JAXB Magic: Streamline XML Without Annotations!
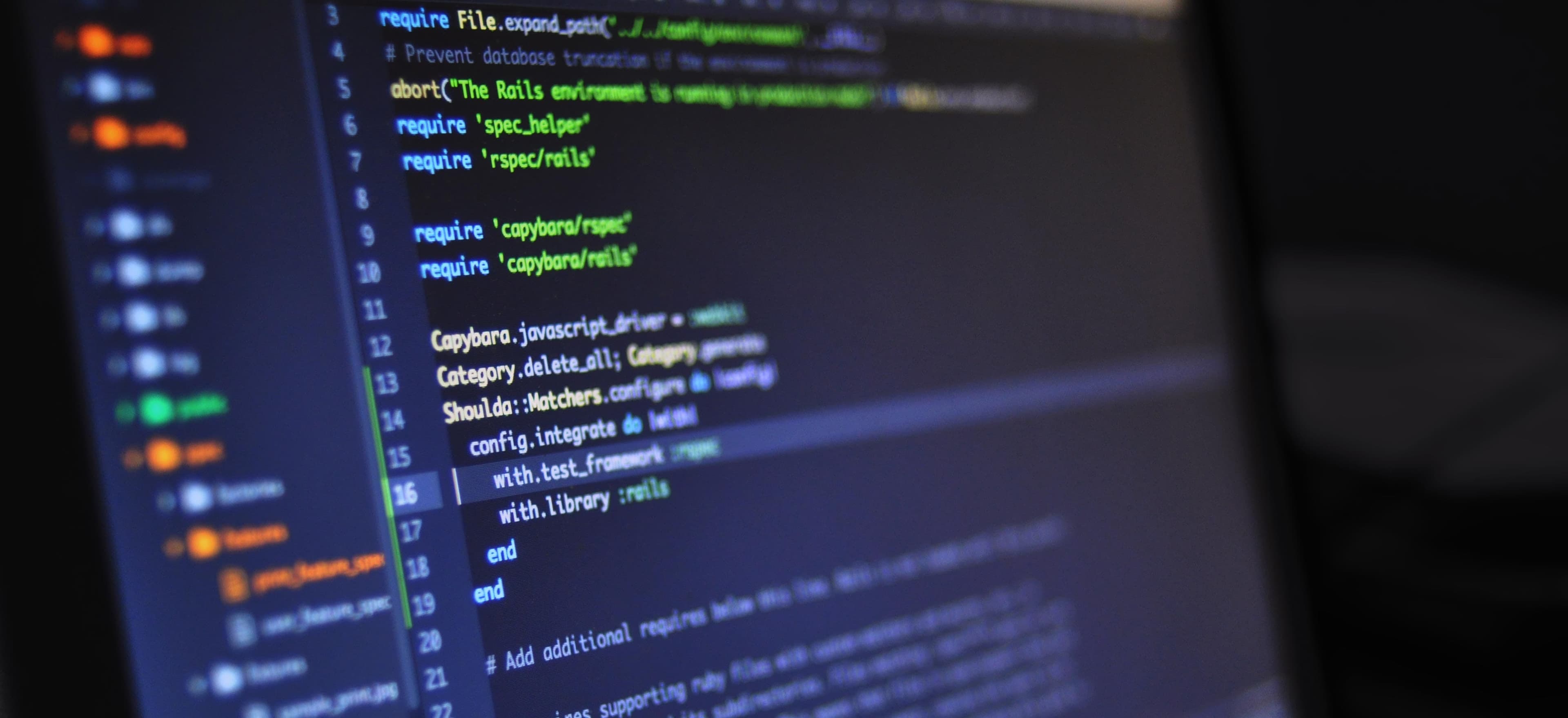
- Published on
JAXB Magic: Streamline XML Without Annotations!
If you've ever worked with XML data in Java, you're probably familiar with the Java Architecture for XML Binding (JAXB). JAXB is a powerful tool that allows for the mapping between XML documents and Java objects, making it easier to work with XML data in a Java application. However, one challenge that developers often face when using JAXB is the clutter that can come from using annotations to configure the XML mapping. In this post, we'll explore how to use JAXB without annotations and unlock the power of XML parsing and marshalling with ease.
What is JAXB?
Java Architecture for XML Binding, or JAXB, is a Java API that allows for the conversion of XML data to and from Java objects. It provides a convenient way to work with XML data in a Java application, simplifying the process of parsing and marshalling XML.
JAXB achieves this by providing a set of annotations that can be used to map XML elements and attributes to Java classes and properties. These annotations allow developers to control how XML data is interpreted and mapped to Java objects.
The Annotation Clutter
While annotations are a powerful tool for configuring JAXB, they can also lead to cluttered code. Annotations are typically added directly to Java classes and properties, and as the number and complexity of the XML mappings increase, so does the amount of annotation code required. This can make the code harder to read, understand, and maintain.
Furthermore, annotations introduce a tight coupling between the XML structure and the Java classes, making it difficult to change the XML structure without modifying the Java classes. This can be a problem when working with XML data that is subject to change or when trying to reuse existing Java code with different XML structures.
Purpose of This Post
The purpose of this post is to demonstrate how to use JAXB without annotations and showcase the benefits of such an approach. By leveraging JAXB's core components and utilizing generic JAXB elements, an ObjectFactory class, and custom adapters, we can streamline the XML handling process and achieve cleaner, more flexible code.
Pre-Requisites
Before we dive into the details of using JAXB without annotations, let's first make sure we have everything we need to get started. Here are the pre-requisites for following along:
- Java JDK version 8 or above
- JAXB API and implementation JARs (e.g.,
jaxb-api.jar
andjaxb-impl.jar
) - A build tool like Maven or Gradle configured with the appropriate dependencies
If you're using Maven, you can include the following snippet in your pom.xml
file:
<dependencies>
<dependency>
<groupId>javax.xml.bind</groupId>
<artifactId>jaxb-api</artifactId>
<version>2.3.1</version>
</dependency>
<dependency>
<groupId>com.sun.xml.bind</groupId>
<artifactId>jaxb-impl</artifactId>
<version>2.3.1</version>
</dependency>
</dependencies>
Once you have the necessary dependencies set up, we're ready to explore the basics of JAXB and learn how to use it without annotations.
Understanding the Basics of JAXB
Before we can dive into using JAXB without annotations, let's first understand the core components of JAXB and how they work together to convert Java objects to and from XML.
JAXBContext
The JAXBContext
is the entry point to JAXB functionality. It provides a way to create Marshaller
and Unmarshaller
instances that are used to marshall (convert Java objects to XML) and unmarshall (convert XML to Java objects) data.
Creating a JAXBContext
without annotations is straightforward. Here's an example:
JAXBContext context = JAXBContext.newInstance("com.example");
In this example, the JAXBContext
is instantiated with the package name containing the Java classes to be used for XML data handling. This creates a context that is aware of the classes within the specified package.
Marshaller
The Marshaller
is responsible for converting Java objects to XML. It provides methods for configuring the marshalling process, such as setting properties and adapters, and an entry point for initiating the marshalling process.
To configure the Marshaller
, you can use the following code snippet as a reference:
Marshaller marshaller = context.createMarshaller();
marshaller.setProperty(Marshaller.JAXB_FORMATTED_OUTPUT, true);
In this example, marshaller.setProperty(Marshaller.JAXB_FORMATTED_OUTPUT, true)
is used to specify that the marshalled XML should be formatted with indentation to improve readability.
Unmarshaller
The Unmarshaller
is responsible for converting XML to Java objects. It provides methods for configuring the unmarshalling process and an entry point for initiating the unmarshalling process.
To create an Unmarshaller
instance, you can use the following code snippet as a reference:
Unmarshaller unmarshaller = context.createUnmarshaller();
Once you have the Unmarshaller
instance, you can use it to unmarshal XML data into Java objects.
Streamlining JAXB Without Annotations
Now that we have a good understanding of the basics of JAXB, let's explore how to use it without annotations. We'll cover three main techniques: using generic JAXB elements, utilizing an ObjectFactory
class, and implementing custom adapters.
Using Generic JAXB Elements
JAXB provides a generic JAXBElement<T>
class that can be used to represent any XML element. This class allows us to specify the type of the element at runtime, which frees us from the need to use annotations on our Java classes.
Here's a simple example to demonstrate the usage of JAXBElement
:
JAXBElement<String> element = new JAXBElement<>(
new QName("http://example.com", "myElement"),
String.class,
"Hello, World!");
In this example, we create a JAXBElement
of type String
with the local name "myElement" and the namespace "http://example.com". The third argument is the actual value of the element, in this case, "Hello, World!".
By using JAXBElement
, we can directly create XML elements without the need for annotations on our Java classes. This approach provides greater flexibility and separation between the XML structure and the Java classes.
Utilizing an ObjectFactory
Another way to use JAXB without annotations is to create an ObjectFactory
class. This class serves as a factory for creating JAXB objects and provides a convenient way to define the structure of the XML without annotations.
To create an ObjectFactory
, simply create a new Java class and add methods for creating the JAXB objects. Here's an example:
public class ObjectFactory {
public MyRootElement createMyRootElement() {
return new MyRootElement();
}
public MyChildElement createMyChildElement() {
return new MyChildElement();
}
// ... additional methods for creating other JAXB objects
}
In this example, the ObjectFactory
class defines methods for creating MyRootElement
and MyChildElement
objects. These methods can be used to create instances of the corresponding JAXB objects, which can then be used for marshalling and unmarshalling XML.
By utilizing an ObjectFactory
, we can define the structure of the XML without the need for annotations on our Java classes. This provides a cleaner separation of concerns and makes it easier to change the XML structure without modifying the Java classes.
Implementing Custom Adapters
In some cases, you may encounter XML data that does not have a straightforward mapping to Java objects. JAXB provides the XmlAdapter
class, which allows you to customize the marshalling and unmarshalling process by providing your own logic for converting between XML and Java representations.
To implement a custom adapter, you need to extend the XmlAdapter
class and override the marshal
and unmarshal
methods. Here's an example:
public class DateAdapter extends XmlAdapter<String, LocalDate> {
@Override
public String marshal(LocalDate value) {
return value.toString();
}
@Override
public LocalDate unmarshal(String value) {
return LocalDate.parse(value);
}
}
In this example, DateAdapter
is a custom adapter that converts LocalDate
objects to and from XML strings. The marshal
method is responsible for converting LocalDate
to a string representation, while the unmarshal
method is responsible for converting a string to a LocalDate
object.
By implementing custom adapters, we can handle complex XML structures and customize the mapping between XML and Java representations. This gives us greater control and flexibility when working with XML data.
Real-World Example
To solidify our understanding of using JAXB without annotations, let's walk through a real-world example. We'll set up a scenario, create the Java model, implement marshalling and unmarshalling, and test the setup.
Setting Up the Example
Consider an XML structure that represents books with titles and authors:
<books>
<book>
<title>Java Programming</title>
<author>John Smith</author>
</book>
<!-- more book elements here -->
</books>
Our goal is to create a Java model that corresponds to this XML structure without using annotations.
Creating the Java Model
To create the Java model, we'll define two classes: Book
and Books
. The Books
class will serve as the root element and contain a list of Book
objects. Here's the Java model:
public class Books {
private List<Book> books;
// constructor, getters, and setters omitted for brevity
}
public class Book {
private String title;
private String author;
// constructor, getters, and setters omitted for brevity
}
The structure of the Java classes mirrors the structure of the XML. Now, let's implement the marshalling and unmarshalling processes.
Implementing Marshalling and Unmarshalling
To marshal the Java objects to XML, we'll use the JAXBContext
, Marshaller
, and ObjectFactory
classes:
JAXBContext context = JAXBContext.newInstance(Books.class);
Marshaller marshaller = context.createMarshaller();
Books books = new Books();
books.setBooks(getBooks()); // assume getBooks() returns a list of Book objects
marshaller.marshal(objectFactory.createBooks(books), new File("books.xml"));
In this example, we create a JAXBContext
for the Books
class, instantiate a Marshaller
, and create a Books
object. We then use the ObjectFactory
to create an instance of the Books
class, passing in our Books
object. Finally, we marshal the Books
object to XML and write it to a file named "books.xml".
To unmarshal XML to Java objects, we'll use the JAXBContext
, Unmarshaller
, and ObjectFactory
classes:
Unmarshaller unmarshaller = context.createUnmarshaller();
Books books = (Books) unmarshaller.unmarshal(new File("books.xml"));
List<Book> bookList = books.getBooks(); // retrieve the list of Book objects
In this example, we create an Unmarshaller
and use it to unmarshal the XML file to a Books
object. We then retrieve the list of Book
objects from the Books
object.
Testing the Setup
To test the marshalling and unmarshalling of our Java objects, we can write a simple test case using a testing framework like JUnit:
@Test
public void testMarshallingAndUnmarshalling() throws JAXBException {
JAXBContext context = JAXBContext.newInstance(Books.class);
Marshaller marshaller = context.createMarshaller();
Unmarshaller unmarshaller = context.createUnmarshaller();
Books books = new Books();
books.setBooks(getBooks());
File xmlFile = new File("books.xml");
// Marshal to XML
marshaller.marshal(objectFactory.createBooks(books), xmlFile);
// Unmarshal XML to Java objects
Books unmarshalledBooks = (Books) unmarshaller.unmarshal(xmlFile);
List<Book> unmarshalledBookList = unmarshalledBooks.getBooks();
// Check if the original and unmarshalled lists are the same
assertEquals(books.getBooks(), unmarshalledBookList);
}
In this test, we create a Books
object with a list of Book
objects and marshal it to XML. We then unmarshal the XML back to Java objects and compare the original and unmarshalled lists to ensure they are the same.
By testing our setup, we can ensure that our marshalling and unmarshalling processes work correctly and validate the integrity of our XML handling code.
Advantages Over Annotated Approach
Using JAXB without annotations provides several advantages over the annotated approach:
- Cleaner code: Without the clutter of annotations, our Java classes are cleaner and easier to read, understand, and maintain.
- Separation of concerns: By separating the XML structure from the Java classes, we achieve better separation of concerns, making it easier to change the XML structure without modifying the Java classes.
- Flexibility: Without the tight coupling introduced by annotations, we have more flexibility in handling XML data. We can handle complex XML structures and customize the mapping between XML and Java representations using generic JAXB elements, an
ObjectFactory
class, and custom adapters.
By leveraging these advantages, we can streamline our XML handling code and simplify the process of working with XML data in Java.
Handling Complexity
While using JAXB without annotations provides a cleaner and more flexible approach to XML handling, it can still be challenging to manage more complex XML structures. In such cases, it's important to use the right tools and techniques to handle the complexity effectively.
Here are some tips to handle complexity when using JAXB without annotations:
- Break down the XML structure: Divide the XML structure into smaller, manageable parts by creating separate Java classes for each element or sub-element. This helps in keeping the code organized and makes it easier to handle complex XML structures.
- Use composition: Utilize composition to represent relationships between XML elements. Instead of embedding one class within another, create separate classes and use references or lists to represent relationships. This makes the code cleaner and provides better separation of concerns.
- Consider schema-first design: If possible, start with an XML Schema Definition (XSD) that defines the XML structure. Generate Java classes from the XSD using tools like JAXB's
xjc
command-line tool or plugins for build systems like Maven or Gradle. This provides a well-defined structure and reduces the chances of errors or inconsistencies in the XML handling code.
By applying these techniques, we can effectively handle complex XML structures and maintain clean and maintainable code.
To Wrap Things Up
In this post, we explored how to use JAXB without annotations to streamline XML handling in Java. By leveraging JAXBElement, an ObjectFactory class, and custom adapters, we can achieve cleaner, more flexible code that is easier to read, understand, and maintain.
We covered the basics of JAXB, including the JAXBContext, Marshaller, and Unmarshaller. We then delved into the main topic of using JAXB without annotations, discussing the usage of generic JAXB elements, an ObjectFactory