Top Tip to Hack-Proof Your Code: Secure Coding Secrets
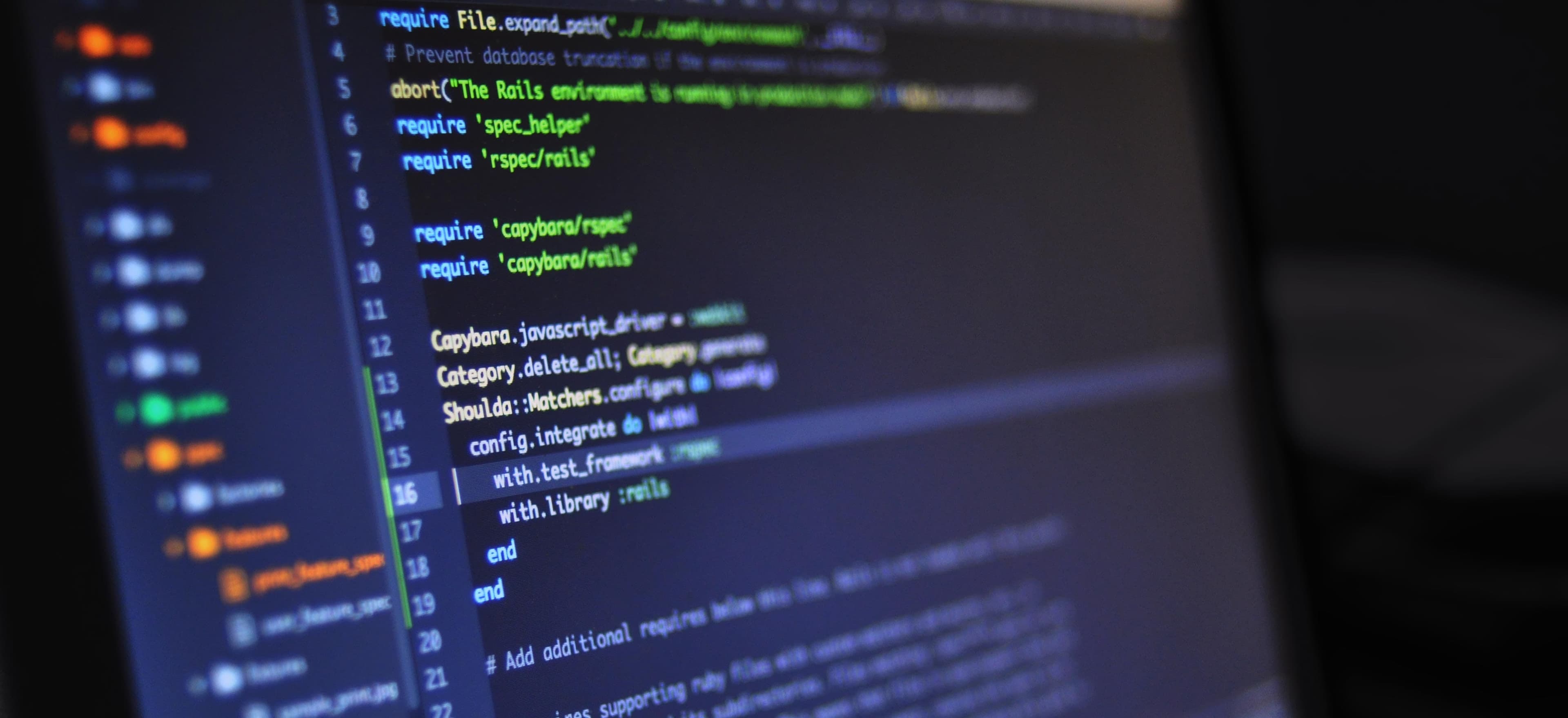
- Published on
Secure Your Java Application: Best Practices to Defend Against Hackers
In the vast and evolving landscape of technology, securing your Java application is not just an option, it's a necessity. Whether you're a seasoned developer or just starting, understanding and applying secure coding practices is vital to safeguard your application. In this post, we'll dive into the details of what secure coding in Java entails and why it's so crucial, along with actionable tips and code snippets to help you fortify your codebase against nefarious entities.
Why Secure Coding Matters in Java
Java, while robust and widely used, is not impervious to attacks. Common vulnerabilities like SQL injection, cross-site scripting (XSS), and insecure data storage can compromise your application’s integrity and your users' trust. Thankfully, with a proactive mindset and the right techniques, you can mitigate these risks.
Proactively Preventing Vulnerabilities
Security is often an afterthought in the development cycle. To build a secure Java application, it's imperative to incorporate security into every stage of software development. With that in mind, let’s explore some critical secure coding practices.
1. Validate Input Religiously
Input validation is your first line of defense. Whenever your application receives data, you need to check its validity. Proper validation can neutralize many threats, such as SQL injections and XSS attacks.
public boolean isValidUsername(String username) {
String pattern = "^[a-zA-Z0-9_.-]{5,20}$";
return username.matches(pattern);
}
This snippet verifies if a username only contains alphanumeric characters, dots, hyphens, and underscores, while also restricting its length. Understand the constraints of your data and craft validation rules accordingly.
2. Employ Prepared Statements for Database Queries
SQL injection is still alarmingly prevalent. One of the most effective remedies is using prepared statements with parameterized queries.
String query = "SELECT * FROM users WHERE email = ? AND password = ?";
try (Connection connection = dataSource.getConnection();
PreparedStatement preparedStatement = connection.prepareStatement(query)) {
preparedStatement.setString(1, email);
preparedStatement.setString(2, hashedPassword);
ResultSet resultSet = preparedStatement.executeQuery();
// Handle resultSet
}
By using prepared statements, you instruct the database to recognize the code and the data separately, making it much more challenging for attackers to inject malicious SQL.
3. Sanitize Data
When you can't validate as strictly as you’d like, sanitizing input and output is essential. For example, when dealing with user-generated HTML content, using a library like JSoup to clean up the HTML can prevent XSS attacks.
String unsafeHtml = "<script>alert('XSS')</script><p>Valid Content</p>";
String safeHtml = Jsoup.clean(unsafeHtml, Whitelist.basic());
// Only "Valid Content" inside <p> tags will be allowed
Sanitizing ensures that potentially hazardous elements are removed or neutralized.
4. Manage Dependencies Wisely
Your application’s security is only as good as the security of its dependencies. Regularly update your libraries and frameworks to patch known vulnerabilities. Tools like OWASP Dependency-Check can identify insecure dependencies so you can act quickly to update them.
5. Encrypt Sensitive Data
Never store sensitive information as plain text. Use encryption to protect data like passwords and personal information.
public String encryptData(String data, SecretKey key) throws GeneralSecurityException {
Cipher cipher = Cipher.getInstance("AES/CBC/PKCS5Padding");
cipher.init(Cipher.ENCRYPT_MODE, key);
byte[] encryptedBytes = cipher.doFinal(data.getBytes(StandardCharsets.UTF_8));
return Base64.getEncoder().encodeToString(encryptedBytes);
}
This function encrypts data using AES with reasonable settings. Always use a secure random key and keep it confidential.
6. Authenticate and Authorize Cautiously
Authentication, determining who a user is, and authorization, determining what they can do, are fundamental security aspects.
public boolean authenticateUser(String username, String passwordHash) {
User user = userRepository.findByUsername(username);
return user != null && user.getPasswordHash().equals(passwordHash);
}
public boolean hasAccess(User user, String resource) {
// Assume role-based access control
return user.getRoles().stream().anyMatch(role -> role.canAccess(resource));
}
Strictly verify user credentials and ensure they have appropriate permissions for each resource.
7. Enforce Secure Communication
Use TLS to secure data in transit. It’s a critical step to thwart man-in-the-middle attacks. Configure your server to use HTTPS instead of HTTP to encrypt web traffic.
8. Handle Exceptions and Logs Properly
Sensitive data can leak through error messages and logs. Always handle exceptions gracefully and sanitize logs.
try {
// risky operations
} catch (Exception e) {
logger.error("An error occurred: {}", e.getMessage());
response.sendError(HttpServletResponse.SC_INTERNAL_SERVER_ERROR, "An internal error occurred");
}
Provide generic error messages to the outside world while keeping detailed logs internally for diagnostics.
9. Implement Security Headers
Security headers are your silent guardians. They instruct browsers to treat your site's content with strict security measures, warding off various types of client-side attacks.
public void addSecurityHeaders(HttpServletResponse response) {
response.setHeader("Content-Security-Policy", "default-src 'self';");
response.setHeader("X-Content-Type-Options", "nosniff");
response.setHeader("X-Frame-Options", "DENY");
response.setHeader("X-XSS-Protection", "1; mode=block");
// Other headers as needed
}
10. Stay Vigilant and Informed
Lastly, keep up with security news and best practices. Follow trusted sources such as the OWASP Top 10 to stay informed about common vulnerabilities and threats.
Wrapping Up: Secure Code Is Your Responsibility
Secure coding in Java isn't just a set of rules; it's a mindset. By integrating the practices mentioned above, you’ll not only protect your application but also contribute to a safer digital world. Remember, every line of secure code is a barrier between hackers and your valuable data.
Security is a journey, not a destination. Embrace it, and watch both your skills and your applications flourish in the robust fortress you've built.