Java 9 ServiceLoader Woes: Overcoming Pitfalls
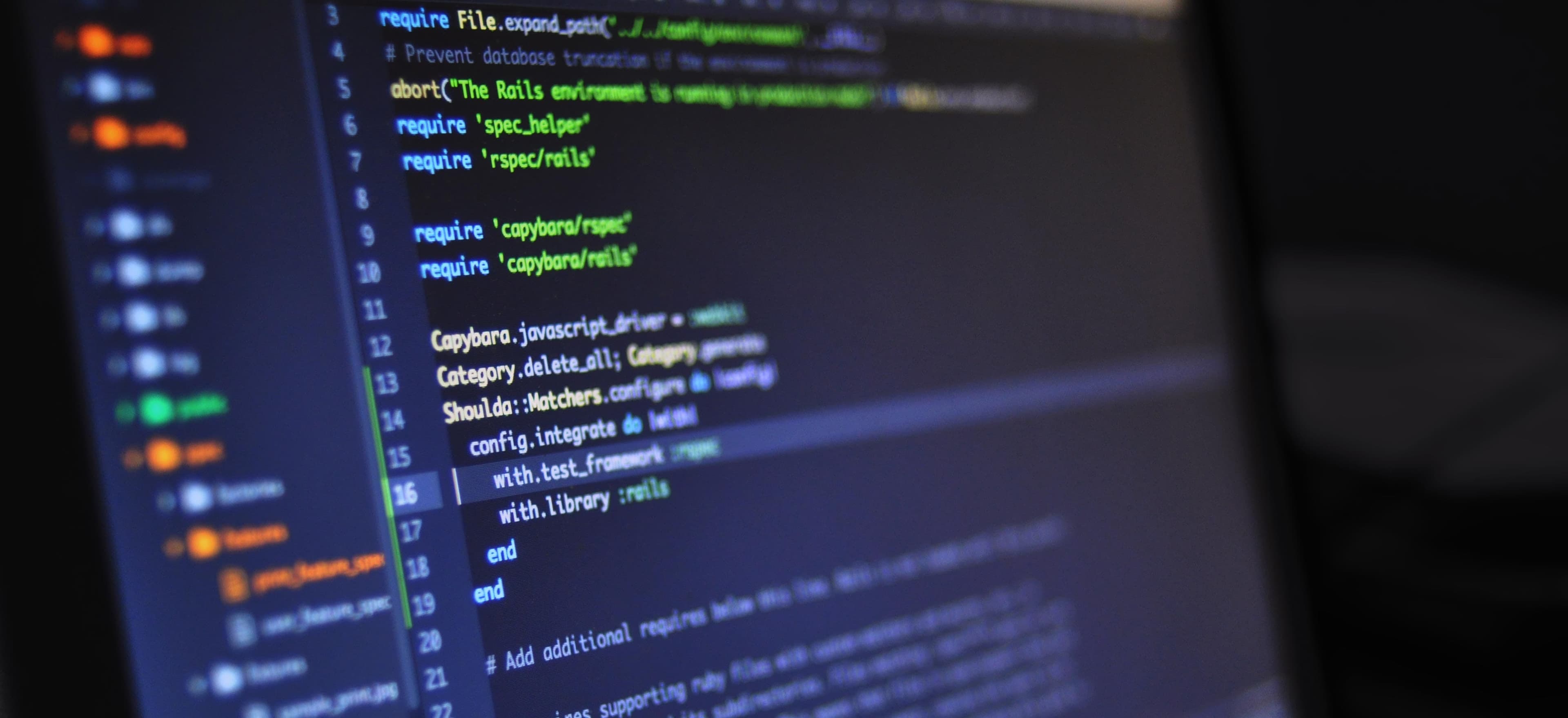
- Published on
Java 9 ServiceLoader: Overcoming Pitfalls
Java 9 brought many new features and improvements to the world of Java programming. One such feature is the enhanced ServiceLoader
utility class, which makes it easier to load services and pluggable components into your application. However, as with any new feature, there are certain pitfalls and challenges that one might encounter when using ServiceLoader
in Java 9. In this blog post, we will explore some of these pitfalls and discuss ways to overcome them.
Understanding the Basics
Before diving into the pitfalls, let's first understand the basic concept behind the ServiceLoader
class. ServiceLoader
is a utility class introduced in Java 6 that allows you to load implementations of a service interface from external modules or libraries. It follows the Service Provider Interface (SPI) design pattern, where the service interface defines the contract and the implementations provide the actual functionality.
The basic workflow of using ServiceLoader
involves four steps:
- Define a service interface that describes the contract for the functionality you want to provide.
- Implement one or more classes that implement the service interface.
- Create a file named
META-INF/services/{fully-qualified-service-interface-name}
in your module's JAR file. - Add the fully-qualified names of the implementation classes to this file.
Now, when you want to load the implementations of the service interface, you simply use the ServiceLoader
class to get an iterator over the implementations. This allows you to dynamically discover and load implementations at runtime, without the need to explicitly list them in your code.
Pitfall 1: Module System Constraints
One of the major changes introduced in Java 9 is the modular system. While the module system brings several benefits, it also introduces some constraints when using ServiceLoader
.
The first pitfall you might encounter is related to the module system's readability constraint. In Java 9, modules have designated dependency relationships, where one module can read another module. However, the module system enforces that the reads relationship is explicit and must be declared in the module declaration (module-info.java
) file.
So, if the module that defines the service interface does not explicitly read the module that contains the implementations, ServiceLoader
will fail to discover the implementations. In such cases, you will encounter a ServiceConfigurationError
.
To overcome this issue, you need to ensure that the module that defines the service interface explicitly reads the module containing the implementations. This can be done by adding a requires
statement in the module-info.java
file.
module myserviceinterface {
// ...
requires myserviceimplementation;
}
Now, when you use ServiceLoader.load()
for the service interface, Java will correctly discover and load the implementations.
Pitfall 2: Using the Wrong Class Loader
When using ServiceLoader
to load implementations, it's important to understand which class loader is being used. This is crucial when dealing with complex class-loading scenarios, such as when your application is running in a container or when you have multiple modules with their own class loaders.
The default behavior of ServiceLoader
is to use the class loader of the current module. However, this may not always be the desired behavior. For example, if your service interface and implementations are located in different modules with separate class loaders, ServiceLoader
will fail to load the implementations.
To overcome this pitfall, you can explicitly specify the class loader to use when calling ServiceLoader.load()
. This ensures that the correct class loader is used to load the implementations.
Class<?> serviceInterface = MyServiceInterface.class;
ClassLoader classLoader = MyImplementationClass.class.getClassLoader();
ServiceLoader<?> serviceLoader = ServiceLoader.load(serviceInterface, classLoader);
By providing the appropriate class loader, you can avoid class loading issues and ensure that ServiceLoader
works as expected.
Pitfall 3: Mixing Modules and Classpath
Another common pitfall is mixing modules and the classpath. In Java 9, you can have a combination of modules and classpath entries in your application. However, when using ServiceLoader
, you need to be careful about how you handle the dependencies and ensure that all required modules are properly resolved.
If you have implementations of the service interface located in modules, but your application is running with a classpath that includes JAR files containing additional implementations, ServiceLoader
will only load the implementations from the modules. The additional classpath entries will be ignored.
To overcome this pitfall, you should ensure that all required modules are included in your module path or upgrade them to modules. This ensures that ServiceLoader
can discover and load the implementations from all relevant sources.
Pitfall 4: Handling Multiple Implementations
The next pitfall is related to handling multiple implementations of the service interface. By design, ServiceLoader
returns an iterator over all the available implementations. However, if you have multiple implementations and you only need one specific implementation, you might end up iterating over all of them unnecessarily.
To address this issue, you can use the ServiceLoader
's findFirst()
method to get the first available implementation. This is particularly useful when you have multiple implementations but only need one specific implementation for your use case.
ServiceLoader<MyServiceInterface> serviceLoader = ServiceLoader.load(MyServiceInterface.class);
Optional<MyServiceInterface> firstImplementation = serviceLoader.findFirst();
if (firstImplementation.isPresent()) {
MyServiceInterface implementation = firstImplementation.get();
// Use the implementation
} else {
// Handle the case when no implementation is available
}
By using findFirst()
, you can efficiently retrieve the first available implementation without the need to iterate over all implementations.
Pitfall 5: Performance Concerns
The last pitfall to be aware of is related to the performance implications of using ServiceLoader
. While ServiceLoader
provides a convenient way to load dynamically discoverable implementations, it is not the most performant option.
When you call ServiceLoader.load()
, it searches for the implementation classes by scanning the classpath or module path. This scanning process can be time-consuming, especially if you have a large number of implementations or a complex classpath.
To mitigate the performance impact, you can consider caching the results of ServiceLoader.load()
and reusing them instead of calling it multiple times. This can significantly improve the performance, especially if the list of implementations doesn't change frequently.
ServiceLoader<MyServiceInterface> serviceLoader = ServiceLoader.load(MyServiceInterface.class);
List<MyServiceInterface> implementations = new ArrayList<>();
for (MyServiceInterface implementation : serviceLoader) {
implementations.add(implementation);
}
// Cache the list of implementations for future use
By caching the list of implementations, you eliminate the need to scan the classpath or module path every time you need to load the implementations.
Closing Remarks
Java 9's ServiceLoader
provides a powerful mechanism to load services and pluggable components dynamically. However, there are certain pitfalls and challenges that you might encounter when using ServiceLoader
. By understanding these pitfalls and following the suggested solutions, you can overcome these challenges and make the most of the ServiceLoader
utility in Java 9.
Remember to ensure module readability, use the correct class loader, avoid mixing modules and the classpath, handle multiple implementations efficiently, and consider performance implications. By doing so, you can harness the power of ServiceLoader
while avoiding common pitfalls.
So go ahead, embrace the Java 9 features, and make your application more modular and extensible with ServiceLoader
. Happy coding!
Further Reading and Resources:
- Official Java 9 documentation on ServiceLoader
- Baeldung's guide on Java 9's ServiceLoader