Mastering Agile: Tackling Iterative Development Hurdles!
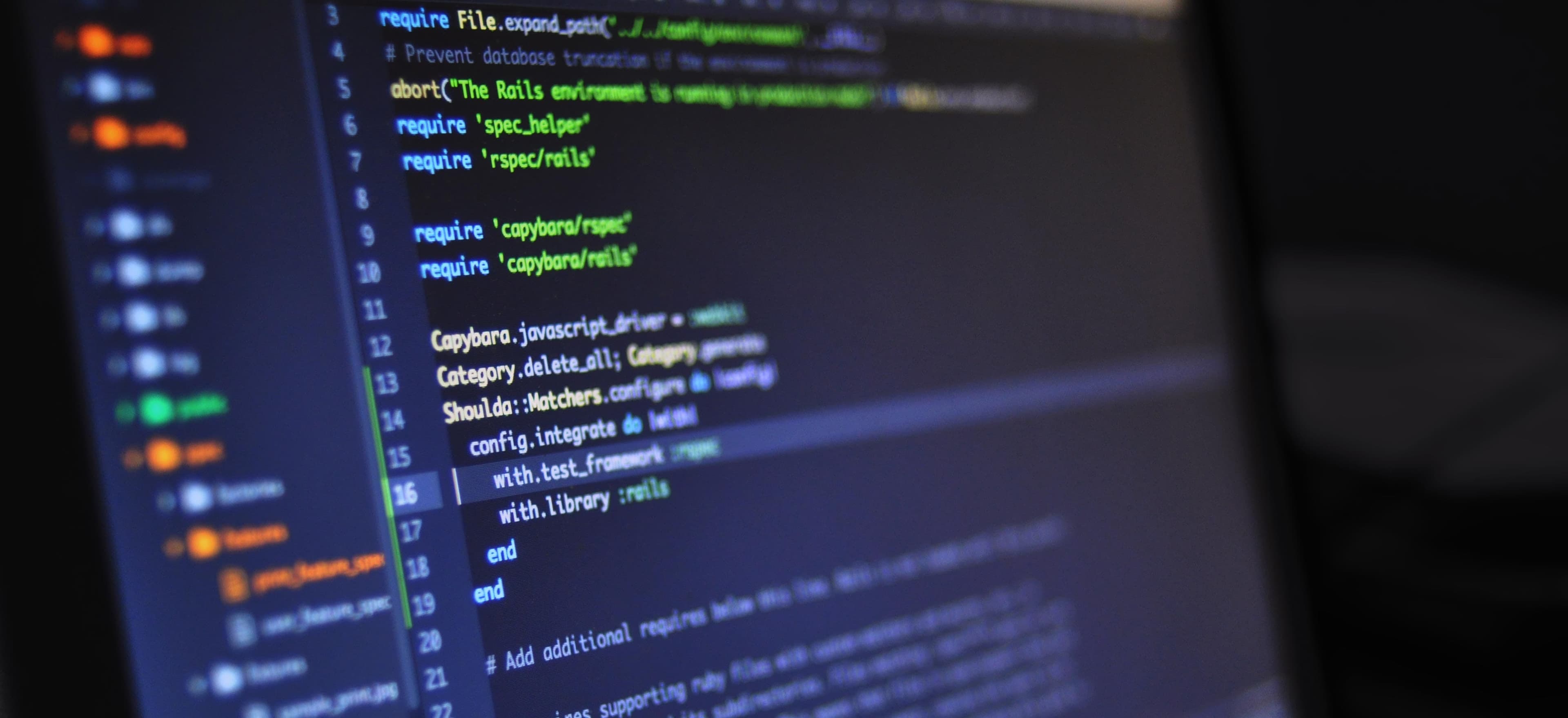
- Published on
Mastering Agile: Tackling Iterative Development Hurdles!
Software development is a dynamic field that constantly evolves with new methodologies, and Agile has emerged as a frontrunner for teams looking to streamline processes and accelerate delivery. However, even with its popularity, Agile implementation can present its own set of hurdles. In this deep dive, we'll dissect common iterative development challenges and present actionable strategies to help teams master Agile principles effectively and efficiently.
Understanding Agile Development
Agile is an iterative approach that promotes continuous iteration of development and testing throughout the software development lifecycle of a project. Unlike the Waterfall model, Agile involves multiple iterations, close collaboration, flexibility, and client involvement.
However, as straightforward as Agile may seem, it often poses unique challenges, especially for teams transitioning from more traditional methodologies. To truly master Agile, one must tackle these iterative development hurdles head-on.
Key Hurdles in Agile Development
Resistance to Change
Resistance to change is a natural human tendency, but in the Agile world, adaptability is critical. Agile's iterative nature demands frequent reassessment, which can be unsettling for teams accustomed to the "set it and forget it" philosophy.
Solution:
Consistent communication and education are vital. Encourage an open mindset by illustrating the benefits of Agile, such as quicker feedback loops and adaptable planning, which can lead to better end products.
Insufficient Planning
In their zest to begin development, teams may skimp on planning, believing the Agile framework will accommodate changes effortlessly. However, inadequate planning can result in confused priorities and aimless iterations.
Solution:
Employ practices like backlog grooming and sprint planning. These ensure that while overall flexibility is maintained, there's also a clear direction for immediate efforts.
Overlooking Technical Debt
Iterative development can sometimes lead to the accumulation of technical debt, as quick releases could mean compromises in code quality or architecture.
Solution:
Incorporate code refactoring and review as a part of your development sprints. This focuses on maintaining a healthy codebase, considering long-term impact rather than just the immediate iteration.
Java in Agile: Implementing Best Practices
As we drill down into specific implementations, let's consider Java – a language known for its robustness and versatility. Even within such a structured environment, Agile can thrive with the right approach.
Example 1: Continuous Integration
Continuous Integration (CI) exemplifies Agile principles by automating the merging and testing of code. A CI server like Jenkins can be used to build your Java applications effectively.
Why Use Jenkins with Java?
- Automated Builds: Jenkins can automatically build your Java projects after each commit, ensuring that integration issues are detected early.
- Flexible: With a multitude of plugins, Jenkins is adaptable to various development needs.
Jenkinsfile Snippet:
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'mvn clean package'
}
}
stage('Test') {
steps {
sh 'mvn test'
}
}
}
post {
success {
echo 'Build and Test Stages Successful!'
}
failure {
echo 'An error occurred, please check the logs.'
}
}
}
Commentary:
This lightweight Jenkinsfile
defines a pipeline with build and test stages for a Java project using Maven (a build automation tool). The simplicity ensures our Agile process remains adaptable, but also structured.
Example 2: Agile Testing with JUnit
Testing is an integral aspect of Agile methodology. With JUnit, Java developers have the power to write and execute tests regularly and efficiently.
Why JUnit for Agile Java Development?
- Feedback Loop: Quick testing enables a rapid feedback loop, essential for Agile iterations.
- Ease of Use: JUnit's annotations and assert mechanisms make writing and reading tests straightforward.
JUnit Test Snippet:
import static org.junit.Assert.assertEquals;
import org.junit.Test;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calculator = new Calculator();
int result = calculator.add(5, 3);
assertEquals(8, result);
}
}
Commentary:
This CalculatorTest
class highlights how simple it is to create a test in JUnit. Our focus here is on quick, repeatable tests that align with Agile's iterative nature, ensuring each function works as expected with every iteration.
Example 3: Pair Programming
Pair programming, two developers working together at one workstation, is an Agile practice that fits nicely within Java development environments.
Why Embrace Pair Programming in Java?
- Code Quality: Real-time code review leads to higher quality and fewer bugs.
- Knowledge Sharing: It fosters cross-skilling and shared ownership of the codebase.
Pair Programming in Practice:
There isn't code to demonstrate pair programming as it is a physical practice, but here’s how you could integrate it into your Java development routine:
- Session Planning: Before starting, outline the task and objectives to keep the session productive.
- Role Rotation: Regularly switch the driver (coder) and the navigator (reviewer) roles to keep both parties engaged.
- Retrospection: After the session, reflect on what went well and what could be improved.
Embracing an Agile Mindset
While integrating Agile practices with Java development or any other environment, remember that Agile is a mindset more than a rigid methodology. Be ready to adapt these strategies as needed.
Celebrate Incremental Achievements
Emphasize the importance of each sprint's success. Celebrating small wins motivates teams and highlights the iterative progress intrinsic to Agile.
Promote Collaboration and Communication
Clear, frequent communication bolsters the collaborative spirit of Agile. Make use of tools like Slack or Microsoft Teams to keep the dialogue flowing among team members.
Uphold Transparency and Reflection
Be transparent with project statuses and encourage honest retrospectives. This applies to both success and failure, enabling the team to continuously learn and improve.
A Final Look
Mastering Agile while navigating its common hurdles in Java development requires a balanced approach. Agile is not a silver bullet—it's a strategic choice that, when implemented with careful consideration for its dynamics, can significantly enhance software development processes.
By engaging with practices like CI, automated testing with JUnit, and pair programming, Java teams can maintain code quality and stay aligned with Agile's emphasis on iteration and adaptability. The journey is continuous, and every iteration brings you closer to Agile mastery. Keep learning, experimenting, and collaborating, and most importantly, keep coding with agility!