Boost Code Reliability with Functional Programming!
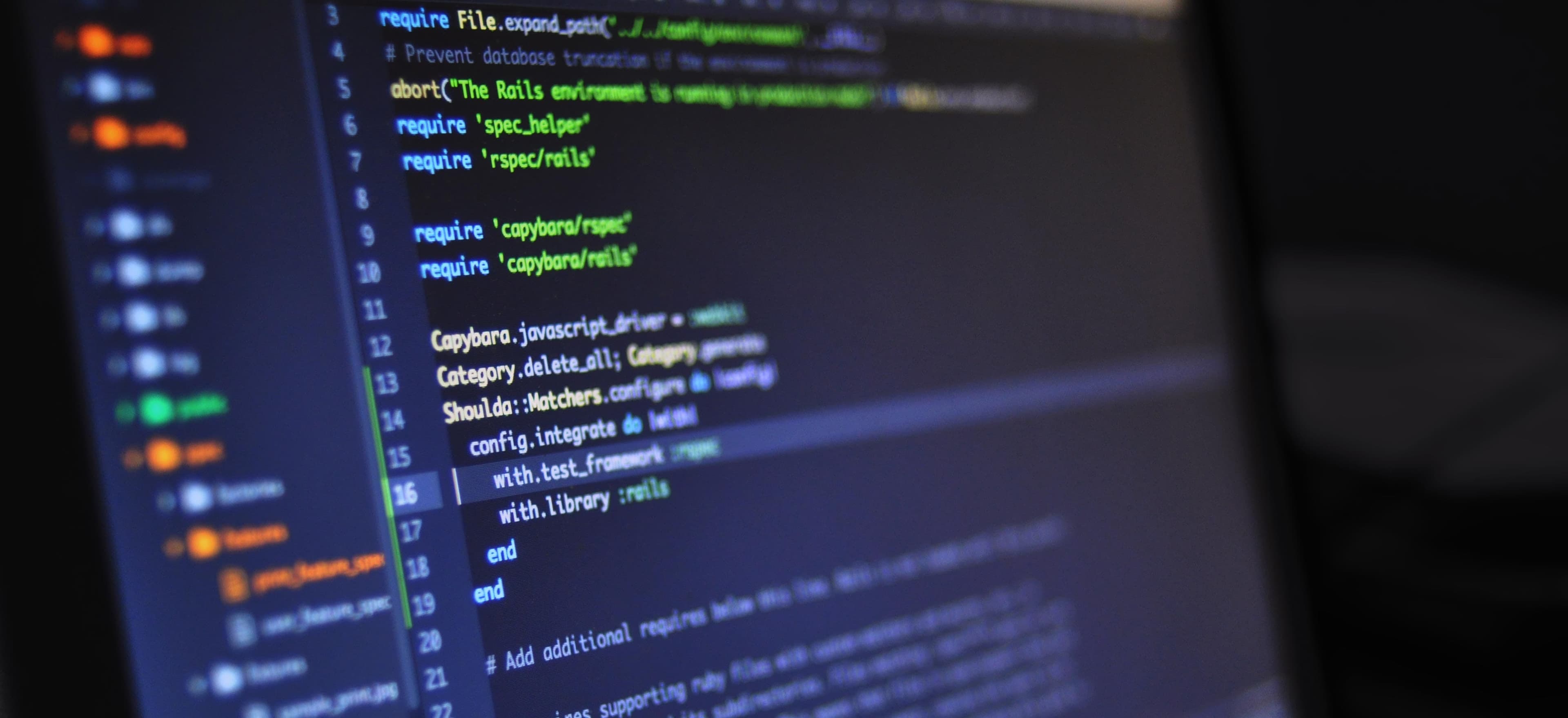
- Published on
Boost Code Reliability with Functional Programming!
In the world of software development, reliability is paramount. A reliable codebase ensures that applications run smoothly and consistently, minimizing the risk of crashes, bugs, and other undesirable outcomes. While there are various methodologies and practices that can improve code reliability, one approach that has gained significant momentum in recent years is functional programming.
Functional programming is a programming paradigm that emphasizes the use of pure functions, immutability, and declarative programming. It promotes the idea of breaking down a complex problem into smaller, reusable functions, which can be composed together to solve larger problems. This approach offers several benefits for code reliability.
1. Eliminating Side Effects and Mutable State
One of the primary sources of bugs and unpredictable behavior in traditional imperative programming is the reliance on mutable state and side effects. Mutable state makes it difficult to reason about code because a variable's value can change at any point in the program's execution. Side effects, such as modifying external variables or performing I/O operations, can introduce invisible dependencies and make code harder to test and debug.
Functional programming mitigates these issues by encouraging the use of immutable data and pure functions. Immutable data cannot be changed once it is created, ensuring that values remain constant throughout the execution. Pure functions, by definition, have no side effects and rely exclusively on their input parameters to produce output. This combination allows for deterministic behavior, making code easier to reason about, test, and debug.
Consider the following example in Java:
// Traditional imperative approach
int counter = 0;
void incrementCounter() {
counter++;
}
// Functional approach
int increment(int value) {
return value + 1;
}
In the imperative approach, the counter
variable is mutable, and the incrementCounter
function modifies its value directly. As a result, the behavior of the code can be unpredictable, especially in concurrent environments. In contrast, the functional approach ensures that the increment
function always returns a new value without modifying any external state.
2. Immutability for Predictable Code
In addition to eliminating side effects, immutability also contributes to more predictable code. With immutable data, once a value is assigned, it cannot be changed. This property provides guarantees that the value will remain constant regardless of the transformations applied to it. This predictability makes it easier to reason about the behavior of the code and ensures that unexpected changes do not occur.
For instance, let's consider a scenario where we have a list of integers that we want to filter based on a condition. In Java, using functional programming principles, we can leverage the Stream
API to achieve this:
List<Integer> numbers = List.of(1, 2, 3, 4, 5);
List<Integer> evenNumbers = numbers.stream()
.filter(n -> n % 2 == 0)
.collect(Collectors.toList());
In this example, the numbers
list remains unchanged throughout the process. Instead of modifying the original list, the filter
operation creates a new stream with the filtered elements. This approach ensures that the numbers
list remains immutable, which facilitates code reasoning and prevents unintentional modifications.
3. Higher-Order Functions and Function Composition
Functional programming encourages the use of higher-order functions, which are functions that can accept other functions as arguments or return functions as results. This feature allows for powerful abstractions and code reuse. Higher-order functions enable developers to write generic code that can handle a variety of use cases, reducing duplication and promoting code consistency.
One popular example of a higher-order function in Java is the map
function.
List<Integer> numbers = List.of(1, 2, 3, 4, 5);
List<Integer> squaredNumbers = numbers.stream()
.map(n -> n * n)
.collect(Collectors.toList());
The map
function applies a transformation to each element in a collection and returns a new collection containing the transformed elements. In this example, the lambda expression n -> n * n
specifies the transformation to be applied to each element. By utilizing higher-order functions like map
, developers can write concise and expressive code, making the intent of the code more apparent and reducing the likelihood of errors.
Another important concept in functional programming is function composition, which allows the combination of multiple functions into a single function. Function composition enhances code readability by enabling developers to express complex operations as a composition of simpler functions, making it easier to reason about the code and identify potential issues.
Function<Integer, Integer> addOne = n -> n + 1;
Function<Integer, Integer> square = n -> n * n;
Function<Integer, Integer> addOneAndSquare = addOne.andThen(square);
int result = addOneAndSquare.apply(3); // 16
In this example, we define three functions: addOne
, square
, and addOneAndSquare
. The addOneAndSquare
function is a composition of the addOne
and square
functions, where the output of addOne
is passed as input to square
. By leveraging function composition, the code becomes more readable, maintainable, and testable.
4. Enhanced Testability
Code reliability is closely tied to testability. Functional programming's emphasis on pure functions and immutability leads to code that is highly testable. Pure functions always produce the same output given the same input, making them ideal candidates for unit testing. Additionally, the absence of side effects simplifies the setup and teardown process for tests, as there are no external dependencies or state that need to be managed.
Consider the following example:
// Traditional imperative approach
public class StringUtils {
public static String reverse(String str) {
StringBuilder builder = new StringBuilder(str);
return builder.reverse().toString();
}
}
// Functional approach
public class StringUtils {
public static String reverse(String str) {
return new StringBuilder(str).reverse().toString();
}
}
In the traditional imperative approach, the reverse
method depends on mutable state (StringBuilder
), which can potentially introduce bugs or inconsistencies during testing. The functional approach, on the other hand, ensures the method is pure and side-effect-free, simplifying the testing process. It becomes easier to verify that the reverse
method produces the expected output for different inputs, increasing code reliability and reducing the likelihood of regression bugs.
Closing the Chapter
Functional programming provides valuable tools and principles to improve code reliability. By eliminating side effects and mutable state, embracing immutability, and leveraging higher-order functions and function composition, developers can create code that is easier to reason about, test, and maintain. Functional programming promotes predictable and testable code, reducing the risk of bugs and increasing the overall reliability of software systems.
As the technology landscape continues to evolve, it becomes increasingly important for developers to adapt and utilize the best practices available. Functional programming offers a solid foundation for building robust and reliable code, ensuring that applications meet the highest standards of quality and resilience.
So why not start exploring functional programming today and boost your code reliability to new heights?
For more in-depth resources on functional programming, consider checking out these links:
Happy coding!