Mastering Spring: One Note-Taking Secret Revealed!
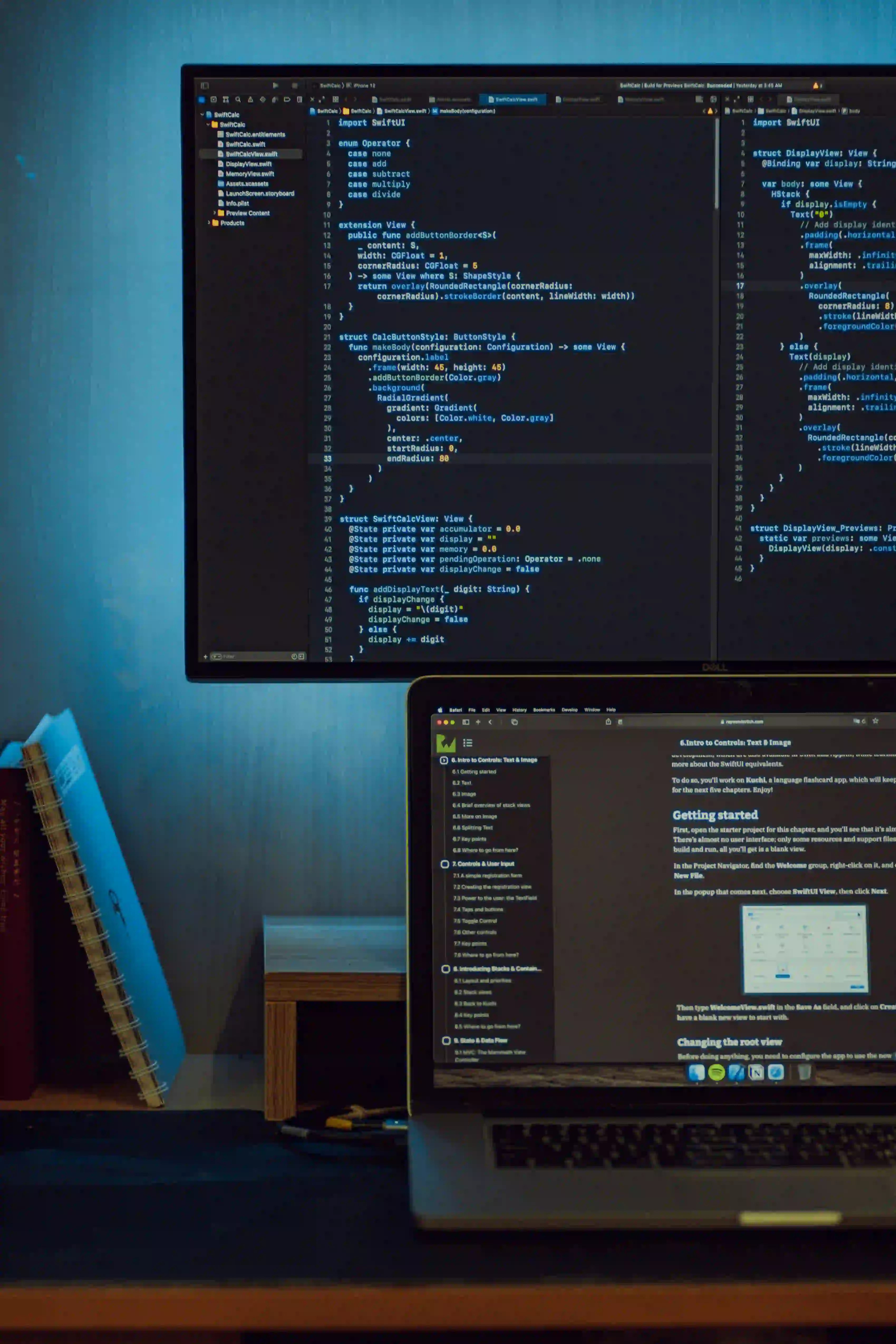
Mastering Spring: One Note-Taking Secret Revealed!
In the world of software development, efficiency is king, and organization is the queen. Tools and frameworks that bolster productivity, such as Spring Framework, reign supreme. This Java-based platform is essential for developers looking to create enterprise-level applications quickly and efficiently, but it does more than just streamline development—it also provides a means to organize and manage the data you interact with. Let's delve into a not-so-talked-about Spring feature: utilizing @Repository
annotation for creating a simple but effective note-taking application.
Why Spring for Notes?
Spring’s robust framework allows for the seamless integration of various modules, making it an excellent choice for both front-end and back-end development. Its dependency injection and aspect-oriented programming features simplify development processes, making our application scalable and maintainable. Moreover, with the use of Spring Boot, it’s possible to get an application up-and-running in minutes.
The best part? The framework's versatility means it can handle anything from complex cloud services to something as straightforward as a note-taking application.
Setting the Stage
Before deep-diving into the implementation, it's crucial to understand Spring's components. Specifically, the Repository pattern is a strategic ally in any developer's arsenal. A Repository in Spring abstracts the data layer, providing a collection-like interface to access domain objects.
Why is this important? Because it separates the business logic from database interactions, making the code cleaner and more understandable. By using @Repository
, Spring bootstraps the necessary plumbing to connect to the database, without the need to write cumbersome boilerplate code.
Now, with the Repository pattern on our side, let's orchestrate the building blocks of our note-taking application.
Project Setup
To start, you'll need to have Java and Maven installed on your machine. Create a new Maven project and include the Spring Boot Starter Data JPA and H2 database dependencies along with Spring Boot Starter Web in your pom.xml
. The H2 database is an in-memory database, which is excellent for quick development and testing purposes.
<dependencies>
<!-- Spring Boot Starter Web -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- Spring Boot Starter Data JPA -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<!-- H2 In-memory Database -->
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
</dependencies>
For a complete guide on Spring Boot dependencies, consider checking Spring Initializr here.
Crafting the Domain
Define the Note
entity that will serve as our domain model. This simple class features properties such as title, content, and timestamps.
@Entity
public class Note {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
@Column(nullable = false)
private String title;
@Column(nullable = false)
private String content;
@Temporal(TemporalType.TIMESTAMP)
private Date createdAt;
// Constructors, Getters, and Setters
}
The @Entity
annotation marks this class as a JPA entity. @Id
denotes the primary key—the unique identifier. @GeneratedValue
allows the H2 database to automatically handle the generation of unique IDs. Other annotations such as @Column
and @Temporal
provide additional details about the columns of the database.
The Data Layer
This is where we introduce our secret—the powerful @Repository
interface. Extend Spring’s JpaRepository
to gain full CRUD functionality without writing any implementation code:
@Repository
public interface NoteRepository extends JpaRepository<Note, Long> {
}
The simplicity is striking—no verbose boilerplate, no mannual SQL queries—just an interface that communicates our intent to Spring, and the framework takes care of the rest.
Exposing Functionality with Services
In-between the data layer and our controllers, we'll have services to handle business logic. Here's a straightforward NoteService
utilizing our NoteRepository
:
@Service
public class NoteService {
private final NoteRepository noteRepository;
@Autowired
public NoteService(NoteRepository noteRepository) {
this.noteRepository = noteRepository;
}
public Note saveOrUpdate(Note note) {
// Perform any business logic if required
return noteRepository.save(note);
}
// Additional methods to interact with the repository
}
The @Service
annotation helps identify this class as a service layer, which is neat for organizational purposes, also communicating to Spring where to implement its Inversion of Control (IoC).
Building the API Layer
Controllers are the front-facing component of our Spring application. Using Spring's @RestController
, we can quickly scaffold our NoteController
:
@RestController
@RequestMapping("/api/notes")
public class NoteController {
private final NoteService noteService;
@Autowired
public NoteController(NoteService noteService) {
this.noteService = noteService;
}
@PostMapping
public ResponseEntity<Note> createOrUpdateNote(@RequestBody Note note) {
Note savedNote = noteService.saveOrUpdate(note);
return new ResponseEntity<>(savedNote, HttpStatus.CREATED);
}
// Additional endpoints to retrieve, update, and delete notes
}
The @RestController
combines @Controller
and @ResponseBody
. This fusion makes it seamless for methods to return a ResponseEntity
that encapsulates the HTTP response: body, status, and headers. The @RequestMapping
defines the base URL for all the API endpoints defined within the controller.
The Probability of Success
The beauty of using Spring lies in its simplicity, as you can witness from the harmonious interplay between Spring components such as the @Repository
, @Service
, and @RestController
.
The path taken here is only the rudimentary foundation of what can become an elaborate note-taking application. Possibilities for expansion include:
- Implementing user authentication to secure notes.
- Incorporating note-sharing functionality.
- Supporting various note formats like Markdown or Rich Text.
- Adding tags and searching capabilities to organize and find notes quickly.
Embrace this knowledge and consider the sheer flexibility it brings to the table. With Spring, the framework isn't just about efficiency—it's about enabling rapid development with architecturally sound patterns that scale as your application grows.
From here on, it’s your creativity and command of Spring's offerings that will dictate the power of your applications. Whether for a personal project or enterprise-grade software, mastering the simplicity hidden within Spring’s complexity is your key to unlocking new levels of development proficiency.