Keep Calm & Circuit Break: Mastering Hystrix in Spring
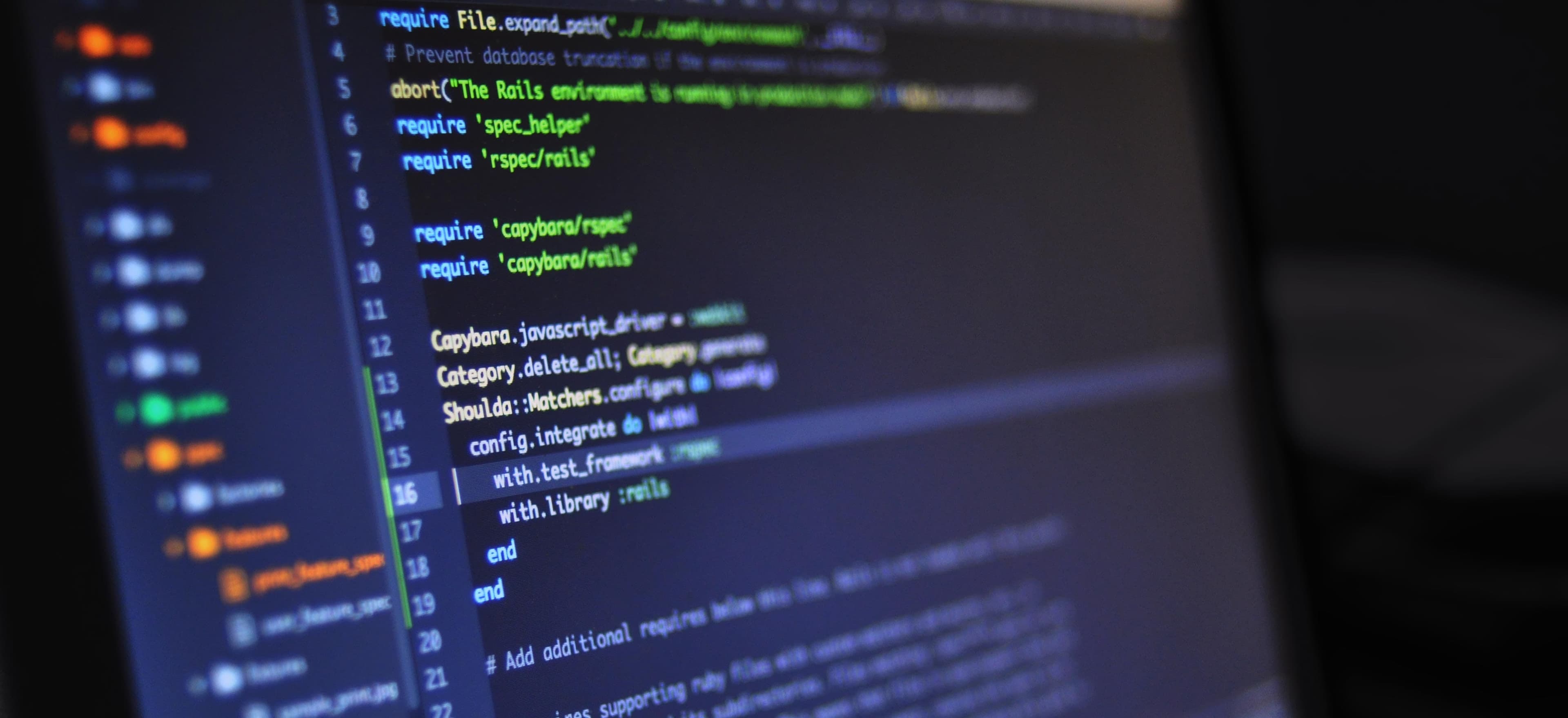
- Published on
Keep Calm & Circuit Break: Mastering Hystrix in Spring
Establishing the Context
Resilience is a critical aspect of building microservices architecture. With the increasing adoption of microservices, ensuring fault tolerance and managing dependencies has become more important than ever. In this article, we will explore Hystrix, a powerful circuit breaker framework for Java applications, specifically within the Spring ecosystem. By the end of this article, you will understand the role of circuit breakers in modern microservices architecture, the features and benefits of using Hystrix in Spring applications, and how to implement and monitor Hystrix in your projects.
The Role of Circuit Breakers in Modern Microservices Architecture
Circuit breakers are not a new concept; they have been in use in electrical systems for years. The basic idea is to protect a network or device from damage caused by overload or short circuits. In software development, the circuit breaker pattern is used to prevent cascading failures in distributed systems, where network failures are inevitable. It allows services to fail gracefully when there are issues with their dependencies.
The circuit breaker pattern works by monitoring the availability of a service and interrupting the calls to the service if it is detected to be in a failed state. This way, the failing service can be isolated, preventing further damage and providing an alternative response or fallback option. The circuit breaker pattern is an essential part of building resilient microservices architecture, ensuring that failures in one service do not affect the entire system.
Understanding Hystrix and its Importance
Hystrix was created by Netflix in 2011 to address the challenges of building fault-tolerant and resilient microservices. It is a powerful open-source Java library that provides fault tolerance and latency tolerance for distributed systems. Hystrix offers features such as fallback methods, real-time monitoring, circuit breaker behavior, and thread isolation.
One of the key advantages of using Hystrix in Spring applications is its ability to handle failures gracefully. By using fallback methods, Hystrix allows developers to provide alternative responses or perform specific actions when a service call fails. This helps in maintaining the stability of the system and avoiding cascading failures.
Hystrix also provides real-time monitoring and metrics that enable developers to gain insights into the performance and health of their microservices. With Hystrix, it becomes easier to identify bottlenecks, monitor the success rate of service calls, and take appropriate actions to ensure the resilience of the system.
Setting Up Hystrix with Spring Boot
Adding Hystrix to a Spring Boot project is straightforward and can be done using either Maven or Gradle. Here is a step-by-step guide on how to do it:
- Open your project's build configuration file (pom.xml for Maven or build.gradle for Gradle).
- Add the Hystrix dependency to your project.
<!-- Include the following snippet in Maven's pom.xml -->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-hystrix</artifactId>
</dependency>
// Include the following snippet in Gradle's build.gradle
compile('org.springframework.cloud:spring-cloud-starter-netflix-hystrix')
- Save the build configuration file.
By adding the Hystrix dependency, Spring Boot will automatically configure Hystrix for your project.
Implementing a Simple Circuit Breaker with Hystrix
To demonstrate the use of Hystrix as a circuit breaker, let's consider a common use case - calling a RESTful API that might fail. We will create a simple service method that incorporates Hystrix annotations to provide fallback behavior in case of failure.
@Service
public class MyService {
@HystrixCommand(fallbackMethod = "fallbackMethod")
public Object myServiceMethod() {
// Call the RESTful API
// Handle successful response
// Handle failure case
}
public Object fallbackMethod() {
// Provide fallback response or perform alternative actions
}
}
In the above example, we have a service method myServiceMethod()
that is annotated with @HystrixCommand
. This annotation specifies that the method should be wrapped in a Hystrix circuit breaker. If any failure occurs during the method execution, Hystrix will call the fallback method fallbackMethod()
instead.
By using the @HystrixCommand
annotation, developers can easily configure the behavior of the circuit breaker, including properties such as timeout, maximum number of concurrent requests, and the maximum threshold for error occurrences. This allows for fine-grained control over the fault tolerance and resilience of the system.
Configuring Fallback Methods with Hystrix
Fallback methods in Hystrix provide a way to handle failures gracefully and provide alternative responses or actions when a service call fails. It is crucial to configure fallback methods appropriately to ensure the stability of the system in case of failures.
Here's an example of a service method with a Hystrix fallback:
@Service
public class MyService {
@HystrixCommand(fallbackMethod = "fallbackMethod")
public Object myServiceMethod() {
// Call the service
if (response.isSuccessful()) {
return response.getBody();
} else {
throw new RuntimeException("Service call failed");
}
}
public Object fallbackMethod() {
// Provide fallback response or perform alternative actions when the service call fails
return "Fallback response";
}
}
In the above example, if the service call in myServiceMethod()
fails, the fallback method fallbackMethod()
will be invoked. The fallback method can return an alternative response or perform any necessary actions to handle the failure gracefully.
When configuring fallback methods, it is essential to consider the specific failure scenarios and design appropriate fallback behavior. For example, in case of a network timeout, the fallback might return a cached result or a default value. In the case of external service failure, the fallback might switch to an alternative service or provide a friendly error message to the user. Each fallback method should be tailored to address the specific failure scenario.
Monitoring with Hystrix Dashboard and Turbine
Hystrix provides powerful tools for monitoring circuit breakers and gaining insights into the performance of microservices. Two popular tools in the Hystrix ecosystem are Hystrix Dashboard and Turbine.
Hystrix Dashboard is a web-based tool that visualizes the metrics and health of Hystrix circuit breakers in real-time. It provides a graphical representation of the success rate, error rate, latency, and other metrics of each circuit breaker. By using Hystrix Dashboard, developers can easily identify problematic services and take appropriate actions to improve the overall resilience of the system.
To set up Hystrix Dashboard in a Spring Boot application, follow these steps:
- Add the Hystrix Dashboard dependency to your project's build configuration file (pom.xml or build.gradle).
- Create a new class
HystrixDashboardApplication
with the following annotations:
@SpringBootApplication
@EnableHystrixDashboard
public class HystrixDashboardApplication {
public static void main(String[] args) {
SpringApplication.run(HystrixDashboardApplication.class, args);
}
}
- Run the application, and the Hystrix Dashboard will be available at
http://localhost:8080/hystrix
.
Turbine is another tool that collects a stream of metrics from multiple Hystrix Dashboard instances and aggregates them into a single view. Turbine enables monitoring of the circuit breakers of multiple microservices in a single dashboard. For more advanced use cases, check out the official documentation or detailed tutorials on how to set up Turbine.
Best Practices and Common Pitfalls
When using Hystrix in Spring applications, it is essential to follow the best practices to ensure the stability and resilience of the system. Here are some best practices to consider:
- Identify critical services: Analyze your microservices architecture and identify the critical services that need circuit breaking and fallback mechanisms to maintain the overall stability of the system.
- Fine-tune Hystrix settings: Configure the Hystrix properties based on your specific needs. Tune the timeout, thread pool size, and other parameters to optimize the performance and resilience of your system.
- Implement fallback methods effectively: Design fallback methods that handle different failure scenarios appropriately. Consider caching, alternative services, and user experience when implementing fallback behavior.
- Monitor and analyze performance: Use Hystrix Dashboard and Turbine to gain insights into the performance and health of your microservices. Monitor the metrics and take appropriate actions to maintain the stability of the system.
- Test Hystrix-enabled applications: Write comprehensive integration and unit tests to ensure that the Hystrix configuration is working as expected. Test the different failure scenarios and verify that the fallback behavior is correctly implemented.
While using Hystrix, it is important to be aware of some common pitfalls and how to avoid them:
- Overusing Hystrix: Hystrix has a performance overhead, and using it for every service call might impact the overall performance of your system. Identify the critical services that need circuit breaking and avoid unnecessary use of Hystrix.
- Applying Hystrix to long-running tasks: Hystrix is not designed for managing long-running tasks; it is primarily focused on managing external dependencies. For long-running tasks, consider other frameworks or technologies designed specifically for that purpose, such as Spring Cloud Task.
- Not handling thread context propagation: When using Hystrix, ensure that the thread context (e.g., request headers, user context) is correctly propagated to the downstream services. Missing thread context can result in unexpected behavior and compromised security.
To Wrap Things Up
In this article, we have explored the role of circuit breakers in modern microservices architecture and the importance of resilience patterns such as Hystrix. We learned about the features and advantages of using Hystrix in Spring applications, and how to set up, implement, and monitor Hystrix in your projects. By following the best practices and avoiding common pitfalls, you can harness the power of Hystrix to build more resilient and fault-tolerant microservices.
Now it's time to experiment with Hystrix in your own projects. Start by adding Hystrix to your Spring Boot applications and gradually implement circuit breakers and fallback methods for critical services. Monitor the performance, fine-tune the configuration, and gain insights into the resilience of your system using Hystrix Dashboard and Turbine.
Remember, resilience is a vital aspect of building distributed systems, and Hystrix is a valuable tool in achieving that resilience. So, keep calm, circuit break, and master Hystrix in your Spring applications!