will automatically resolve and download the updated versions during the build process.
Step 4: Apply Code Changes
Once the dependencies are updated, it's time to address code-related changes. Use the findings from the code analysis tools and manually review the codebase to identify areas that require modification.
Leverage the refactoring capabilities of your preferred IDE or code editor to automate code changes wherever possible. Update deprecated APIs, replace outdated constructs with newer ones, and refactor any incompatible code to align with Java 17 standards. Remember to pay specific attention to the new features introduced in Java 17 and update the codebase to leverage their benefits.
Step 5: Test Thoroughly
Testing is a critical step in the migration process. Perform thorough testing to ensure that the application works as expected in the Java 17 environment. This includes both functional and non-functional testing, such as unit testing, integration testing, and performance testing.
Automate testing wherever possible by using testing frameworks and tools that are compatible with Java 17. This ensures consistent and repeatable testing, reducing the chances of human error and providing faster feedback on any potential issues.
Step 6: Monitor and Optimize
After the migration is complete, continually monitor the application's performance and behavior in the Java 17 environment. Employ monitoring tools and incorporate logging and error tracking mechanisms to identify any performance bottlenecks or compatibility issues that may have been missed during testing.
Fine-tune the application based on the monitoring data and optimize performance where necessary. Regularly assess the application's performance in production and make proactive improvements to enhance its efficiency and stability.
Tools and Resources for a Smooth Transition
During the migration process, various tools and resources can help streamline the transition to Java 17. Here are some notable ones:
- IDEs: Integrated development environments like IntelliJ IDEA, Eclipse, and NetBeans provide comprehensive refactoring capabilities and code analysis tools that significantly aid in the migration process.
- Static Analysis Tools: Tools such as SonarQube, Checkstyle, and PMD can assist in identifying potential issues and providing suggestions for code improvements.
- Community Guides and Resources: Online communities such as Stack Overflow, Java User Groups (JUGs), and blogs are excellent sources of information and practical advice for migrating from Java 8 to Java 17. Engaging with the Java community can provide valuable insights and best practices.
Closing the Chapter
Java 17 marks a significant leap forward for the Java ecosystem, offering powerful features, enhanced performance, and improved security. While Java 8 has been a reliable and popular choice over the years, upgrading to Java 17 LTS can unlock a whole new realm of possibilities for businesses.
By upgrading to Java 17, organizations gain access to features like sealed classes, pattern matching for instanceof
, text blocks, and records, which enhance code readability, maintainability, and developer productivity. Additionally, Java 17 comes with other notable improvements that can boost performance, provide enhanced garbage collection, and improve security.
Migrating from Java 8 to Java 17 may pose challenges, but with careful planning, thorough testing, and the right tools and resources, businesses can navigate the transition successfully. It's important to update dependencies, refactor code, test rigorously, and optimize the application post-migration.
As businesses embark on the journey to Java 17, community engagement and knowledge sharing become invaluable. Whether it's sharing migration experiences, recommending useful tools, or providing tips for a smoother transition, the collective knowledge of the Java community can enrich the migration process and foster a strong sense of community around Java development.
So, are you ready to make the leap from Java 8 to Java 17? Share your experiences, tools, and tips to further enhance the knowledge base and join the thriving community embracing the future of Java development.