Optimizing JVM: Track Key Metrics with Groovy & JMX!
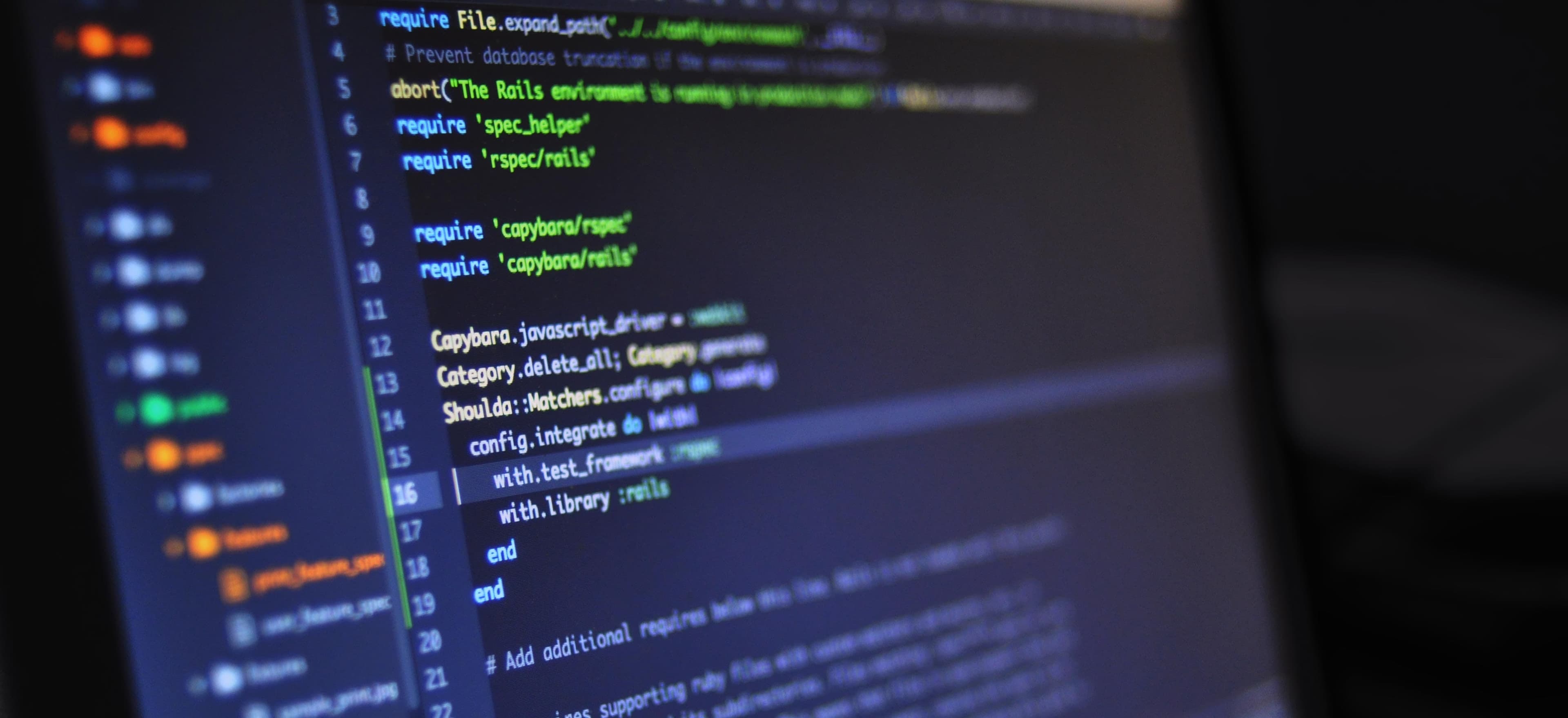
- Published on
Optimizing JVM: Track Key Metrics with Groovy & JMX!
The Java Virtual Machine (JVM) is the bedrock upon which Java applications build their performance. As your application code becomes more intricate, understanding and optimizing for JVM's many intricate tunings can mean the difference between a sleek, responsive app and a sluggish one. In this blog post, we'll dive into how you can effectively track key JVM metrics using Groovy and the Java Management Extensions (JMX), leveraging these tools to fine-tune your applications for optimal performance.
By parsing through JVM's minutiae, we can make data-driven decisions, armed with actionable insights. We prove that such an approach is not only beneficial but essential in the competitive landscape where performance often separates the leaders from the pack. Let's enhance your Java application by mastering JVM monitoring and tuning.
Understanding JMX
Before diving into the code, let’s familiarize ourselves with JMX (Java Management Extensions). JMX is a standard part of the Java Platform, which provides a simple and flexible way to monitor and manage resources like applications, devices, and service-driven networks. With JMX, developers can access performance and configuration information of Java applications within the JVM at runtime.
Why Groovy?
Apache Groovy is a powerful, optionally typed, and dynamic language that integrates seamlessly with the Java platform. It shares many Java strengths but brings additional power features inspired by languages like Python, Ruby, and Smalltalk. Groovy's scripting capabilities make it an excellent tool for writing succinct and readable scripts used for monitoring and managing Java applications. By combining Groovy with JMX, we can craft powerful scripts to keep our fingers on the pulse of JVM health and performance.
Key Metrics to Track in JVM
When analyzing JVM performance, the following are crucial metrics to track:
- Heap Memory Usage: It's the runtime data area from which memory for all class instances and arrays are allocated.
- Thread Count: The number of live threads including daemon and non-daemon threads.
- Garbage Collection: Details of the garbage collection events, including counts and time consumed.
- Class Loading: The number of classes loaded, as well as the number loaded and unloaded during application runtime.
- CPU Usage: The amount of CPU time consumed by the JVM, which directly impacts performance.
Tracking Metrics with Groovy and JMX
Now, let's look at how to set up a simple Groovy script to access these metrics through JMX.
Prerequisites:
- Install a Java Development Kit (JDK)
- Set
JAVA_HOME
environment variable - Install Groovy
- Configure Groovy in your
PATH
environment variable
Establishing JMX Connection
First, we’ll establish a connection to the JVM's MBean server using Groovy's JmxBuilder
.
import groovy.jmx.builder.JmxBuilder
JmxBuilder jmxBuilder = new JmxBuilder()
def mBeanServerConnection = jmxBuilder.connector(serverUrl: "service:jmx:rmi:///jndi/rmi://localhost:1099/jmxrmi").connect()
Here, we create a JmxBuilder
instance and establish a connection to the local JVM's MBean server. Replace "localhost:1099"
with the IP and port of your remote JVM if necessary.
Monitoring Heap Memory Usage
Heap memory is a common cause of bottlenecks; hence, monitoring is paramount.
def heapMemoryUsage = mBeanServerConnection.getAttribute(new ObjectName("java.lang:type=Memory"), "HeapMemoryUsage")
println "Heap Memory Usage: " + heapMemoryUsage
The above snippet fetches the HeapMemoryUsage
attribute, giving us a composite data structure with details about initial memory, used memory, committed memory, and maximum memory available.
Analyzing Thread Count
The number of threads in use can signal processing health or contention issues.
def threadMBean = new ObjectName("java.lang:type=Threading")
def threadCount = mBeanServerConnection.getAttribute(threadMBean, "ThreadCount")
println "Thread Count: $threadCount"
This lets you monitor thread utilization and diagnose potential deadlocks or contention situations.
Garbage Collection Metrics
Understanding the garbage collection behavior helps with memory management tuning.
def gcMBeanNames = mBeanServerConnection.queryNames(new ObjectName("java.lang:type=GarbageCollector,name=*"), null)
gcMBeanNames.each { gcMBeanName ->
def collectionCount = mBeanServerConnection.getAttribute(gcMBeanName, "CollectionCount")
def collectionTime = mBeanServerConnection.getAttribute(gcMBeanName, "CollectionTime")
println "$gcMBeanName: Collections: $collectionCount, Collection time: $collectionTime ms"
}
This code iterates through all available garbage collector MBeans and reports on their performance.
Class Loading Statistics
Dynamic class loading is central to Java's flexibility, but it's crucial to observe its behavior for any anomalies.
def classLoadingMBean = new ObjectName("java.lang:type=ClassLoading")
def loadedClassCount = mBeanServerConnection.getAttribute(classLoadingMBean, "LoadedClassCount")
def totalLoadedClassCount = mBeanServerConnection.getAttribute(classLoadingMBean, "TotalLoadedClassCount")
def unloadedClassCount = mBeanServerConnection.getAttribute(classLoadingMBean, "UnloadedClassCount")
println "Class Loading: Loaded: $loadedClassCount, Total Loaded: $totalLoadedClassCount, Unloaded: $unloadedClassCount"
This lets you keep an eye on dynamic class loading performance and patterns.
CPU Usage
Lastly, while CPU usage is not directly accessible via standard JMX beans, you can retrieve it from the OperatingSystem MBean for overall system-level metrics.
def osMBean = new ObjectName("java.lang:type=OperatingSystem")
def processCpuLoad = mBeanServerConnection.getAttribute(osMBean, "ProcessCpuLoad")
def systemCpuLoad = mBeanServerConnection.getAttribute(osMBean, "SystemCpuLoad")
println "CPU Load: Process: ${processCpuLoad * 100}% System: ${systemCpuLoad * 100}%"
This provides a rough estimate of how much CPU is being used by the JVM and how much is used by the entire system.
Lessons Learned
Regular JVM monitoring is not a luxury but a necessity for maintaining optimum application performance. Combining the flexibility of Groovy with the robustness of the JMX framework provides a compelling toolkit for JVM tuning and management, enabling developers to write concise and powerful scripts that extract valuable performance metrics.
By keeping tabs on metrics like heap memory usage, thread counts, garbage collection activity, class loading statistics, and CPU usage, you can make informed decisions about how to optimize your Java applications. These insights can lead to code adjustments, configuration tweaks, or infrastructure upgrades, all focused on enhancing your applications' performance characteristics.
Remember that optimization is an ongoing process, with the potential for continuous improvement. Tools and strategies evolve, so keeping your skills sharp and your knowledge current is essential to stay ahead in the performance game.
Looking for more in-depth discussions on JVM performance and tuning? Oracle has an expansive guide on JVM performance tuning, providing more complex scenarios and configurations. Another resource worth exploring is the Groovy documentation which will equip you with ample knowledge to further weave Groovy’s power into your Java applications.
Stay proactive and turn JVM monitoring and tuning into a strategic advantage for your Java applications. Happy performance enhancing!