Maximizing Efficiency: Optimizing Connection Pool Sizes
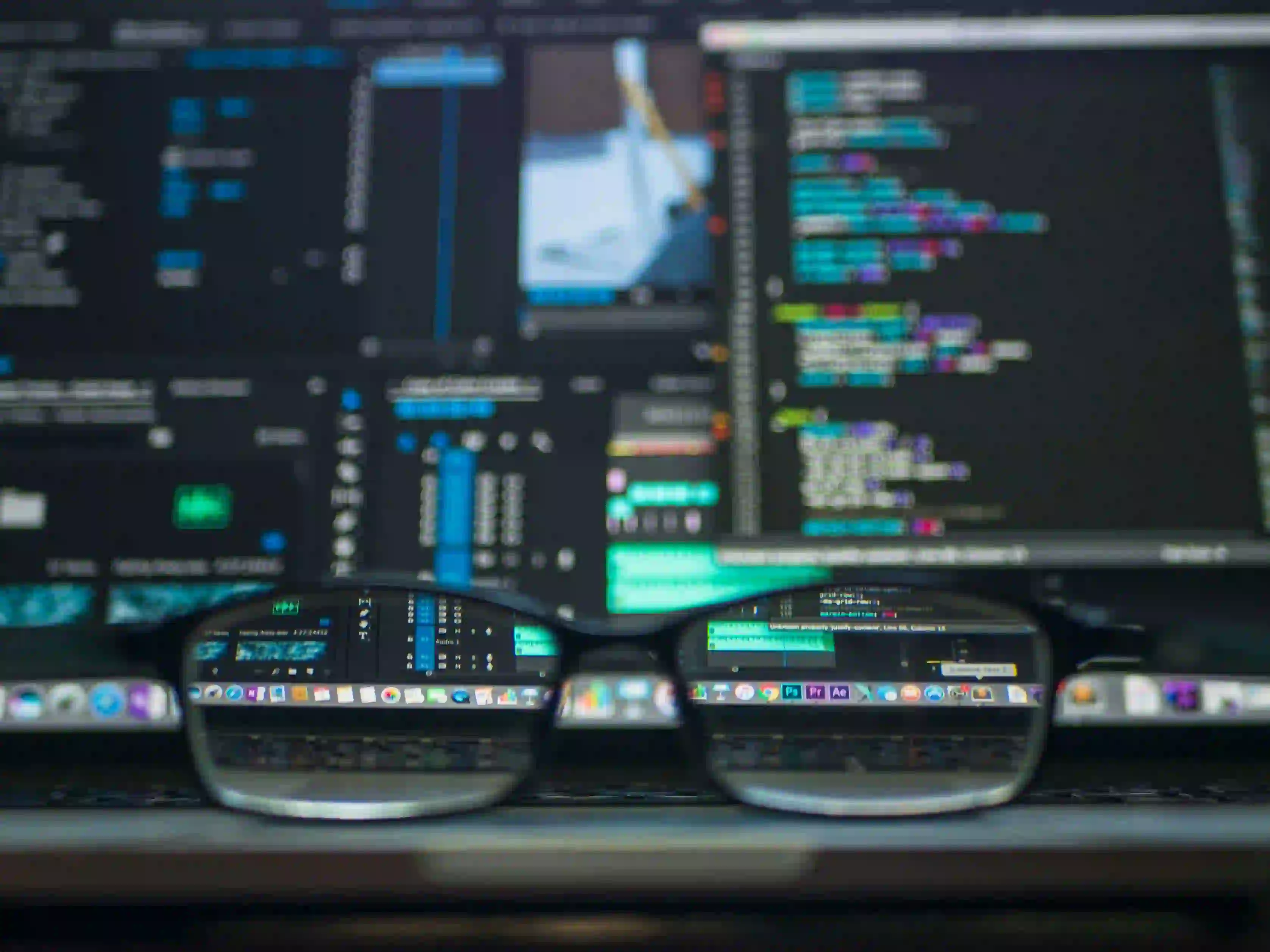
Maximizing Efficiency: Optimizing Connection Pool Sizes
In any Java application that interacts with a database, managing database connections is crucial for achieving optimal performance and scalability. One common approach for achieving this is by using connection pooling. Connection pooling allows for reusing existing database connections rather than creating a new one for every request.
Connection pooling provides numerous benefits, including reducing the overhead of establishing new connections, improving response times, and preventing performance degradation caused by connection exhaustion. However, it's essential to strike a balance between the number of connections in the pool and the application's performance.
Understanding Connection Pooling
Before we delve into connection pool optimization, let's briefly understand the concept of connection pooling. A connection pool is a cache of database connections maintained by the application server or a standalone connection pooling library.
When the application requests a connection, the pool checks if there's an idle, available connection. If so, it returns the connection from the pool. Otherwise, if the pool hasn't reached its maximum size limit, it creates a new connection and adds it to the pool. Once the application is done with a connection, it releases it back to the pool for reuse.
Determining an Optimal Pool Size
While connection pooling offers performance benefits, setting an optimal pool size requires careful consideration. Excessive connections can lead to resource wastage, whereas a small pool may cause connection pooling and performance bottlenecks.
The optimal connection pool size varies based on several factors, including:
1. Application Traffic Patterns
The number of connections needed by an application heavily depends on the application's traffic patterns. Peak load periods, expected concurrent users, and request processing times can all impact the required pool size.
Analyzing historical data and monitoring system metrics can help identify patterns and estimate connection requirements.
2. Database Server Capacity
Understanding the database server's capacity is crucial. Consider factors such as the maximum number of concurrent connections the server can handle, the available memory, and the CPU utilization. Allocating more connections than the server can handle may result in diminishing returns and unnecessary resource utilization.
3. Application Architecture
The architecture of the application plays a significant role in determining an optimal connection pool size. Applications with multiple components or microservices may require more connections to efficiently handle parallel requests.
4. Network Latency
If the application communicates with a remote database server with high latency, it's wise to increase the pool size to compensate for potential connection delays.
5. Available System Resources
Analyzing the available system resources, including memory and CPU, is crucial for determining an optimal connection pool size. Oversaturating the system resources with connections can lead to degraded performance and errors.
Benchmarking and Tuning Connection Pool Sizes
To find the optimal connection pool size, we can follow a systematic approach involving benchmarking and tuning.
Step 1: Start With a Reasonable Default
In most scenarios, starting with a conservative default pool size is a good practice. A small pool size allows monitoring and identification of performance bottlenecks without overloading the database server.
A reasonable default value to start with is often around 10-20 connections.
Step 2: Monitor Database Server Metrics
While the application is running, monitor the database server metrics such as CPU utilization, memory utilization, and disk I/O. If the server is under-utilized and there's room for more connections, gradually increase the connection pool size.
However, keep in mind that increasing the pool size indefinitely won't always lead to performance improvements. At a certain point, increasing the pool size further will have diminishing returns.
Step 3: Analyze Connection Usage Patterns
Analyze connection usage patterns by logging connection acquisition and release events. Look for connections that are consistently idle for prolonged periods. If there's a high number of idle connections, it may indicate an oversized pool.
On the other hand, if the pool frequently runs out of connections and requests have to wait for a connection to become available, it suggests an undersized pool.
Step 4: Stress Test the Application
Perform stress tests on the application by simulating a heavy load and monitoring the application's performance metrics. Track metrics such as response time, throughput, and error rates.
During stress testing, gradually increase the pool size and observe how it affects the performance metrics. This iterative approach allows finding the ideal pool size that offers the best performance under heavy load.
Step 5: Analyze the Results
Analyze the results of the stress testing and fine-tune the pool size based on the performance metrics. Look for the point where the performance metrics stabilize or show a diminishing return.
Keep in mind that optimal pool sizes can change over time as the application evolves, traffic patterns shift, or the database server capacity increases. Regularly revisit and reassess the pool size to ensure optimal performance.
Connection Pool Configuration Examples
Let's explore a few examples of configuring connection pools using popular connection pool libraries.
1. HikariCP
HikariCP is a high-performance, JDBC connection pool library known for its low latency and efficient resource management. To configure HikariCP, we can specify various attributes, including minimum and maximum pool sizes:
HikariConfig config = new HikariConfig();
config.setMaximumPoolSize(20);
config.setMinimumIdle(10);
config.setConnectionTimeout(30000);
config.setIdleTimeout(600000);
config.setJdbcUrl("jdbc:mysql://localhost:3306/mydatabase");
config.setUsername("username");
config.setPassword("password");
HikariDataSource dataSource = new HikariDataSource(config);
Here, we configure the maximum pool size to 20 and the minimum idle connections to 10. We set the connection timeout to 30 seconds and the idle timeout to 10 minutes.
2. Apache Commons DBCP
Apache Commons DBCP is another popular connection pooling library. It allows us to configure attributes like the initial size, maximum active connections, maximum idle connections, and more:
BasicDataSource dataSource = new BasicDataSource();
dataSource.setInitialSize(10);
dataSource.setMaxActive(20);
dataSource.setMaxIdle(10);
dataSource.setMinIdle(5);
dataSource.setUrl("jdbc:mysql://localhost:3306/mydatabase");
dataSource.setUsername("username");
dataSource.setPassword("password");
In this example, we set the initial pool size to 10, the maximum active connections to 20, and the maximum idle connections to 10. The minimum idle connections are set to 5.
Final Thoughts
Optimizing connection pool sizes is crucial for maximizing the efficiency and performance of Java applications interacting with databases. Finding the right balance between the pool size and the application's needs requires benchmarking, monitoring, and analyzing the system's performance metrics.
By following the systematic approach outlined in this article, you can fine-tune the connection pool size and ensure optimal utilization of system resources, improved response times, and scalable application architecture.
Remember, connection pool sizes are not set in stone. Regularly revisit and reassess the pool size as your application evolves and traffic patterns change. Making connection pooling optimization a continuous process will ensure your application remains efficient and capable of handling increasing workloads.
For further reading on connection pooling and database optimization, check out the following resources:
- connectionpool - Java Connection Pooling Library
- [Choosing a JDBC Connection Pooling Library](https://www.baeldung.com/java-connection-pooling