Overcome Spring Batch CSV Parsing Hurdles Effortlessly
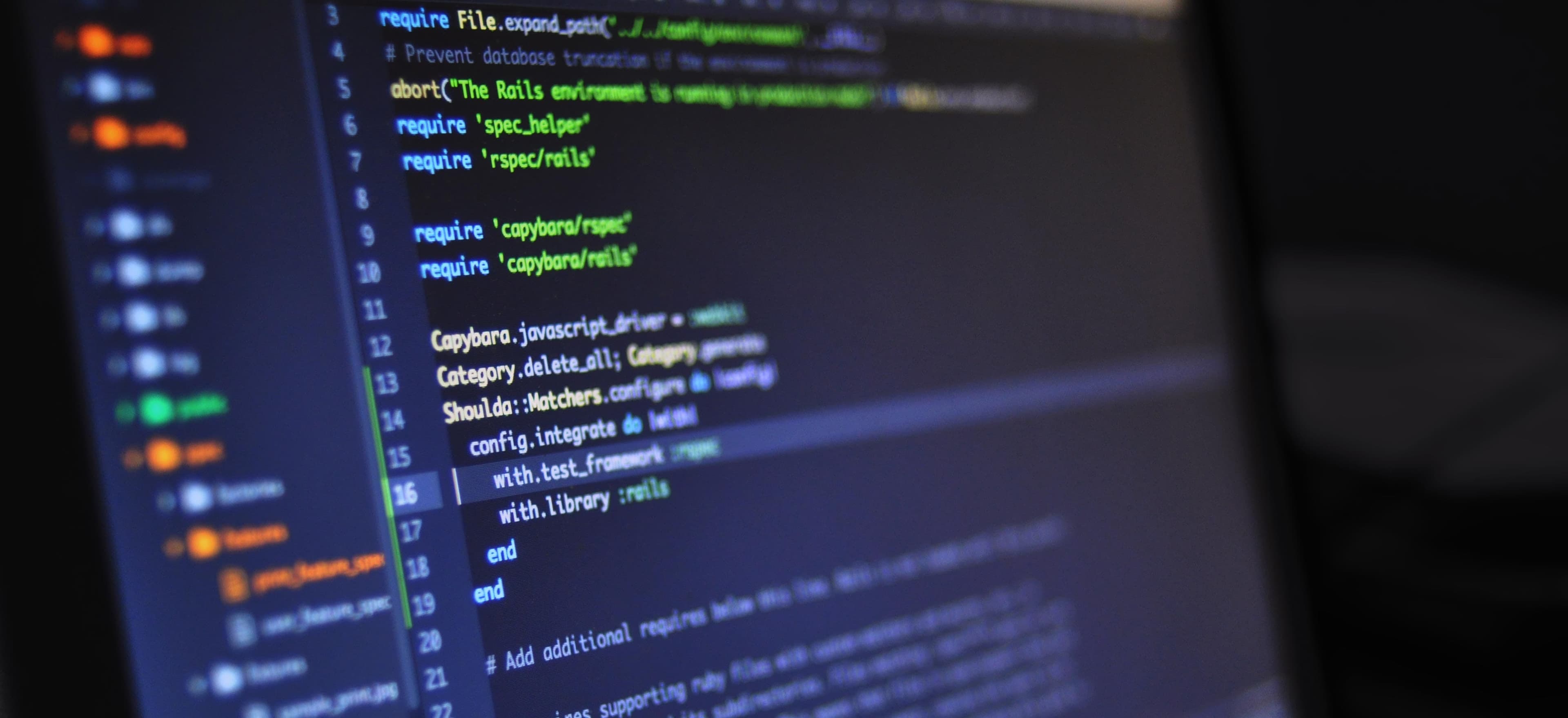
- Published on
Overcome Spring Batch CSV Parsing Hurdles Effortlessly
Managing large amounts of data can be a challenging task, especially when dealing with CSV files. Spring Batch, a lightweight framework built on top of the popular Spring Framework, provides a powerful solution for handling batch processing tasks. However, parsing CSV files with Spring Batch can sometimes become a hurdle. In this blog post, we will explore some common CSV parsing challenges and demonstrate how to overcome them effortlessly.
Understanding the CSV Format
Before diving into the challenges, it's important to have a clear understanding of the CSV (Comma-Separated Values) format. CSV files consist of rows and columns, with each row representing a set of data elements separated by commas (or other delimiters). The first row often contains headers defining the names of the columns.
For example, consider the following CSV file:
Name,Age,City
John,25,New York
Mary,30,San Francisco
In this example, the headers are "Name", "Age", and "City", and we have two data rows representing two individuals.
Challenge 1: Handling Different Delimiters
While CSV files typically use commas as delimiters, it's not uncommon to encounter files that use different delimiters such as tabs or semicolons. Thankfully, Spring Batch provides a flexible way to handle different delimiters.
To specify a custom delimiter, we can use the DelimitedLineTokenizer
class and set its delimiter
property accordingly. Let's take a look at an example:
@Bean
public FlatFileItemReader<Person> itemReader() {
FlatFileItemReader<Person> reader = new FlatFileItemReader<>();
reader.setResource(new ClassPathResource("data.csv"));
DefaultLineMapper<Person> lineMapper = new DefaultLineMapper<>();
DelimitedLineTokenizer tokenizer = new DelimitedLineTokenizer();
tokenizer.setDelimiter(";"); // Set the delimiter to semicolon
tokenizer.setNames("name", "age", "city");
BeanWrapperFieldSetMapper<Person> fieldSetMapper = new BeanWrapperFieldSetMapper<>();
fieldSetMapper.setTargetType(Person.class);
lineMapper.setLineTokenizer(tokenizer);
lineMapper.setFieldSetMapper(fieldSetMapper);
reader.setLineMapper(lineMapper);
return reader;
}
In this example, we create a DelimitedLineTokenizer
and set its delimiter to semicolon. We then specify the names of the columns using the setNames
method. Finally, we configure the DelimitedLineTokenizer
with the DefaultLineMapper
and the BeanWrapperFieldSetMapper
to map the CSV data to our Person
object.
Challenge 2: Ignoring Header Rows
In many cases, CSV files contain header rows that we don't want to process as actual data. Spring Batch provides an easy way to skip header rows without requiring any manual intervention.
To skip header rows, we can use the DefaultLineMapper
class and its linesToSkip
property. Let's modify our previous example to skip the header row:
@Bean
public FlatFileItemReader<Person> itemReader() {
FlatFileItemReader<Person> reader = new FlatFileItemReader<>();
reader.setResource(new ClassPathResource("data.csv"));
DefaultLineMapper<Person> lineMapper = new DefaultLineMapper<>();
DelimitedLineTokenizer tokenizer = new DelimitedLineTokenizer();
tokenizer.setDelimiter(";");
tokenizer.setNames("name", "age", "city");
BeanWrapperFieldSetMapper<Person> fieldSetMapper = new BeanWrapperFieldSetMapper<>();
fieldSetMapper.setTargetType(Person.class);
lineMapper.setLineTokenizer(tokenizer);
lineMapper.setFieldSetMapper(fieldSetMapper);
lineMapper.setLinesToSkip(1); // Skip the first line (header row)
reader.setLineMapper(lineMapper);
return reader;
}
By setting the linesToSkip
property of the DefaultLineMapper
to 1, we instruct Spring Batch to skip the first line.
Challenge 3: Handling Missing Columns
Another common challenge when parsing CSV files is dealing with missing or optional columns. If a column is missing in a row, it can cause parsing errors or result in unexpected behavior.
To handle missing columns gracefully, we can use the FieldSetMapper
interface provided by Spring Batch. This interface allows us to customize how the CSV data is mapped to our domain objects.
Let's look at an example where the "City" column is optional, and if it's missing, we want to set it to a default value:
public class PersonFieldSetMapper implements FieldSetMapper<Person> {
@Override
public Person mapFieldSet(FieldSet fieldSet) {
Person person = new Person();
person.setName(fieldSet.readString("name"));
person.setAge(fieldSet.readInt("age"));
String city = fieldSet.readString("city");
person.setCity(StringUtils.isEmpty(city) ? "Unknown" : city);
return person;
}
}
@Bean
public FlatFileItemReader<Person> itemReader() {
FlatFileItemReader<Person> reader = new FlatFileItemReader<>();
reader.setResource(new ClassPathResource("data.csv"));
DefaultLineMapper<Person> lineMapper = new DefaultLineMapper<>();
DelimitedLineTokenizer tokenizer = new DelimitedLineTokenizer();
tokenizer.setDelimiter(";");
tokenizer.setNames("name", "age", "city");
lineMapper.setLineTokenizer(tokenizer);
lineMapper.setFieldSetMapper(new PersonFieldSetMapper());
reader.setLineMapper(lineMapper);
return reader;
}
In this example, we create a custom PersonFieldSetMapper
that implements the FieldSetMapper
interface. Inside the mapFieldSet
method, we read the "name" and "age" fields as usual. For the "city" field, we check if it's empty using StringUtils.isEmpty
from the Apache Commons Lang library. If it's empty, we set it to the default value "Unknown".
By customizing the FieldSetMapper
, we can handle missing columns in a flexible and elegant way.
Challenge 4: Handling Quotes and Escaping
CSV files often include values that are enclosed in quotes, and can also contain characters that need to be escaped, such as the delimiter itself. Spring Batch provides built-in support for handling quotes and escaping in CSV files.
To handle quotes and escaping, we need to create a custom DelimitedLineTokenizer
and set its QuoteCharacter
and EscapeCharacter
properties. Let's take a look at an example:
@Bean
public FlatFileItemReader<Person> itemReader() {
FlatFileItemReader<Person> reader = new FlatFileItemReader<>();
reader.setResource(new ClassPathResource("data.csv"));
DefaultLineMapper<Person> lineMapper = new DefaultLineMapper<>();
CustomDelimitedLineTokenizer tokenizer = new CustomDelimitedLineTokenizer();
tokenizer.setDelimiter(",");
tokenizer.setNames("name", "age", "city");
tokenizer.setQuoteCharacter('"'); // Set the quote character
tokenizer.setEscapeCharacter('\\'); // Set the escape character
lineMapper.setLineTokenizer(tokenizer);
lineMapper.setFieldSetMapper(new BeanWrapperFieldSetMapper<>() {{
setTargetType(Person.class);
}});
reader.setLineMapper(lineMapper);
return reader;
}
In this example, we create a custom CustomDelimitedLineTokenizer
that extends DelimitedLineTokenizer
and set the QuoteCharacter
and EscapeCharacter
properties. We set the quote character to double quotation marks (") and the escape character to a backslash ().
With this configuration, Spring Batch will handle quotes and escaping properly when parsing the CSV file.
A Final Look
CSV parsing can be a challenging task, but with Spring Batch, we can overcome these hurdles effortlessly. By understanding the CSV format, handling different delimiters, ignoring header rows, handling missing columns, and handling quotes and escaping, we can effectively parse CSV files and process the data using Spring Batch.
Spring Batch provides a robust and flexible framework for handling batch processing tasks, and by employing its features properly, we can tackle complex data processing requirements with ease.
So, the next time you encounter CSV parsing challenges, don't fret! Spring Batch has got you covered. Happy coding!
Resources: