Java Woes: Solving Set Duplication Glitches!
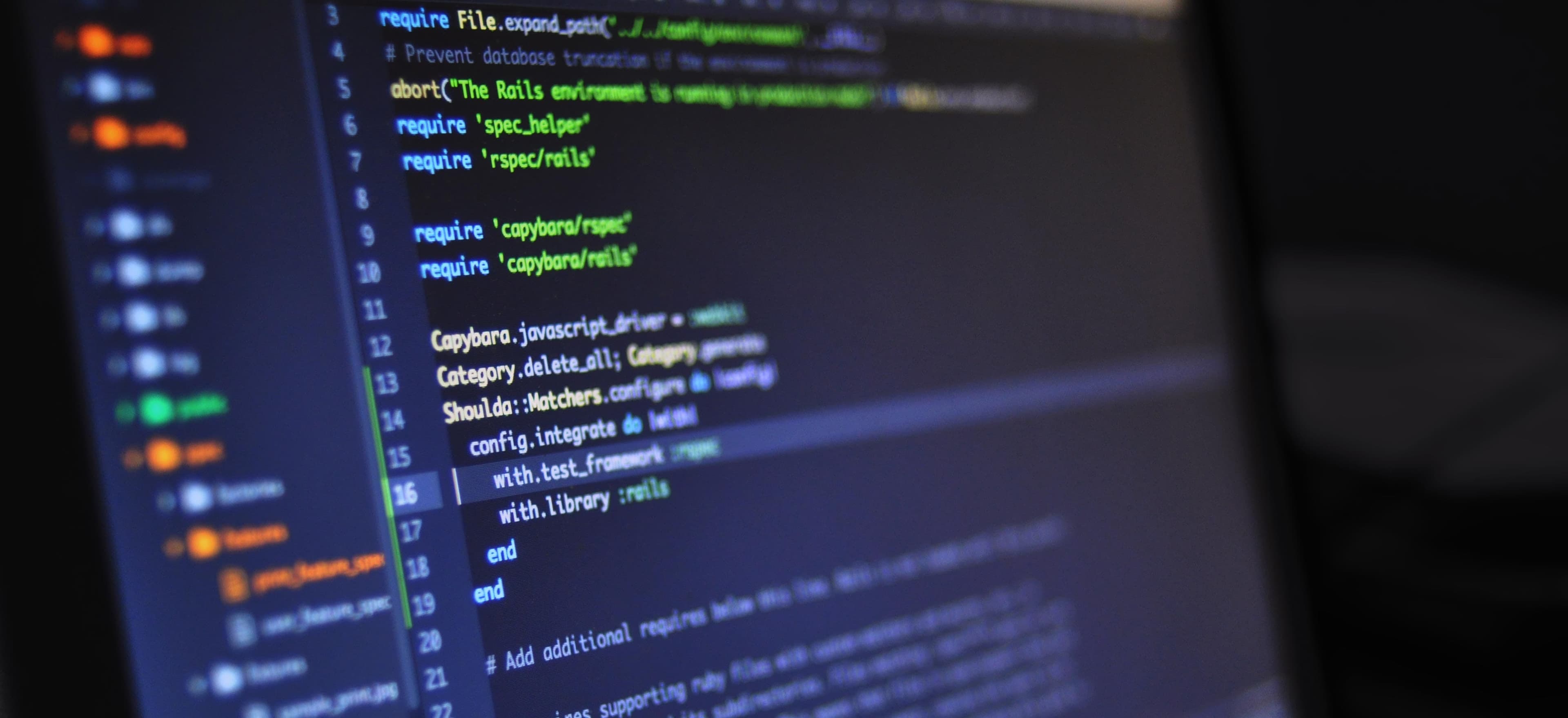
- Published on
Java Woes: Solving Set Duplication Glitches!
Dealing with data collections in Java, a common predicament programmers face is ensuring the uniqueness of elements, especially when working with custom objects. The Java Set
interface is the go-to solution when duplicates are a no-no. But what happens when you find duplicates sneaking into your Set
? Let's dive deep and exterminate these pesky duplications!
Understanding the Set Interface in Java
Before we tackle the glitches, let's recap what a Set
is. A Set
is a collection that contains no duplicate elements. It models the mathematical set abstraction and is part of the Java Collections Framework. Java offers several implementations like HashSet
, LinkedHashSet
, and TreeSet
, each with its own strengths and use-cases.
A HashSet
, for instance, stores elements in a hash table and is renowned for its speedy operations like add
, remove
, and contains
. On the other hand, a LinkedHashSet
maintains a doubly-linked list across its elements, preserving their insertion order.
Set<String> hashSet = new HashSet<>();
hashSet.add("Java");
hashSet.add("Python");
hashSet.add("Java"); // Attempting to add a duplicate
System.out.println(hashSet); // Outputs: [Python, Java]
In the above snippet, "Java" is not duplicated. Why? Because HashSet
employs the hashCode()
and equals()
methods to ascertain uniqueness.
The Duplication Glitch and Its Culprits
Now, assume you've crafted a Set
of your own objects and you're witnessing duplicates. Perplexing? Often, the glitches arise due to:
- Inconsistent
equals()
andhashCode()
: A cardinal rule in Java — if any two objects are equal according to theequals(Object)
method, they must also have the same hash code. - Mutable Fields used in
equals()/hashCode()
: Changing fields that contribute to the hash calculation after insertion into aSet
can cause chaos.
Let's investigate each issue with an example and provide a solution.
Inconsistent equals()
and hashCode()
A Java class uses the methods equals()
and hashCode()
to compare objects and determine their uniqueness respectively. Failure to override them, or doing so incorrectly, can lead to unpredictable behavior.
Consider this custom class Person
:
public class Person {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
// Standard getters and setters...
}
Now, let's add Person
instances to a HashSet
:
Set<Person> people = new HashSet<>();
people.add(new Person("Alice", 30));
people.add(new Person("Alice", 30));
System.out.println(people.size()); // Outputs: 2
Wait, didn't we add the same person twice? Not overriding equals()
and hashCode()
means each Person
instance is deemed unique despite having the same properties.
The Fix:
Override the equals()
and hashCode()
methods to ensure Person
objects with the same name and age are treated as equal.
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Person person = (Person) o;
if (age != person.age) return false;
return name != null ? name.equals(person.name) : person.name == null;
}
@Override
public int hashCode() {
int result = name != null ? name.hashCode() : 0;
result = 31 * result + age;
return result;
}
Mutable Fields in equals()/hashCode()
Suppose fields that are part of the hashCode
computation can be modified post object creation. In that case, the object's hash value can change while in a Set
, leading to duplicates when it shouldn't.
Person alice = new Person("Alice", 30);
Set<Person> people = new HashSet<>();
people.add(alice);
// Alice has a birthday
alice.setAge(31);
// Let's try adding Alice again
people.add(alice);
System.out.println(people.size()); // Outputs: 2
The mutated age
has altered the hash code, causing the Set
to misidentify the updated alice
.
The Fix:
To prevent such scenarios:
- Use immutable fields for
hashCode()
andequals()
methods. - If immutability isn't viable, avoid updating those fields post-creation or understand the implication of doing so.
- Alternatively, refrain from using such objects as keys in hash-based collections.
public final class ImmutablePerson {
// Immutable fields
private final String name;
private final int age;
// Constructor and getters...
@Override
public boolean equals(Object o) {
// Implementation as shown above
}
@Override
public int hashCode() {
// Implementation as shown above
}
}
Additional Considerations: Consistency and Performance
While we focus on eliminating duplications, remember that performance and consistency are paramount. Efficient hashCode()
implementations speed up lookups in hash-based collections. Concurrently, maintain consistency between equals()
and hashCode()
to avoid unpredictable collection behaviors.
Lessons Learned and Further Reading
To sum up, a well-implemented equals()
and hashCode()
is key to ensuring that the Set
interface in Java operates as expected. Be wary of mutable objects and uphold the contract between the two methods — it's the bedrock of Java collection behavior.
For more insights, Oracle's guidelines on equals()
and hashCode()
offer great depth (Oracle Guidelines) and the Java Collections Framework documentation (JCF Overview) provides broader knowledge of collection behaviors in Java.
Remember, with great power (of coding) comes great responsibility. Keep these tips in your arsenal, and maintain the sanctity of the Set
!