Build & Deploy: Simplify Docker with Gradle in 4 Steps!
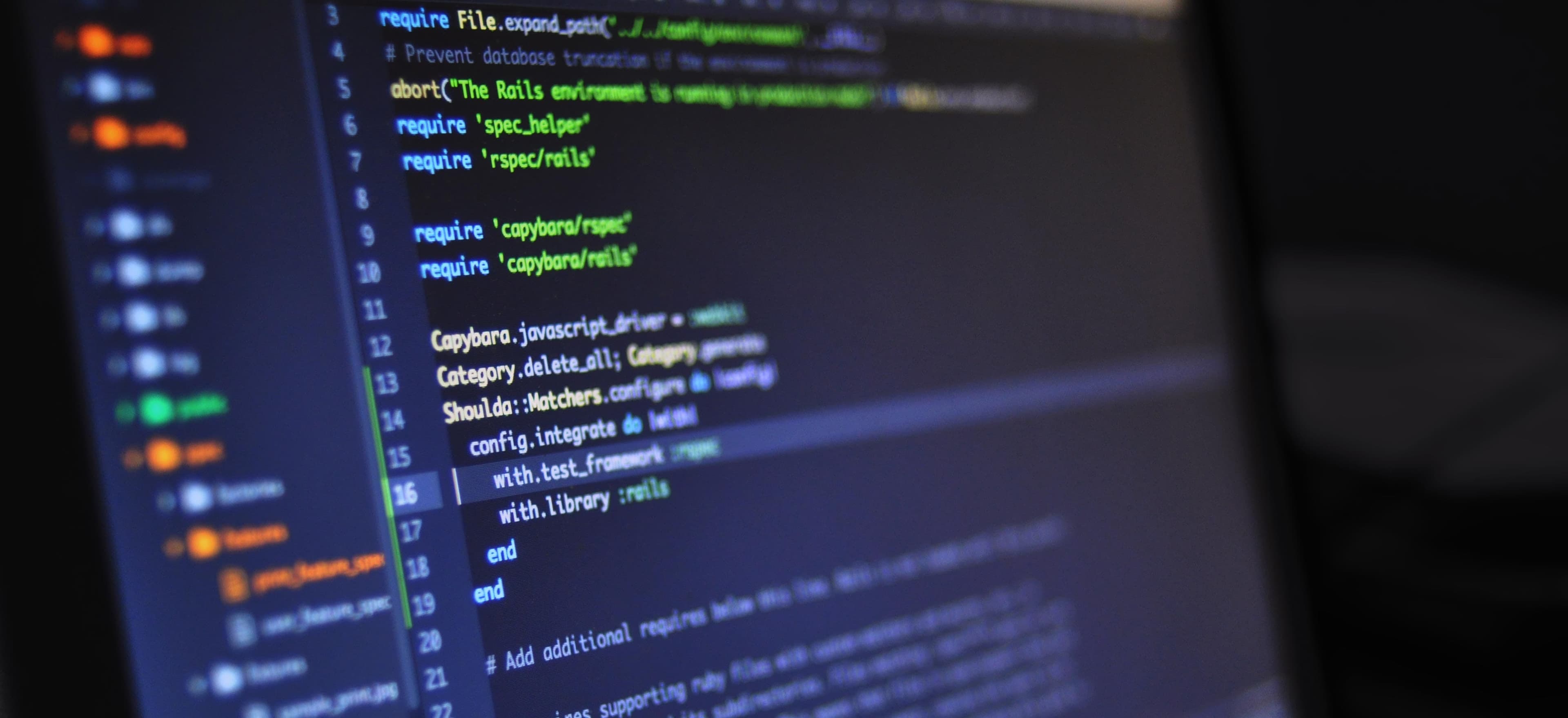
- Published on
Build & Deploy: Simplify Docker with Gradle in 4 Steps!
Docker has revolutionized the way we build, ship, and run applications. It provides a lightweight and portable environment that ensures consistency across different platforms. However, setting up Docker containers can sometimes be a complex and time-consuming task. In this blog post, we will explore how to simplify Docker containerization using Gradle, a powerful build automation tool for Java projects.
Step 1: Installing Docker
Before we dive into setting up Docker with Gradle, let's make sure Docker is installed on your machine. Docker provides installation guides for various operating systems on their official website. You can check the installation instructions for your specific OS here. Once Docker is installed, you can verify the installation by running the following command in your terminal:
docker version
If Docker is installed correctly, you should see the version details.
Step 2: Creating a Dockerfile
A Dockerfile is a text document that contains all the commands a user could call on the command line to assemble an image. It acts as a recipe for building Docker images. To create a Dockerfile, you need to define the base image, copy the necessary files, and specify any additional configurations or dependencies. Let's create a basic Dockerfile for our Java project:
# Use an official Java runtime as the base image
FROM openjdk:11-jre-slim
# Set the working directory inside the container
WORKDIR /app
# Copy the executable JAR file and any necessary resources
COPY build/libs/my-application.jar .
# Specify the command to run when the container starts
CMD ["java", "-jar", "my-application.jar"]
In this example, we are using the official OpenJDK 11 runtime as the base image. We set the working directory to /app
inside the container and copy the executable JAR file (my-application.jar
) to that directory. Lastly, we specify the command to run when the container starts, which is running the JAR file using java -jar
.
Step 3: Configuring Gradle for Docker
Now that we have our Dockerfile ready, let's configure Gradle to build and publish our Docker image as part of our build process. We can achieve this using the com.bmuschko.docker-remote-api
plugin, which integrates Docker with Gradle.
To add the plugin to your Gradle project, open your build.gradle
file and add the following code:
plugins {
id 'com.bmuschko.docker-remote-api' version '6.2.4'
}
docker {
name "${project.group}/${project.name}:${project.version}"
files dockerfiles.buildDir, file('Dockerfile')
buildArgs = [JAR_FILE: jar.archivePath]
}
This code block adds the com.bmuschko.docker-remote-api
plugin and configures the Docker image name, Dockerfile location, and build arguments. The JAR_FILE
build argument is used to specify the path of the JAR file generated by Gradle. Make sure to specify the correct JAR file path if your project structure differs.
Step 4: Building and Deploying Docker Image
With Gradle and Docker properly configured, we are ready to build and deploy our Docker image. Gradle provides a set of tasks that allows us to easily interact with Docker. Run the following command to build the Docker image:
./gradlew dockerBuildImage
This command will build the Docker image based on the Dockerfile defined earlier and the current state of your Java project. If everything goes well, you should see the Docker image being built and tagged with the specified name.
To deploy the Docker image to a Docker registry, you can run the following command:
./gradlew dockerPushImage
This command will push the built Docker image to the specified Docker registry. You need to configure the Docker registry details in your build.gradle
file. Refer to the plugin documentation for more information on configuring Docker registry.
Congratulations! You have successfully built and deployed a Docker image for your Java project using Gradle. You can now run the Docker container locally or on a remote server using the Docker image. Docker provides various commands to manage containers, such as docker run
, docker stop
, and docker logs
. You can find more information and examples in the Docker documentation.
To Wrap Things Up
In this blog post, we have explored how to simplify Docker containerization using Gradle. By following the four steps outlined in this tutorial, you can now easily build and deploy Docker images for your Java projects. Gradle simplifies the process by providing a plugin that integrates Docker seamlessly into your build process. This allows for more efficient and automated containerization, reducing the complexity and time required for setting up Docker containers.
However, it's worth mentioning that this is just an introduction to Docker with Gradle. There is a lot more you can explore, such as multi-stage builds, Docker-compose integration, and continuous integration with Docker. These topics are beyond the scope of this blog post, but you can find more information in the Docker and Gradle documentation.
Happy containerizing with Docker and Gradle!