Revamping Java EE: Tackling Outdated Design Patterns
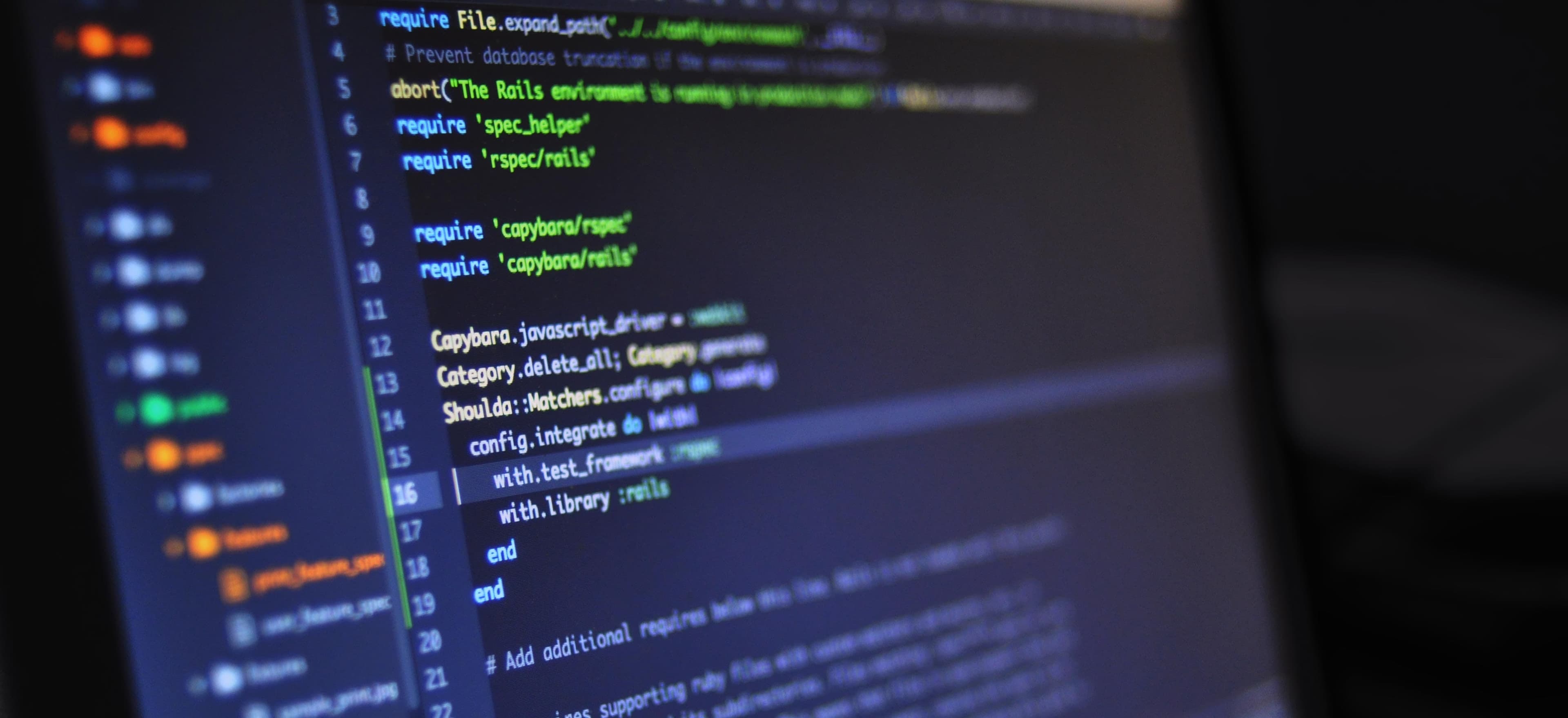
- Published on
Revamping Java EE: Tackling Outdated Design Patterns
Setting the Stage:
In the world of enterprise software development, Java EE (Enterprise Edition) has been a significant player for many years. It has provided a robust and scalable platform for building large-scale applications. However, as technology continues to evolve, Java EE is faced with the challenge of keeping up with the changing landscape. In this article, we will explore the outdated design patterns in Java EE and discuss modern alternatives to revamp the platform.
What is Java EE?
Java EE, formerly known as J2EE (Java 2 Platform, Enterprise Edition), is a highly popular platform used for developing enterprise-scale applications. It provides a set of specifications and APIs that enable developers to create scalable, secure, and transactional applications. Java EE is based on Java SE (Standard Edition) and extends its capabilities to support enterprise-level features.
Java EE is built around various design patterns and follows a component-based architecture. It offers a vast ecosystem of frameworks, libraries, and tools to simplify the development process and enhance productivity.
Common Outdated Java EE Design Patterns
Over the years, certain design patterns in Java EE have become outdated due to their limitations and inefficiencies. Let's take a look at some of these patterns and discuss their challenges.
Singleton Session Beans
Singleton Session Beans were widely used in earlier versions of Java EE for managing stateful components. These beans retained state across multiple method invocations, allowing them to serve as a central point of access for multiple clients. However, they suffered from significant drawbacks. They lacked flexibility and were difficult to test and maintain. The tight coupling between the Singleton Session Bean and its clients made it challenging to introduce changes without affecting the entire system.
J2EE Blueprints
J2EE Blueprints were architectural patterns that provided guidelines for building large-scale, monolithic applications using Java EE. They focused on designing applications as a cohesive whole, with tightly coupled components. While this approach worked well for certain scenarios, it had limitations in terms of scalability and flexibility. Updating or scaling specific parts of the application became challenging due to the tightly coupled nature of the components.
Entity Beans
Entity Beans were used for implementing the Object-Relational Mapping (ORM) in Java EE applications. They aimed to provide a seamless integration between the Java objects and the underlying database. However, the implementation of Entity Beans suffered from performance issues and complicated configuration. Managing relationships between entities and dealing with complex queries became cumbersome, leading to performance bottlenecks and decreased productivity.
Modern Alternatives to Outdated Patterns
To address the limitations and challenges of outdated Java EE design patterns, modern alternatives have emerged that provide better solutions. Let's explore some of these alternatives and understand how they tackle the inefficiencies of the old patterns.
Context and Dependency Injection (CDI)
CDI is a modern dependency injection framework that offers flexible and loosely coupled components in Java EE applications. It eliminates the need for Singleton Session Beans by providing a more dynamic and customizable approach to managing stateful components. CDI allows for the contextual instantiation and management of beans, which leads to greater flexibility and easier testing. It also promotes better separation of concerns by allowing for the injection of dependencies into the components.
// CODE SNIPPET: CDI example
@ApplicationScoped
public class InventoryService {
// ...
}
In the above example, the CDI annotation @ApplicationScoped
signifies that the InventoryService
is a stateless business component. It can be easily injected into other components, promoting loose coupling and reusability.
Java Persistence API (JPA)
JPA is a modern ORM framework that provides a simplified and efficient way of mapping Java objects to a relational database. It offers a high-level, object-oriented approach to database access and eliminates the need for complex Entity Beans. JPA provides annotations to define the persistence mappings, relationships, and queries, making it easier to work with data persistence.
// CODE SNIPPET: JPA example
@Entity
@Table(name = "inventory")
public class Inventory {
// ...
}
In the above example, the @Entity
annotation marks the Inventory
class as a persistent entity. JPA automatically maps the Java objects to the corresponding database table and provides convenient methods for CRUD operations.
These modern alternatives, CDI and JPA, are just a few examples of how Java EE has evolved to address the limitations of outdated design patterns. They bring improved performance, flexibility, and ease of use to Java EE applications.
Code Snippets and Commentary
To provide a better understanding of the modern alternatives, let's compare specific outdated patterns with their modern counterparts.
Singleton Session Beans vs. CDI
Outdated Pattern: Singleton Session Beans
// CODE SNIPPET: Singleton Session Bean example
@Singleton
public class InventoryService {
// ...
}
Singleton Session Beans were once used to manage stateful components. However, they suffered from inflexibility and were challenging to test and maintain.
Modern Pattern: CDI
// CODE SNIPPET: CDI example
@ApplicationScoped
public class InventoryService {
// ...
}
CDI provides a more dynamic and flexible approach to managing stateful components. The @ApplicationScoped
annotation signifies that the InventoryService
is a stateless business component.
CDI offers benefits such as easier testing, improved performance, and better integration with modern Java EE applications.
J2EE Blueprints vs. Microservices
Outdated Pattern: J2EE Blueprints
// CODE SNIPPET: J2EE Blueprint example
public class MonolithicApplication {
// ...
}
J2EE Blueprints promoted the monolithic approach to building large-scale applications in Java EE. While this approach had its merits, it posed challenges in terms of scalability and flexibility.
Modern Pattern: Microservices Architectures
// CODE SNIPPET: Microservices example
@Path("/inventory")
public class InventoryResource {
// ...
}
Microservices architecture, on the other hand, focuses on breaking down large applications into smaller, independent services. Each service is responsible for a specific business domain and can be independently developed, deployed, and scaled.
Microservices offer advantages such as improved scalability, flexibility, and alignment with continuous delivery and deployment practices.
Migrating From Old to New
Migrating from outdated design patterns to modern alternatives requires careful planning and implementation. Here's a step-by-step guide to help with the transition:
-
Analyze the existing application: Evaluate the current design patterns in use and identify the potential candidates for modernization.
-
Evaluate the benefits: Understand the benefits that the modern alternatives offer and how they align with your application's requirements and goals.
-
Design the migration strategy: Create a roadmap for migrating the components from old patterns to new ones. Determine the sequencing and dependencies between the components.
-
Refactor the code: Modify the existing components based on the new patterns. Update the annotations, refactor the codebase, and ensure proper integration with the modern alternatives.
-
Update configuration and dependencies: Update the configuration files and dependencies to align with the modern alternatives. This might involve modifying the build scripts, dependency management tools, and deployment configurations.
-
Test thoroughly: Implement comprehensive unit and integration tests to ensure that the migrated components function as expected. Perform regression testing to identify any unforeseen issues.
-
Deploy and monitor: Deploy the updated application in a controlled environment and monitor its performance. Keep an eye out for any issues or bottlenecks that may have been introduced during the migration process.
It's essential to follow best practices during the migration process. Utilize version control systems to track changes, incorporate automated testing strategies, and consider deployment considerations such as containerization or cloud-native architectures.
The Last Word
Java EE has been a fundamental platform for enterprise software development for many years. However, to keep up with the evolving technology landscape, it is crucial to revamp the outdated design patterns that Java EE relies on. Migrating from old patterns to modern alternatives can bring numerous benefits such as improved flexibility, scalability, and performance.
In this article, we explored several outdated design patterns in Java EE and discussed modern alternatives such as CDI and JPA. We also provided code snippets and comparisons to highlight the differences between these patterns. Additionally, we offered a step-by-step guide to help with the migration process.
It is imperative for Java EE developers to embrace change and modernize their applications. By adopting modern design patterns and frameworks, developers can create more efficient, maintainable, and scalable applications that align with current industry standards.
So, take the leap and revamp your Java EE applications with modern design patterns for a brighter and more prosperous future.
Checkout our other articles