Java 11 Preview: Unveiling the Latest Features!
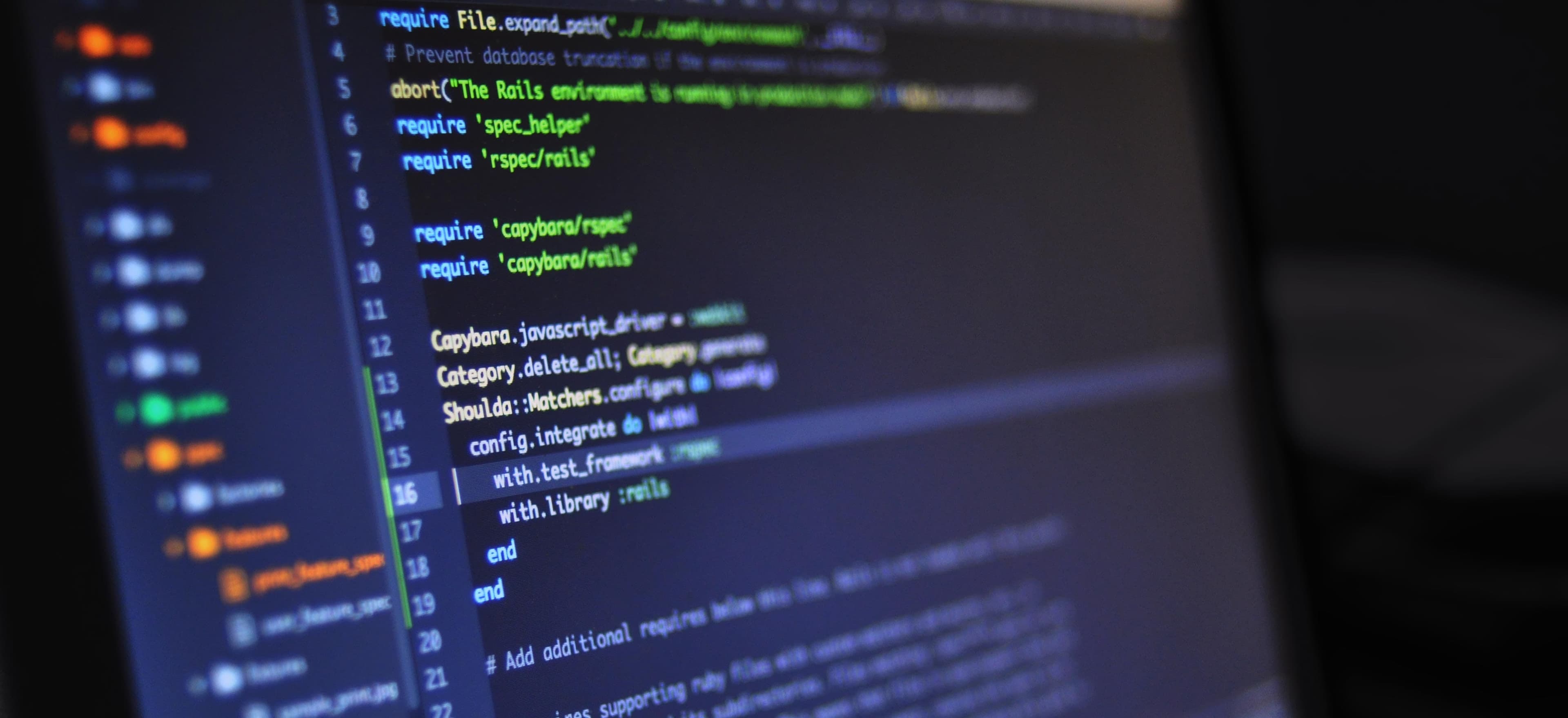
- Published on
Java 11 Preview: Unveiling the Latest Features!
Java, the ever-popular programming language, has been a dominating force in the software development industry for decades. With its robustness, portability, and vast ecosystem, Java has proven time and time again to be a reliable choice for developers.
In September 2018, Java 11 was released, bringing along a slew of new features and enhancements. In this article, we'll take a closer look at some of the most exciting additions to the Java 11 ecosystem.
1. Local-Variable Syntax for Lambda Parameters
One of the most significant changes in Java 11 is the introduction of the var
keyword for lambda parameters. Until now, lambda expressions required explicit typing for their parameters, which could make the code more verbose and harder to read.
With Java 11, you can now use the var
keyword in lambda expressions, allowing for a more concise and expressive coding style. Let's take a look at an example:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
names.forEach((var name) -> System.out.println(name));
In this code snippet, the var
keyword replaces the explicit type of the lambda parameter. This feature improves code readability, especially when dealing with complex lambda expressions.
2. Epsilon: A No-Op GC
Java 11 introduces a new experimental garbage collector called Epsilon. Epsilon is designed to be a no-op garbage collector, meaning it doesn't perform any actual garbage collection. This feature is primarily targeted towards applications that don't require memory management, such as testing or benchmarking frameworks.
The Epsilon garbage collector can be enabled using the -XX:+UnlockExperimentalVMOptions -XX:+UseEpsilonGC
flags when launching a Java application.
Although Epsilon doesn't provide any memory management, it can be useful for reducing the overhead of garbage collection, especially in scenarios where memory allocation and deallocation are not critical.
3. Nest-Based Access Control
Java 11 introduces nest-based access control, a feature that aims to improve the security and encapsulation of nested classes. In previous versions of Java, nested classes had access to private members of their enclosing classes. However, this could lead to potential security vulnerabilities if the nested class was subclassed or overriden.
With nest-based access control, the JVM enforces a stronger access control among nested classes. This means that only the nestmate classes have access to each other's private members, preventing unintended access from outside the nest.
class Outer {
private String outerPrivateField;
class Inner {
private String innerPrivateField;
}
}
In this example, the Outer
class and the Inner
class are considered nestmates. They can access each other's private members, but classes outside the nest cannot access these private fields.
4. HTTP Client API
Java 11 comes with a new built-in HTTP client API to support both synchronous and asynchronous communication with HTTP servers. This API replaces the old HttpURLConnection
class and provides a more intuitive and flexible way of working with HTTP requests and responses.
The new HTTP client API supports HTTP/1.1 and HTTP/2 protocols, as well as WebSocket. It also includes features such as request/response streaming, automatic redirect following, and support for asynchronous processing.
Here's an example of how to make a simple HTTP GET request using the new API:
import java.net.URI;
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
public class HttpClientExample {
public static void main(String[] args) throws Exception {
HttpClient httpClient = HttpClient.newHttpClient();
HttpRequest httpRequest = HttpRequest.newBuilder()
.uri(new URI("http://example.com"))
.GET()
.build();
HttpResponse<String> httpResponse = httpClient.send(httpRequest, HttpResponse.BodyHandlers.ofString());
System.out.println(httpResponse.body());
}
}
This code snippet demonstrates how easy it is to send an HTTP GET request and retrieve the response using the new HTTP client API. With this new addition, Java developers no longer need external libraries or frameworks to handle HTTP communication.
5. Launch Single-File Source-Code Programs
Starting from Java 11, you can run single-file Java source code programs directly using the java
command, without the need for a separate compilation step. This feature is especially useful for quick prototyping, scripting, or small-scale applications.
To run a single-file Java program, you simply pass the source file to the java
command:
$ java HelloWorld.java
In previous versions of Java, you would need to run javac
to compile the source file into bytecode before executing it with java
. This new feature streamlines the process, making it more convenient for simple development tasks.
Lessons Learned
Java 11 brings a wide range of enhancements and new features to the table. From lambda expressions to garbage collection and HTTP client improvements, the latest version of Java continues to evolve and improve developer productivity.
The new features in Java 11 offer enhanced flexibility, security, and performance for both new and existing codebases. By staying up to date with the latest Java releases, developers can take full advantage of these features and make their code more concise and efficient.
If you're interested in learning more about Java 11 and its features, be sure to check out the official Java SE 11 Documentation and explore the possibilities that this powerful programming language has to offer.
Checkout our other articles