Boost Your Graphs: Choosing Between Neo4j Specific vs Generic Relationships
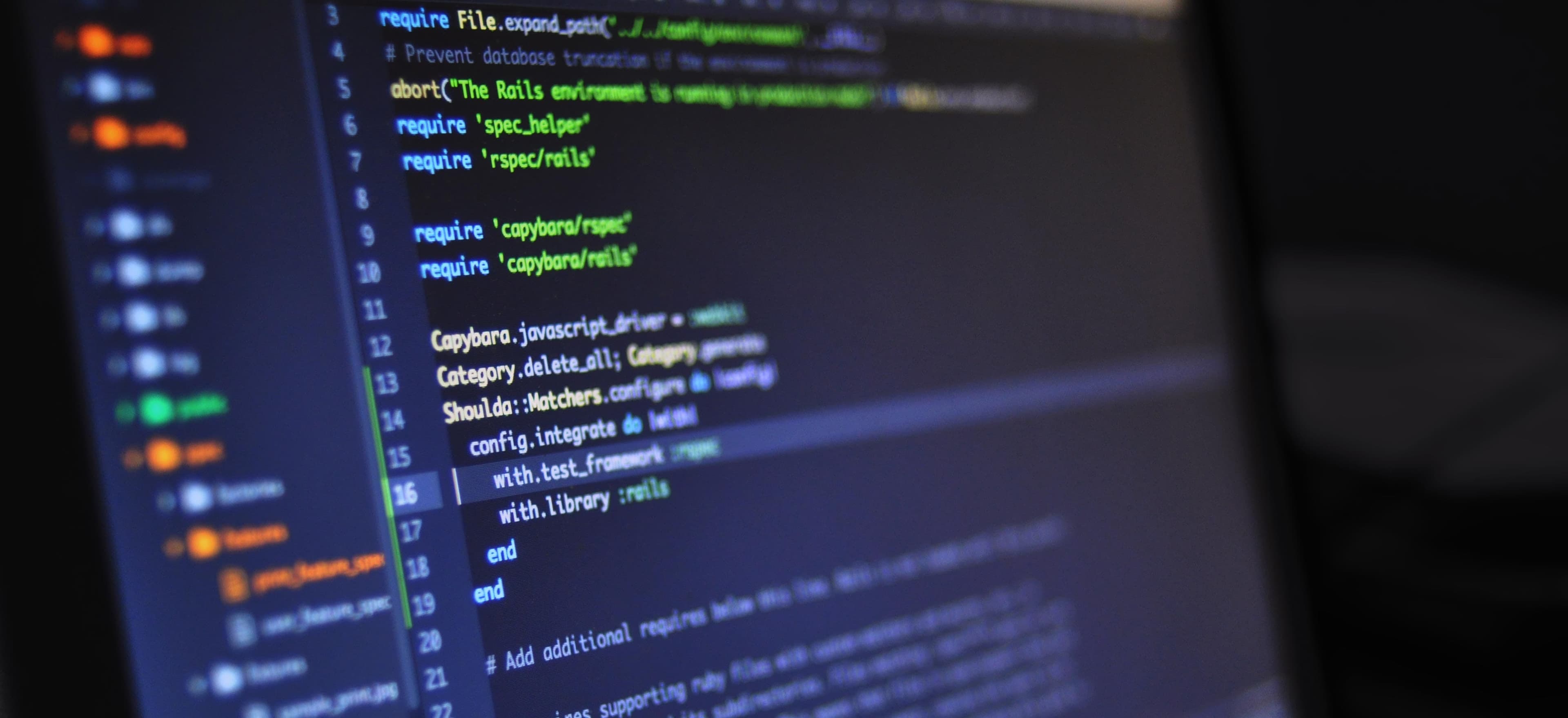
- Published on
Boost Your Graphs: Choosing Between Neo4j Specific vs Generic Relationships
When it comes to working with graph databases like Neo4j, one of the key decisions you need to make is how to model your relationships. Neo4j provides two approaches for modeling relationships - specific relationships and generic relationships. Both approaches have their advantages and disadvantages, and understanding the trade-offs is crucial for choosing the right approach for your use case.
In this article, we will explore the differences between specific and generic relationships in Neo4j, discuss their pros and cons, and provide guidelines on when to use each approach.
Specific Relationships
Specific relationships in Neo4j are relationships that have a specific type defined during modeling. In other words, you explicitly define the relationship type when creating the relationship between nodes. For example, you might have relationship types like "FOLLOWS", "LIKES", or "WORKS_AT".
To create a specific relationship in Neo4j, you use the CREATE
or MERGE
clause with the relationship type specified. Here's an example:
// Create a specific relationship
CREATE (u1:User)-[:FOLLOWS]->(u2:User)
Specific relationships offer several benefits:
1. Improved Query Performance
One of the main advantages of specific relationships is improved query performance. Since the relationship type is known and fixed, Neo4j can optimize the query execution plan based on the specific relationship type. This can result in faster query execution times compared to generic relationships.
2. Clearer and More Expressive Data Model
By using specific relationships, you can create a more expressive and meaningful data model. Relationship types act as additional information that enriches your graph, making it easier to understand the semantics of the data. This can be particularly useful when working with complex domains or when you need to enforce certain constraints on the relationships.
3. Better Query Readability and Maintainability
Specific relationships contribute to better query readability and maintainability. When working with specific relationships, your queries become more self-explanatory as the relationship types provide context and meaning to the queries. This makes it easier for developers to understand and maintain the queries over time.
However, specific relationships also have some limitations:
1. Increased Schema Complexity
Using specific relationships can lead to increased schema complexity. As your graph grows in size and complexity, you might end up with a large number of specific relationship types to cover all the possible relationships between nodes. This can make the schema harder to manage and maintain.
2. Limited Flexibility
Another drawback of specific relationships is their limited flexibility. Once you define a specific relationship type, you need to stick with it. This can be problematic if the relationship type needs to evolve or change over time. Modifying or extending specific relationships can be challenging and may require schema changes and data migration.
Generic Relationships
On the other hand, generic relationships in Neo4j are relationships that don't have a specific type defined during modeling. Instead, the relationship type is determined dynamically based on properties or labels attached to the relationship.
To create a generic relationship in Neo4j, you omit the relationship type in the CREATE
or MERGE
clause. Here's an example:
// Create a generic relationship
CREATE (u1:User)-[r]->(u2:User)
Generic relationships offer some advantages as well:
1. Simplified Schema
One of the main benefits of generic relationships is a simplified schema. Instead of having a large number of specific relationship types, you can create a more generic schema by using a single relationship type for multiple purposes. This can make the schema more flexible and easier to manage as your graph evolves.
2. Dynamic Relationship Type
With generic relationships, you have the flexibility to determine the relationship type dynamically. This can be useful when the relationship type is not known in advance or when it needs to be calculated based on certain conditions or properties of the nodes. Generic relationships allow you to model more complex and dynamic relationships in your graph.
However, generic relationships also have some drawbacks:
1. Performance Impact
Compared to specific relationships, generic relationships can have a performance impact on query execution. Since the relationship type is not known in advance, Neo4j may need to perform additional lookups or scans to determine the correct relationship type at runtime. This can result in slower query execution times, especially when dealing with large graphs.
2. Reduced Query Readability and Maintainability
Generic relationships may lead to reduced query readability and maintainability. Without the explicit relationship types, queries become less self-explanatory and can be harder to understand, especially when working with complex data models. This can make it more challenging for developers to work with and maintain the queries over time.
Choosing Between Specific and Generic Relationships
Now that we have discussed the pros and cons of specific and generic relationships in Neo4j, let's talk about when to use each approach.
Use Specific Relationships When:
- Query performance is a critical factor, and you want to optimize query execution by leveraging the specific relationship types.
- Your data model requires clear and expressive relationships to represent the semantics of the domain.
- Query readability and maintainability are important, and you want your queries to be self-explanatory.
Use Generic Relationships When:
- Schema simplicity is a priority, and you want to avoid managing a large number of specific relationship types.
- You need the flexibility to determine the relationship type dynamically based on runtime conditions or properties.
- Performance is not a primary concern, and the benefits of the dynamic relationship type outweigh the potential performance impact.
It's important to note that specific and generic relationships are not mutually exclusive. You can use a combination of both approaches within your graph database, based on the specific needs of your use case.
Closing Remarks
Choosing between specific and generic relationships in Neo4j is an important decision that can significantly impact the performance, maintainability, and flexibility of your application. By understanding the trade-offs and considering the characteristics of your data model, you can make an informed decision that best suits your use case.
Remember to prioritize factors like performance, schema complexity, query readability, and the need for dynamic relationship types when making your choice. And don't forget that you can always adapt and evolve your data model as your application grows and requirements change.
For more information on working with relationships in Neo4j, you can refer to the official documentation. Happy graph modeling!