Unraveling Iterators: Solving Collection Traversal Woes
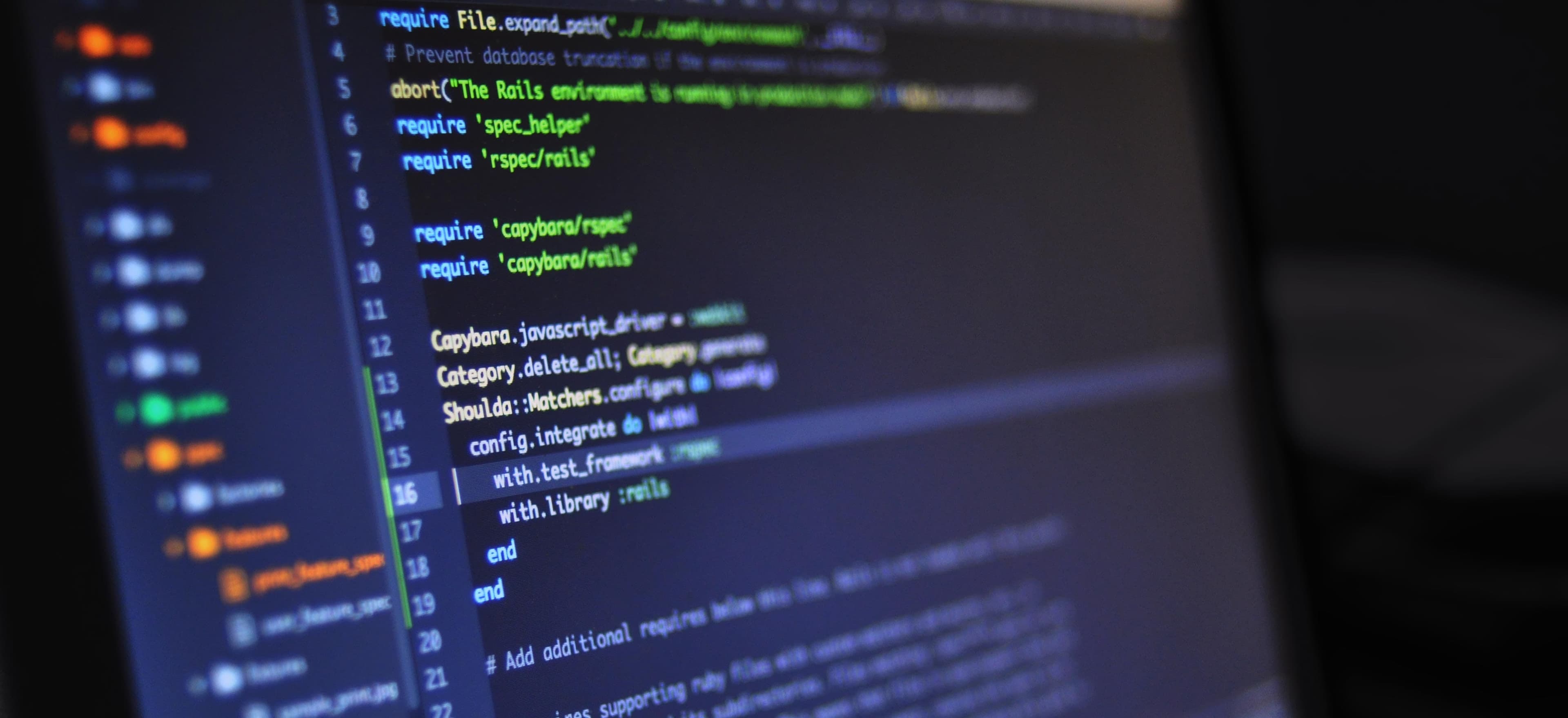
- Published on
Unraveling Iterators: Solving Collection Traversal Woes
As a Java developer, you're likely familiar with the concept of iterators, which provide a way to loop over elements in a collection. While they are powerful tools, they can also be a source of frustration when not used correctly. In this blog post, we will explore iterators in depth, discuss common pitfalls, and provide solutions to help you avoid these issues.
What is an Iterator?
An iterator is an object that allows you to traverse a collection, such as a List or Set, and perform operations on its elements. It provides methods like next()
, hasNext()
, and remove()
, which allow you to iterate over each element and perform actions as needed.
Here is an example of how you might use an iterator to loop over a List of strings and print each element:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
Iterator<String> iterator = names.iterator();
while (iterator.hasNext()) {
String name = iterator.next();
System.out.println(name);
}
The hasNext()
method checks if there is a next element in the collection, and next()
retrieves that element. This loop will output:
Alice
Bob
Charlie
Iterators are handy because they allow you to iterate over elements of a collection without exposing its internal implementation. This provides encapsulation and flexibility to change the underlying data structure without modifying your iteration code.
Common Pitfalls with Iterators
-
ConcurrentModificationException: One common issue when working with iterators is the
ConcurrentModificationException
. This occurs when you modify the underlying collection while iterating over it. Let's look at an example:List<String> names = new ArrayList<>(); names.add("Alice"); names.add("Bob"); names.add("Charlie"); for (String name : names) { if (name.equals("Bob")) { names.remove(name); // throws ConcurrentModificationException } }
In this example, we are trying to remove the element "Bob" from the list while iterating over it. However, this will throw a
ConcurrentModificationException
because the iterator detects that the collection has been modified.To avoid this issue, we should use the iterator's
remove()
method instead:Iterator<String> iterator = names.iterator(); while (iterator.hasNext()) { String name = iterator.next(); if (name.equals("Bob")) { iterator.remove(); // removes "Bob" without throwing an exception } }
By using the
remove()
method provided by the iterator, we can safely remove elements from the collection without triggering the exception. -
Nested Iteration: Another pitfall to be aware of is nested iteration. Suppose you want to iterate over a collection of lists and perform some action on each element of each list. Here's an example:
List<List<Integer>> collections = new ArrayList<>(); collections.add(Arrays.asList(1, 2, 3)); collections.add(Arrays.asList(4, 5, 6)); for (List<Integer> collection : collections) { for (Integer element : collection) { System.out.println(element); } }
While this code appears to work fine, it's not the most efficient approach. It creates a new iterator for each inner list for every outer list iteration. This can be problematic for large collections, as it incurs unnecessary overhead.
A more optimal solution is to use a single iterator for all the collections:
Iterator<List<Integer>> outerIterator = collections.iterator(); while (outerIterator.hasNext()) { List<Integer> innerCollection = outerIterator.next(); Iterator<Integer> innerIterator = innerCollection.iterator(); while (innerIterator.hasNext()) { Integer element = innerIterator.next(); System.out.println(element); } }
By using a single iterator for both the outer and inner collections, we eliminate the need for creating new iterators for every iteration.
Enhancing Iteration with Java 8
Java 8 introduced the Stream API, which provides a more concise and expressive way to iterate and manipulate collections. Streams are a sequence of elements that can be processed in parallel or sequentially.
Let's take a look at how we can use streams to solve some common iteration problems.
-
Filtering Elements: Suppose you have a list of strings, and you want to filter out the elements that start with the letter "A" and print them. Here's how you can do it using streams:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie"); names.stream() .filter(name -> name.startsWith("A")) .forEach(System.out::println);
The
filter()
method takes a predicate, which is a functional interface representing a condition. In this example, we pass a lambda expression that checks if the name starts with "A". TheforEach()
method then prints the filtered names. -
Mapping Elements: Suppose you have a list of integers, and you want to create a new list that contains the squares of each number. Using streams and the
map()
method, you can achieve this in a concise way:List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5); List<Integer> squares = numbers.stream() .map(number -> number * number) .collect(Collectors.toList()); System.out.println(squares);
The
map()
method transforms each element of the stream according to the provided lambda expression. In this case, it squares each number. Thecollect()
method then collects the transformed elements into a new list.
Closing the Chapter
Iterators are powerful tools for traversing collections in Java. However, they can also be a source of frustration if not used correctly. By understanding the common pitfalls and adopting best practices, such as using the iterator's remove()
method or leveraging the Stream API in Java 8, you can avoid many common issues associated with collection traversal.
In this blog post, we covered the basics of iterators, common pitfalls such as ConcurrentModificationException
, and how to address them. We also explored how to enhance iteration using the Stream API in Java 8. By incorporating these techniques into your Java development, you can improve the efficiency and reliability of your collection traversals.
Happy iterating!
Additional Resources