Java Validation Woes: Ensuring Data Integrity!
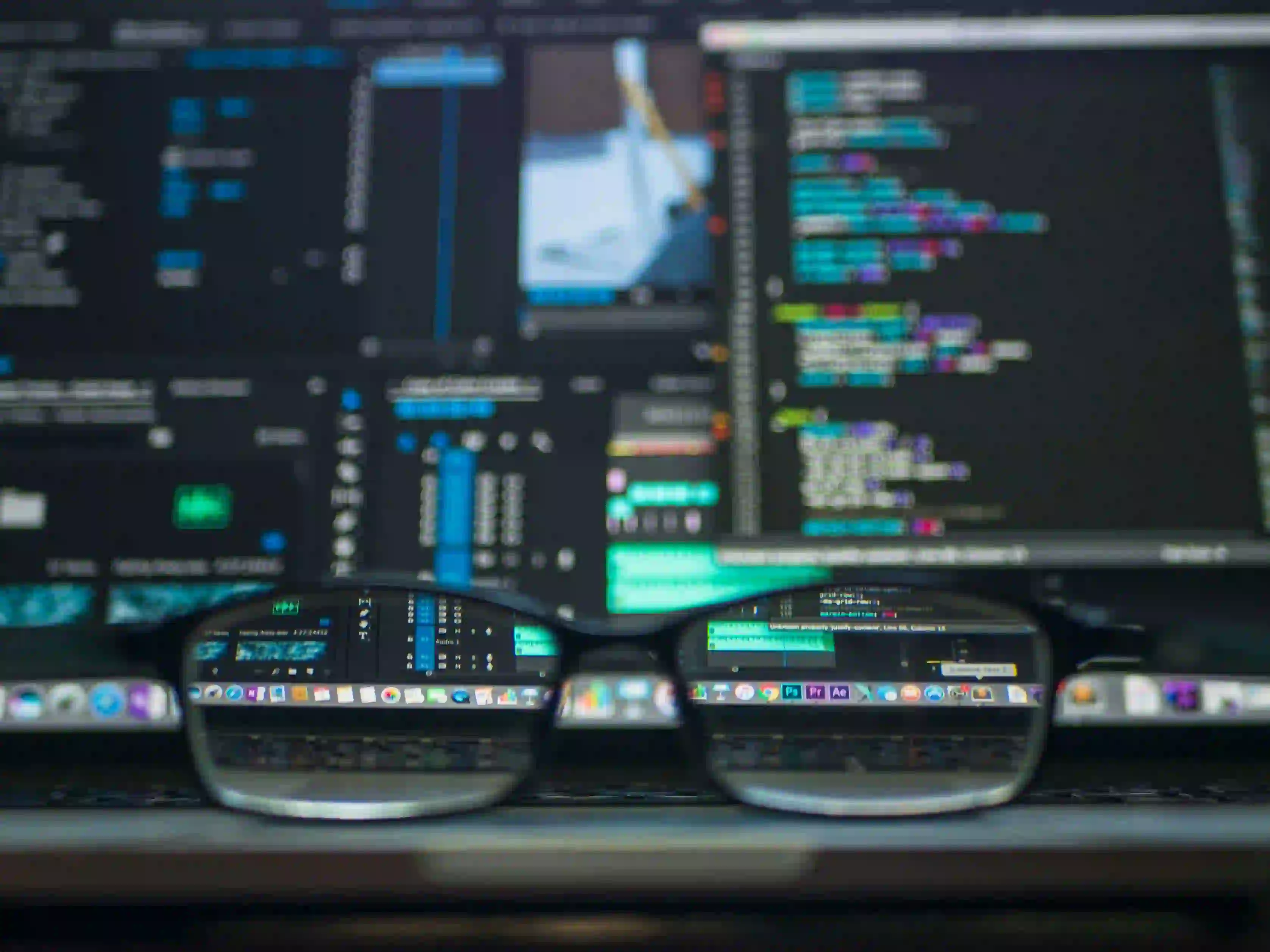
Java Validation Woes: Ensuring Data Integrity!
In the world of software development, ensuring the integrity and validity of data is paramount. As the backbone of all logical operations, data that isn’t properly vetted can lead to a plethora of bugs, security holes, and system crashes. When it comes to Java, a beloved programming language that's widely used in enterprise environments, data validation is often considered a necessary evil—a tedious, yet crucial, aspect of the coding process.
Luckily, Java developers don’t need to reinvent the wheel. In this blog post, we’ll dive into Java frameworks and built-in features that help maintain our data's integrity effortlessly, along with best practices to keep your validation logic both robust and maintainable. Mastering these tools and techniques is essential for writing secure, efficient, and reliable Java code.
Why Validate?
Data validation is the process of verifying that the data being entered into a system meets a set of predefined criteria. It's not just about preventing obvious errors but also about defending the system against malicious input that might exploit vulnerabilities in your code.
Before we plunge into Java-specific tools, let’s crystalize why skipping data validation can be catastrophic:
- Security Risks: Unvalidated input can lead to SQL injections, and cross-site scripting attacks, among others.
- Data Corruption: Without checks, bad data can pollute your database, leading to inaccurate reports, and broken business logic.
- System Instability: Unexpected data types or formats can cause exceptions and crashes.
Enter Bean Validation API (JSR 380)
Java offers a robust, annotation-based validation framework called Bean Validation API. This framework lets you declare your validation logic using annotations directly in your domain models.
Why is this approach supreme?
- Declarative: Validations are expressed through annotations, leading to cleaner and more readable code.
- Reusable: The same validation logic can be used across different layers (e.g., web, service, persistence).
- Extensible: You can create custom validators if the built-in constraints don’t fit the bill.
Here's an introductory example:
import javax.validation.constraints.*;
public class User {
@NotNull(message = "Username cannot be null")
@Size(min = 5, max = 15, message = "Username must be between 5 and 15 characters")
private String username;
@Email(message = "Email should be valid")
private String email;
// Standard getters and setters
}
In the snippet above, we ensure that the username
is not null and that it conforms to a specific size. The email is also checked to be a well-formed email address. When an instance of User
is submitted, these annotations are processed, and any violation results in a descriptive error message.
To activate validation, you would typically use a Validator
:
import javax.validation.*;
import java.util.Set;
public class ValidationExample {
private static Validator validator;
static {
ValidatorFactory factory = Validation.buildDefaultValidatorFactory();
validator = factory.getValidator();
}
public static void main(String[] args) {
User user = new User();
user.setUsername("Jo");
user.setEmail("john.doe@example.com");
Set<ConstraintViolation<User>> violations = validator.validate(user);
for (ConstraintViolation<User> violation : violations) {
System.out.println(violation.getMessage());
}
}
}
The validator.validate(user)
method checks our User
instance against the specified constraints and returns a set of violations which we can then iterate over and process accordingly.
Hibernate Validator: A Bean Validation API Implementation
Although the Bean Validation API is just a specification, there's a widely-used implementation known as Hibernate Validator. It’s fast, reliable, and provides additional features on top of the standard constraint annotations.
Use Case: Method Validation
Beyond validating object fields, Hibernate Validator allows you to validate method parameters and return values. Here’s how:
import javax.validation.constraints.Min;
import javax.validation.executable.ExecutableValidator;
import java.lang.reflect.Method;
import javax.validation.*;
import java.util.Set;
public class Calculator {
public @Min(0) int multiply(@Min(1) int a, @Min(1) int b) {
return a * b;
}
public static void main(String[] args) throws NoSuchMethodException {
Calculator calculator = new Calculator();
Method method = calculator.getClass().getMethod("multiply", int.class, int.class);
Object[] parameterValues = {5, -1};
ValidatorFactory factory = Validation.buildDefaultValidatorFactory();
Validator validator = factory.getValidator();
ExecutableValidator executableValidator = validator.forExecutables();
Set<ConstraintViolation<Calculator>> violations =
executableValidator.validateParameters(
calculator,
method,
parameterValues
);
violations.forEach(violation -> System.out.println(violation.getMessage()));
}
}
In the above Calculator
example, we're ensuring that the method parameters and the return value adhere to our defined constraints: the arguments, as well as the result, must not be less than 1.
Best Practices for Data Validation
To keep your validation game strong, here are some best practices to follow:
- Centralize Validation Logic: Resist the temptation to scatter validation logic throughout the codebase.
- Validate Early, Validate Often: The earlier you catch an invalid input, the less damage it can do.
- Fail Fast: When invalid data is detected, the application should halt further processing immediately.
Key Takeaways
Data validation is non-negotiable in Java development. The Bean Validation API and Hibernate Validator are powerful allies in this quest, simplifying validations via annotations, reducing boilerplate and leaving less room for human error.
By embracing these tools and incorporating solid validation practices, Java developers can ensure that their data is secure, consistent, and as bug-free as possible—paving the way for superior software that stands the test of time.
Embrace validation. Embrace integrity. Happy coding!