Securing Code on a Budget: SMEs Can Do It Too!
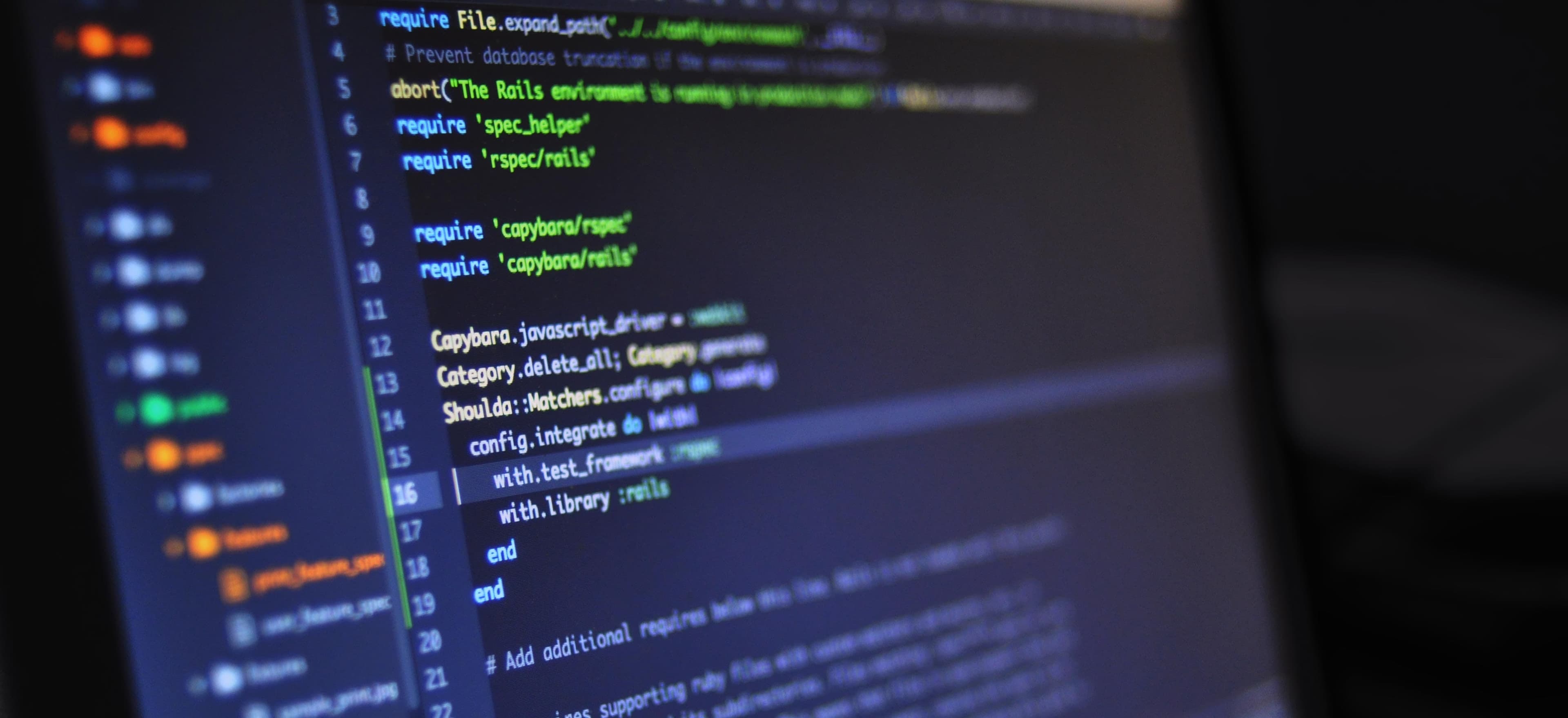
- Published on
Securing Code on a Budget: SMEs Can Do It Too!
In the digital era, an alarming number of cyber threats loom over businesses, especially small to medium-sized enterprises (SMEs). With tight budget constraints, SMEs often struggle to match the cybersecurity measures that larger corporations can afford. However, securing code isn't always about having the deepest pockets; it's about being smart, diligent, and resourceful. In this blog post, we'll explore some cost-effective strategies for SMEs to secure their Java code without breaking the bank.
Understanding the Importance of Secure Code
Cybersecurity isn't just a buzzword; it's a critical component of your business ecosystem. SMEs may think they are not likely targets for cyberattacks, but in truth, their often less-guarded systems make them ideal for attackers. The repercussions of a security breach can be huge, including financial losses, compromised customer data, and a tarnished reputation.
Java, being one of the most used programming languages, is frequently targeted by cybercriminals. The OWASP Foundation, an international non-profit organization dedicated to web security, often highlights how common vulnerabilities can be exploited in languages like Java.
Budget-Friendly Security Practices for Java Code
1. Embrace Secure Coding Standards
One of the most cost-effective approaches to secure coding is to write secure code from the get-go. This means following best practices and standards that help prevent common security vulnerabilities. Java developers should acquaint themselves with the Secure Coding Guidelines for Java SE provided by Oracle.
Example:
// Bad Practice: User input directly used in SQL query
String query = "SELECT * FROM users WHERE username = '" + username + "'";
// Good Practice: Prepared statements prevent SQL injection
PreparedStatement stmt = connection.prepareStatement("SELECT * FROM users WHERE username = ?");
stmt.setString(1, username);
ResultSet results = stmt.executeQuery();
Why: Prepared statements are crucial in preventing SQL injection attacks, a common and dangerous form of cyber assault where an attacker can inject malicious SQL code.
2. Perform Security Audits and Code Reviews
Frequent security audits and peer code reviews can catch potential security issues early. When budgets are limited, tools like FindBugs or its spiritual successor SpotBugs, which is open-source, can aid in identifying common security flaws in Java applications.
Example:
// A code flaw that might be identified by a security audit tool
public void doSomething(String input) {
Runtime.getRuntime().exec(input);
}
Why: Allowing direct execution of input without any sanitization or validation can lead to command injection vulnerabilities. Automated tools can often flag risky API calls like Runtime.exec()
.
3. Leverage Open Source Security Tools
Open-source doesn't mean low quality. There are numerous open-source security tools tailored for Java applications that can match the efficiency of their paid counterparts. For instance, the OWASP Dependency-Check can analyze project dependencies and identify any known vulnerabilities.
Example:
To incorporate OWASP Dependency-Check in a Maven project, add the following to your pom.xml
file:
<plugin>
<groupId>org.owasp</groupId>
<artifactId>dependency-check-maven</artifactId>
<version>INSERT_LATEST_VERSION</version>
</plugin>
Why: Keeping third-party dependencies up-to-date and free of vulnerabilities is critical, as outdated components are a common vector for attacks.
4. Educate Your Team
A well-informed team is your first line of defense. Invest in training your developers in secure coding practices. Many free resources and low-cost training platforms are available online to brush up on Java security knowledge.
5. Use Built-in Java Security Features
Java comes packed with a variety of built-in security features. Don't reinvent the wheel; use these robust libraries to manage critical aspects like authentication, authorization, cryptography, and session management.
Example:
// Secure random number generation
SecureRandom secureRandom = new SecureRandom();
// Use Java's cryptographic libraries for password hashing
MessageDigest md = MessageDigest.getInstance("SHA-256");
byte[] hashedPassword = md.digest(password.getBytes(StandardCharsets.UTF_8));
Why: Cryptographically secure random numbers are essential for token generation, and using Java's built-in MessageDigest
class for hashing is far safer than attempting to roll out your own cryptographic algorithms.
6. Implement Proper Exception Handling
Revealing too much information in error messages can lead to information leakage. Ensure that your exception handling is secure and doesn't expose sensitive system data.
Example:
try {
// Risky operation
} catch (Exception e) {
// Bad Practice: e.printStackTrace can reveal inner workings
e.printStackTrace();
// Good Practice: Log the exception and show a generic error message
logger.log(Level.SEVERE, "An error occurred", e);
response.sendError(HttpServletResponse.SC_INTERNAL_SERVER_ERROR, "An unexpected error occurred");
}
Why: This pattern ensures that while the developers get the information they need for debugging (through logging), users only see generic error messages that don't reveal anything exploitable.
Final Considerations
For SMEs, securing Java code efficiently and budget-friendly is not a luxury but a necessity. By adopting secure coding practices, investing in security audits and peer reviews, leveraging free or open-source security tools, training teams, and utilizing Java's own security features, SMEs can greatly enhance their cybersecurity posture. Implementing proper exception handling also ensures that errors don't become vulnerabilities. Remember, security is not a one-time action but an ongoing process.
Securing your enterprise's Java code requires continual effort and attention. With these strategies in play, you can bolster your defenses and protect your business without incurring exorbitant expenses. Always stay updated with the latest security trends and threats, and strive to evolve your security measures. It's not just about protecting code; it's about safeguarding your business's future.