Ace the Loop: Master Java For-Each Interview Qs!
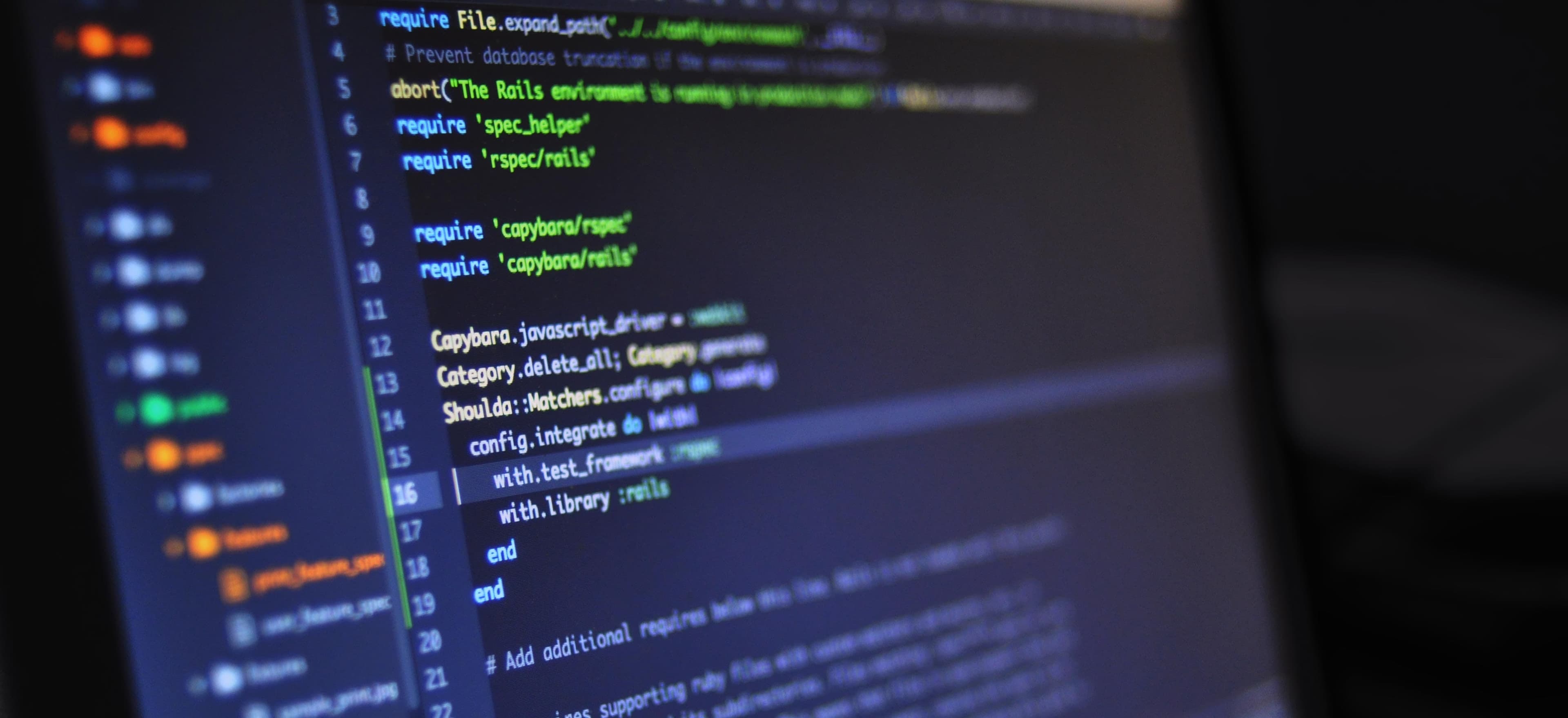
- Published on
Ace the Loop: Master Java For-Each Interview Qs!
A Brief Overview
Java developers, especially those preparing for interviews, often encounter questions related to the for-each loop. The for-each loop, introduced in Java 5, provides a simplified way to iterate over arrays and collections. In this blog post, we will explore the syntax and advantages of the for-each loop, and cover some common interview questions that can help you solidify your understanding of this topic.
Understanding the Java For-Each Loop
What is the For-Each Loop?
The for-each loop, also known as the enhanced for loop, is a looping construct introduced in Java 5. Its purpose is to simplify and streamline the process of iterating over arrays, collections, and other iterable objects. It provides a more readable and less error-prone alternative to the traditional for loop.
Syntax of the For-Each Loop
The syntax of the for-each loop is as follows:
for (type item : iterable) {
// code to be executed for each item
}
In this syntax:
type
is the type of the elements in the iterable.item
is a variable that represents each element in the iterable.iterable
is the array, collection, or any other iterable object that you want to iterate over.
The for-each loop automatically assigns each element of the iterable to the item
variable, and the code block inside the loop is executed for each element.
Let's take a simple example to understand the syntax better. Suppose we have an array of numbers and we want to print each number using a for-each loop:
int[] numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
System.out.println(num);
}
The above code will output:
1
2
3
4
5
Compared to the traditional for loop, the for-each loop provides a more concise and readable way to iterate over an array. It eliminates the need for maintaining an index variable and simplifies the code.
When to Use the For-Each Loop
The for-each loop is ideal for situations where you need to iterate over an entire collection or array and don't require the index of each element. It is particularly useful when working with collections, as it abstracts away the details of iteration and provides a more elegant way to process each element.
Advantages of Using For-Each
Using the for-each loop offers several advantages over the traditional for loop:
-
Readability: The syntax of the for-each loop is more concise and easier to understand than the traditional for loop. It clearly expresses the intent of iterating over each element in a collection or array.
-
Reduced Error Potential: The for-each loop reduces the chance of making errors in loop conditions, as it handles the iteration internally. Developers don't need to worry about indexing and avoiding off-by-one errors.
-
No ConcurrentModificationException: When iterating over immutable collections, the for-each loop provides immunity to the
ConcurrentModificationException
that can occur when modifying a collection while iterating over it. This is because the for-each loop uses an internal iterator that doesn't allow modifications.
It's important to note that the for-each loop is read-only; you can't modify the underlying collection or array while iterating using the for-each loop.
Common Interview Questions
Basic Questions
Q1: Explain the For-Each Loop
The for-each loop is a looping construct introduced in Java 5 that simplifies the process of iterating over arrays, collections, and other iterable objects. It iterates over each element in the iterable and automatically assigns it to a variable. Here's an example that demonstrates the for-each loop syntax:
int[] numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
System.out.println(num);
}
The above code will output each number in the numbers
array.
Q2: Can you modify the underlying collection/array while using a for-each loop?
No, it is not possible to directly modify the underlying collection or array while iterating using the for-each loop. If you try to modify the collection, it will result in a ConcurrentModificationException
. This exception occurs because the for-each loop uses an internal iterator that track changes in the collection during iteration. If a modification is detected, the exception is thrown to prevent potential inconsistencies in the iteration process.
Intermediate Questions
Q3: Compare For-Each with Iterator Pattern
The for-each loop and the Iterator
pattern are both used for iterating over collections in Java, but they have some differences. Here's a comparison between the two:
- The for-each loop is a simplified way to iterate over collections introduced in Java 5. It automatically handles the iteration process and abstracts away the details of using an iterator.
- The
Iterator
pattern, on the other hand, is a more flexible and powerful mechanism for iterating over collections. It provides additional methods likeremove()
to modify the collection during iteration, which is not possible with the for-each loop. - Use the for-each loop when you need to iterate over the entire collection and don't need to modify it during iteration. Use the
Iterator
pattern when you require more control and flexibility over the iteration process, such as removing elements while iterating or iterating over a subset of the collection.
In terms of performance, the for-each loop and the Iterator
pattern have similar performance characteristics.
Q4: How does for-each handle null elements in a collection?
The for-each loop handles null elements in a collection without any issues. During iteration, if an element in the collection is null, the for-each loop assigns the value null
to the corresponding variable. Here's an example that demonstrates this:
List<String> names = new ArrayList<>();
names.add("Alice");
names.add(null);
names.add("Bob");
for (String name : names) {
System.out.println(name);
}
The above code will output:
Alice
null
Bob
Advanced Questions
Q5: Is it possible to iterate over a Map with For-Each? How?
Yes, it is possible to iterate over a Map
using the for-each loop. To iterate over a map, you can use the entrySet()
method of the Map
interface, which returns a set view of the key-value pairs. Here's an example that demonstrates how to iterate over a map using the for-each loop:
Map<String, Integer> population = new HashMap<>();
population.put("New York", 8623000);
population.put("Los Angeles", 3990456);
population.put("Chicago", 2705994);
for (Map.Entry<String, Integer> entry : population.entrySet()) {
String city = entry.getKey();
int count = entry.getValue();
System.out.println(city + ": " + count);
}
The above code will output each city along with its population.
Q6: Performance implications of using For-Each in multi-threaded applications
When using the for-each loop in multi-threaded applications, there are potential performance considerations to keep in mind. The for-each loop is designed for a single-threaded environment and does not provide any built-in thread-safety guarantees. If the underlying collection or array is modified concurrently by multiple threads, it can lead to unexpected behavior and race conditions.
To ensure thread-safety when using the for-each loop in a multi-threaded context, you should use appropriate synchronization mechanisms, such as using locks or other concurrency control constructs.
Tips for Answering For-Each Loop Questions in Interviews
To effectively answer for-each loop questions during interviews, consider the following tips:
-
Understand the underlying operation: Have a clear understanding of how the for-each loop works and its purpose in simplifying iteration over arrays and collections.
-
Be aware of the collections framework: Familiarize yourself with the different types of collections in Java and their specific characteristics. Understand which collections are iterable and can be used with the for-each loop.
-
Practice the syntax: Practice writing code snippets that use the for-each loop to iterate over different types of iterable objects. Pay attention to the correct syntax and the placement of the colon and variable.
Practice with Examples
- Problem: Given an array of strings, write a for-each loop to print each string in reverse.
String[] names = {"Alice", "Bob", "Charlie"};
for (String name : names) {
for (int i = name.length() - 1; i >= 0; i--) {
System.out.print(name.charAt(i));
}
System.out.println();
}
Output:
ecilA
boB
eilrahC
- Problem: Write a for-each loop to calculate the sum of all the even numbers in a list of integers.
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
int sum = 0;
for (int num : numbers) {
if (num % 2 == 0) {
sum += num;
}
}
System.out.println("Sum of even numbers: " + sum);
Output:
Sum of even numbers: 30
To Wrap Things Up
The for-each loop is a powerful construct in Java that simplifies the process of iteration over arrays, collections, and other Iterable objects. Its advantages include improved code readability, reduced chances of errors, and immunity to ConcurrentModificationException
when used with immutable collections. By mastering the for-each loop and understanding its nuances, you'll be well-prepared to answer interview questions related to this topic.
Remember to practice regularly and familiarize yourself with the various scenarios in which the for-each loop can be used. The more comfortable you become with this loop, the better prepared you'll be for interviews and real-world Java programming challenges.