Fixing JAXB Generation Issues from XSD to XML
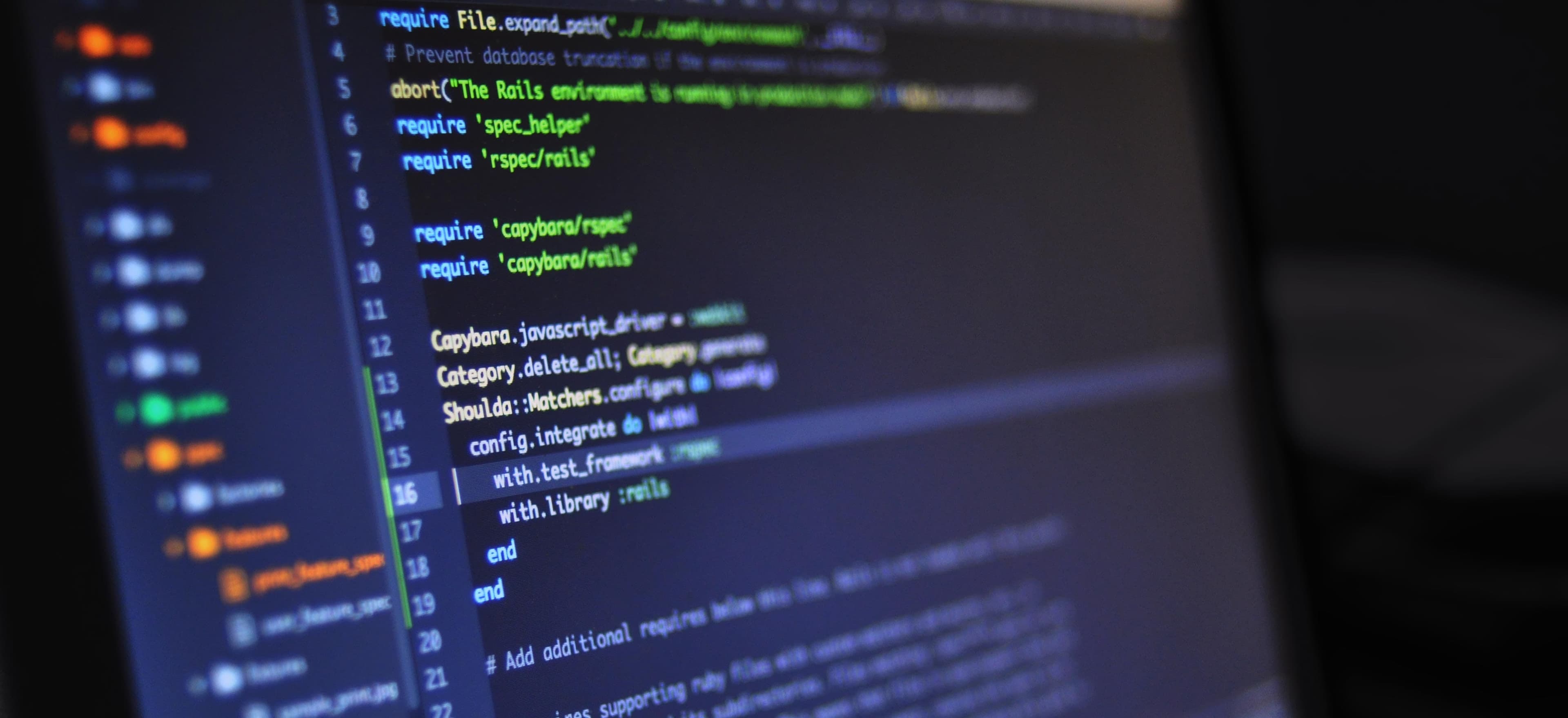
- Published on
Fixing JAXB Generation Issues from XSD to XML
If you've ever worked with XML and Java, you know the usefulness and power of JAXB (Java Architecture for XML Binding). JAXB allows you to generate Java classes from an XML schema (XSD), and then provides a way to marshal and unmarshal XML data to and from those generated classes. It's a great tool for working with XML data in a Java application.
However, there may be times when you run into issues during the generation of JAXB classes from an XSD. These issues could range from missing classes to incorrect mappings. In this blog post, we will discuss some common problems and provide solutions to fix them.
1. Missing Classes
One common issue that can occur during the JAXB generation process is missing classes. This can happen when the XSD references other XSDs that are not on the classpath. In such cases, JAXB is unable to locate the necessary classes and fails to generate the desired Java classes.
To fix this issue, you need to ensure that all the referenced XSDs are available on the classpath. You can do this by adding the necessary dependencies to your project's build file (e.g., Maven pom.xml
or Gradle build.gradle
). Once you have added the dependencies, rebuild your project, and try generating the JAXB classes again. This should resolve the missing classes issue.
2. Incorrect Package or Class Names
Another common issue that can arise is incorrect package or class names in the generated JAXB classes. This can occur when the XSD has different namespace or naming conventions than what you expect in your Java code.
To resolve this issue, you can use the @XmlRootElement
and @XmlType
annotations provided by JAXB to specify the desired package and class names for the generated classes. By adding these annotations to the XSD file, you can control the package and class names that will be generated by JAXB.
For example, suppose your XSD has a complex type named "Employee" in the namespace "http://example.com/schema". You want the generated Java class to be in the package "com.example.model" and have the class name "EmployeeModel". You can achieve this by adding annotations to the XSD as follows:
<xs:complexType name="Employee">
...
</xs:complexType>
@XmlRootElement(name = "Employee")
@XmlType(name = "EmployeeModel", namespace = "http://example.com/schema", propOrder = { "name", "age", "salary" })
public class EmployeeModel {
...
}
By using these annotations, you can customize the package and class names according to your requirements.
3. Invalid XML Schema
Sometimes, the issue lies with the XSD itself. It may contain invalid or unsupported schema definitions that JAXB is unable to process correctly. In such cases, you need to validate and fix the XSD before generating the JAXB classes.
To validate the XSD, you can use online XML validation tools or specialized XML editors that provide schema validation features. These tools will highlight any errors or warnings in the XSD, allowing you to fix them. Once you have resolved the issues, regenerate the JAXB classes, and the problem should be resolved.
4. Conflicting JAXB Annotations
JAXB provides a set of annotations that allow you to customize the generated Java classes, such as @XmlElement
, @XmlAttribute
, and @XmlRootElement
. However, using these annotations without considering their interactions can lead to conflicts and unexpected results.
One common conflict occurs when you have multiple annotations with the same name in the XSD. For example, if you have two elements with the same name but different types, JAXB will generate a single Java property with conflicting annotations.
To resolve this issue, you can use the @XmlElementWrapper
annotation provided by JAXB. This annotation allows you to introduce an additional container element to wrap the conflicting elements. By doing so, you can differentiate between the elements and avoid conflicts.
<xs:element name="item" type="xs:string"/>
<xs:element name="item" type="xs:int"/>
@XmlElementWrapper(name = "items")
@XmlElements({
@XmlElement(name = "item", type = String.class),
@XmlElement(name = "item", type = int.class)
})
private List<Object> items;
Using the @XmlElementWrapper
annotation, you wrap the conflicting elements in an additional container element called "items". This allows JAXB to generate the appropriate Java property without any conflicts.
5. Customizing Data Types
Sometimes, the default data types used by JAXB may not match your requirements. For example, JAXB may generate a java.util.Calendar
type for a datetime field, but you may prefer to use java.time.LocalDateTime
instead.
To override the default data types, you can use the @XmlJavaTypeAdapter
annotation provided by JAXB. This annotation allows you to specify a custom adapter that converts the XML representation to the desired Java type.
For example, suppose you have a datetime element in your XSD that you want to map to java.time.LocalDateTime
. You can create a custom adapter as follows:
public class LocalDateTimeAdapter extends XmlAdapter<String, LocalDateTime> {
public LocalDateTime unmarshal(String value) {
return LocalDateTime.parse(value);
}
public String marshal(LocalDateTime value) {
return value.toString();
}
}
@XmlJavaTypeAdapter(LocalDateTimeAdapter.class)
private LocalDateTime datetime;
By using the @XmlJavaTypeAdapter
annotation, you specify the custom adapter for the datetime field. JAXB will use this adapter to marshal and unmarshal the datetime values to and from java.time.LocalDateTime
.
My Closing Thoughts on the Matter
Generating JAXB classes from XSD is a powerful feature that allows you to work with XML data seamlessly in Java. However, during the generation process, you may encounter various issues such as missing classes, incorrect package or class names, invalid schema, conflicting annotations, or default data types that don't match your requirements.
By following the solutions discussed in this post, you can overcome these issues and generate the JAXB classes successfully. Just remember to ensure that all referenced XSDs are available on the classpath, customize package and class names using annotations, validate and fix the XSD if necessary, resolve conflicting annotations using @XmlElementWrapper
, and customize data types using @XmlJavaTypeAdapter
.
With these fixes in your toolkit, you'll be able to generate JAXB classes without any hassle and work with XML data effortlessly in your Java applications.
References:
- JAXB API Documentation
- Maven Central Repository