Adapting Legacy Code: Mastering the Adapter Pattern
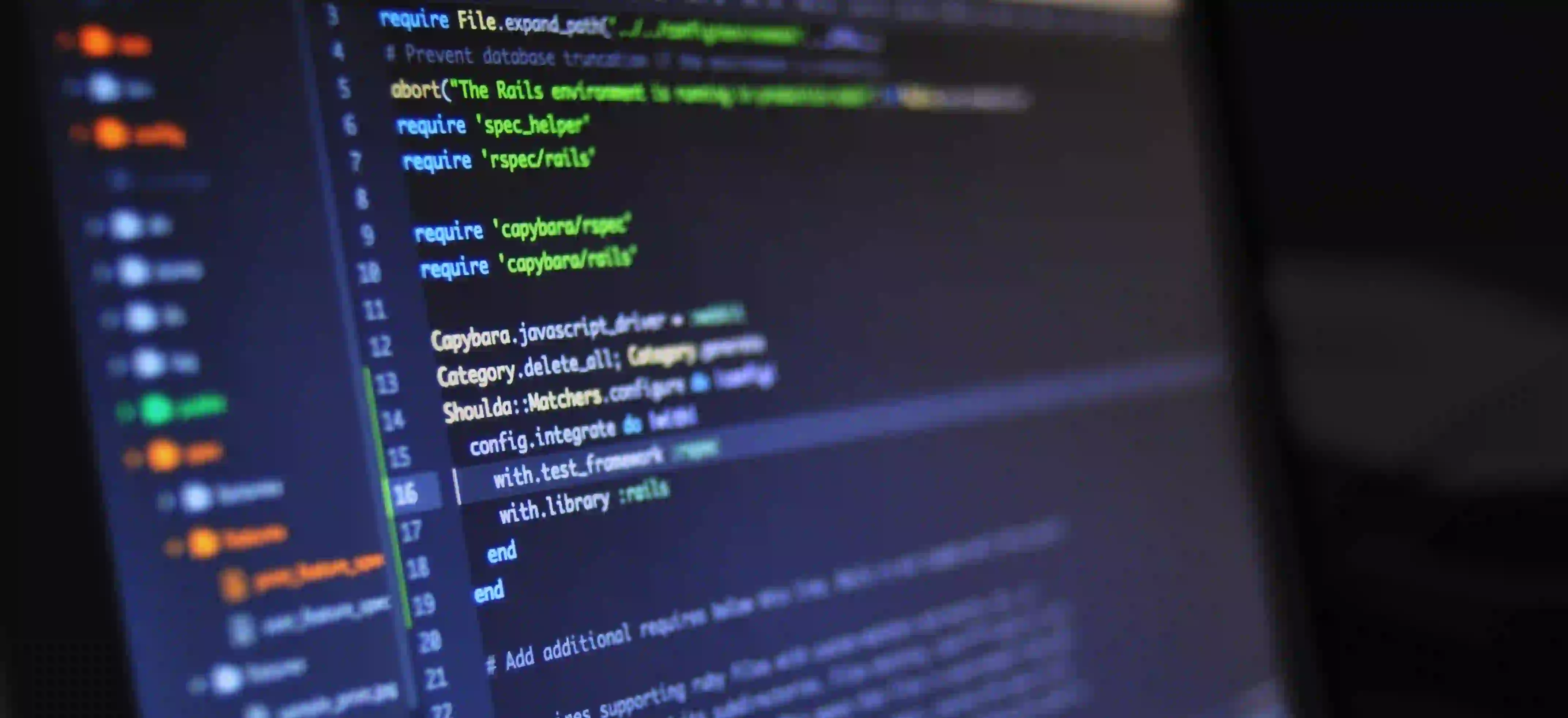
Adapting Legacy Code: Mastering the Adapter Pattern in Java
A Brief Overview
Legacy code is a common challenge in software development. It refers to outdated code that may be difficult to understand or modify due to its age or lack of documentation. When working with legacy code, integrating it with newer systems or interfaces can be a complex task. This is where the Adapter pattern comes in.
In this article, we will explore the Adapter pattern in detail and learn how it can be used to seamlessly integrate legacy code with modern systems in Java. We will also discuss the importance of design patterns in code maintainability and scalability, and how the Adapter pattern is a valuable addition to any programmer's toolkit.
The Adapter Pattern Explained
The Adapter pattern is a structural design pattern that allows incompatible interfaces to work together. It acts as a bridge between two incompatible interfaces, converting the interface of one class into another interface that clients expect. In other words, the Adapter pattern allows classes with incompatible interfaces to work together by providing a common interface.
To understand this concept better, let's consider a real-world analogy. Imagine you are traveling to a foreign country and need to charge your electronic devices. However, the electrical outlets in that country have a different shape than the plugs on your devices. In this scenario, you would need to use a power adapter. The power adapter serves as an intermediary between the different plug shapes, allowing you to connect your devices to the foreign outlets.
Similarly, in software development, the Adapter pattern serves as the power adapter, enabling the integration of incompatible interfaces. It allows us to reuse existing legacy code by adapting it to work with modern systems or interfaces.
There are two types of adapters: Object Adapter and Class Adapter.
-
Object Adapter: In the object adapter, the adapter class contains an instance of the legacy class and implements the interface required by the client. It acts as a wrapper, delegating the requests from the client to the legacy class.
-
Class Adapter: In the class adapter, the adapter class extends both the target interface and the legacy class. It inherits the functionality of the legacy class while implementing the target interface. This type of adapter requires multiple inheritance, which is not supported in Java. However, it can be achieved using multiple interfaces and delegation.
Why Use the Adapter Pattern
The Adapter pattern has several benefits that make it a valuable tool in Java development:
-
Software integration: When integrating third-party libraries or implementing APIs, the interfaces may not align perfectly with the existing codebase. The Adapter pattern allows us to bridge the gap between these interfaces and the code we are working with.
-
Code reuse: Legacy code is often valuable and robust. The Adapter pattern allows us to reuse this code by adapting it to work with newer systems or interfaces. This saves time and effort in rewriting or refactoring the existing code.
-
Simplicity: The Adapter pattern simplifies the integration process by providing a common interface. It encapsulates the complexities of working with legacy code and shields the client from unnecessary details.
In scenarios where we need to integrate legacy code with modern systems or interfaces, the Adapter pattern proves to be a useful solution. It allows us to seamlessly combine code from different sources, ensuring compatibility and maintainability in our software projects.
Real-Life Java Example: Implementing an Adapter
Let's consider a scenario where we have a legacy billing system that uses a different interface from our new payment processing system. We want to integrate the legacy billing system with our new payment processing system without modifying either system's code.
/**
* Adapter Pattern implementation in Java.
*/
class AdapterExample {
public static void main(String[] args) {
// Instantiate the legacy billing system
LegacyBillingSystem legacyBillingSystem = new LegacyBillingSystem();
// Create an adapter instance
PaymentProcessingAdapter adapter = new PaymentProcessingAdapter(legacyBillingSystem);
// Use the adapter to process a payment
adapter.processPayment("John Doe", 100.0);
}
}
/**
* Legacy Billing System with an incompatible interface.
*/
class LegacyBillingSystem {
public void performPayment(String customerName, double amount) {
// Legacy code for payment processing
}
}
/**
* Payment Processing System interface.
*/
interface PaymentProcessingSystem {
void processPayment(String customerName, double amount);
}
/**
* Adapter class that adapts the LegacyBillingSystem to the PaymentProcessingSystem interface.
*/
class PaymentProcessingAdapter implements PaymentProcessingSystem {
private LegacyBillingSystem legacyBillingSystem;
public PaymentProcessingAdapter(LegacyBillingSystem legacyBillingSystem) {
this.legacyBillingSystem = legacyBillingSystem;
}
@Override
public void processPayment(String customerName, double amount) {
// Adapt the method call to the legacy billing system
legacyBillingSystem.performPayment(customerName, amount);
}
}
In the above example, we have a LegacyBillingSystem class that represents the legacy billing system with an incompatible interface. We want to integrate it with the new PaymentProcessingSystem interface. To achieve this, we create a PaymentProcessingAdapter class that implements the PaymentProcessingSystem interface and adapts the legacy billing system by delegating the payment processing to it.
By using the adapter, we can now seamlessly process payments using the new PaymentProcessingSystem interface, even though the underlying implementation is the legacy billing system.
Let's take a closer look at each part of the code:
-
In the main method of the AdapterExample class, we create an instance of the LegacyBillingSystem and an instance of the PaymentProcessingAdapter.
-
The PaymentProcessingAdapter constructor takes an instance of the LegacyBillingSystem as a parameter and stores it in a private field. This allows the adapter to access and delegate to the legacy billing system.
-
The PaymentProcessingAdapter class implements the PaymentProcessingSystem interface and overrides the processPayment method. In the overridden method, we adapt the method call to the legacy billing system by invoking the performPayment method.
By following this approach, we can integrate legacy code with modern systems or interfaces without modifying either system's code, facilitating a seamless transition between them.
Best Practices for Implementing the Adapter Pattern in Java
When implementing the Adapter pattern in Java projects, there are some best practices to follow:
-
Naming conventions: Choose meaningful and descriptive names for your adapter classes. Use names that reflect the purpose of the adapter and make it clear what interfaces it is bridging.
-
Interfaces: Utilize interfaces in the adapter pattern to encourage loose coupling between classes. Interfaces provide a contract for how classes interact with each other, making the code more flexible and maintainable.
-
Avoid overuse: While the adapter pattern is a valuable tool, make sure to use it judiciously. Overusing the pattern can lead to unnecessary complexity in the codebase. Instead, consider refactoring or redesigning the code when appropriate.
By following these best practices, you can ensure that your adapter implementations are well-structured, readable, and maintainable.
Testing Your Adapter Implementation
Testing is crucial when working with design patterns, including the Adapter pattern, as it ensures the correctness and reliability of the code. In Java, one popular framework for writing unit tests is JUnit. Let's consider how we can write test cases for an adapter pattern implementation using JUnit.
import org.junit.jupiter.api.Assertions;
import org.junit.jupiter.api.Test;
/**
* Test cases for Adapter Pattern implementation in Java.
*/
class AdapterPatternTest {
@Test
void testPaymentAdapter() {
LegacyBillingSystem legacyBillingSystem = new LegacyBillingSystem();
PaymentProcessingAdapter adapter = new PaymentProcessingAdapter(legacyBillingSystem);
// Test the adapter by processing a payment
adapter.processPayment("Jane Smith", 200.0);
// TODO: Add assertions to verify the payment was processed correctly
Assertions.fail("TODO: Implement test assertions");
}
}
In the example above, we define a test case class called AdapterPatternTest. The testPaymentAdapter method creates an instance of the LegacyBillingSystem and the PaymentProcessingAdapter, similar to the previous example.
The test method then calls the processPayment method on the adapter and asserts some conditions to verify that the payment was processed correctly. In this example, we have left the assertions as a TODO comment for you to implement, as the specific assertions will depend on the requirements and behavior of your code.
Writing comprehensive test cases ensures that your adapter implementation is functioning as expected and provides confidence in the correctness of your code.
Lessons Learned: The Adapter as a Bridge to Modernization
In conclusion, the Adapter pattern is a powerful tool for integrating legacy code into modern systems or interfaces. It allows us to reuse valuable legacy code while seamlessly integrating it with newer technologies. By adapting incompatible interfaces, the Adapter pattern simplifies the integration process and promotes code reuse and maintainability.
In software development, design patterns like the Adapter pattern play a crucial role in achieving code maintainability and scalability. They provide reusable solutions to common problems, enabling developers to write cleaner, more robust code.
As Java developers, it is essential to understand and master design patterns like the Adapter pattern. By practicing their implementation and exploring other design patterns, we can continuously improve the quality and efficiency of our codebase.
Further Reading & Resources
- Official Java Documentation on Design Patterns
- Design Patterns: Elements of Reusable Object-Oriented Software by Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides
- Design Patterns for Humans - A beginner-friendly guide to understanding design patterns
- Stack Overflow - A community of programmers where you can find discussions and solutions related to design patterns and Java development.