Banish Bugs: Craft Clean Unit Tests with Patterns!
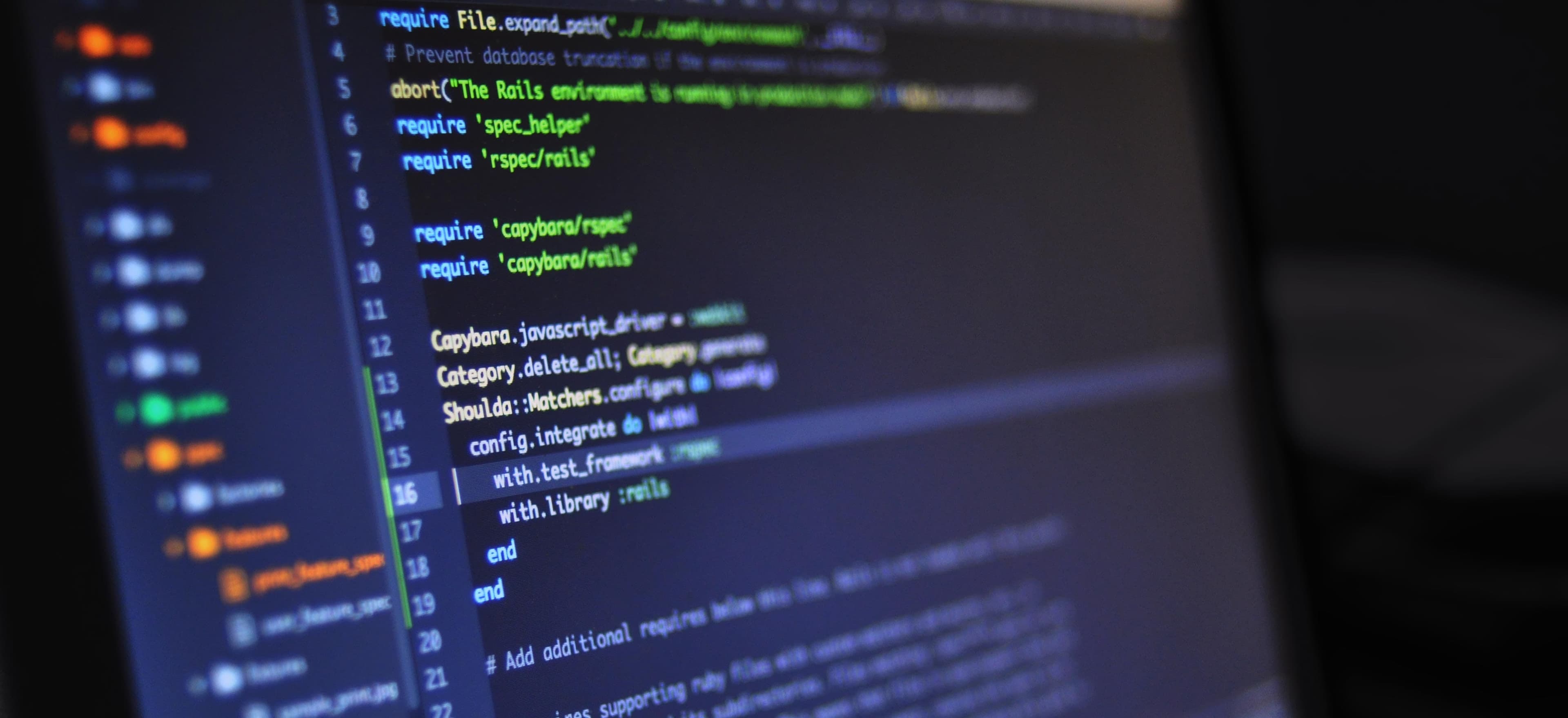
- Published on
Banish Bugs: Craft Clean Unit Tests with Patterns!
Picture this: A world where every push to production brings peace rather than palpitations, where confidence in code correctness is the norm, and chasing after bugs feels like a relic of a bygone era. This utopia is closer than you think! It's nestled within the realm of robust unit testing, an indispensable practice for Java developers serious about quality.
Today, we're diving deep into the art of crafting clean and effective unit tests that not only secure your Java code against regressions but also make your future development a breeze. So buckle up; it's time to turn unit tests from a dreaded chore into your most reliable ally.
Why Focus on Unit Testing?
In the world of software development, it's often said that code without tests is broken by design. Unit tests are the fine-tooth combs of coding—they catch the tiny, elusive bugs that evade coarser debugging techniques. By isolating individual parts of your program (the "units") and verifying their correctness, you safeguard functionality and enable smoother iterations.
Why should we care so much? Because unit tests:
- Give instant feedback on code changes.
- Act as live documentation for your code base.
- Help maintain and extend software with confidence.
- Save significant debugging and maintenance time.
Now that we've established the Why, let's pounce on the How—with Patterns!
Pattern #1: Arrange-Act-Assert (AAA)
Clear structure is the bedrock of good unit tests. Enter the Arrange-Act-Assert pattern, a pillar of test clarity.
@Test
public void givenCalculator_whenAdditionPerformed_thenCorrectResult() {
// Arrange
Calculator calculator = new Calculator();
int expected = 5;
// Act
int result = calculator.add(2, 3);
// Assert
assertEquals(expected, result);
}
Why this pattern works:
- Arrange: Set up the objects and prerequisites needed for the test.
- Act: Execute the method or feature you're testing.
- Assert: Check that the behavior or output is as expected.
Simple? Yes. Effective? Absolutely! It mirrors the thought process of a developer and keeps your tests organized and easy to read.
Pattern #2: Test Data Builders
Magic numbers and unclear object creation can muddy your tests. That's where Test Data Builders come into play, streamlining object generation into readable scripts.
@Test
public void whenBuildingEmployee_thenEmployeeHasExpectedAttributes() {
// Arrange
Employee employee = anEmployee()
.withName("John Doe")
.withRole("Developer")
.withSalary(70000)
.build();
// Act & Assert
assertAll("employee",
() -> assertEquals("John Doe", employee.getName()),
() -> assertEquals("Developer", employee.getRole()),
() -> assertEquals(70000, employee.getSalary())
);
}
Why use builders?
- Encapsulate and abstract the creation complexities.
- Make tests concise and expressive.
- Promote reuse and maintainability.
When tests read like stories, understanding and maintenance cease to be chores.
Pattern #3: Mocks and Stubs
Sometimes your unit relies on components that are complex, like database connections. Mocks and stubs let you simulate these dependencies, focusing your tests on the unit alone.
@Test
public void whenVerifyingOrder_thenRepositoryShouldBeCalled() {
// Arrange
OrderRepository mockRepository = mock(OrderRepository.class);
OrderService service = new OrderService(mockRepository);
Order order = new Order();
// Act
service.placeOrder(order);
// Assert
verify(mockRepository, times(1)).save(order);
}
Why the buzz about mocks and stubs?
- Isolate the unit from external dependencies.
- Make tests predictable and repeatable.
- Accelerate test execution.
This lets your tests zero in on what really matters—the business logic.
Pattern #4: Test-Driven Development (TDD)
TDD is not just a testing pattern—it's a whole philosophy! Write the test before the feature. Sounds backwards? It's essentially future-proofing your code.
TDD in action:
- Write a test for a non-existent feature—watch it fail (red).
- Implement the minimal amount of code to pass the test (green).
- Refine the code with confidence (refactor).
@Test
public void whenConvertingMPHtoKPH_thenSuccessfullyConverted() {
// Arrange
SpeedConverter converter = new SpeedConverter();
// Act
double kph = converter.mphToKph(10);
// Assert
assertEquals(16.09, kph, 0.01);
}
Now, build the SpeedConverter
's mphToKph
method to pass this test.
TDD benefits:
- Prioritize requirements and design.
- Ensure high test coverage from the get-go.
- Reduce bugs and promote cleaner, modular code.
A study by IBM found that TDD can result in reducing defect density by 40% to 90%. That's code quality you can measure!
Pattern #5: Continuous Integration (CI)
While not a direct unit testing pattern, CI is the highway your tests drive on. Each commit triggers an automated test suite, catching issues early and often.
The CI mantra:
- Integrate frequently.
- Automate tests.
- Keep the build green.
With tools like Jenkins, Travis CI, or GitHub Actions, Java developers can sleep easy, knowing that any commit sinning against the test suite is instantly outed.
Pattern #6: The Single Concept per Test
Each test should verify one aspect of your code, and do it well. Resist the urge to consolidate; clarity trumps conciseness in testing.
@Test
public void whenIncrementingCount_thenCountIsCorrect() {
// Arrange
Counter counter = new Counter();
// Act
counter.increment();
// Assert
assertEquals(1, counter.getCount());
}
This test wouldn't be the place to also check serialization of the Counter
class. Focus!
Tips for Sustaining Test Clarity and Effectiveness
- Name your tests descriptively.
- Keep test logic simple: If you're writing complex tests, you might be writing complex code.
- Test one thing at a time.
- Use helper methods to reduce duplication.
Clean unit tests serve as your guide through the treacherous terrain of software bugs. Employing these patterns keeps your test suite maintainable, understandable, and reliable. It's time to embrace their power and transform the testing landscape of your Java projects.
So, next time you're about to write some Java code, remember: a little structured testing goes a long way in crafting robust software. With the right patterns and practices, your tests can become the most valuable assets in your developer toolbox. Banish those bugs and happy coding!